Maximizing Spring Cache: Overcoming Performance Bottlenecks
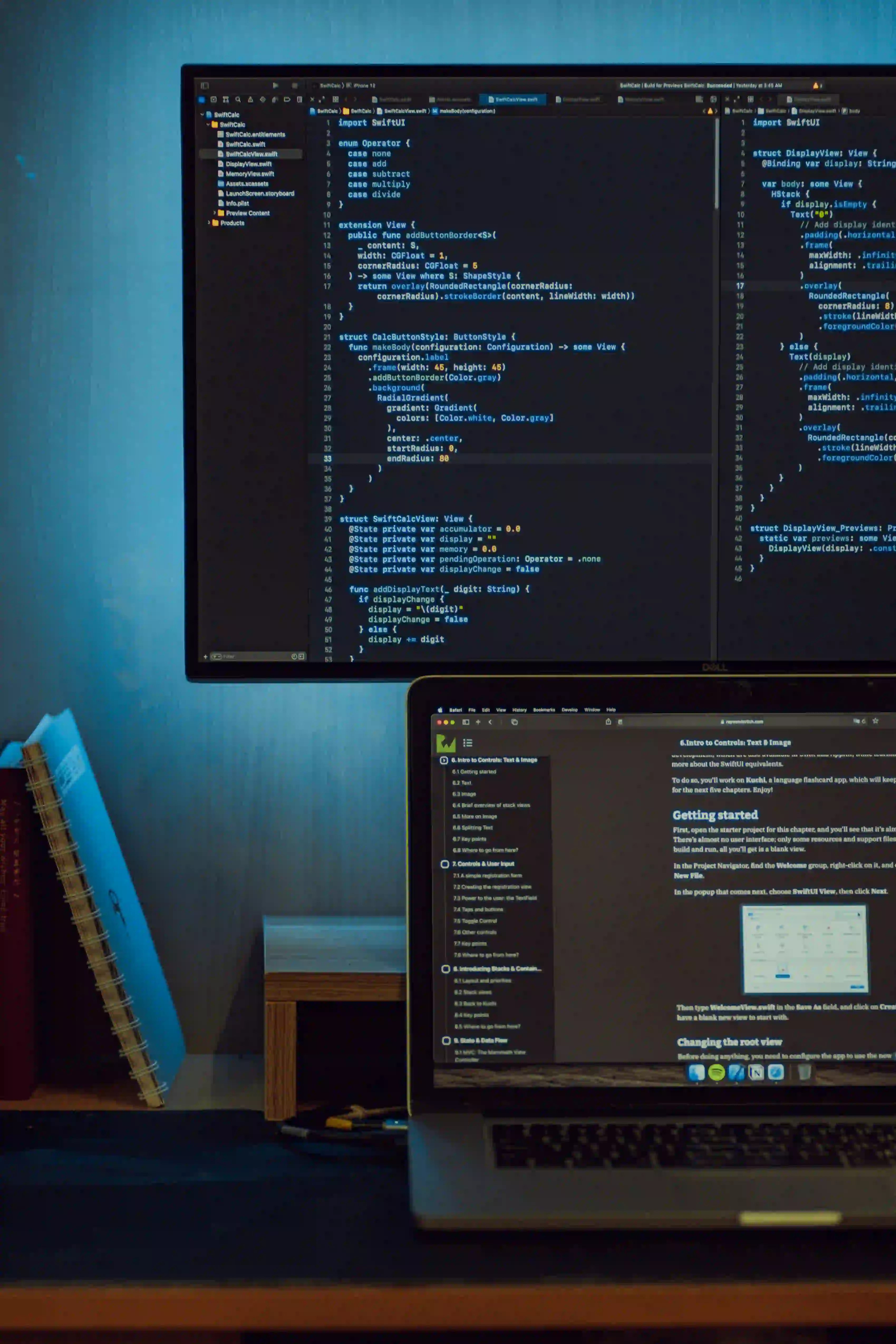
Maximizing Spring Cache: Overcoming Performance Bottlenecks
In modern software development, optimizing performance is crucial for delivering applications that can scale effectively. Spring Framework has made caching more straightforward, allowing developers to implement caching mechanisms with minimal overhead. However, knowing how to maximize Spring Cache and avoid performance bottlenecks is essential. In this blog, we'll explore the key concepts of caching in Spring, common pitfalls, and proven strategies to enhance performance.
Understanding Spring Cache
What is Spring Cache?
Spring Cache is an abstraction provided by the Spring Framework that allows developers to manage caching operations declaratively. The primary goal of caching is to store the results of expensive operations, so future identical requests can be served quickly from the cache instead of re-executing the operation.
