Common AJAX Form Issues in Spring MVC with jQuery
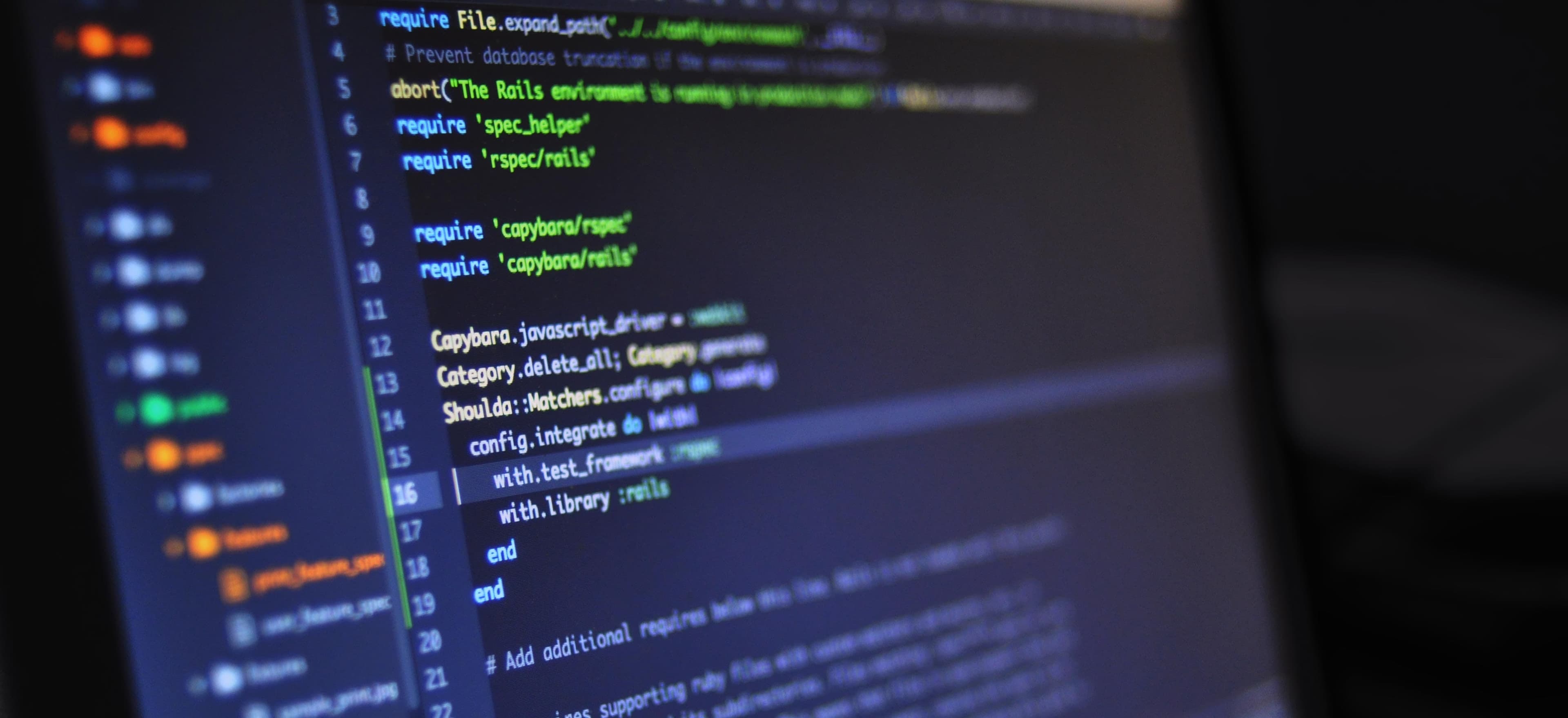
- Published on
Common AJAX Form Issues in Spring MVC with jQuery
AJAX (Asynchronous JavaScript and XML) has transformed the way web applications interact with users by allowing data to be sent and received asynchronously without refreshing a web page. This approach can significantly enhance user experience. However, when integrating AJAX with Spring MVC, developers often encounter common challenges that can hinder functionality. This post will delve into these issues and provide solutions that can optimize your AJAX forms using Spring MVC and jQuery.
Understanding AJAX in Spring MVC
Before diving into issues and solutions, let’s briefly discuss how AJAX works within the Spring MVC framework.
In a typical AJAX request, the JavaScript in your web application interacts with a server endpoint, which is often a controller in Spring MVC. The server processes the request and returns data, such as JSON, without needing to reload the entire page. This is achieved using jQuery's $.ajax
method or shorthand methods like $.get
or $.post
.
