Leveraging Java for Data Science: Django vs. Spring Boot
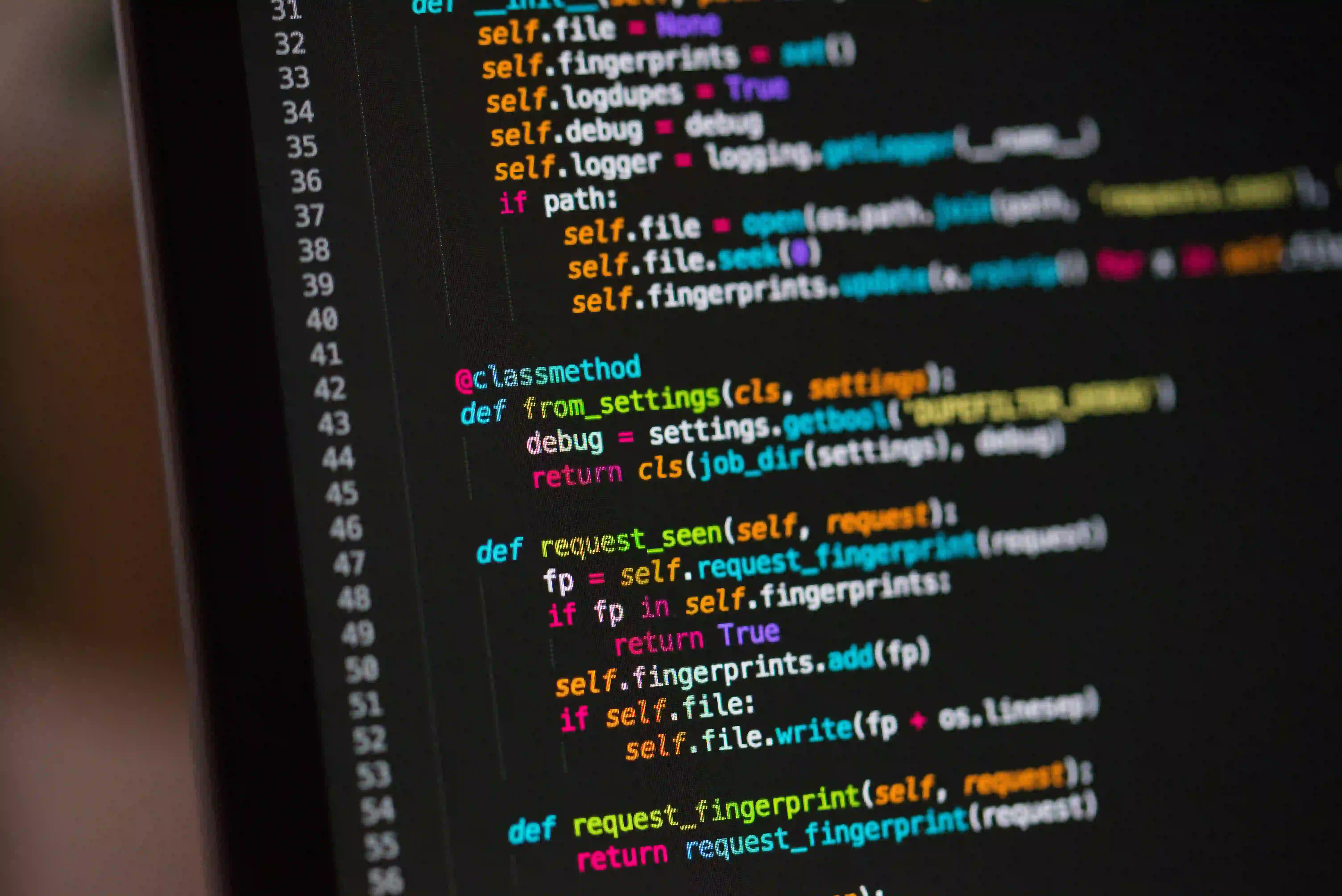
Leveraging Java for Data Science: Django vs. Spring Boot
Data science is an evolving field that requires robust tools and frameworks for efficient management and analysis of data. While Python has often been lauded as the go-to language for data science due to its simplicity and powerful libraries, Java has emerged as a strong contender, especially in enterprise-level applications. This blog post aims to compare two popular frameworks—Django and Spring Boot—in the context of data science, focusing on how Java can be leveraged effectively alongside Python.
