Crafting Compelling Narratives in Java App Development
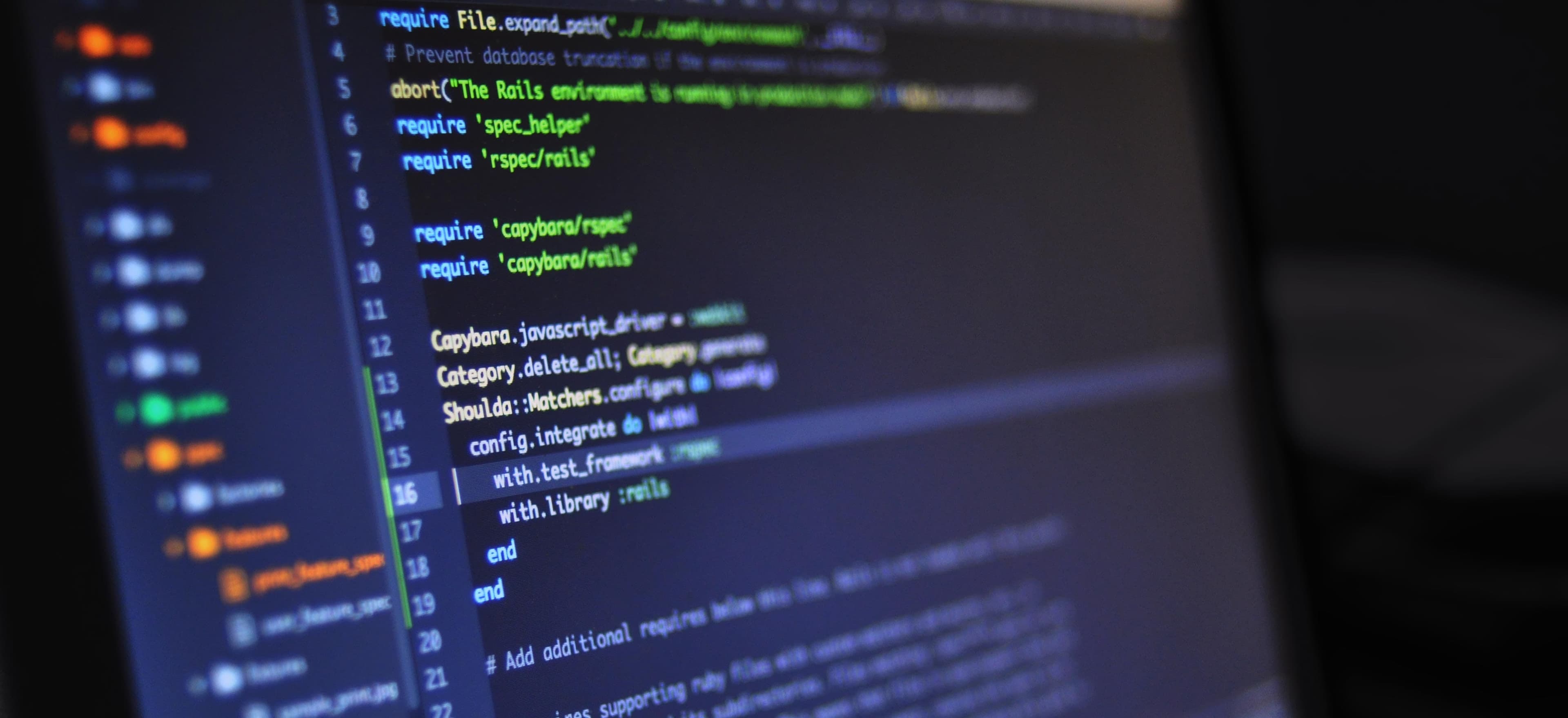
- Published on
Crafting Compelling Narratives in Java App Development
In the world of software development, particularly with Java, the importance of narrative is often overlooked. Developers focus on the syntax, the algorithms, and even the structure of the code. However, crafting compelling narratives can significantly enhance user experience (UX) and overall product value. This article will delve into how storytelling can be implemented in Java application development, drawing parallels to the necessity of effective storytelling highlighted in the article “How Poor Storytelling Can Ruin UX Design” (tech-snags.com/articles/poor-storytelling-ux-design).
