Enhance Java Operations: Master Map, Filter & Reduce Techniques
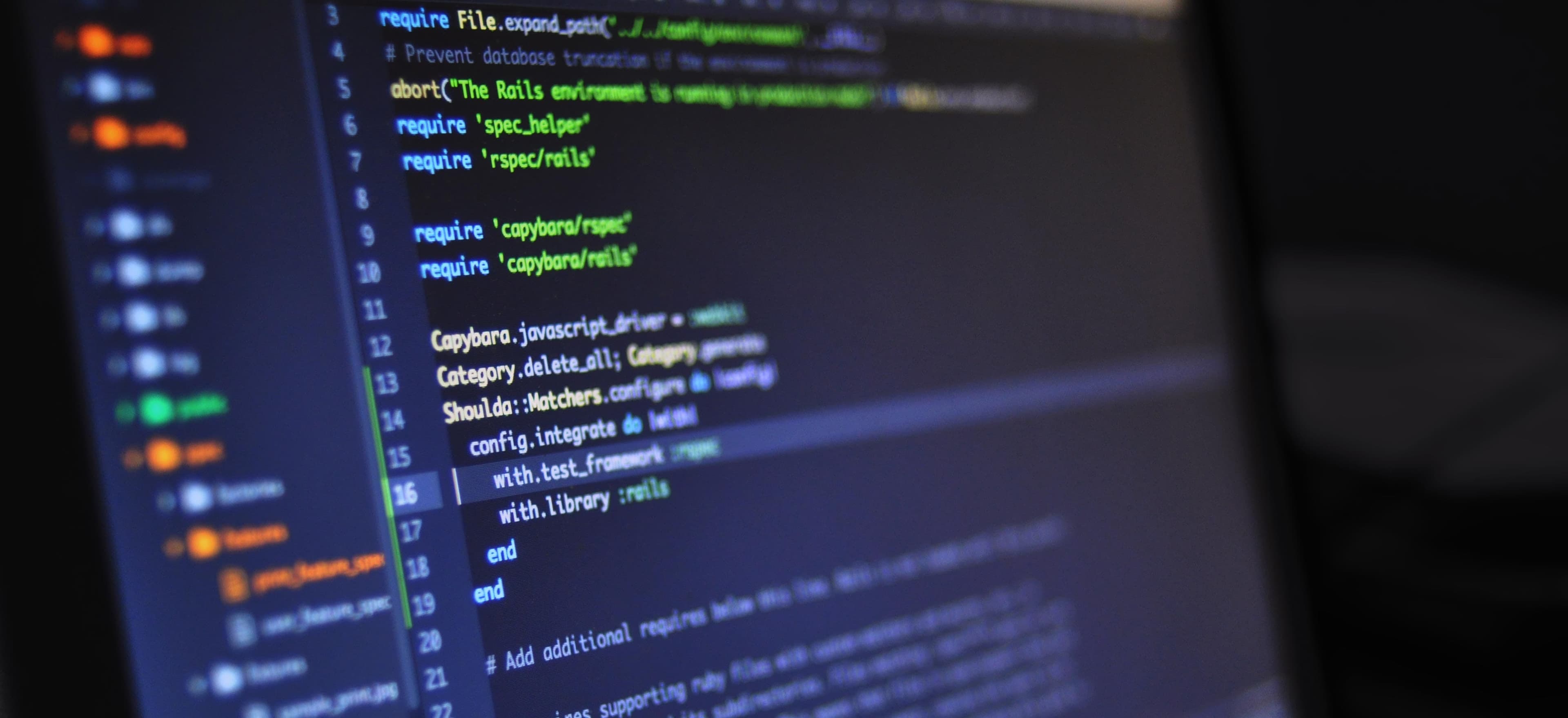
- Published on
Enhance Java Operations: Master Map, Filter & Reduce Techniques
In the world of programming, especially in Java, manipulating data collections efficiently is a fundamental skill for every developer. One popular way to handle collections is through the powerful trio of functional programming techniques: Map, Filter, and Reduce. These techniques not only improve code readability but also boost performance when used properly. In this blog, we'll dive deep into these concepts, demonstrating their practical applications with clear code examples and explanations.
Understanding Map, Filter, and Reduce
Before we leap into code, let's briefly explain the terms.
-
Map: This operation transforms each element of a collection into a new form. For instance, if you have a list of integers and you wish to square each number, the map function allows you to accomplish this elegantly.