Understanding Django and Spring Boot
Django: The Python Powerhouse
Django is a high-level Python web framework that promotes rapid development and clean, pragmatic design. It encourages developers to build robust applications quickly without sacrificing quality. One of its primary strengths is in its ability to handle database-driven applications efficiently, making it a go-to choice for data science applications.
If you're interested in understanding the benefits of Django specifically for data science, you might want to check out the article titled Boosting Data Science Work with Django: Key Benefits.
Spring Boot: The Java Juggernaut
On the flip side, Spring Boot is an extension of the Spring framework that simplifies the development of new Java applications. It offers a microservices architecture and is designed to make it easy to create stand-alone, production-grade Spring-based applications. With its robustness and scalability, Spring Boot can be an effective framework for developing data-centric applications.
Comparison of Django and Spring Boot for Data Science
Performance
When it comes to performance, Java applications generally outrun those written in Python due to Java's Just-In-Time (JIT) compilation and lower-level access to system resources. In data science, where performance can significantly impact the efficiency of data processing and analysis, Spring Boot can leverage Java's performance benefits.
Code Example: Simple REST API in Spring Boot
@RestController
public class DataController {
@GetMapping("/data")
public ResponseEntity<List<Data>> getAllData() {
List<Data> dataList = dataService.getAllData();
return new ResponseEntity<>(dataList, HttpStatus.OK);
}
}
In this snippet, we create a simple REST API using Spring Boot. The framework handles various aspects like routing and response handling, enabling quick deployment and scalability.
Ecosystem and Libraries
Python's rich ecosystem of libraries for data science, including NumPy, Pandas, and TensorFlow, makes it exceedingly compelling for data manipulation and machine learning. However, Java is not devoid of powerful libraries. Libraries like Weka, Deeplearning4j, and Apache Spark offer comparable functionalities.
Code Example: Reading Data with Apache Spark in Java
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class DataReader {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("Data Reader")
.master("local")
.getOrCreate();
Dataset<Row> dataFrame = spark.read().json("data.json");
dataFrame.show();
}
}
In this example, we use Apache Spark to read a JSON file. Spark allows distributed data processing, which is vital for handling massive datasets that are common in data science.
Community Support and Learning Curve
Django undeniably boasts a more straightforward learning curve, particularly for developers familiar with Python. This makes it an excellent option for data professionals who are transitioning from analytics to application development.
On the other hand, Spring Boot has a steeper learning curve but offers rich documentation and a strong community. The investment in learning Spring Boot can pay off, especially for complex applications requiring diverse functionalities.
Scalability
One of the critical factors for any data-driven application is scalability. Spring Boot shines in this department due to its microservices architecture, allowing developers to build applications that can grow as needed. Django can scale but often requires more effort to manage dependencies and interactions between components as the application grows.
Security
Data security is paramount in data science, especially when handling sensitive information. Both Django and Spring Boot have robust security features. Django comes with built-in protections against common vulnerabilities like SQL injection and cross-site scripting. Spring Boot also provides excellent security features when integrated with the Spring Security module, giving developers the tools to secure their applications effectively.
Best Practices for Data Science Applications in Java
Opt for Microservices Architecture
Using a microservices architecture with Spring Boot can significantly enhance the scalability and maintainability of data science applications. Developing each component of your application as independent services allows for more effortless updates and scaling.
Code Example: Microservice Configuration in Spring Boot
spring:
application:
name: data-service
server:
port: 8080
This configuration file showcases how simple it is to set up a Spring Boot microservice. Each service can manage its data independently, thereby improving both performance and complexity management.
Monitor and Optimize
Data science applications often run long workloads. Employ monitoring tools to keep track of resource usage and performance bottlenecks. Using Java's built-in monitoring tools or third-party ones like Prometheus can provide insights into your application’s efficiency.
Collaborate with Data Scientists
Encouraging collaboration between developers and data scientists can lead to better application performance and more relevant data workflows. By understanding each other's requirements and challenges, teams can design applications that are both functional and tailored to specific data science needs.
Closing Remarks
While Python remains a dominant force in data science, Java and frameworks like Spring Boot present viable alternatives for enterprise-level applications. Their performance, scalability, and ecosystem of libraries can suit various data-related tasks that demand reliability and efficiency.
As data science continues to evolve, familiarizing oneself with multiple languages and frameworks will be beneficial. Whether you focus on Django or Spring Boot, each has unique strengths that can channel your data science endeavors into successful outcomes.
For further insights into utilizing Django for data science, be sure to read Boosting Data Science Work with Django: Key Benefits.