Handling JavaScript Array Values in Java-Based Web Apps
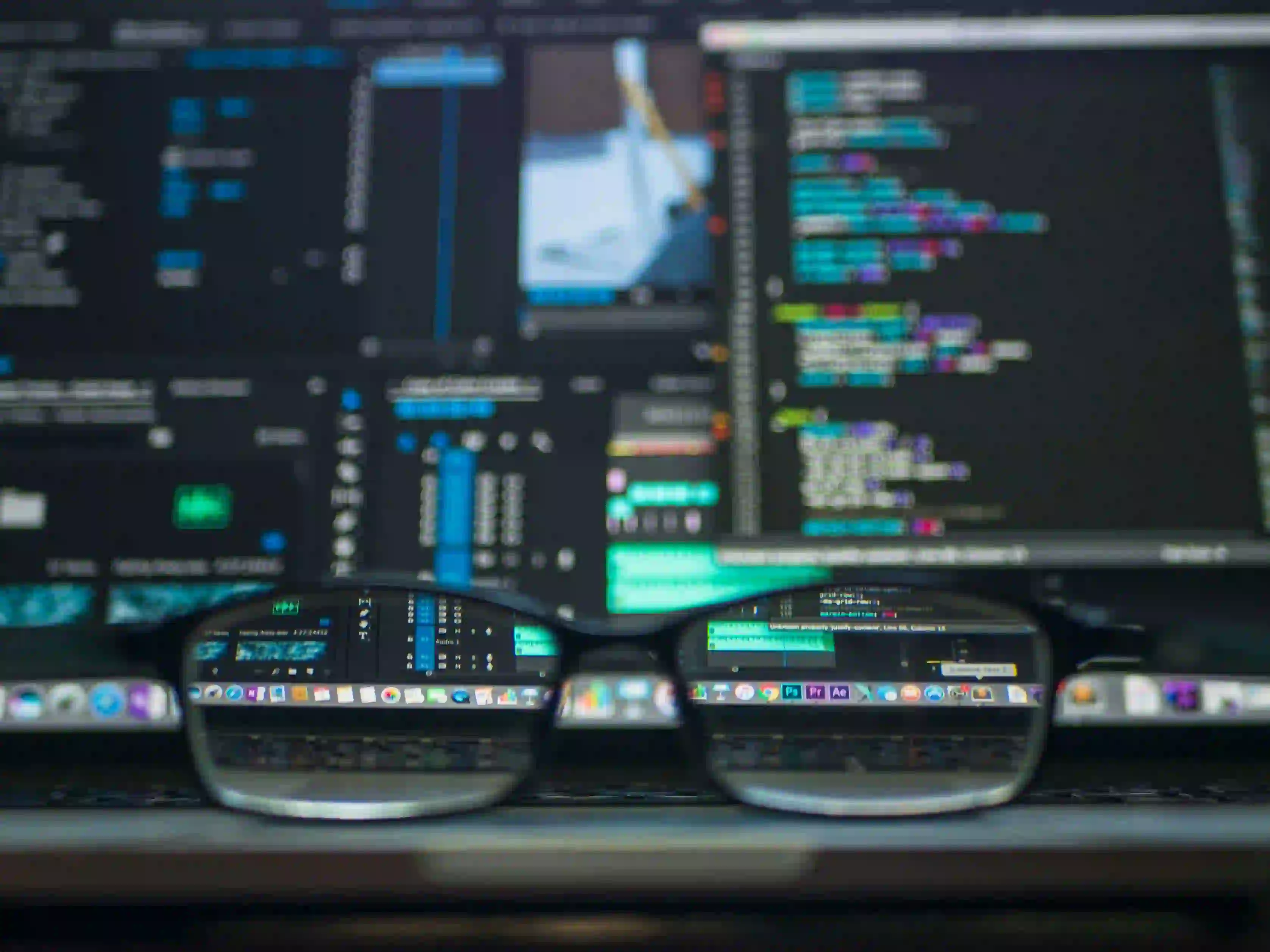
Handling JavaScript Array Values in Java-Based Web Apps
In today's web development landscape, bridging the gap between JavaScript and Java has become essential. Java powers the backend of many applications, while JavaScript is the dynamic scripting language that breathes life into the frontend. As a developer, understanding how to effectively manipulate JavaScript array values from your Java-based web applications is crucial. This article will delve into strategies for handling JavaScript array values in Java, focusing on both the technical and practical implications.
