Mastering State Changes: Avoiding Signal Overloads!
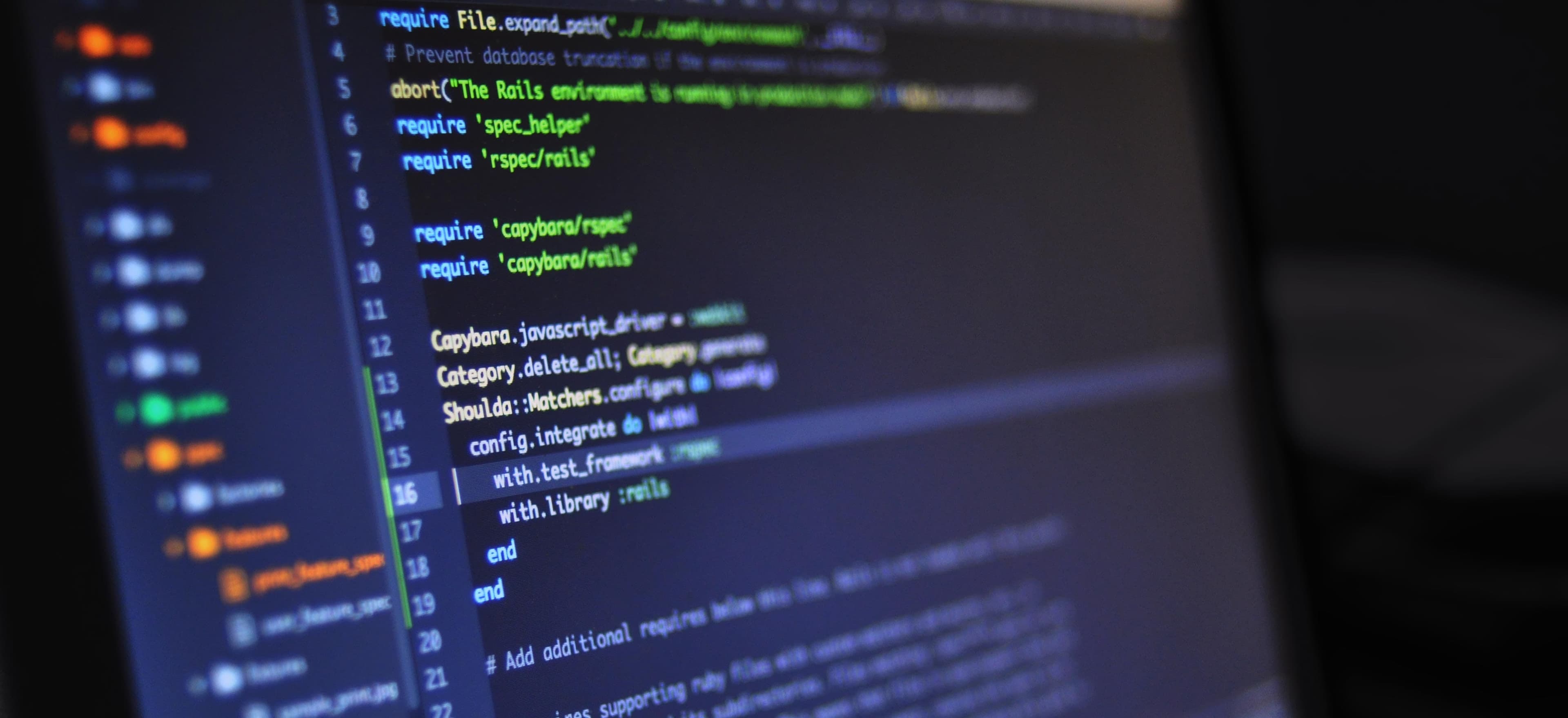
- Published on
Mastering State Changes: Avoiding Signal Overloads in Java
In modern application development, managing state changes efficiently is crucial for creating reactive, performant systems. With the rise of complex ecosystems often involving multiple components and services, developers need to ensure that they are not overwhelming their systems with unnecessary signals. In this blog post, we'll dive deep into managing state changes in Java, avoiding signal overloads, and implementing best practices for robust application design.
Understanding State Changes
At its core, a state change refers to any alteration to the current condition of an object. In Java, this can involve any change to the attributes or values of a class or structure. In applications, especially those built on modern frameworks like Spring or Java FX, managing these state changes efficiently is imperative to avoid heavy resource usage and ensuring seamless operation.
