Overcoming TDD Hurdles in Java Development Projects
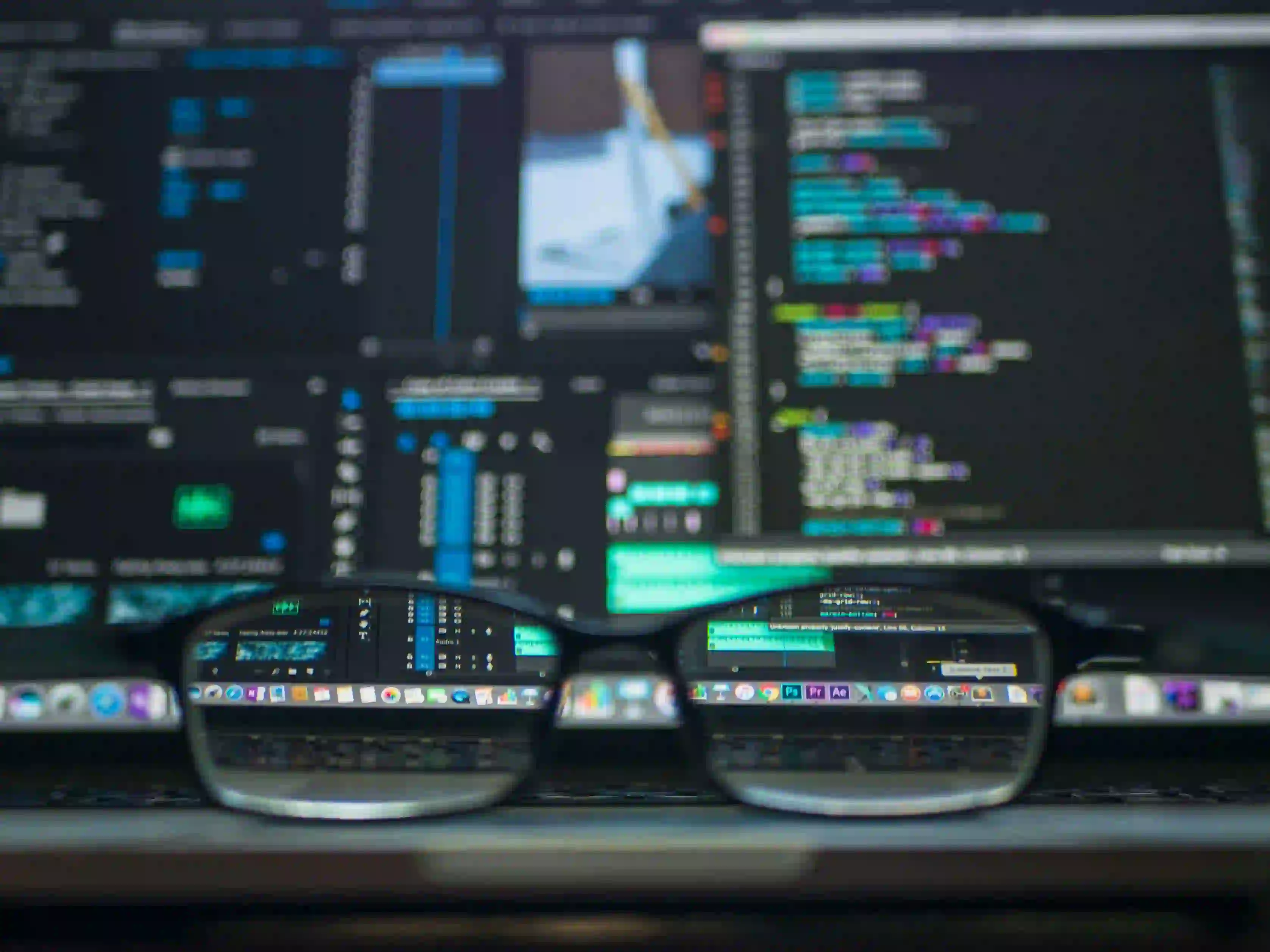
Overcoming TDD Hurdles in Java Development Projects
Test-Driven Development (TDD) is a robust approach to software development that emphasizes writing tests before writing the actual code. This technique, when implemented correctly, can lead to cleaner, more reliable, and maintainable code. However, as with any methodology, TDD comes with its own set of challenges, particularly in Java development projects. This article will explore some common hurdles faced in TDD and how to effectively navigate them to enhance your development workflow.
What is Test-Driven Development?
TDD is a development practice where you write a test for a piece of code before you write the code itself. It follows a simple cycle known as Red-Green-Refactor:
- Red: Write a failing test for the new functionality.
