Resolving JavaScript Conflicts with Wow Slider in Java Apps
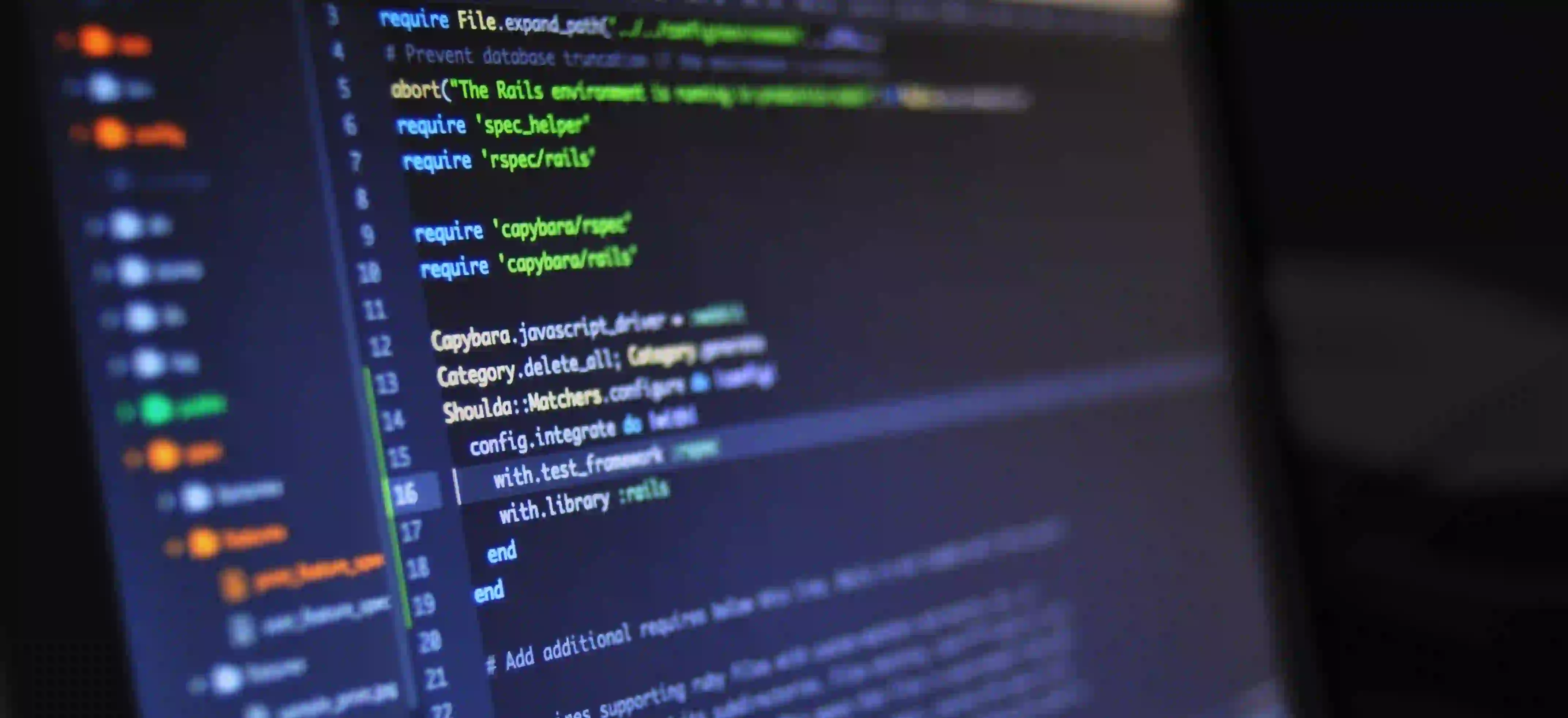
Resolving JavaScript Conflicts with Wow Slider in Java Apps
The web has irrevocably shifted towards dynamic, interactive experiences, making JavaScript a critical component of modern web applications. Java developers, while primarily focused on Java, often need to interact with JavaScript libraries to enhance their applications. Among these libraries is the Wow Slider, a powerful and attractive image slider. However, integrating Wow Slider into Java applications can sometimes lead to JavaScript conflicts that can affect functionality. This blog post will delve into how to resolve these conflicts efficiently.
