Enhancing Java Applications with Django for Data Science
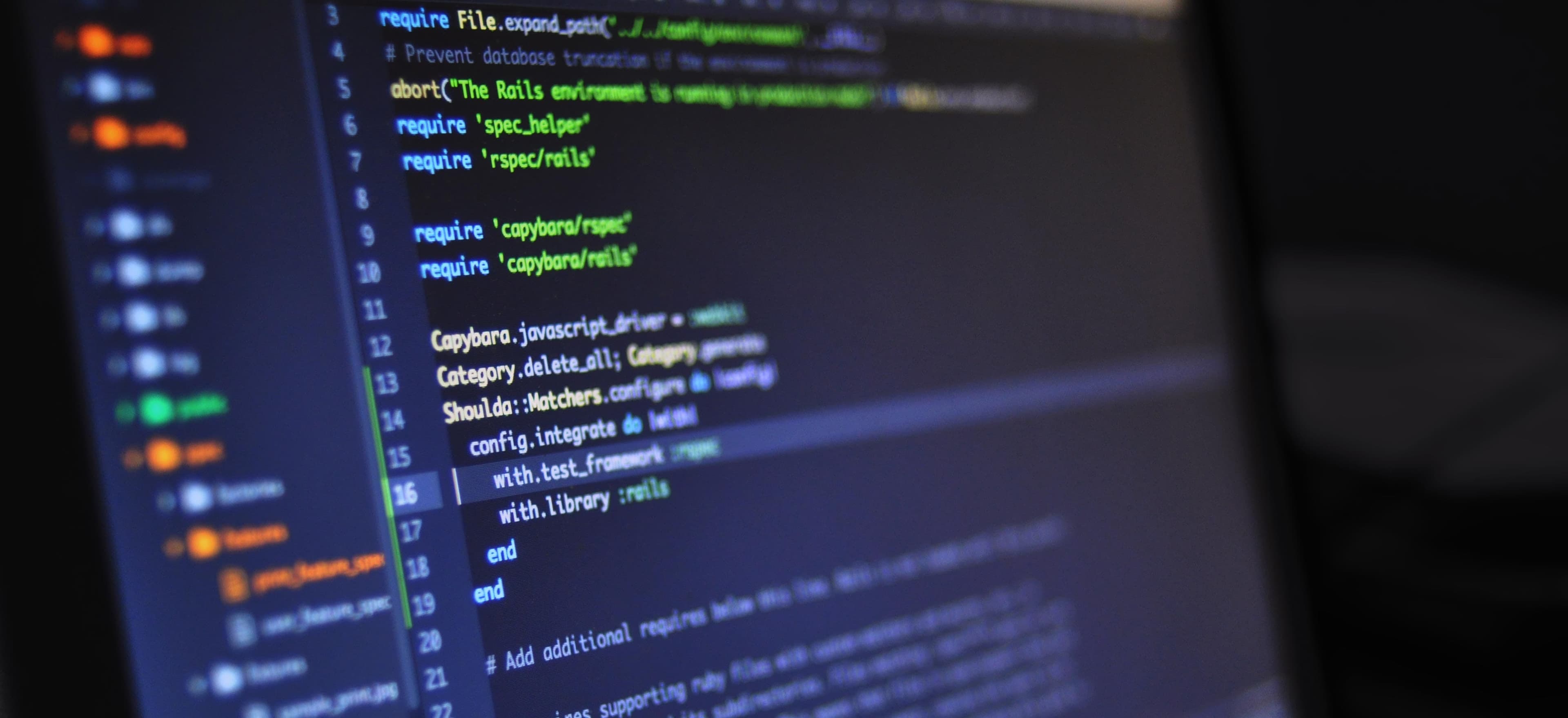
- Published on
Enhancing Java Applications with Django for Data Science
In the evolving landscape of software development, integrating multiple technologies is essential for creating robust applications. One significant combination that has been gaining attention recently is the synergy between Java applications and Django for data science projects. This article focuses on enhancing Java applications using Django, highlighting the advantages and providing practical insights on how to implement this integration effectively.
Understanding the Technologies
Java Overview
Java is a high-level, versatile programming language that is widely used for building enterprise-level applications. Its robustness, security features, and rich ecosystem make it a preferred choice among developers. Whether it's web applications, mobile apps, or backend services, Java's capabilities are nearly limitless.
