Managing Dynamic Data Input in Java: A Practical Guide
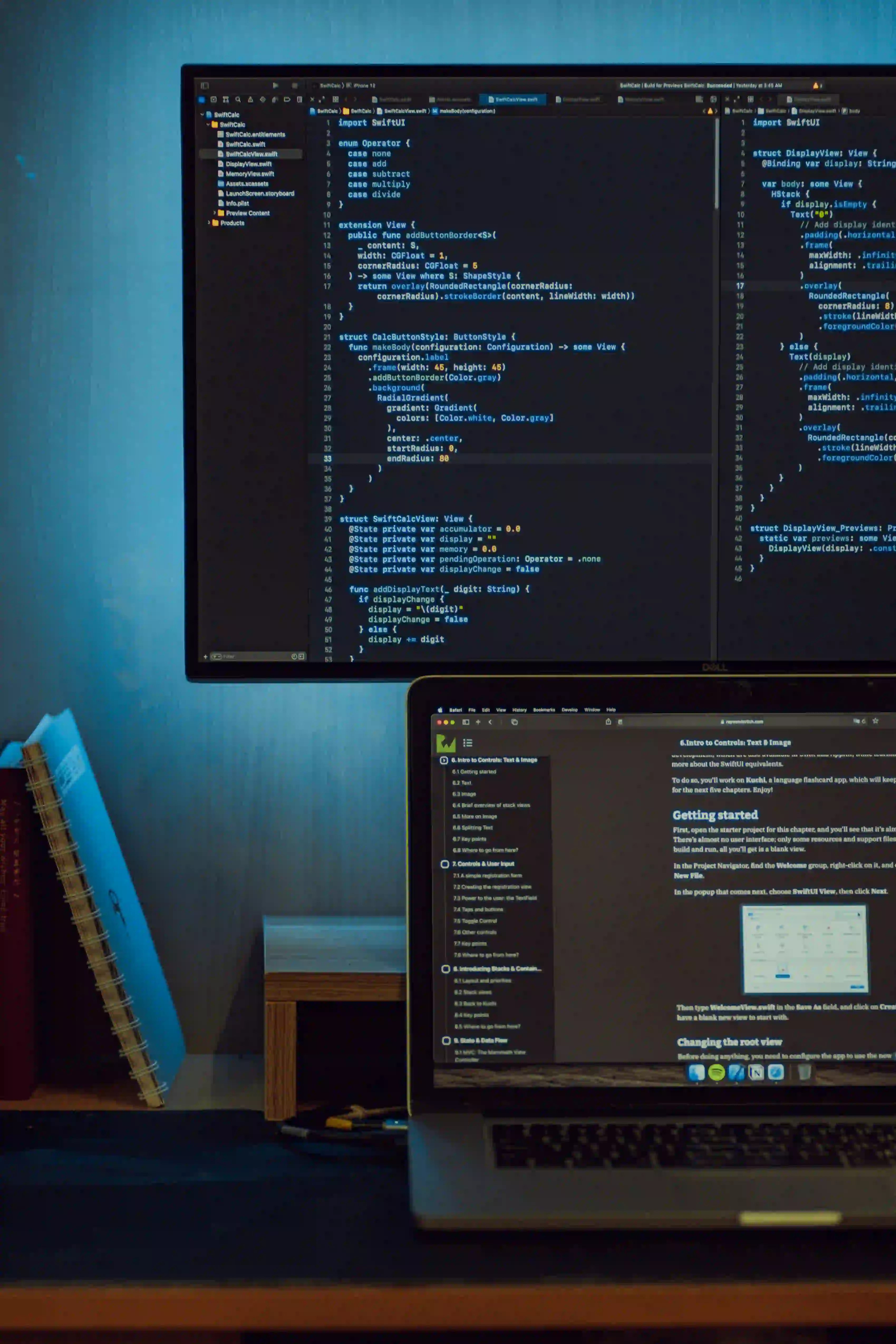
Managing Dynamic Data Input in Java: A Practical Guide
In today's programming landscape, handling dynamic data input is a common necessity. From web applications to mobile apps, developers frequently encounter situations where they need to manage and store dynamic values efficiently. In this guide, we will explore various aspects of managing dynamic data input in Java, including best practices, code examples, and insightful analysis to enrich your understanding.
Understanding Dynamic Data
Dynamic data refers to data that changes or is generated at runtime. This can be from user input, APIs, or other external sources. For instance, consider a web form where users can input multiple fields that are added or removed dynamically.
