Navigating Dynamic Fragments: Overcoming Common Java Challenges
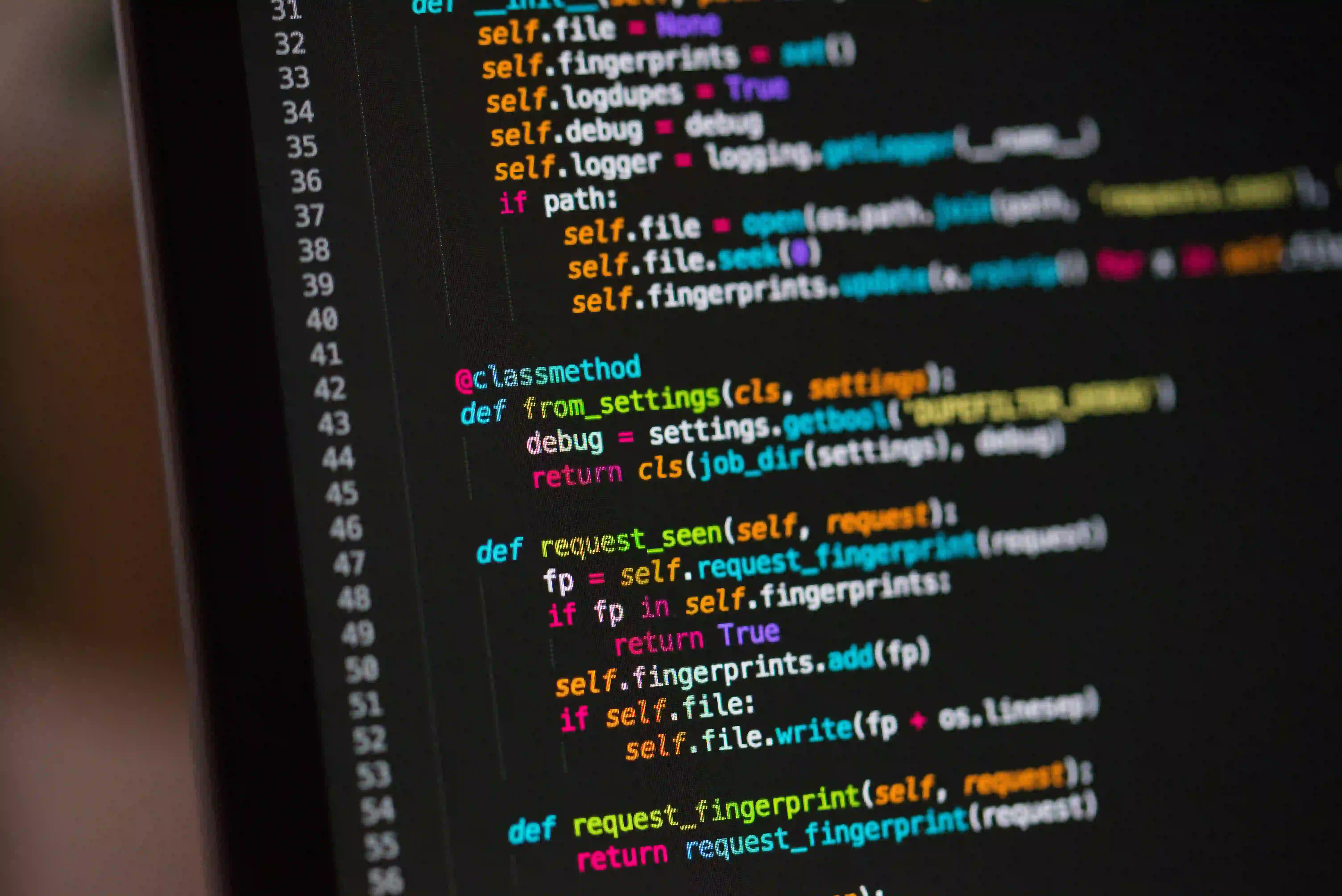
Navigating Dynamic Fragments: Overcoming Common Java Challenges
When it comes to developing dynamic applications in Java, the use of fragments has become increasingly popular, especially in Android development. Dynamic fragments allow you to create modular components that can adapt to different screen sizes and resolution. However, incorporating them can lead to a variety of challenges. In this blog post, we will explore how to navigate through these challenges and enhance your application’s user experience.
