Streamlining Java Database Connectivity for Multi-Database Apps
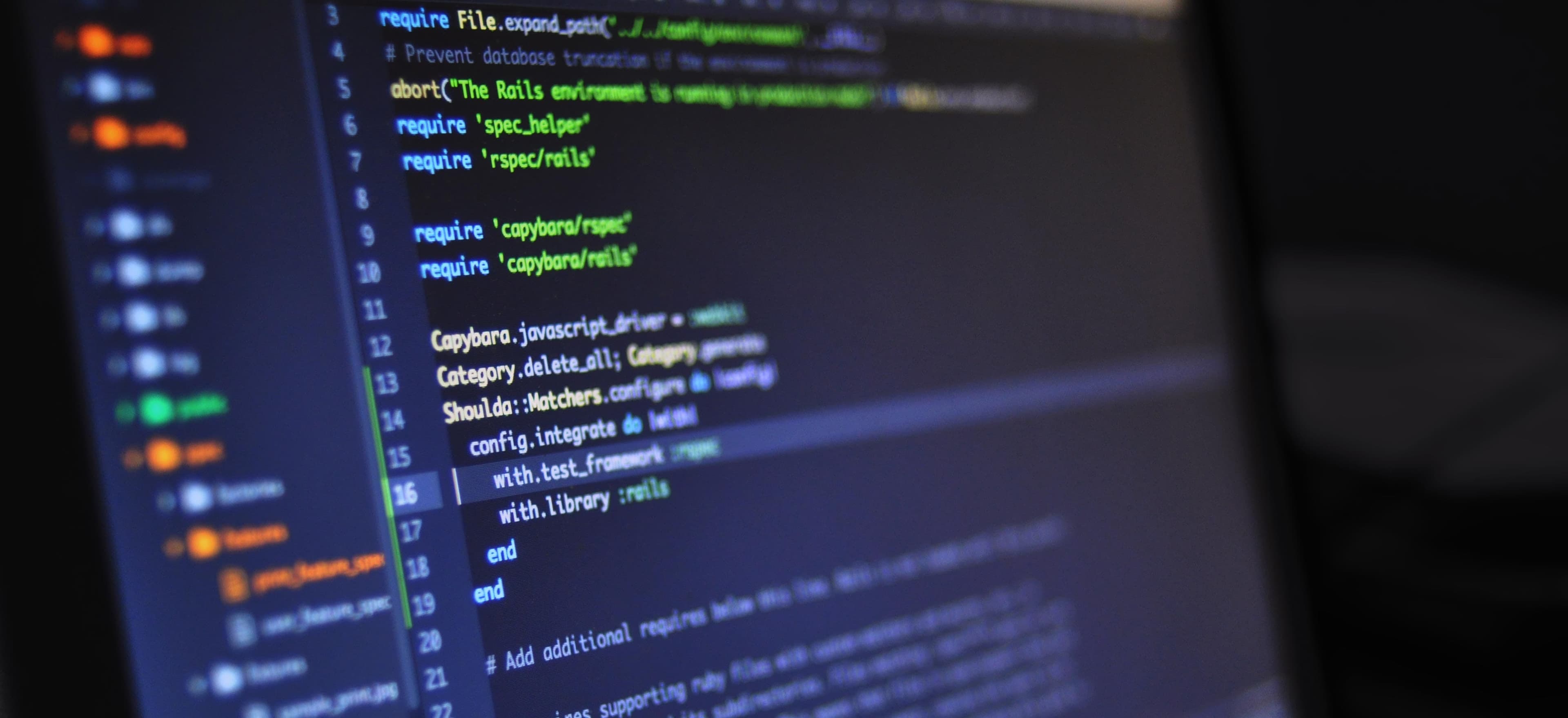
- Published on
Streamlining Java Database Connectivity for Multi-Database Apps
In today's dynamic tech landscape, applications often need to interact with multiple databases. This requirement can lead to complexity, especially when managing diverse data sources within a single Java application. In this article, we will explore strategies for streamlining Java Database Connectivity (JDBC) when working with multi-database applications. By the end, you will have a clear understanding of best practices to make your code cleaner, more efficient, and easier to maintain.
Understanding Java Database Connectivity (JDBC)
Java Database Connectivity (JDBC) is an API that enables Java applications to connect to various databases. JDBC provides the low-level framework for querying and updating the data, but it also requires careful management of resources.