Django Overview
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It is particularly favored in data science due to its simplicity and the ability to integrate machine learning libraries effortlessly. With its comprehensive features such as an ORM, templating engine, and built-in admin panel, Django is an excellent choice for developing data-oriented applications.
The Need for Integration
With the rise of data science and machine learning, many Java applications are seeking to leverage powerful data analysis and visualization capabilities. However, building these functionalities directly in Java can often be complex and resource-intensive. This is where Django shines.
Using Django to handle the data science aspect allows developers to harness Python's extensive libraries, such as Pandas, NumPy, and Matplotlib, while still maintaining the core Java application. This integration can streamline workflows and enhance the overall functionality of your application.
Key Advantages of Integrating Java with Django
-
Seamless Data Science Capabilities: By integrating Django, Java applications can utilize advanced data science libraries that are not natively available in Java. This opens the door to powerful analysis and machine learning models.
-
Rapid Development: Django's philosophy of "batteries included" allows for faster development cycles. You can prototype rapidly using Django, and then integrate this prototyping with your Java application.
-
Enhanced Frontend Options: Django's templating system makes it easy to render complex data visualizations that Java alone may find cumbersome.
-
Improved Collaboration: Data scientists and Java developers can work more collaboratively. Data scientists can focus on building models in Python while Java developers maintain their robust applications.
-
Microservices Architecture: The integration supports the microservices architecture, where you can run Django and Java applications independently, communicating through APIs.
Practical Steps to Integrate Django with Java
Now that we understand the benefits, let’s discuss how to integrate Django with Java applications effectively.
Step 1: Setting Up Your Django Application
Firstly, ensure that you have Django installed. You can create a new Django project by executing the following commands:
# Install Django if not already installed
pip install Django
# Create a new Django project
django-admin startproject mydataapp
Next, navigate into your project’s directory:
cd mydataapp
Then, create the necessary application for your data processing:
python manage.py startapp data_processor
Step 2: Creating APIs with Django
For your Java application to communicate with Django, you'll need to expose APIs. This can be done using Django Rest Framework (DRF). Install it by running:
pip install djangorestframework
You can create a simple API view in your data_processor/views.py
:
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
class DataAnalysisAPIView(APIView):
def post(self, request):
# Extract data from the request
input_data = request.data.get('input_data')
# Perform some data analysis using Python libraries
analysis_result = ... # Your analysis logic here
return Response({"result": analysis_result}, status=status.HTTP_200_OK)
Step 3: Configuring URL Routing
Configure your URL routing in data_processor/urls.py
to point to your new API view:
from django.urls import path
from .views import DataAnalysisAPIView
urlpatterns = [
path('analyze/', DataAnalysisAPIView.as_view(), name='data_analysis'),
]
Then, include this URL configuration in your main urls.py
file:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include('data_processor.urls')),
]
Step 4: Making HTTP Requests from Java
Now that your Django API is set up, it's time to consume this API from your Java application. Below is an example using the HttpURLConnection
class available in Java:
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class DataAnalysisClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8000/api/analyze/");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setDoOutput(true);
String inputJson = "{\"input_data\": \"your_data_here\"}";
try (OutputStream os = conn.getOutputStream()) {
byte[] input = inputJson.getBytes("utf-8");
os.write(input, 0, input.length);
}
if (conn.getResponseCode() == HttpURLConnection.HTTP_OK) {
// Handle success response
System.out.println("Data analysis request sent successfully.");
} else {
System.out.println("Request failed with code: " + conn.getResponseCode());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Step 5: Testing the Integration
Once you have both your Django server and Java application running, you can test the entire setup. Use the following command to start your Django server:
python manage.py runserver
Then execute your Java application. You should see a response indicating whether your request was successfully processed.
Final Thoughts
Integrating Django into your Java applications not only enhances data processing capabilities but also allows you to tap into the vast Python ecosystem for data science. This partnership fosters efficiency and is ideal for projects requiring robust backend services alongside sophisticated data analysis.
For further insights on how Django can boost your data science projects, refer to our previous article, "Boosting Data Science Work with Django: Key Benefits" at configzen.com/blog/boosting-data-science-with-django-benefits.
By following the practical steps outlined in this guide, you can effectively leverage Python's data science capabilities while maintaining a strong Java application foundation. The result? An efficient, powerful application ready to tackle the demands of modern data science.
Checkout our other articles