-
Filter: As the name suggests, filter is used to select certain elements from a collection based on specific criteria. For example, you can filter a list of integers to find only the even numbers.
-
Reduce: This operation is utilized to aggregate all elements of a collection into a single value. For example, summing up all the elements in a list is a common use case.
Setting Up Your Java Environment
Before you can effectively apply Map, Filter, and Reduce, you need to be equipped with the latest Java Development Kit (JDK). Make sure you have JDK 8 or higher, as these functional programming techniques are part of the Stream API introduced in Java 8.
Installing JDK
If you haven't already installed JDK, you can download it from the Oracle website. Follow the setup instructions specific to your operating system.
Utilizing Streams with Map, Filter, and Reduce
Let's explore how to implement these operations using Java Streams.
Example 1: Map Operation
Here's a simple example to understand the Map operation:
import java.util.List;
import java.util.stream.Collectors;
import java.util.Arrays;
public class MapExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using map to square each number
List<Integer> squaredNumbers = numbers.stream()
.map(number -> number * number)
.collect(Collectors.toList());
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
}
}
Why Use Map?
The map()
function takes each element from the numbers
list, squares it, and then returns a new collection with the squared values. This shows how easily transformations can be handled in a functional style, improving both clarity and conciseness.
Example 2: Filter Operation
Next, let's see the Filter operation in action:
import java.util.List;
import java.util.stream.Collectors;
import java.util.Arrays;
public class FilterExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Using filter to find even numbers
List<Integer> evenNumbers = numbers.stream()
.filter(number -> number % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6]
}
}
Why Use Filter?
The filter()
method applies a predicate (in this case, checking if a number is even) and creates a new collection containing only those elements that meet the criteria. Using filter, you can maintain a clear separation of concerns in your code. A developer can easily read and understand that this portion is designed to select even numbers from the list.
Example 3: Reduce Operation
Finally, let’s explore the Reduce operation:
import java.util.List;
import java.util.Arrays;
public class ReduceExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using reduce to calculate the sum of the numbers
int sum = numbers.stream()
.reduce(0, (total, number) -> total + number);
System.out.println(sum); // Output: 15
}
}
Why Use Reduce?
The reduce()
method combines all elements in the stream into a single result based on the operation provided. In this case, it sums all the numbers in the list. This operation is particularly useful for aggregating values and can be leveraged for more complex operations where multiple values are derived into one.
Combining Map, Filter, and Reduce
You can chain these operations together seamlessly for more complex data processing. Consider the following example:
import java.util.List;
import java.util.stream.Collectors;
import java.util.Arrays;
public class CombinedExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Combine map, filter, and reduce
int sumOfSquaresOfEvenNumbers = numbers.stream()
.filter(number -> number % 2 == 0) // Step 1: Filter even numbers
.map(number -> number * number) // Step 2: Square the even numbers
.reduce(0, (total, number) -> total + number); // Step 3: Sum the squares
System.out.println(sumOfSquaresOfEvenNumbers); // Output: 56
}
}
Why Combine These Operations?
Chaining these methods allows for a more expressive and concise representation of data transformations and reductions. Moreover, it promotes a functional programming style that can lead to cleaner, more maintainable code. Each operation is a clear step in the overall process.
Common Pitfalls to Avoid
While implementing Map, Filter, and Reduce techniques, developers can encounter several common pitfalls. To effectively harness the potential of these operations, it's important to be aware of:
- Overusing Streams: Don't convert to Streams unnecessarily. The overhead of creating a stream might outweigh the benefits for smaller collections.
- Null Handling: Ensure the collection you are streaming is not null; otherwise, you'll run into a NullPointerException.
- Order of Operations: Remember that the order of operations matters. Filtering before mapping can improve performance by reducing the number of elements that need to be processed.
For more insights on arrays and common pitfalls, you can refer to the article titled Mastering Arrays: Avoid Common Pitfalls with Map, Filter & Reduce.
My Closing Thoughts on the Matter
Mastering Map, Filter, and Reduce in Java can immensely improve your code's quality and performance. These operations empower you to manipulate collections in a functional style, enhancing both readability and maintainability.
As you practice these techniques, remember the importance of clean code and comprehensive documentation. The efficient combination of these functional programming concepts not only streamlines your workflow but also serves as a solid foundation for advanced topics in Java development.
With practice, these operations will become second nature, helping you craft elegant solutions to complex problems. Happy coding!
Checkout our other articles