Addressing Java Framework Challenges with Fresh Solutions
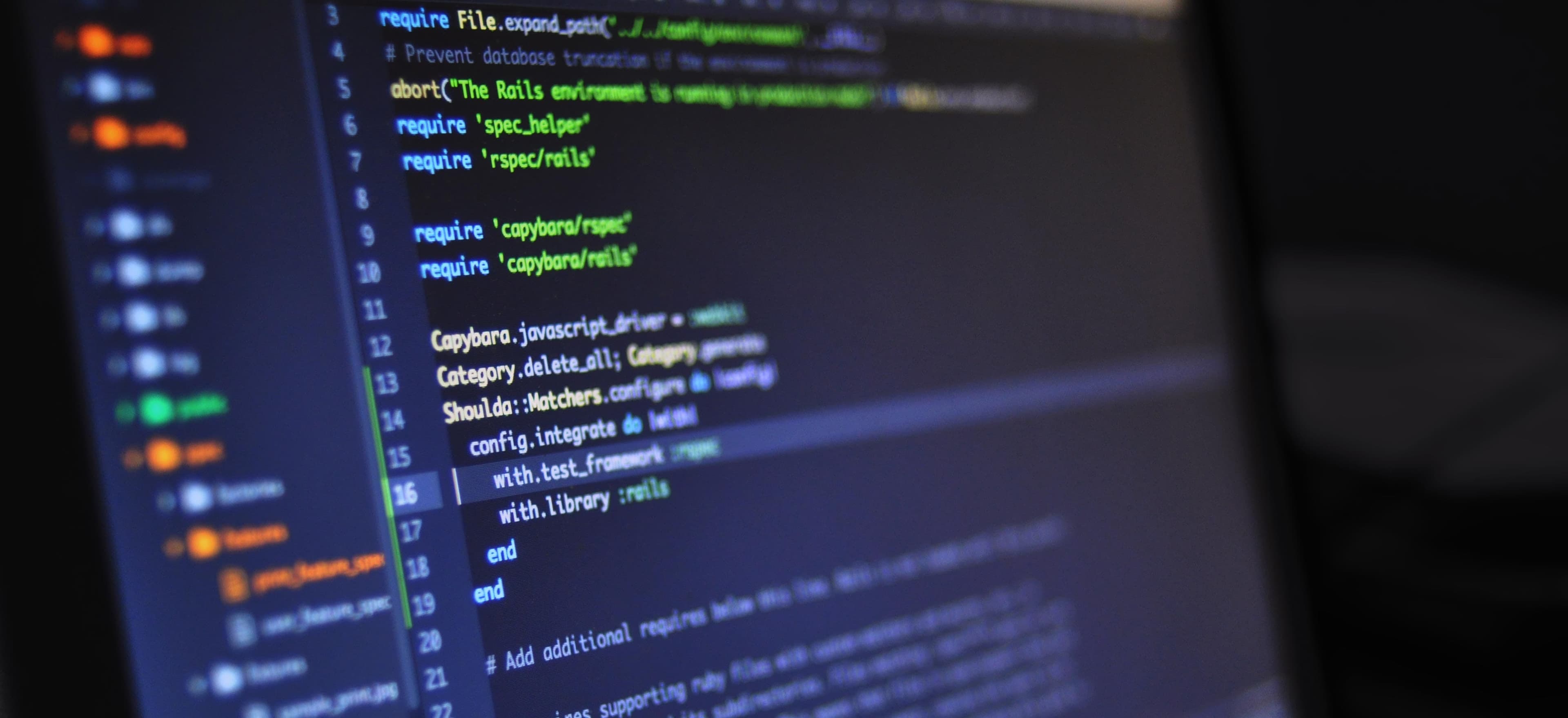
- Published on
Addressing Java Framework Challenges with Fresh Solutions
Java has been a stalwart in the world of programming for over two decades, powering countless applications and systems. Yet, with its popularity comes a variety of challenges that developers face continuously. Whether it's managing dependencies, maintaining version control, or ensuring performance, Java framework developers often seek innovative ways to navigate these hurdles.
This blog post will explore common issues developers encounter while using Java frameworks and provide fresh, pragmatic solutions. We'll analyze why these challenges arise and how to tackle them efficiently, all while utilizing solid code examples for clarity and understanding.
A Quick Overview of Java Frameworks
Before diving into the challenges, it’s crucial to understand what Java frameworks are. A framework provides a structure for application development, offering reusable code and predefined ways of building specific types of applications. Popular frameworks like Spring, Hibernate, and JavaServer Faces (JSF) streamline tasks and reduce development time.
Yet, as frameworks grow more robust, they often introduce complexities that require deeper knowledge and strategy to manage effectively.