Benefits of Caching
- Performance Improvement: By reducing the number of expensive computations, caching can significantly enhance application performance.
- Reduced Latency: Cached data can be retrieved faster than querying the database or recomputing results.
- Lower Resource Consumption: Caching results can lower CPU and memory usage, leading to better scalability.
Getting Started with Spring Cache
To enable caching in your Spring application, you need the following dependencies in your pom.xml
if you use Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
Enabling Caching
You can enable caching support in your Spring Boot application by annotating your main class with @EnableCaching
.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class CacheApplication {
public static void main(String[] args) {
SpringApplication.run(CacheApplication.class, args);
}
}
Simple Cache Example
Here’s a straightforward example using the @Cacheable
annotation. This annotation tells Spring to cache the result of the method based on the method parameters.
import org.springframework.stereotype.Service;
import org.springframework.cache.annotation.Cacheable;
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
simulateSlowService(); // Simulate a slow service
return fetchUserFromDatabase(id);
}
private void simulateSlowService() {
try {
Thread.sleep(3000); // Simulating a delay
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
}
private User fetchUserFromDatabase(Long id) {
// Fetch user logic
return new User(id, "Sample User");
}
}
Why Use Caching?
In the above code snippet, we have a method getUserById
that simulates a slow service by sleeping for three seconds. By using the @Cacheable
annotation, the first call to getUserById(1L)
will take three seconds, while subsequent calls with the same ID will return immediately with the cached result.
For a deeper dive into how caching works, check the Spring Cache Documentation.
Common Bottlenecks in Spring Cache
While caching is an effective strategy for performance optimization, it has its challenges. Here are some common bottlenecks developers should be aware of:
1. Over-Caching
Storing too much data can lead to memory issues. Make sure to set an appropriate cache size and evict old data regularly.
spring.cache.ehcache.config=classpath:ehcache.xml
In your ehcache.xml
, you can define your cache size:
<ehcache>
<diskStore path="java.io.tmpdir"/>
<cache name="users"
maxEntriesLocalHeap="1000"
eternal="false"
timeToIdleSeconds="300"
timeToLiveSeconds="600"/>
</ehcache>
2. Cache Miss Penalties
Cache misses can lead to performance penalties. Optimize your caching strategy by monitoring cache hits and misses. For diagnostics, consider using tools like Spring Boot Actuator.
3. Cache Consistency
As data updates occur, synchronizing with the cache can be challenging. Use @CachePut
to update cached values and @CacheEvict
to clear outdated cache entries.
Example of Using @CacheEvict
@CacheEvict(value = "users", key = "#user.id")
public void updateUser(User user) {
// Logic to update user
}
4. Caching Non-Deterministic Methods
Methods with side effects or non-deterministic results should not be cached. When you cache such methods, unexpected behaviors might arise.
Optimization Strategies for Spring Cache
Now that we’ve identified some common pitfalls, let’s explore practical strategies to optimize caching in a Spring application.
1. Use the Right Cache Implementation
Selecting the proper caching solution is critical to performance. Spring supports several cache providers including Ehcache, Caffeine, and Hazelcast. Each comes with unique features:
- Ehcache: Simple to set up and integrates seamlessly with JPA.
- Caffeine: A high-performance, in-memory caching library that offers advanced features, including automatic expiration.
- Hazelcast: Suitable for distributed caching in cloud environments.
2. Configure Cache Timeouts Wisely
Setting timeouts is critical for maintaining cache control. Avoid stale data by configuring expiration policies correctly. Here’s an example using Caffeine in application.properties
:
spring.cache.caffeine.spec=expireAfterWrite=10m, maximumSize=1000
3. Fine-Grained Caching
Instead of caching entire objects or datasets, cache individual fields or smaller subsets of data. This strategy minimizes memory usage and allows for finer control over cache behavior.
4. Monitor and Analyze Cache Performance
Use monitoring tools such as Prometheus and Grafana to track cache performance over time. Metrics like cache hit ratios can provide valuable insights for optimizations.
5. Bulk Operations
When fetching multiple records, consider implementing bulk queries and cache those results. This can reduce the load compared to fetching items one by one.
A Final Look
Mastering caching in a Spring application is a vital skill that can lead to massive performance improvements. By understanding the fundamentals of Spring Cache and implementing the strategies discussed above, developers can maximize their application's efficiency while minimizing bottlenecks.
For further reading, dive into the Spring Framework Cache documentation and explore additional performance tuning options.
By optimizing caching strategies effectively, you ensure your application can handle increased load without sacrificing performance—an essential requirement in today’s fast-paced digital world. Happy coding!