Navigating Java Streams: Tackle Array Pitfalls Effectively
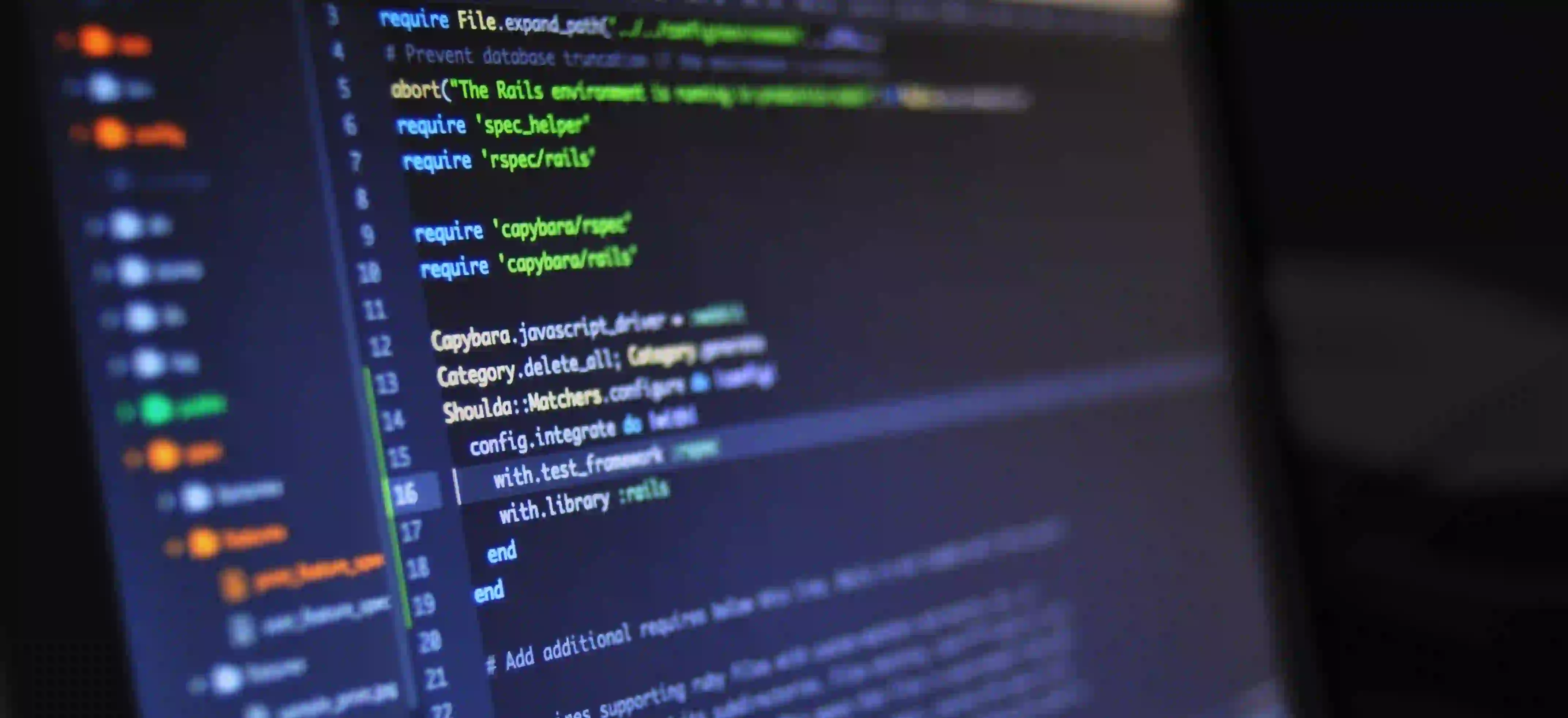
Navigating Java Streams: Tackle Array Pitfalls Effectively
Java Streams provide a powerful abstraction for working with sequences of data, but their true potential is often underutilized—especially when dealing with arrays. This blog post will guide you through the fundamentals of Java Streams and how to effectively implement them to navigate common pitfalls when working with arrays.
If you're looking for a more thorough explanation of how to master arrays and utilize functional programming with them, check out the article Mastering Arrays: Avoid Common Pitfalls with Map, Filter & Reduce.
What are Java Streams?
Introduced in Java 8, Streams are sequences of elements supporting sequential and parallel aggregate operations. They can be thought of as a conduit for processing data in a functional style. Streams can be created from collections, lists, sets, and arrays.
Key Features of Streams
- Functional Style: Streams enable you to express complex data processing queries succinctly.
- Lazy Evaluation: Operations such as map and filter are not executed until the terminal operation is invoked. This can lead to performance improvements.