When dealing with multiple databases, complexity arises not only in connecting to each database but also in maintaining the code efficiently. Here is a high-level breakdown of the JDBC steps you typically follow:
- Load the database driver.
- Establish a connection to the database.
- Create a statement object.
- Execute queries or updates.
- Process the results.
- Close the resources.
Here’s a basic example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class BasicJDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "username";
String password = "password";
try {
// Step 1: Load the database driver
Class.forName("com.mysql.cj.jdbc.Driver");
// Step 2: Establish a connection
Connection connection = DriverManager.getConnection(url, user, password);
// Step 3: Create a statement object
Statement statement = connection.createStatement();
// Step 4: Execute queries
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
// Step 5: Process the results
while (resultSet.next()) {
System.out.println("User ID: " + resultSet.getInt("id"));
System.out.println("Username: " + resultSet.getString("username"));
}
// Step 6: Close the resources
resultSet.close();
statement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this code snippet, we establish a JDBC connection and perform a simple SELECT operation. However, managing multiple databases requires a more sophisticated handling of connections.
Best Practices for Multi-Database JDBC Management
1. Configuration Management
Using configuration files for database connection parameters is a recommended approach. Properties files, XML, or even environment variables can be used to externalize configuration, making it easier to manage multiple database settings without hard coding values.
# db.properties
db1.url=jdbc:mysql://localhost:3306/mydb1
db1.user=username1
db1.password=password1
db2.url=jdbc:mysql://localhost:3306/mydb2
db2.user=username2
db2.password=password2
Accessing these configurations can be done using a utility class.
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class PropertiesUtil {
public static Properties loadProperties(String filePath) {
Properties properties = new Properties();
try (FileInputStream fis = new FileInputStream(filePath)) {
properties.load(fis);
} catch (IOException e) {
e.printStackTrace();
}
return properties;
}
}
This way, you can quickly add or modify database configurations without altering your code.
2. Connection Pooling
Establishing and closing a connection every time can severely impact performance. Use connection pooling libraries like HikariCP or Apache DBCP, which manage multiple connections efficiently.
Here’s a brief setup using HikariCP:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.1</version>
</dependency>
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class ConnectionPool {
private static HikariDataSource ds;
public static void init() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10);
ds = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return ds.getConnection();
}
}
3. Abstracting Database Logic
Creating a data access layer (DAL) using DAO patterns can significantly enhance your architecture. By isolating database logic, you retain the flexibility to change the underlying database without impacting other parts.
public interface UserDao {
void addUser(User user);
User getUser(int id);
}
public class UserDaoImpl implements UserDao {
@Override
public void addUser(User user) {
try (Connection conn = ConnectionPool.getConnection();
PreparedStatement ps = conn.prepareStatement("INSERT INTO users (username) VALUES (?)")) {
ps.setString(1, user.getUsername());
ps.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public User getUser(int id) {
User user = null;
try (Connection conn = ConnectionPool.getConnection();
PreparedStatement ps = conn.prepareStatement("SELECT * FROM users WHERE id = ?")) {
ps.setInt(1, id);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
user = new User(rs.getInt("id"), rs.getString("username"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return user;
}
}
Leveraging ORM Frameworks
In modern applications, Object Relational Mapping (ORM) frameworks like Hibernate can greatly simplify database interactions and eliminate boilerplate code.
Here’s a simple use case with Hibernate:
- Configure your
hibernate.cfg.xml
with multiple data sources. - Use the
SessionFactory
to create sessions for each database.
By propagating the burden of SQL management to Hibernate, you can focus on your business logic.
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">username</property>
<property name="hibernate.connection.password">password</property>
</session-factory>
</hibernate-configuration>
Referring to Previous Work
For developers handling multi-database setups, it's also insightful to explore how others have simplified their connection strategies. The article titled "Simplifying Multi-Database Connections on One Host" provides practical insights and approaches. You can find it here.
Final Considerations
Navigating multiple databases within a Java application can seem daunting at first, but by following the practices discussed above, you can streamline your JDBC connections effectively. Leveraging configuration management, connection pooling, and the DAO pattern will not only ease the process but also enhance performance and maintainability.
By embracing the power of ORM frameworks, you take another step towards efficient database management.
Make sure to explore the linked article above for more detailed strategies in managing your multi-database connections. Happy coding!