Mastering Java Streams for Efficient Database Queries
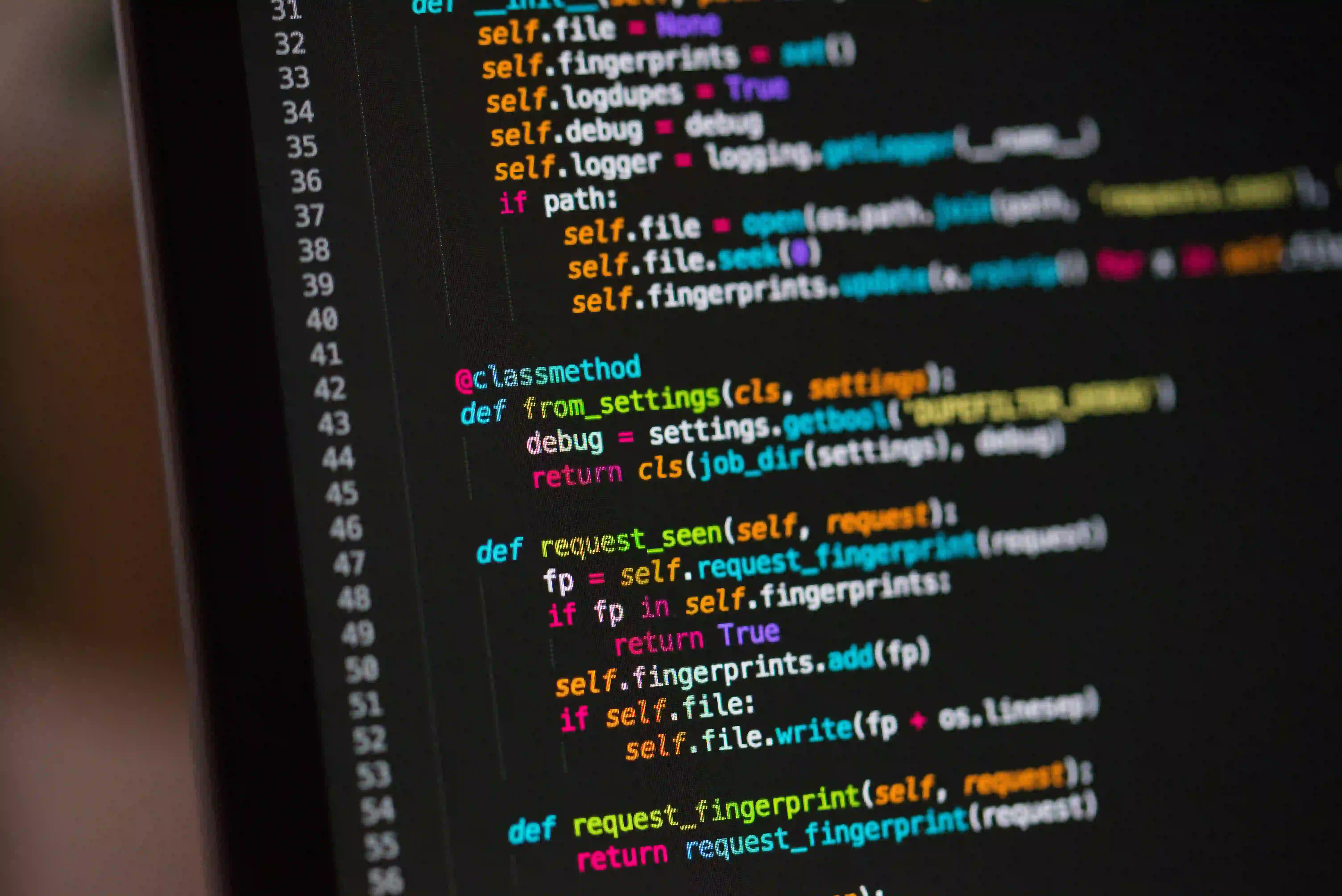
Mastering Java Streams for Efficient Database Queries
Java Streams, introduced in Java 8, revolutionized the way developers handle collections of data. They provide an elegant and efficient way to process sequences of elements. Besides improving code readability, they also allow for the execution of complex queries in a functional style. In this blog post, we'll explore how to harness the power of Java Streams for efficient database queries, including practical code snippets and explanations.
What Are Java Streams?
Java Streams represent a sequence of elements that can be processed in a functional style. They provide an abstraction over the underlying data source, enabling operations such as filtering, mapping, and reducing. Unlike collections, streams do not store data themselves; they operate on data from a source (like a collection, array, or I/O channel).
Benefits of Using Java Streams
- Conciseness: Streams allow you to express complex data processing tasks in a more readable format.