The Role of Storytelling in Software Development
Storytelling is not just for writers or filmmakers; it's a fundamental aspect of human communication that can be harnessed in the digital landscape. A compelling narrative can guide users through the application, making it more engaging and simpler to navigate. When you present information in a cohesive story, users will find it more relatable and easier to remember.
Why Use Storytelling?
- User Engagement: A strong narrative makes the application more engaging.
- Guided Experience: It helps in guiding users through complex workflows.
- Brand Loyalty: A relatable and engaging story fosters a connection between the user and your brand.
Developing a Narrative Through Code
While writing code, you might wonder: how does one weave a narrative into the programming aspect of app development? Here, we’ll explore some techniques that involve storytelling at both the level of design and implementation.
1. Understand Your Audience
Before writing a single line of code, take time to understand your audience. This involves research to identify what they need and expect from your application.
Here's an example of how you can create user personas in Java:
import java.util.*;
class UserPersona {
String name;
String age;
String profession;
List<String> needs;
UserPersona(String name, String age, String profession, List<String> needs) {
this.name = name;
this.age = age;
this.profession = profession;
this.needs = needs;
}
void displayInfo() {
System.out.println("User Persona: " + name);
System.out.println("Age: " + age);
System.out.println("Profession: " + profession);
System.out.println("Needs: " + needs);
}
}
public class Main {
public static void main(String[] args) {
List<String> needsList = Arrays.asList("Fast response time", "User-friendly interface", "Easy navigation");
UserPersona user = new UserPersona("Alice", "28", "Software Engineer", needsList);
user.displayInfo(); // Display user persona
}
}
Why This Code Matters
By defining a UserPersona
class, we create a model for our target users. Every app should cater to its audience's needs. Understanding them informs design decisions that shape the narrative as users interact with the application. This alignment is vital for a positive user experience.
2. Implementing User Stories
Once you understand your users, create user stories. This allows you to map out the journey users take when engaging with your application. You can use a simple structure, such as: "As a [user type], I want to [goal] so that [reason]."
Example
Imagine a task management app. You might have user stories like this:
- As a busy professional, I want to categorize my tasks so that I can prioritize my work effectively.
- As a student, I want to set reminders for deadlines so that I don't miss any submissions.
These narratives can guide your programming approach.
3. Crafting User Interfaces That Tell Stories
The user interface (UI) is the first interaction users have with your application. It should visually narrate the intended message. Let's see how we can utilize a simple Java Swing GUI to improve user engagement.
import javax.swing.*;
import java.awt.event.*;
public class TaskManagerApp {
public static void main(String[] args) {
JFrame frame = new JFrame("Task Manager");
JButton categorizeButton = new JButton("Categorize Tasks");
categorizeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(frame, "Task Categorization helps prioritize workloads!");
}
});
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(categorizeButton);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Why the UI Example Works
In this example, when the user clicks the "Categorize Tasks" button, a message dialog pops up, reinforcing the significance of the action they are about to undertake. This reinforces the narrative of task prioritization, keeping users engaged with their goals.
4. Feedback Establishes Continuity
Part of any compelling narrative is feedback. Users need to know they are making progress. Utilize Java features to provide feedback based on user interactions.
For instance, integrating console feedback or using a logging system will help users understand what actions were successful, what failed, and next steps.
public void completeTask(String task) {
// Logic to complete the task
System.out.println(task + " has been marked as completed");
}
Importance of Feedback
Providing users with feedback summaries—like completed tasks—creates a continuous storyline of their experience within your app. This not only affirms their decisions but also inspires confidence in your application.
5. Testing the Story
Testing shouldn't just focus on functionality; it should assess if the narrative holds. A/B testing can help in identifying which story elements resonate more with users.
Bringing It All Together
Building narratives into Java application development isn't just about catchy graphics or appealing language—it's an intrinsic part of how users interact with, remember, and return to your application. From understanding user needs and crafting well-formed user stories to integrating informative user interfaces and continuous feedback, every aspect creates a compelling tale for users.
Engaging in these practices can lead to higher user satisfaction and loyalty, something every developer strives for. As you develop your Java applications, don't forget to weave a captivating story as you code. For further insights on how poor narratives can impact user experience, refer to the mentioned article “How Poor Storytelling Can Ruin UX Design” (tech-snags.com/articles/poor-storytelling-ux-design).
Resources
By investing time in Narratives, you won’t just create applications but establish lasting relationships with your users. Happy coding!
Checkout our other articles