- Green: Write the minimal code necessary to pass the test.
- Refactor: Clean up the code while ensuring all tests still pass.
By adhering to this cycle, developers can maintain a focus on producing high-quality software.
Common Challenges in TDD
-
Time Commitment
One of the most frequent complaints about TDD is the time it requires. Developers often feel that writing tests consumes valuable time that could be used to write production code. This can be misleading. While it may seem time-consuming initially, TDD can lead to significant time savings in the long run by preventing bugs and reducing the amount of debugging required.
-
Understanding Requirements
Writing effective tests requires a deep understanding of the requirements. If requirements are vague or constantly changing, it can lead to frustrations in the TDD process. A well-defined requirements document can mitigate this issue. Additionally, engaging stakeholders in the development process can provide clarity.
-
Complex Codebase
When working within a complex Java codebase, writing tests for existing code can be daunting. Legacy code typically lacks tests, making it challenging to ensure that new tests do not introduce issues. Refactoring code to incorporate TDD can be tricky but is often necessary for cleaner, more manageable code.
-
Testing Third-Party Libraries
Reliance on third-party libraries can present challenges in writing tests. Developers may find themselves needing to stub or mock the library's functionality, which can complicate the testing process. Tools like Mockito can be used effectively to create mocks in your tests.
☕snippet.javaimport static org.mockito.Mockito.*; public class UserServiceTest { private UserService userService; private UserRepository userRepository; @Before public void setUp() { userRepository = mock(UserRepository.class); userService = new UserService(userRepository); } @Test public void testAddUser() { User user = new User("Alice", "alice@example.com"); when(userRepository.save(user)).thenReturn(user); User savedUser = userService.addUser(user); assertEquals("Alice", savedUser.getName()); verify(userRepository).save(user); } }
In this example, Mockito is used to create a mock
UserRepository
so that theUserService
can be tested without needing to rely on the actual database implementation. This isolation ensures that our unit tests are straightforward and not influenced by external factors. -
Over-Testing vs. Under-Testing
Finding the right balance between over-testing and under-testing is crucial within TDD. Over-testing can lead to an abundance of tests that may not provide value, while under-testing can create gaps in coverage. Strive for adequate coverage, focusing on testing business logic rather than trivial setters and getters.
A common approach is to use metrics to evaluate the effectiveness of your tests. For example, integrating code coverage tools like JaCoCo makes it easy to track how much of your code is being tested.
-
Skills and Experience Level
Not all developers are familiar with TDD or its philosophies. An unmet skills gap can severely hinder your project. Investing in training for your team can help everyone align on TDD principles. Encourage a culture of pair programming, where experienced developers can guide less experienced team members through the TDD cycle.
Best Practices for Effective TDD in Java
-
Start Small
Begin with smaller classes or methods. This reduces complexity, making your tests simpler to write and easier to maintain.
-
Use a Good Testing Framework
JUnit is the de facto standard testing framework for Java. It provides structures for writing and executing tests efficiently. Make sure your team is familiar with its features.
☕snippet.javaimport org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; class CalculatorTest { @Test void testAddition() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
This snippet shows a simple unit test for an addition method. JUnit helps to set up assertions to verify the system behaves as expected.
-
Incorporate Continuous Integration
Incorporate a Continuous Integration (CI) pipeline to run your tests automatically upon each code change. CI tools like Jenkins or GitHub Actions can streamline this process, ensuring that testing is ongoing and consistent.
-
Refactor Regularly
Refactoring isn't just for cleaning up production code. It allows you to improve your tests too. Regularly revisiting your tests ensures they remain relevant and efficient.
-
Review and Retrospect
With each sprint or project phase, review your TDD processes. What worked? What didn’t? Engaging your team in retrospective discussions can offer insights and improve practices moving forward.
In Conclusion, Here is What Matters
In conclusion, while TDD can present distinct challenges for Java developers, it offers valuable benefits that can enhance the quality of your code. By acknowledging these hurdles and implementing best practices, developers can create a testing culture that emphasizes quality and efficiency. Ultimately, TDD is a powerful methodology that, when understood and executed properly, can lead to significant improvements in software development.
For those interested in diving deeper, consider checking out the article "Common Challenges Faced in Test-Driven Development" available at tech-snags.com/articles/common-challenges-test-driven-development. This resource elaborates on some of the issues mentioned here and offers further insights into effective TDD practices.
By employing the strategies outlined in this article, you can effectively overcome the hurdles of TDD and focus on crafting robust Java applications that stand the test of time.