Common Challenges and Fresh Solutions
1. Dependency Management
Issue
One of the most prominent challenges in using Java frameworks is dependency management. Conflicts can arise when libraries rely on different versions of the same dependency, leading to a phenomenon known as "JAR hell."
Solution
Using a build tool like Maven or Gradle can significantly alleviate dependency complications. They help in managing library versions and transitive dependencies in a clean manner.
<!-- Maven example: pom.xml -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.8</version>
</dependency>
In this snippet, the dependency for Spring Context is clearly defined, allowing Maven to resolve the necessary libraries automatically. Why use a build tool? It centralizes and simplifies dependency management.
2. Learning Curve and Complexity
Issue
Java frameworks often come with a steep learning curve, particularly when they possess intricate configurations. Developers new to a framework may find the documentation overwhelming and challenging to grasp.
Solution
Break down the learning process into modules. Focusing only on the necessary parts of a framework based on the project requirements can significantly increase retention and understanding. Also, consider utilizing Spring Boot, which simplifies application configuration.
// Spring Boot application main class
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
This snippet showcases Spring Boot's capability to configure applications with minimal code. Why is this beneficial? It reduces boilerplate code, allowing developers to concentrate on business logic instead of configuration details.
3. Performance Overheads
Issue
Heavy frameworks can introduce performance overheads, especially if they are not optimized for the specific application use case. Long startup times and increased memory consumption can stall development and affect application performance.
Solution
Profiling your application is crucial to identify bottlenecks. Tools like VisualVM or YourKit can help monitor application performance. Additionally, consider optimizing configuration settings specific to your development environment.
// Example of configuring Hibernate for performance
Properties properties = new Properties();
properties.put("hibernate.hbm2ddl.auto", "update");
properties.put("hibernate.dialect", "org.hibernate.dialect.MySQL5Dialect");
properties.put("hibernate.show_sql", "true");
In this example, optimized Hibernate properties are set to enhance performance. Why is tuning important? Proper configurations can drastically improve resource utilization, allowing applications to run more efficiently.
4. Lack of Community Support
Issue
Some frameworks may have limited support or community guidance. This can lead to challenges in troubleshooting and implementing best practices, particularly for niches or new technologies.
Solution
Engaging with community forums, such as Stack Overflow or the framework's official documentation pages, can yield answers to common issues. Regular updates on technology trends and community sharing can provide newer insights and solutions.
For instance, reading guide articles such as Overcoming Common Challenges with HydePHP's First Release can give developers a fresh perspective on handling similar challenges.
5. Security Vulnerabilities
Issue
Like all technologies, Java frameworks are prone to security issues, particularly if outdated versions of dependencies are used.
Solution
Regularly monitoring for vulnerabilities in your chosen frameworks is essential. Use tools such as OWASP Dependency-Check or Snyk to identify and rectify security flaws in libraries.
<!-- Maven dependency for OWASP Dependency-Check -->
<plugin>
<groupId>org.owasp.maven.plugins</groupId>
<artifactId>dependency-check-maven</artifactId>
<version>6.1.6</version>
<configuration>
<failBuildOnCVSS>7</failBuildOnCVSS>
</configuration>
</plugin>
This configuration enables OWASP Dependency-Check in a Maven project, automatically checking for critical security vulnerabilities. Why is proactive security critical? It ensures a safer coding environment and protects sensitive data.
6. Testing Complexity
Issue
Many developers struggle with testing their Java applications due to the complexity of interacting frameworks. Unit, integration, and functional tests can become convoluted, slowing down the development process.
Solution
Utilizing testing frameworks like JUnit together with Mockito can help streamline the process for unit testing.
import static org.mockito.Mockito.*;
public class UserServiceTest {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
@Test
public void testFindUserById() {
when(userRepository.findById(1)).thenReturn(new User("John"));
User user = userService.findUserById(1);
assertEquals("John", user.getName());
}
}
In this snippet, Mockito is utilized to create a mock UserRepository, simplifying the testing process. Why is mocking useful? It allows for isolated testing of service logic without requiring complex database interactions.
Key Takeaways
Navigating the complexities of Java frameworks doesn't have to be an insurmountable task. By leveraging tools, breaking learning curves into manageable parts, and optimizing processes, developers can address common challenges effectively.
As technology evolves, new frameworks and methodologies will arise, and we should always be willing to learn and adapt. Whether you are just beginning your journey in Java frameworks or are an experienced developer, remember that solutions to these challenges often lie within the community and the vast resources available online.
For more insights and strategies, don't forget to check out Overcoming Common Challenges with HydePHP's First Release. Happy coding!