Why Are State Changes Important?
State changes are vital in applications due to:
- User Interactions: Every interaction by a user typically results in a state change.
- Data Processing: In applications processing large datasets, changes must be managed efficiently to keep applications responsive.
- System Performance: Overloading the system with unnecessary state change signals can lead to sluggish performance and a poor user experience.
Understanding Signal Overload
Signal overload occurs when too many changes are being processed simultaneously, causing a bottleneck in the system. This can lead to a situation where the application becomes unresponsive. The problem often stems from:
- Redundant State Changes: Sending multiple updates for the same state change.
- Event Storming: Triggering too many events in a short period.
Best Practices to Avoid Signal Overloads
Avoiding signal overloads requires a combination of design patterns, best practices, and architecture considerations. Below are key strategies to consider when designing your Java applications.
1. Debouncing State Changes
Debouncing is a technique used to limit the rate at which a function is executed. It ensures that a function is not called multiple times in quick succession.
Here’s how you could implement debouncing in your Java application:
import java.util.Timer;
import java.util.TimerTask;
public class StateManager {
private Timer timer;
private static final int DEBOUNCE_DELAY = 300; // milliseconds
public void onStateChange(Runnable stateChangeAction) {
if (timer != null) {
timer.cancel();
}
timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
stateChangeAction.run();
}
}, DEBOUNCE_DELAY);
}
}
Explanation of Code
In this code snippet:
- A
Timer
is used to schedule tasks. - When a state change occurs, previous timers are canceled to ensure only the latest action is executed.
DEBOUNCE_DELAY
allows for a suitable wait time before executing the action.
Debouncing is especially useful for actions like form submission or real-time search filtering.
2. Throttling State Changes
Throttling is similar to debouncing but limits the number of times a function can be executed over time. Rather than wait for a specific period of inactivity, throttling enforces a set interval for the function calls.
Here’s an example of how you could implement throttling:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ThrottledStateManager {
private ScheduledExecutorService executor;
private Runnable stateChangeAction;
private volatile boolean isThrottled = false;
private static final int THROTTLE_DELAY = 1000; // milliseconds
public ThrottledStateManager() {
executor = Executors.newSingleThreadScheduledExecutor();
}
public void onStateChange(Runnable stateChangeAction) {
this.stateChangeAction = stateChangeAction;
if (!isThrottled) {
isThrottled = true;
executor.execute(() -> {
try {
stateChangeAction.run();
} finally {
isThrottled = false;
}
});
executor.schedule(() -> isThrottled = false, THROTTLE_DELAY, TimeUnit.MILLISECONDS);
}
}
}
Explanation of Code
In this implementation:
- We use a
ScheduledExecutorService
to execute state changes. - The
isThrottled
flag prevents another state change from executing until theTHROTTLE_DELAY
has passed. - This avoids overwhelming the application with multiple state changes while ensuring updates are processed within a controlled timeframe.
3. State Change Batching
When multiple state changes occur in a short period, consider batching them together. Instead of sending each state change individually, accumulate changes and apply them as a single update.
import java.util.ArrayList;
import java.util.List;
public class BatchingStateManager {
private final List<Runnable> stateChangeQueue = new ArrayList<>();
private boolean isApplyingChanges;
public void queueStateChange(Runnable stateChangeAction) {
stateChangeQueue.add(stateChangeAction);
applyChanges();
}
private void applyChanges() {
if (isApplyingChanges) return; // Prevent re-entrance
isApplyingChanges = true;
for (Runnable action : stateChangeQueue) {
action.run();
}
stateChangeQueue.clear();
isApplyingChanges = false;
}
}
Explanation of Code
Key takeaways from this approach include:
- State changes are stored in a queue (
stateChangeQueue
) until they are applied all at once. - This approach reduces overhead caused by multiple event handlers executing individually.
Batching is particularly effective when working with UI updates or in situations where multiple changes are likely to be applied in sequence.
Wrapping Up
Mastering state changes and avoiding signal overloads is critical in developing responsive and efficient Java applications. By implementing techniques such as debouncing, throttling, and batching, developers can effectively manage state changes without overwhelming the system.
For further reading, explore Java's concurrency utilities for a deeper understanding of asynchronous programming patterns and their impact on state management:
As you apply these techniques in your projects, keep user experience at the forefront. Managing state changes is not just a technical requirement; it is integral to building engaging and efficient applications. Happy coding!
Checkout our other articles