Tackling Dynamic Fragmentation Issues in Java Applications
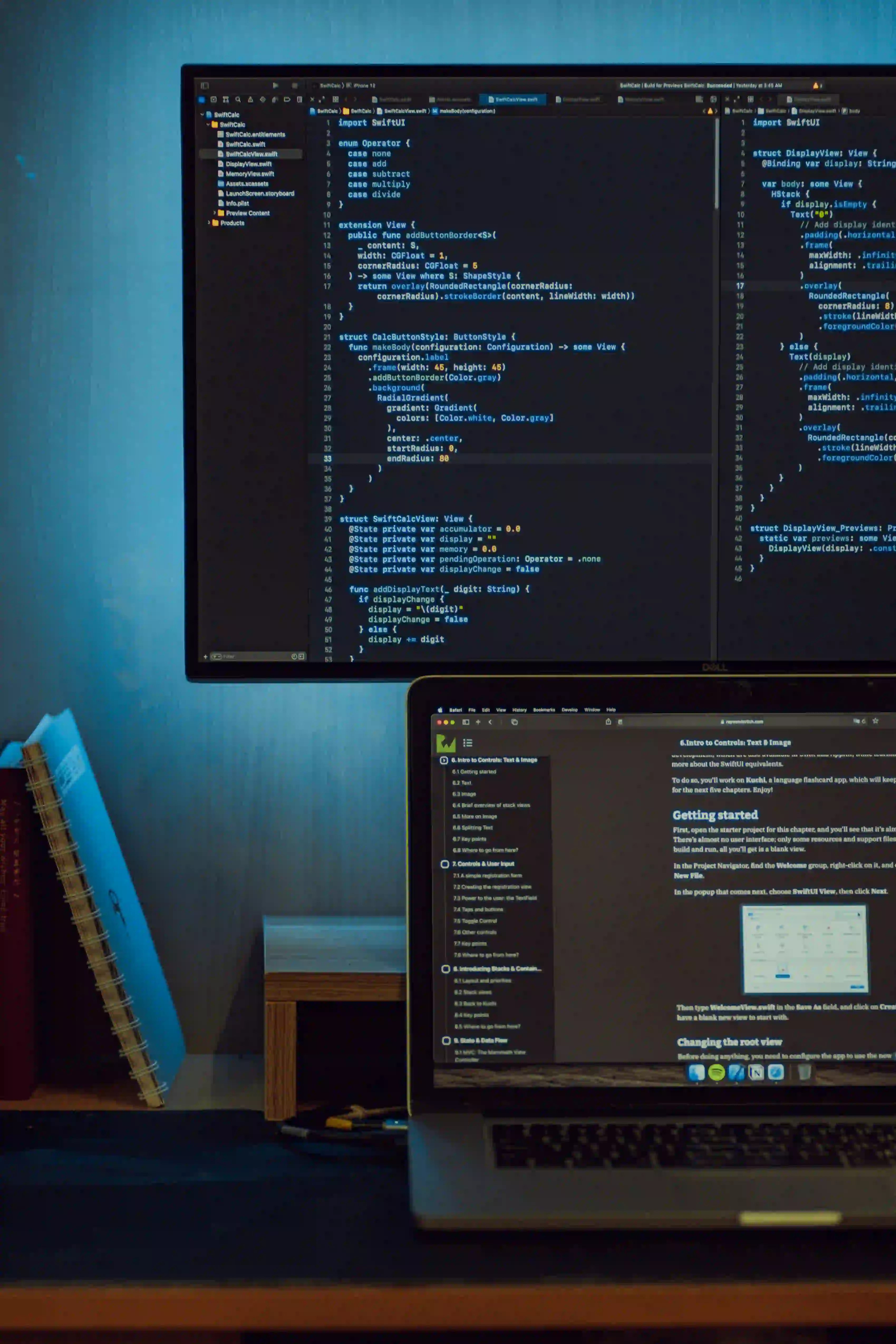
Tackling Dynamic Fragmentation Issues in Java Applications
Dynamic fragmentation is a critical issue in the realm of Java applications that can significantly impact performance and memory utilization. In this blog post, we will delve into the root causes of dynamic fragmentation, discuss its effects, and explore effective strategies to mitigate these problems. Understanding these concepts is essential for any developer looking to create efficient, scalable applications.
