Overcoming Common Challenges in Selenium Google Search Automation
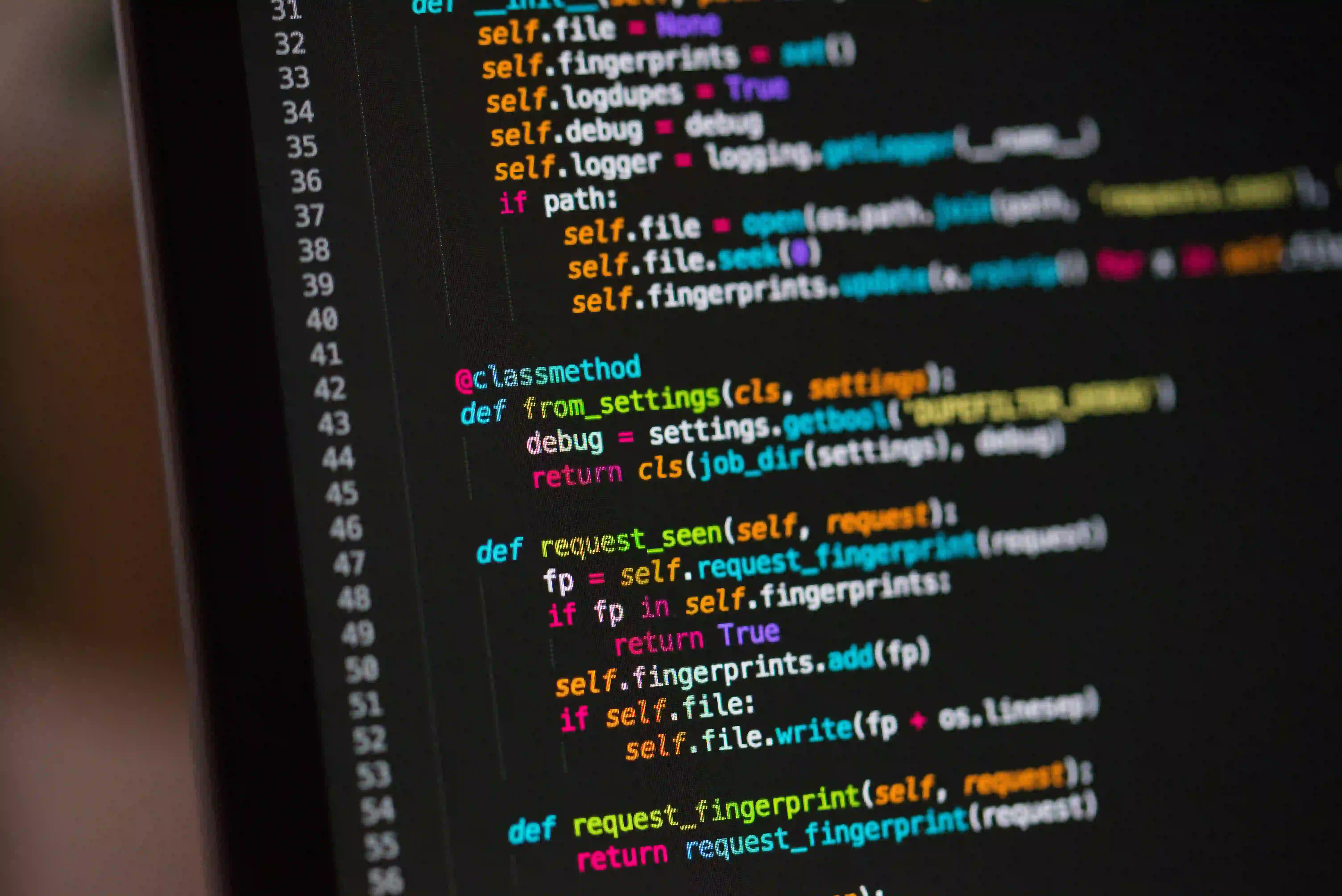
Overcoming Common Challenges in Selenium Google Search Automation
Selenium is a powerful tool for automating web applications, and one of its popular use cases is automating Google search queries. You might start off with the intention of writing a simple script to search for "Selenium automation," but soon find yourself facing unexpected challenges. In this blog post, we will explore these challenges and guide you on how to overcome them, ensuring a smooth automation experience.
Why Use Selenium for Google Search Automation?
Before diving into the challenges, it's worth discussing why you would use Selenium for this task:
- Efficiency: Automating repetitive searches saves time.
- Testing: Ensuring your web application interacts correctly with Google searches.
- Data Collection: Gathering search results for analysis.
