Overcoming Test-Driven Development Hurdles in Java
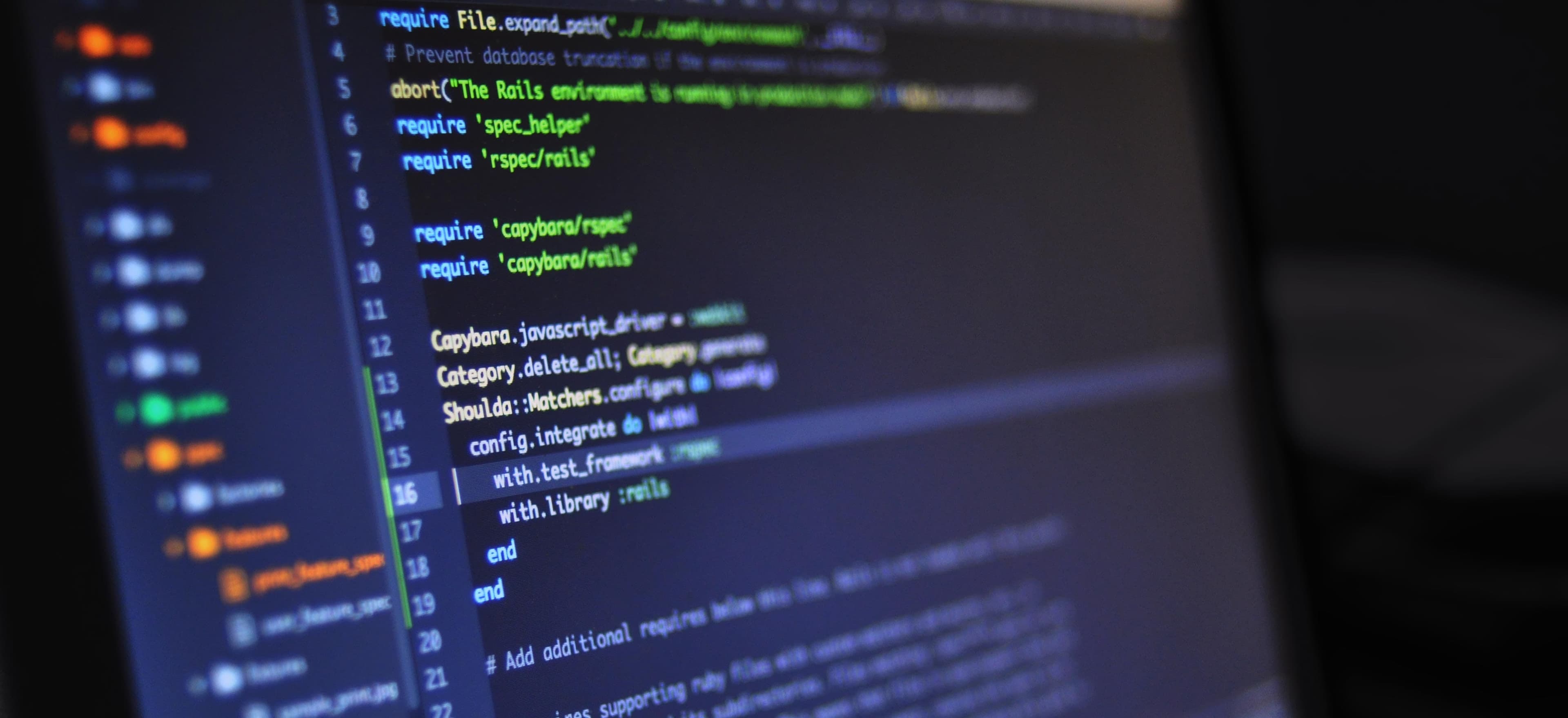
- Published on
Overcoming Test-Driven Development Hurdles in Java
Test-Driven Development (TDD) is a software development methodology that emphasizes writing tests before code. It can lead to cleaner code and fewer bugs. However, many developers encounter various challenges when adopting TDD, particularly in Java. In this blog post, we will explore common hurdles developers face in TDD and provide actionable strategies for overcoming them.
If you are interested in understanding the challenges faced during TDD, you may want to check out Common Challenges Faced in Test-Driven Development.
Understanding TDD Basics
Before diving deep into the challenges, it's important to clarify the basic steps of TDD. TDD follows a cycle often summarized as "Red, Green, Refactor":
- Red: Write a test for a new feature that fails (since the feature is not implemented yet).
