Tackling Java Framework Challenges: Lessons from HydePHP
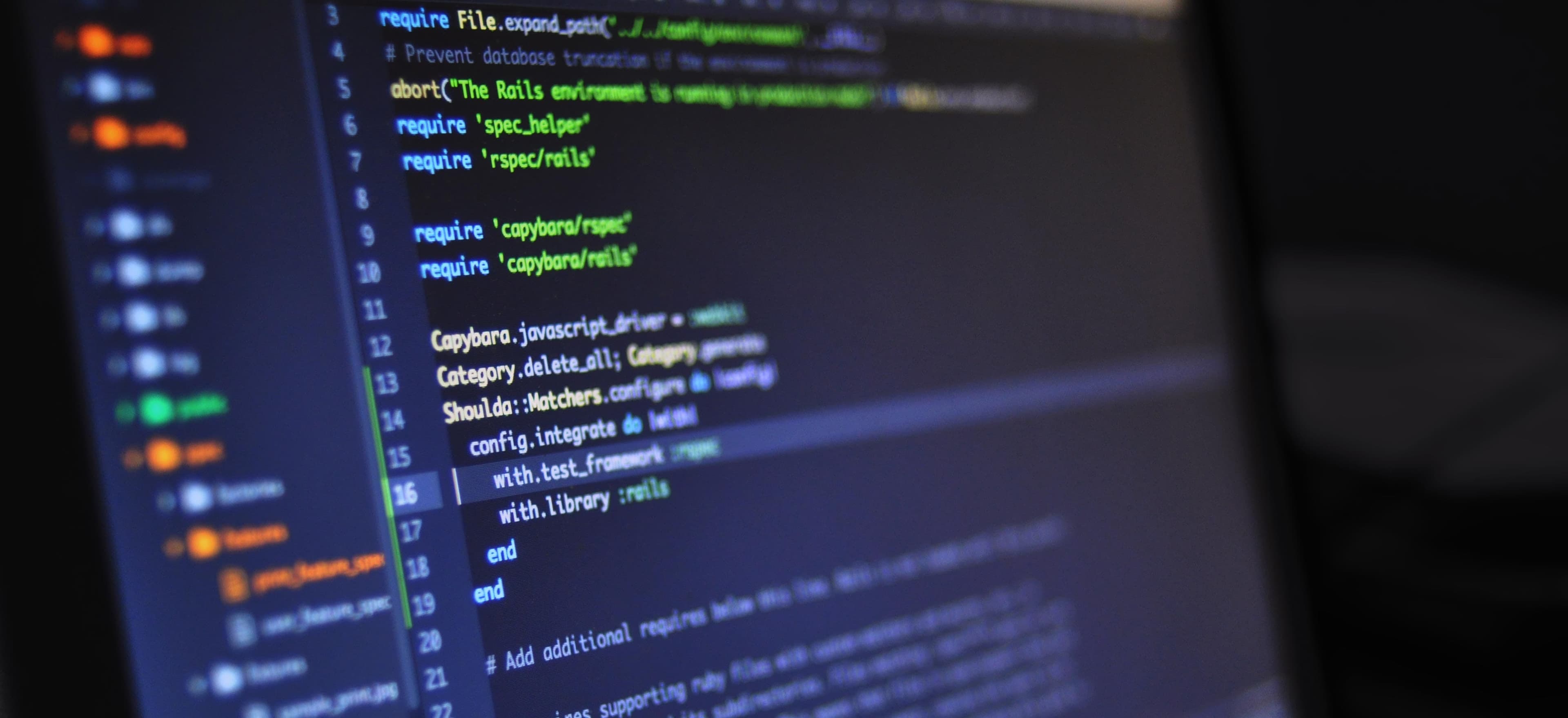
- Published on
Tackling Java Framework Challenges: Lessons from HydePHP
As a developer, you face numerous challenges while working with any programming framework. Often these challenges are compounded by the complexity and variety of frameworks available today. The rise of Java as a versatile, object-oriented programming language has led to the development of numerous frameworks, each with its strengths and weaknesses. While Java frameworks like Spring, Hibernate, and JavaServer Faces (JSF) are powerful, they are not without hurdles.