Understanding the Basics
Before exploring the intricate interactions between JavaScript and Java, let's clarify what we mean by JavaScript arrays. An array in JavaScript is a collection of data stored in a single variable. Arrays can easily hold not just strings and numbers but also objects and nested arrays. They are versatile data structures that enable developers to encapsulate various data types for more manageable coding.
For those interested in how to manipulate dynamic text box values using JavaScript, you can check out an insightful article titled Storing Dynamic Text Box Values in JavaScript Arrays.
Passing Data from JavaScript to Java
AJAX Calls
One of the primary ways to send JavaScript array values to a Java backend is through AJAX (Asynchronous JavaScript and XML) calls. AJAX allows us to send and retrieve data asynchronously without needing to refresh the web page. Using AJAX makes it seamless to manage data without disrupting the user experience.
Here is a simple example of how to use AJAX to send a JavaScript array to a Java servlet:
// Sample JavaScript array
const arrayData = ['apple', 'banana', 'orange'];
// Sending the data using AJAX
const xhr = new XMLHttpRequest();
xhr.open('POST', '/yourJavaServlet', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function () {
if (xhr.readyState === XMLHttpRequest.DONE) {
console.log('Response:', xhr.responseText);
}
};
// Convert the array data into JSON format
xhr.send(JSON.stringify(arrayData));
Why JSON?
Using JSON (JavaScript Object Notation) for data interchange is advantageous for several reasons:
- Simplicity: JSON is easy to read and write, making it a widely accepted data format.
- Compatibility: Both Java and JavaScript can easily parse JSON data, ensuring smooth integration.
- Lightweight: JSON is less verbose compared to XML, which can speed up communication between client and server.
Java Code for Handling Incoming Data
On the Java side, you will have to set up a servlet to handle the incoming data sent from the client-side JavaScript. Below is an example of a Java servlet that processes the JSON array sent via AJAX:
import java.io.BufferedReader;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/yourJavaServlet")
public class YourJavaServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Read the incoming JSON data
StringBuilder jsonBuilder = new StringBuilder();
String line;
BufferedReader reader = request.getReader();
while ((line = reader.readLine()) != null) {
jsonBuilder.append(line);
}
// Parse the received JSON data
String jsonArrayString = jsonBuilder.toString();
String[] arrayData = new Gson().fromJson(jsonArrayString, String[].class);
// Process the array data
for (String item : arrayData) {
System.out.println("Received: " + item);
}
// Respond back
response.setContentType("application/json");
response.getWriter().write("{\"status\":\"success\"}");
}
}
Why Use Gson?
In the example above, the Gson library is employed to convert JSON into Java objects. This library is popular among developers for its ease of use and efficiency when dealing with JSON serialization and deserialization.
- Serialization: Converts Java objects into JSON format.
- Deserialization: Converts JSON data back into Java objects.
Using third-party libraries, such as Gson, simplifies this relatively complex task, improving the maintainability of your code.
Handling Data Corrections
If user-input data needs to be validated or corrected, the best practice is to include validation logic in both the JavaScript and Java layers. JavaScript provides instant feedback while Java ensures that the data meets your backend architecture's requirements.
Client-Side Validation Example
Before sending data to the server, you can implement a client-side validation:
function validateArrayData(dataArray) {
// Validate the content of the array
return dataArray.every(item => typeof item === 'string' && item.trim() !== '');
}
if (validateArrayData(arrayData)) {
// Proceed with the AJAX call
// code from above here
} else {
alert('Please provide valid input.');
}
Server-Side Validation Example
Similarly, your server-side logic should also validate the incoming data:
// Example of additional validation
if (arrayData == null || arrayData.length == 0) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST, "Empty array data received");
return;
}
Final Thoughts
Handling JavaScript array values in Java-based web applications is a vital skill that can greatly enhance your application's performance and user experience. By effectively passing data through AJAX and processing it through Java servlets, you can create highly interactive and dynamic web applications.
Be sure to employ robust validation techniques both in JavaScript and Java. This ensures data integrity and enhances the overall security of your application.
For further insights on managing dynamic elements in web development, including dynamic text box values in JavaScript, take a look at the article: Storing Dynamic Text Box Values in JavaScript Arrays.
Additional Resources
- Java Documentation
- JavaScript Array Official Documentation
- AJAX with Java: A Complete Guide
Through understanding and implementation, the interplay between Java and JavaScript can transform your web applications into powerful tools capable of providing seamless user experiences. Happy coding!