What is Dynamic Fragmentation?
Dynamic fragmentation occurs when a program allocates and deallocates memory in a manner that leaves small, unusable blocks scattered throughout the heap space. This issue is particularly prevalent in applications that frequently allocate and free memory for objects. Over time, this fragmentation can lead to wasted memory and performance degradation.
The Impact of Dynamic Fragmentation
-
Increased Memory Usage: As memory becomes fragmented, the system may have to allocate more memory than necessary, leading to higher resource consumption.
-
Slower Performance: Fragmentation can slow down memory allocation processes because the memory manager has to sift through these scattered blocks to find a suitable allocation.
-
OutOfMemoryError: In severe cases, fragmented memory can lead to
OutOfMemoryError
, where the application fails to allocate the necessary memory despite available total heap memory.
To illustrate the importance of tackling dynamic fragmentation, consider this anecdote: a leading e-commerce platform encountered significant performance issues during peak shopping seasons. Upon further investigation, the team discovered that dynamic fragmentation in their Java application was the root cause, leading them to refactor their memory management strategy.
Understanding Memory Management in Java
Java has built-in memory management through its Garbage Collection (GC) mechanism. However, the way that memory is allocated and deallocated can still lead to fragmentation. To understand this better, let's highlight how objects are created and cleaned up in Java:
public class DynamicMemory {
public static void main(String[] args) {
// Allocate memory for objects
for (int i = 0; i < 100; i++) {
String str = new String("Fragmentation Issue " + i);
// Simulating different usage patterns
if (i % 3 == 0) {
// Deallocating some objects
str = null; // Eligible for garbage collection
}
// Imagine further processing here
}
}
}
Why This Matters
In the code above, we allocate a new String object in a loop. After every third iteration, we nullify the reference, which allows the GC to clean it up. As memory is dynamically freed and allocated, it may lead to fragmented space in between - hence, the dynamic fragmentation issue arises.
Strategies to Mitigate Fragmentation
- Minimize Object Creation: One effective way to combat fragmentation is by reducing the frequency of object creation. Instead, use object pooling or consider immutability, where applicable:
import java.util.ArrayList;
import java.util.List;
public class ObjectPool {
private final List<String> pool = new ArrayList<>();
public String acquireObject() {
if (pool.isEmpty()) {
return new String();
}
return pool.remove(pool.size() - 1);
}
public void releaseObject(String obj) {
pool.add(obj);
}
}
In the code above:
- Object Pooling offers a way to reuse objects rather than constantly creating new ones. This can help maintain a healthier memory state and minimize fragmentation.
-
Use Appropriate Data Structures: Select data structures that suit your requirements. For example,
ArrayList
might lead to fragmentation if utilized inadequately; utilizing aLinkedList
could be beneficial for frequent additions and removals. -
Monitor Garbage Collection: Keep an eye on GC logs to understand memory behavior in your application. Enhance your GC configurations to find a good balance between throughput and pause times.
Advanced Techniques
For sophisticated Java applications, you may consider:
-
Custom Memory Allocators: These allocators can ensure memory blocks are filled efficiently, reducing fragmentation.
-
Off-Heap Memory Management: Tools like
Apache Arrow
or libraries likenetty
allow dealing with off-heap memory, which can be more manageable than the traditional Java heap.
For a deeper insight into managing fragmentation specifically in dynamic environments, check out the article titled Mastering Dynamic Fragments: Common Challenges Uncovered. Understanding these strategies can further aid developers in minimizing dynamic fragmentation issues.
To Wrap Things Up
Dynamic fragmentation poses a considerable challenge in Java applications, affecting both memory utilization and performance. By understanding its causes, implementing efficient code practices, and monitoring memory usage effectively, Java developers can significantly reduce the impact of fragmentation.
Whether through minimizing object creation, employing appropriate data structures, or even considering advanced memory management solutions, there are numerous strategies developers can adopt to mitigate the effects of dynamic fragmentation.
By prioritizing memory management, you can ensure that your Java applications perform optimally, even under heavy loads. Remember to stay informed and continuously refine your approach as best practices evolve in the ever-changing landscape of software development.
Additional Resources
Engage with these resources to enhance your understanding of Java and its memory management capabilities. Happy coding!