This dynamic behavior is akin to handling arrays in JavaScript, as explained in the article Storing Dynamic Text Box Values in JavaScript Arrays. However, Java provides robust ways to manage such scenarios, offering a rich API and data structures to facilitate flexible data handling.
Key Concepts
- Collections Framework: Java provides a rich Collections framework that includes List, Set, and Map interfaces, which makes handling dynamic collections of objects straightforward.
- Event Handling: Capturing user input dynamically often involves event listeners and handling methods.
- Concurrency: Managing dynamic data must often account for multiple threads, particularly in web applications or multi-user environments.
The Role of the Java Collections Framework
The Java Collections Framework is fundamental when it comes to managing dynamic data. We'll focus primarily on the ArrayList
, which provides a resizable array implementation.
Creating an ArrayList
First, let’s look at how you can create an ArrayList and add elements to it:
import java.util.ArrayList;
public class DynamicDataExample {
public static void main(String[] args) {
ArrayList<String> dynamicList = new ArrayList<>();
dynamicList.add("Item 1"); // Adding dynamic data
dynamicList.add("Item 2");
System.out.println("Dynamic List: " + dynamicList);
}
}
Why Use ArrayList?
An ArrayList
is dynamic, meaning it can grow and shrink as needed. If you try to add more items than it was initialized for, it automatically expands its capacity. This is vital in scenarios where we don’t know the number of inputs in advance.
Removing Items
Dynamic data input is not just about adding data, but also removing it. Here’s an example of how to remove an item:
public static void removeItem(ArrayList<String> list, String item) {
if (list.remove(item)) {
System.out.println(item + " has been removed.");
} else {
System.out.println(item + " was not found in the list.");
}
}
// Usage
removeItem(dynamicList, "Item 1"); // Removes Item 1
Handling User Input Dynamically
In a desktop or web application, handling user input in real-time is essential. We often use GUI frameworks like JavaFX to visually represent dynamic data inputs.
Example with JavaFX
Let’s create a simple JavaFX application to manage a list of names. Users can add and remove names dynamically.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.util.ArrayList;
public class DynamicInputApp extends Application {
private ArrayList<String> namesList = new ArrayList<>();
private ListView<String> listView = new ListView<>();
private TextField inputField = new TextField();
@Override
public void start(Stage primaryStage) {
VBox vbox = new VBox(inputField, listView);
Button addButton = new Button("Add Name");
addButton.setOnAction(e -> addName());
Button removeButton = new Button("Remove Selected Name");
removeButton.setOnAction(e -> removeName());
vbox.getChildren().addAll(addButton, removeButton);
Scene scene = new Scene(vbox, 300, 250);
primaryStage.setTitle("Dynamic Data Input Example");
primaryStage.setScene(scene);
primaryStage.show();
}
private void addName() {
String name = inputField.getText();
namesList.add(name);
listView.getItems().add(name);
inputField.clear();
}
private void removeName() {
String selectedName = listView.getSelectionModel().getSelectedItem();
namesList.remove(selectedName);
listView.getItems().remove(selectedName);
}
public static void main(String[] args) {
launch(args);
}
}
Explaining the Code
- GUI Elements: We create a
TextField
for user input and aListView
for display. - Adding Names: On clicking the "Add Name" button, the name from the input field is added to both the
ArrayList
and theListView
. - Removing Names: The selected name can be removed from both the list and the
ArrayList
.
This example shows how dynamic data can be effectively managed with the help of Java's GUI capabilities and ArrayList for backend data structure.
Event Handling
Java Event Handling is crucial when dealing with dynamic data input. Whether using Swing, JavaFX, or another GUI framework, listeners allow you to trigger actions based on user interactions.
Why Use Event Listeners?
Event listeners enable you to create a responsive user interface. You can capture events like button clicks, text entries, or list selections. This results in applications that feel intuitive and user-friendly.
Implementing an Event Listener
In the JavaFX example above, the setOnAction
method is used to register actions to buttons. Here’s a breakdown:
addButton.setOnAction(e -> addName());
This line registers the addName
method to be called whenever the "Add Name" button is clicked.
The Bottom Line
Managing dynamic data input in Java is essential for developing responsive applications. The use of the Java Collections Framework, especially the ArrayList
, allows developers to handle data's unpredictabilities efficiently. Event handling further enhances interactivity, ensuring users have a seamless experience.
For those interested in client-side dynamic applications, you might find it insightful to check the foundational concepts presented in the article on Storing Dynamic Text Box Values in JavaScript Arrays.
By mastering these techniques and concepts, you will be well-equipped to handle various dynamic data input scenarios in your Java applications. Whether you're building an enterprise solution or a personal project, understanding these principles can greatly enhance both functionality and user experience.