- Ease of Parallelism: Streams can be effortlessly switched to parallel operations for improved performance on multi-core processors.
Getting Started with Streams
To use Streams, we need to understand their basic operations:
- Creating Streams: Streams can be created from various data sources such as Collections and arrays.
- Intermediate Operations: Operations that transform the data in the Stream, such as
map
andfilter
. - Terminal Operations: Operations that produce a result or side effect, such as
forEach
,collect
, orreduce
.
Here's how to create a stream from an array:
String[] names = {"Alice", "Bob", "Charles"};
Stream<String> nameStream = Arrays.stream(names);
Why Use Streams?
- Readability: Your intention becomes immediately clear to anyone reading your code.
- Reduced Boilerplate: Forget the loops; Streams allow you to write succinct code.
- Efficient Data Processing: Stream operations can often be performed in a single pass.
Common Pitfalls with Java Streams
While Streams can be extremely beneficial, they also come with their own set of common pitfalls, especially when dealing with arrays. Here are a few to watch out for:
1. Modifying the Underlying Array
One common mistake is modifying the original data structure while using streams. The operations are generally not meant for mutation; they are best suited for read-only scenarios. If mutation is required, consider a more explicit approach.
String[] names = {"Alice", "Bob", "Charles"};
Stream.of(names)
.map(name -> {
if (name.equals("Bob")) return "Robert";
return name;
})
.forEach(System.out::println);
Commentary:
In the above code snippet, we should avoid modifying the names array directly. Instead, we create a new mapping for the name "Bob". This approach ensures our array remains intact and avoids unintended side effects.
2. Not Handling Empty Streams
Handling empty arrays or collections can often lead to NullPointerExceptions or unexpected behavior. Always ensure that your operations account for potential emptiness.
String[] names = {};
Arrays.stream(names)
.map(String::toUpperCase)
.findFirst()
.ifPresent(System.out::println); // Does nothing if the array is empty
Commentary:
Using findFirst()
is a great way to handle elements in a stream; however, on an empty array, it will simply do nothing. Incorporating methods like ifPresent()
prevents null risks and account for potentially empty streams.
3. Forgetting to Collect Results
Stream operations are often terminated by a collection step—typically with collect()
. Forgetting this can lead to an overlooked pipeline.
List<String> upperCaseNames = Arrays.stream(names)
.map(String::toUpperCase)
.collect(Collectors.toList());
Commentary:
In this code snippet, forgetting to call .collect(Collectors.toList())
would result in a Stream that is not properly converted to a List, rendering the processed data unutilized.
Efficient Data Filtering
Filtering data using Streams can be profoundly effective but also requires careful consideration of conditions.
Example: Filtering Names Longer than 3 Characters
String[] names = {"Kim", "Sam", "Allison", "Bob"};
List<String> longNames = Arrays.stream(names)
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
System.out.println(longNames); // Output: [Allison]
Commentary:
Filter operations only keep elements that satisfy the condition. This code is clean, focused, and reads naturally, representing what you want to achieve.
The Power of Mapping
One of the great advantages of Streams is the map
function, which allows you to transform data easily.
Example: Mapping Names to Their Lengths
int[] nameLengths = Arrays.stream(names)
.mapToInt(String::length)
.toArray();
System.out.println(Arrays.toString(nameLengths)); // Output: [3, 3, 7, 3]
Commentary:
Here, mapToInt()
transforms our String array into an array of integers reflecting the lengths of each name. This succinct transformation shows how Streams simplify data manipulation.
Advanced: Reduce Operations
The reduce
function can be used to combine Stream elements into a single result.
Example: Calculating the Total Length of Names
int totalLength = Arrays.stream(names)
.mapToInt(String::length)
.reduce(0, Integer::sum);
System.out.println(totalLength); // Output: Total length of all names
Commentary:
In this example, we effectively accumulate the total length of all names in one concise statement. The reduce
function is instrumental for cases where you want to summarize or combine data.
The Bottom Line
Java Streams transform the huge landscape of Java programming by introducing a functional style of dealing with sequences of data. While they offer powerful capabilities, it's essential to be aware of common pitfalls when working with arrays. The practices outlined above—avoiding mutability, handling empty streams, ensuring results are collected, and making the most of filtering, mapping, and reducing—will pave the way for efficient programming.
For a deeper dive into how to handle arrays in Java with practical examples, do not forget to visit Mastering Arrays: Avoid Common Pitfalls with Map, Filter & Reduce. Here, you will find additional insights and solidify your understanding of effective array manipulation.
With careful attention to detail, you can navigate the world of Java Streams and unlock their potential to write clean, efficient, and readable code!