Mastering Java 8 Streams: Tackling Grouping Mistakes
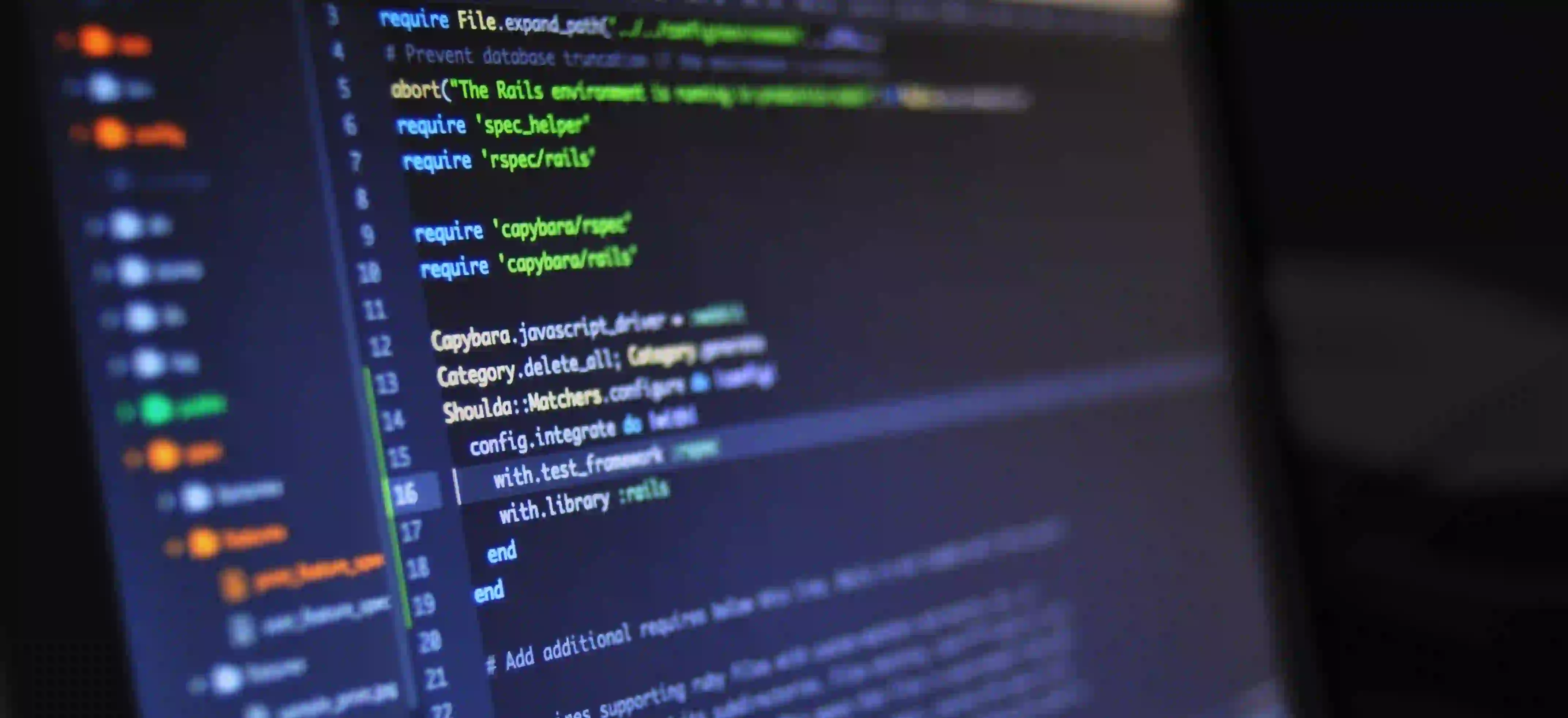
Mastering Java 8 Streams: Tackling Grouping Mistakes
Java 8 introduced a powerful feature called Streams which revolutionized data handling by allowing developers to write more readable and concise code. Among the various operations available in Java Streams, grouping is one of the most useful — yet, it's also where many developers stumble. In this post, we will delve into how to effectively use grouping in Streams while addressing common mistakes to help you truly master this aspect of Java.