Understanding Fragments in Java
At its core, a fragment represents a portion of your user interface in an activity. Unlike traditional Java classes, fragments are designed to be reusable components that encapsulate their own lifecycle, inputs, and outputs. This modularity fosters better code organization, making it easier to maintain and update applications.
Why Use Fragments?
- Reusability: Create diverse UIs from the same set of components.
- User Interface Flexibility: Fragments can be added, removed, or replaced as needed.
- Lifecycle Management: Each fragment maintains its own lifecycle, offering better resource management.
Example of a Simple Fragment
Let's look at how to create a simple fragment in Java.
public class MyFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_my, container, false);
}
}
Why use onCreateView? This method is crucial for inflating the fragment’s layout and creating the view hierarchy.
Common Challenges with Dynamic Fragments
While using fragments, developers often encounter several challenges. This blog aims to address these challenges and provide solutions.
1. Managing Fragment Transactions
When manipulating fragments dynamically, understanding fragment transactions is critical. Attempting to add or replace fragments without proper management can lead to a fragmented user experience.
Solution
Use the FragmentTransaction
class for all fragment-related operations. Here is an example of how to properly handle fragment transactions:
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = new MyFragment();
transaction.replace(R.id.fragment_container, fragment);
transaction.addToBackStack(null); // Allows the user to navigate back
transaction.commit();
Why add to back stack? This allows users to navigate back to previously displayed fragments seamlessly, improving user experience.
2. Handling Fragment Lifecycle Events
Understanding the fragment lifecycle can be perplexing. Developers often forget to implement lifecycle methods such as onPause()
, onResume()
, etc.
Solution
Be thorough in managing all lifecycle states:
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Perform initial setup here
}
@Override
public void onPause() {
super.onPause();
// Handle pause-specific functions
}
Why care about lifecycle events? Not managing these events properly can lead to memory leaks and degraded performance.
3. Passing Data Between Fragments
Sharing data between fragments can be tricky. Many developers tend to use static variables, leading to tightly coupled components.
Solution
Leverage the Bundle
to pass data:
MyFragment fragment = new MyFragment();
Bundle args = new Bundle();
args.putString("key", "value");
fragment.setArguments(args);
In your fragment, you can retrieve the data in onCreate
:
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
String value = getArguments().getString("key");
}
}
Why use bundles? This method maintains a level of decoupling between components and adheres to best practices.
4. Configuration Changes
Configuration changes, such as screen rotations, can destroy and recreate fragments, leading to lost state or unexpected behavior.
Solution
To handle configuration changes, override the onSaveInstanceState()
method to save the state:
@Override
public void onSaveInstanceState(@NonNull Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("key", "value");
}
When the fragment is recreated, retrieve the data in onCreate()
:
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (savedInstanceState != null) {
String value = savedInstanceState.getString("key");
}
}
Why save instance state? This technique ensures that fragment state is preserved during configuration changes.
5. Updating UI from Background Threads
Background operations often require updating the UI, which can only be done on the main thread, leading to potential thread-safety issues.
Solution
Utilize the Handler
or runOnUiThread()
method to safely update UI components:
runOnUiThread(new Runnable() {
@Override
public void run() {
// Update UI elements here
}
});
Why use Handler? This approach ensures that your UI updates are safe and prevent crashes due to accessing the UI from non-UI threads.
Final Thoughts
Navigating the world of dynamic fragments in Java can be challenging but mastering these elements will immensely improve your application's robustness and user experience. By addressing fragment transactions, lifecycle events, data passing, configuration changes, and UI updates, you’ll build a far more reliable and interactive application.
For those looking to delve deeper into fragment management, I recommend checking out the article "Mastering Dynamic Fragments: Common Challenges Uncovered" at infinitejs.com/posts/mastering-dynamic-fragments-challenges. This article explores additional issues and offers solutions based on real-world scenarios.
By including these best practices and approaches in your development work, you’ll be better equipped to face common challenges and ensure a seamless user experience in your Java applications. Happy Coding!