Common Challenges in Google Search Automation
1. Handling Dynamic Content
Google's homepage is a dynamic page that frequently updates its content. Elements may not be loaded immediately, making it necessary to wait for them.
Solution: Use Selenium's explicit waits.
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com");
// Create an explicit wait condition
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// Wait until the search box is visible
WebElement searchBox = wait.until(ExpectedConditions.visibilityOfElementLocated(By.name("q")));
searchBox.sendKeys("Selenium automation");
searchBox.sendKeys(Keys.RETURN);
2. Navigating Through Captchas
Sometimes, Google may present a CAPTCHA challenge if it detects unusual behavior (e.g., too many search queries in a short time).
Solution: Implementing randomized wait times and session management can reduce the risk.
// Randomizing wait time
int randomWait = new Random().nextInt(5000) + 1000; // Between 1-5 seconds
Thread.sleep(randomWait);
This technique helps mimic human-like behavior and reduces the likelihood of hitting CAPTCHA.
3. Locator Strategy
Choosing the correct locator is crucial. Hard-coded locators may break due to updates on Google’s page.
Solution: Utilize robust locator strategies like XPath or CSS selectors. Here’s an effective code snippet for finding the search button:
// Using CSS Selector
WebElement searchButton = driver.findElement(By.cssSelector("input[name='btnK']"));
searchButton.click();
Using flexible locators will future-proof your scripts against minor UI changes.
4. Handling Pop-ups and Dialogs
Google might present various pop-ups, such as consent forms or notifications, which can interfere with your automation.
Solution: Write code to dismiss these pop-ups when they appear.
try {
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(5));
WebElement consentButton = wait.until(ExpectedConditions.elementToBeClickable(By.id("consent-button-id")));
consentButton.click();
} catch (TimeoutException e) {
System.out.println("No consent popup appeared.");
}
5. Managing Browser Sessions
Selenium opens a new browser window for automation every time it runs. This can lead to issues due to session limits imposed by Google.
Solution: Use cookies to manage sessions.
// Store and load cookies
Set<Cookie> cookies = driver.manage().getCookies();
driver.manage().deleteAllCookies();
for (Cookie cookie : cookies) {
driver.manage().addCookie(cookie);
}
By storing cookies, you maintain sessions even after closing and opening the browser.
6. Capturing and Analyzing Results
After searching, you would want to scrape the results and analyze them. However, extracting meaningful information requires attention to detail.
Solution: Use appropriate locators and structure the extraction logic effectively.
List<WebElement> results = driver.findElements(By.cssSelector("h3"));
for (WebElement result : results) {
System.out.println(result.getText());
}
This code snippet extracts and prints all the titles from the search results. Make sure to adhere to Google’s scraping policies.
7. Ensuring Code Maintainability
As your automation project grows, maintaining the code quality becomes essential. Embedding comments and breaking the code into methods can enhance readability.
Solution: Modular code structure.
public void performGoogleSearch(String query) {
WebDriver driver = new ChromeDriver();
try {
driver.get("https://www.google.com");
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys(query + Keys.RETURN);
} finally {
driver.quit();
}
}
8. Debugging Failed Tests
Failing tests can be tricky. Understanding the reason behind a failure requires proper debugging techniques.
Solution: Use logging.
private static final Logger logger = Logger.getLogger(MyTests.class.getName());
logger.info("Navigating to Google");
driver.get("https://www.google.com");
Logging provides insight into the execution flow and captures errors for easier troubleshooting.
Helpful Libraries and Tools
While Selenium provides a robust framework for automation, leveraging libraries can enhance your capabilities:
- JUnit: For writing and running tests.
- TestNG: Offers advanced functionality for running tests in parallel.
- SLF4J: Used for logging.
- Apache Commons Lang: Can be helpful for various utility functions.
Best Practices for Google Search Automation
- Rate Limiting: Be cautious of the number of requests sent to Google.
- No Hard-Coded Values: Use variables instead of hard-coded values for better adaptability.
- Modular Design: Structure your code in a modular format to simplify debugging and enhancements.
- Respect Robots.txt: Ensure you respect the guidelines specified by Google’s robots.txt file to avoid being blocked.
Closing Remarks
While Selenium provides an excellent framework for automating Google searches, several challenges can arise that require structured approaches for resolution. By employing best practices, leveraging the correct tools, and staying informed about the dynamic nature of web pages, you can create effective and robust automation scripts.
For more detailed guides and examples on Selenium, consider checking out the Selenium HQ documentation or exploring community forums like Stack Overflow.
Now, armed with this knowledge, you can create automation scripts that are not only functional but also resilient to changes. Happy automating!