Understanding Wow Slider
Wow Slider is a popular jQuery plugin that allows for the creation of stunning image sliders. It is known for its smooth animations and versatile features. You can view more about it on the official website Wow Slider.
When using Wow Slider in Java-based applications, especially those that use frameworks like Spring or JavaServer Faces (JSF), it's crucial to ensure that JavaScript functions are executed correctly. Errors can arise due to conflicting libraries or improper initialization sequences.
Common Issues with Wow Slider
Before we discuss solutions, let’s list some common issues you may face when integrating Wow Slider in Java apps:
- Conflicting JavaScript libraries
- Incorrect jQuery version
- Multiple DOM manipulations leading to race conditions
Example: Initializing Wow Slider
Here’s how we typically initialize a Wow Slider:
<!-- Include jQuery and Wow Slider -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" type="text/css" href="path/to/wowslider.css" />
<div id="wowslider-container">
<div class="ws_images">
<ul>
<li><img src="image1.jpg" alt="Image 1" /></li>
<li><img src="image2.jpg" alt="Image 2" /></li>
</ul>
</div>
</div>
<script>
$(document).ready(function() {
$('#wowslider-container').wowSlider();
});
</script>
Why This Code?
- jQuery Inclusion: Ensure jQuery is included before Wow Slider. If not, it may result in errors.
- Initialization: The
$(document).ready()
method ensures the DOM is fully loaded before applying the Wow Slider.
Troubleshooting JavaScript Conflicts
Identifying Conflicts
If your Wow Slider doesn’t function as expected, you might encounter JavaScript conflicts. Here’s how to identify them:
- Open the Browser Console: Use the F12 key to open developer tools in your browser. Inspect for any JavaScript errors.
- Check Library Versions: Ensure that jQuery is compatible with Wow Slider. Sometimes, a newer version of jQuery may have breaking changes.
- Look for Duplicate Libraries: Ensure that jQuery or any other library are not loaded multiple times.
Resolving Conflicts
After identifying conflicts, you can use the following strategies:
-
jQuery No Conflict Mode: Use jQuery’s
noConflict()
to avoid conflicts with other libraries that use the$
symbol.🟨snippet.jsvar jQueryjQ = jQuery.noConflict(); jQueryjQ(document).ready(function() { jQueryjQ('#wowslider-container').wowSlider(); });
-
Loading Order: Ensure that the libraries are loaded in the correct order (jQuery first, then Wow Slider).
-
Single Instance: Avoid multiple references to jQuery. Use a single instance to prevent loading different versions.
Example Code with Comments
Here’s an optimized code example demonstrating all the aforementioned practices:
<!DOCTYPE html>
<html>
<head>
<title>Wow Slider Example</title>
<link rel="stylesheet" type="text/css" href="path/to/wowslider.css" />
</head>
<body>
<div id="wowslider-container">
<div class="ws_images">
<ul>
<li><img src="image1.jpg" alt="Image 1" /></li>
<li><img src="image2.jpg" alt="Image 2" /></li>
</ul>
</div>
</div>
<!-- Load jQuery and Wow Slider -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="path/to/wowslider.js"></script>
<script>
// Use noConflict to prevent issues with other JavaScript libraries
var jQueryjQ = jQuery.noConflict();
jQueryjQ(document).ready(function() {
jQueryjQ('#wowslider-container').wowSlider();
});
</script>
</body>
</html>
Why This Example Works
- Separation of Concerns: HTML structure is clean without inline styles, while functionality is strictly managed in the JavaScript section.
- No Conflict: By using
noConflict()
, we prevent any issues arising from potential name conflicts with other libraries. - Modularity: The code can be reused and adapted easily into other Java applications.
Testing Your Implementation
After making the changes:
- Test in Multiple Browsers: Sometimes issues may appear on specific browsers due to varying support for JavaScript functionalities.
- Use Tools: Employ tools like Lint to check for JavaScript errors and code quality.
In Conclusion, Here is What Matters
Integrating Wow Slider into Java applications involves understanding both Java and JavaScript ecosystems. By identifying and resolving potential conflicts, developers can harness the full potential of Wow Slider and deliver captivating experiences to users.
For more insights on resolving specific JavaScript conflicts, check out the article titled Fixing Wow Slider Issues in Internet Explorer.
Following these guidelines should alleviate most integration headaches, allowing you to focus on developing your Java application without distractions. Happy coding!