Interestingly, many of the challenges faced in Java development echo those encountered in other languages, including PHP. A prime example of this can be seen in the article titled Overcoming Common Challenges with HydePHP's First Release, where the HydePHP framework confronts various obstacles during its development phase. Here, we will explore some of these challenges in the context of Java frameworks and highlight lessons that can be learned and applied across languages.
Understanding Framework Challenges
Framework challenges can be broadly categorized into three groups:
-
Usability Issues: Confusing APIs, unclear documentation, and lack of proper tools can drastically hinder development productivity.
-
Performance Bottlenecks: Sometimes frameworks can introduce performance overhead which could affect the speed of application execution.
-
Community Support: New frameworks sometimes lack adequate community support, which can lead to fewer resources for troubleshooting and sharing knowledge.
Usability Issues
Usability is paramount in determining how quickly developers can adapt to a framework. Java frameworks, like any other frameworks, come with intricate setups that may not always be straightforward.
Example: Spring Framework Setup
Consider the Spring Framework. The initial configuration can be daunting for newcomers. Let's look at a basic setup of a Spring application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Why This Matters: The @SpringBootApplication
annotation simplifies the configuration by combining multiple annotations. However, this abstraction may obscure what is happening behind the scenes. Understanding how these annotations work is vital for debugging and extending functionality later on.
It's equally important to pair such configurations with solid documentation. Clear guidelines, such as those found in Spring's official documentation, can help mitigate usability concerns.
Performance Bottlenecks
Performance is critical in application development. Frameworks can unintentionally introduce inefficiencies. For instance, Hibernate, a popular ORM in Java, is known for its efficiency but can suffer from performance issues if not configured correctly.
Example: Hibernate Session Management
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
// Your code to persist an object
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
} finally {
session.close();
}
Why This Matters: The key here is to understand session management in Hibernate. Not closing sessions can lead to memory leaks, while unnecessary session opening and closing can lead to increased latency. Therefore, proper session handling is fundamental to maintaining application performance.
Community Support
The strength of a framework can often be judged by the size of its community. A robust community provides resources, plugins, and tools that can substantially enhance productivity.
Bridging the Gap
The HydePHP framework's article discusses the challenges they faced in garnering initial community support. This problem can be likened to frameworks like Play or Vert.x in the Java ecosystem.
To address these issues, developers can contribute to community efforts. Whether that means writing blogs, creating tutorial videos, or answering queries in forums, every bit helps build a knowledge base. Utilizing platforms like Stack Overflow ensures that you can find answers while at the same time contribute back by sharing your solutions.
Addressing Framework Challenges
Based on our examination of the issues faced in Java frameworks and insights drawn from HydePHP, let's discuss some solutions that can alleviate these challenges.
Improved Documentation
This might seem straightforward, but clear, well-structured documentation is often overlooked. Developers should receive comprehensive guides, code examples, and best practices linked directly to API references.
Performance Monitoring Tools
Integrating performance monitoring tools can significantly enhance the visibility of your applications. By employing tools such as JMeter or New Relic, developers can gauge where performance can be optimized.
Community Engagement
Actively engaging with your preferred framework's community can yield significant advantages. Join forums, participate in meetups, or contribute to open-source projects. This strategy will keep you updated and plugged into the latest developments.
Closing Remarks
In the fast-evolving landscape of programming frameworks, understanding challenges and applying lessons learned is invaluable. The principles we’ve drawn from HydePHP's experience are equally applicable within the scope of Java development.
By making conscious choices in usability, performance considerations, and engaging with the community, developers can create remarkable applications that are not only functional but also efficient.
For further insights into overcoming common challenges with frameworks, I highly recommend reading the article Overcoming Common Challenges with HydePHP's First Release.
Happy coding!
Checkout our other articles