Basic Example
$.ajax({
type: "POST",
url: "submitForm",
data: $("#myForm").serialize(),
success: function(response) {
// Handle the response
alert("Form submitted successfully!");
}
});
In this code snippet, we serialize the form and send it to the submitForm
endpoint via a POST request. The success function alerts the user once the form is successful.
Common AJAX Form Issues
Understanding the common issues you might face will help ensure smooth integration between Spring MVC and jQuery.
1. Misconfigured Request Mapping
Issue
One of the most frequent issues is a misconfigured request mapping in your Spring Controller. If the URL in the AJAX call doesn't match the mapping, the request won't reach your controller.
Solution
Ensure that your URL matches the mapping in your controller:
@Controller
public class MyController {
@PostMapping("/submitForm")
@ResponseBody
public String handleForm(@RequestParam String data) {
// Processing logic
return "Success";
}
}
Verify that /submitForm
in your AJAX call matches @PostMapping("/submitForm")
.
2. CSRF Protection
Issue
Spring Security includes Cross-Site Request Forgery (CSRF) protection by default. If your application uses Spring Security, any AJAX POST request will fail unless it includes the CSRF token.
Solution
You can obtain the CSRF token from the Spring Security context and send it with your AJAX request. Here’s how you can do that:
<meta name="_csrf" content="${_csrf.token}"/>
<meta name="_csrf_header" content="${_csrf.headerName}"/>
Then include it in your JavaScript:
$.ajaxSetup({
beforeSend: function(xhr) {
xhr.setRequestHeader($('#_csrf_header').attr('content'), $('#_csrf').attr('content'));
}
});
This code snippet gets the CSRF token from the meta tag and adds it to the AJAX header, ensuring that your requests are secure.
3. Proper Response Handling
Issue
Another common mishap is failing to handle server responses correctly. If the server returns an error or unexpected response format, it can lead to confusion in your client-side logic.
Solution
Always handle different status codes accordingly. Here’s how you might refine your AJAX call with enhanced response handling:
$.ajax({
type: "POST",
url: "submitForm",
data: $("#myForm").serialize(),
success: function(response) {
alert(response);
},
error: function(xhr, status, error) {
alert("Error: " + xhr.responseText);
}
});
In this improved version, the error
function catches any errors, providing clearer information about what went wrong.
4. Data Serialization
Issue
Sometimes, the form data is not sent as you expect, particularly with complex data types such as arrays or nested objects.
Solution
Make sure you're using serialize()
or serializeArray()
correctly, depending on your needs. If you need a more complex structure, consider converting the form data to JSON:
var formData = {
name: $("#name").val(),
email: $("#email").val()
};
$.ajax({
type: "POST",
url: "submitForm",
contentType: "application/json",
data: JSON.stringify(formData),
success: function(response) {
alert(response);
}
});
This method explicitly structures the data, reducing the chance for serialization issues.
5. CORS Issues
Issue
Cross-Origin Resource Sharing (CORS) can become problematic when your API is hosted on a different domain than your client application. This may lead to blocked requests.
Solution
You can allow CORS in your Spring application by implementing a CorsMapping
.
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://yourdomain.com");
}
This code allows CORS for all paths from a specified origin. Always limit your allowed origins to only those you trust to mitigate security risks.
Best Practices for AJAX Forms in Spring MVC
- Use Promises: Leverage jQuery’s promise pattern to handle asynchronous tasks more cleanly, chaining multiple actions if needed.
$.post("submitForm", $("#myForm").serialize())
.done(function(response) {
console.log("Response:", response);
})
.fail(function(xhr) {
console.error("Error:", xhr.responseText);
});
-
Validate Inputs: Always validate input fields before sending data to the server to enhance data integrity and security.
-
Loading Indicators: Use a loading spinner or message to inform users that processing is ongoing, enhancing the overall user experience.
$("form").submit(function(event) {
event.preventDefault(); // Prevent default form submission
$("#loading").show(); // Show loading indicator
$.ajax({
// ... your AJAX setup
}).always(function() {
$("#loading").hide(); // Hide loading after AJAX completes
});
});
The Last Word
Integrating AJAX with Spring MVC can significantly improve the interactivity of your web applications. However, common pitfalls like misconfigured request mappings, CSRF protection, and error handling can derail the process if not addressed.
By understanding these common issues and implementing the suggested solutions, you can streamline your AJAX forms effectively. Always remember to embrace best practices to ensure clean, efficient, and user-friendly implementations.
For further reading and deeper insights into Spring MVC and AJAX integration, consider visiting the Spring Framework Documentation.
Happy coding!
Checkout our other articles