- Parallelism: Stream operations can be easily parallelized, taking advantage of multi-core processors.
- Laziness: Streams support lazy evaluation, meaning they compute values only when necessary.
Setting Up Java Streams with Databases
Before diving into code, ensure you have the following dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
For this demonstration, we'll be using H2 as our in-memory database.
Example Setup
Let's imagine we have a simple entity class representing a Customer
.
@Entity
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Constructors, Getters, and Setters
public Customer(String name, String email) {
this.name = name;
this.email = email;
}
}
We'll also create a repository interface for CRUD operations.
public interface CustomerRepository extends JpaRepository<Customer, Long> {
List<Customer> findByEmailEndingWith(String domain);
}
Fetching Data with Java Streams
Using Java Streams allows us to easily filter, sort, and manipulate the data obtained from the database. Let's consider a scenario where we want to find customers whose email addresses end with a specific domain.
Here's how we can implement this with Streams:
@Autowired
private CustomerRepository customerRepository;
public List<Customer> findCustomersByEmailDomain(String domain) {
return customerRepository.findAll()
.stream()
.filter(customer -> customer.getEmail().endsWith(domain))
.collect(Collectors.toList());
}
Breakdown:
customerRepository.findAll()
: Retrieves all customers from the database..stream()
: Converts the list into a Stream, allowing us to use Stream operations..filter(...)
: Filters customers based on their email domain..collect(Collectors.toList())
: Collects the filtered data back into a List.
Why Use Streams Here?
Streams can significantly simplify the logic. In the past, you would have needed a loop and conditionals to achieve the same result, which could lead to more boilerplate code. With Streams, the logic is clearer and more maintainable.
Advanced Filtering and Mapping
Imagine we want not only to filter customers but also transform their data. For instance, we may want to extract just the names of customers whose emails match a specific domain.
public List<String> findCustomerNamesByEmailDomain(String domain) {
return customerRepository.findAll()
.stream()
.filter(customer -> customer.getEmail().endsWith(domain))
.map(Customer::getName)
.collect(Collectors.toList());
}
Explanation
.map(Customer::getName)
: This transforms the stream ofCustomer
objects into a stream of Strings representing customer names, enabling further processing or direct presentation.
Handling Optional Values in Streams
When working with databases, it's common to encounter nullable values. Streams can gracefully handle Optional
types, making your code more reliable and expressive.
Here’s how to fetch a customer by their ID if it exists:
public Optional<Customer> getCustomerById(Long id) {
return customerRepository.findById(id)
.stream()
.findFirst();
}
Key Points
findById(id)
returns anOptional<Customer>
, which is a container that may or may not hold a value..stream()
: Converts theOptional
into a Stream that can be further processed.
If the customer exists, getCustomerById
will return a non-empty Optional
. If not, it will return an empty one. This eliminates the need for null checks, resulting in cleaner code.
Performance Considerations
Java Streams can significantly improve the performance of your application, especially when dealing with large datasets. However, it's crucial to be mindful of how you use them.
- Parallel Streams: You can easily switch to parallel processing for CPU-intensive operations.
return customerRepository.findAll()
.parallelStream()
.filter(customer -> customer.getEmail().endsWith(domain))
.map(Customer::getName)
.collect(Collectors.toList());
-
Lazy Evaluation: Streams are lazily evaluated, meaning that the data isn’t processed until it is necessary, thus reducing unnecessary computations.
-
Avoid Side Effects: Streams are best suited for stateless operations. Avoid using mutable objects or shared state, as it can complicate parallel execution and lead to errors.
Integrating Streams with Spring Data JPA
If you're using Spring Data JPA, you can perform queries directly utilizing the Stream API. For example, this method will apply an aggregation based on the first letter of customer names:
public Map<Character, List<Customer>> groupCustomersByInitial() {
return customerRepository.findAll()
.stream()
.collect(Collectors.groupingBy(customer -> customer.getName().charAt(0)));
}
Explanation:
Collectors.groupingBy(...)
: Groups the customers based on the first character of their names, resulting in a Map.
Bringing It All Together
Java Streams offer a powerful way to simplify data manipulation and retrieval, especially when coupled with Spring Data JPA. By adopting this functional approach, developers not only enhance the readability of their code but also leverage improved performance through features such as parallel processing and lazy evaluation.
To further explore Java Streams, consider checking out the official Java documentation on Streams or explore practical examples on platforms like Baeldung.
As you integrate Java Streams into your database queries, remember to balance performance with clarity. Adopt best practices, and your Java applications will not only be maintainable, but also efficient and modern.
Happy coding!