Understanding Java Streams
Before diving into grouping, it's important to grasp what a Stream is. In Java, a Stream represents a sequence of elements that can be processed in parallel or sequentially. It provides a functional-style approach to programming, allowing developers to work with data collections more intuitively.
Key Features of Streams
- Laziness: Computation on data is deferred until necessary, improving performance.
- Functional Operations: Streams support a variety of operations such as filtering, mapping, and reducing.
- Ability to Handle Collections: Efficiently process collections like lists and sets.
For more in-depth exploration, refer to the Java documentation on Streams.
Grouping in Java Streams
The grouping operation in Streams is performed using the Collectors.groupingBy
method. This method groups elements based on a classification function and returns a Map
where keys are the result of applying the classification function, and values are the Lists of items.
Basic Example of Grouping
Consider the following scenario: you have a list of students, and you want to group them by their grades.
Here’s how you can achieve that:
import java.util.*;
import java.util.stream.Collectors;
class Student {
String name;
int grade;
Student(String name, int grade) {
this.name = name;
this.grade = grade;
}
public int getGrade() {
return grade;
}
@Override
public String toString() {
return name + " (" + grade + ")";
}
}
public class GroupingExample {
public static void main(String[] args) {
List<Student> students = Arrays.asList(
new Student("Alice", 90),
new Student("Bob", 80),
new Student("Charlie", 90),
new Student("David", 70)
);
Map<Integer, List<Student>> groupedByGrade =
students.stream()
.collect(Collectors.groupingBy(Student::getGrade));
groupedByGrade.forEach((grade, studentList) -> {
System.out.println("Grade " + grade + ": " + studentList);
});
}
}
Explanation
- Creating the Student Class: The
Student
class helps hold name and grade information. - Stream Pipeline: We leverage the
stream
method to create a stream from the list. - Grouping Students: We use
Collectors.groupingBy
with a method reference to group students by their grade.
Common Grouping Mistakes
While using Collectors.groupingBy
, developers often encounter several pitfalls. Below, we will tackle some of these mistakes along with corrections.
Mistake 1: Forgetting to Import Necessary Classes
One of the most straightforward mistakes is overlooking necessary imports. Always remember to include:
import java.util.*;
import java.util.stream.Collectors;
Mistake 2: Using Null Values in Grouping
When your data contains null values, it can lead to unexpected NullPointerExceptions. Always account for null entries:
List<Student> students = Arrays.asList(
new Student("Alice", 90),
null,
new Student("Charlie", 90)
);
// Avoid null by filtering
Map<Integer, List<Student>> groupedByGrade =
students.stream()
.filter(Objects::nonNull) // Ensure no null entries
.collect(Collectors.groupingBy(Student::getGrade));
Mistake 3: Grouping with Unsupported Data Types
Sometimes, developers attempt to group unsupported or incompatible data types. Always ensure that the classification function returns a compatible type.
Mistake 4: Not Understanding the Result
The result of groupingBy
is a Map. It is beneficial to familiarize yourself with the structure of the output:
Map<Integer, List<Student>> result = // result from groupingBy
Each key corresponds to the grouped element (the grade), and each value is a List of objects that had that key.
Intermediate Operations: Counting and More
Grouping can be taken a step further with intermediary operations. For instance, counting how many students belong to each grade category can be done using Collectors.counting()
along with Collectors.groupingBy
.
Map<Integer, Long> gradeCounts =
students.stream()
.collect(Collectors.groupingBy(Student::getGrade, Collectors.counting()));
gradeCounts.forEach((grade, count) ->
System.out.println("Grade " + grade + ": " + count + " students")
);
In this example, we added a counting operation to the grouping, outputting how many students fall under each grade.
Closing Remarks
In the world of Java 8 programming, mastering Streams and grouping is essential for writing clean and efficient code. By understanding the mechanics and common pitfalls associated with grouping, you can enhance your problem-solving abilities in Java significantly.
Should you be interested in expanding your knowledge further, take a look at articles discussing the power of Java Streams or the intricacies of Collectors.
By practicing these concepts and staying aware of common mistakes, you'll be on your way to becoming a proficient Java developer capable of harnessing the full power of Java Streams. Happy coding!