- Green: Write the minimal amount of code needed to make the test pass.
- Refactor: Clean up the code while keeping the tests green.
Example: A Simple Calculator
To illustrate this concept, let's take the example of a simple calculator that supports basic operations like addition.
Step 1: Write the Test (Red)
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this example, we first write a failing test. The add
method doesn't exist yet, so this will trigger an error.
Step 2: Implement the Code (Green)
public class Calculator {
public int add(int a, int b) {
return a + b; // Minimal implementation
}
}
By implementing the add
method, we ensure that our test now passes.
Step 3: Refactor
At this stage, our simple implementation doesn't need refactoring since it's clean and simple. In more complex methods, this would involve removing duplications and enhancing readability.
Common Hurdles in TDD
While the methodology seems straightforward, many developers face hurdles that can hinder their progress. Below are some of the common challenges and their solutions.
1. Lack of Understanding of TDD Principles
The Problem
Many developers find it hard to grasp the "why" behind TDD. They might write tests but struggle to see the value in it.
The Solution
Educate yourself and your team about the benefits of TDD. Understanding that it results in fewer defects and a more maintainable codebase can help. Take the time to read articles or consume resources on TDD, such as the one referenced earlier.
2. Resistance to Change
The Problem
Transitioning to TDD requires a shift in mindset and workflow, which can be met with resistance from team members accustomed to traditional development methods.
The Solution
Start small. Implement TDD in a new feature or module without forcing the entire team to adopt it immediately. Create an environment where TDD can be adopted iteratively.
3. Difficulty in Writing Good Tests
The Problem
Not all tests are created equal. Writing meaningful tests that genuinely capture edge cases can be difficult, resulting in poor coverage.
The Solution
Focus on writing tests that cover various scenarios, including boundary cases and expected exceptions. Don't hesitate to refactor tests for clarity. For instance, ensure that your Calculator
class also handles erroneous inputs:
@Test(expected = IllegalArgumentException.class)
public void testAdditionWithNull() {
Calculator calculator = new Calculator();
calculator.add(null, 3); // Expecting an exception
}
4. Time Constraints
The Problem
TDD can initially consume more development time, leading some developers to skip writing tests in favor of quick feature deployment.
The Solution
Emphasize long-term gains over short-term wins. Good TDD practices can reduce time spent on debugging and fixing issues down the line.
To help mitigate time pressures, you can implement Continuous Integration (CI) processes that automatically run your tests. Tools like Jenkins or CircleCI help ensure that tests run every time code is pushed.
5. Complexity in Test Maintenance
The Problem
As the codebase grows, maintaining the tests can become cumbersome. Tests may become fragile, making it challenging to modify the code without breaking the tests.
The Solution
Regularly review and refactor both your code and your tests. Emphasize the importance of clear, concise tests. Consider employing mocking frameworks like Mockito to isolate units of code during testing.
import static org.mockito.Mockito.*;
import org.junit.Test;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User user = new User("Alice");
userService.createUser(user);
verify(mockRepo).save(user); // Verify that save was called on the mock
}
}
In the example above, we not only test functionality but also verify interactions with other objects, making our tests more robust.
6. Integration Testing Challenges
The Problem
Writing unit tests is one thing, but integration testing, which usually involves multiple modules, can quickly spiral into complexity.
The Solution
Look into leveraging integration testing frameworks such as Spring Test for Java applications. Moreover, always establish a clear strategy that defines what aspects of your application require integration testing and which can be efficiently tested through unit tests.
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
public class ApplicationIntegrationTest {
@Autowired
private ApplicationContext context;
@Test
public void testApplicationContext() {
assertNotNull(context); // Ensure the context loads successfully
}
}
The above code snippet shows how to conduct an integration test using Spring Boot. Integration testing helps you to understand how components work together, which is crucial for large applications.
Final Considerations
Transitioning to TDD can be a challenging journey, especially in Java, where the learning curve can include a deeper understanding of object orientation and design patterns. However, the benefit of cleaner, more maintainable code outweighs the initial hurdles.
By educating yourself about TDD, tackling resistance iteratively, focusing on meaningful tests, effectively managing time, maintaining clear tests, and planning for integration testing, you will be well on your way to successfully implementing TDD in your Java projects.
For those seeking to understand more about the barriers surrounding TDD, I encourage you to revisit Common Challenges Faced in Test-Driven Development. Happy coding!
Checkout our other articles