Streamlining Java Object Conversion: Common Pitfalls
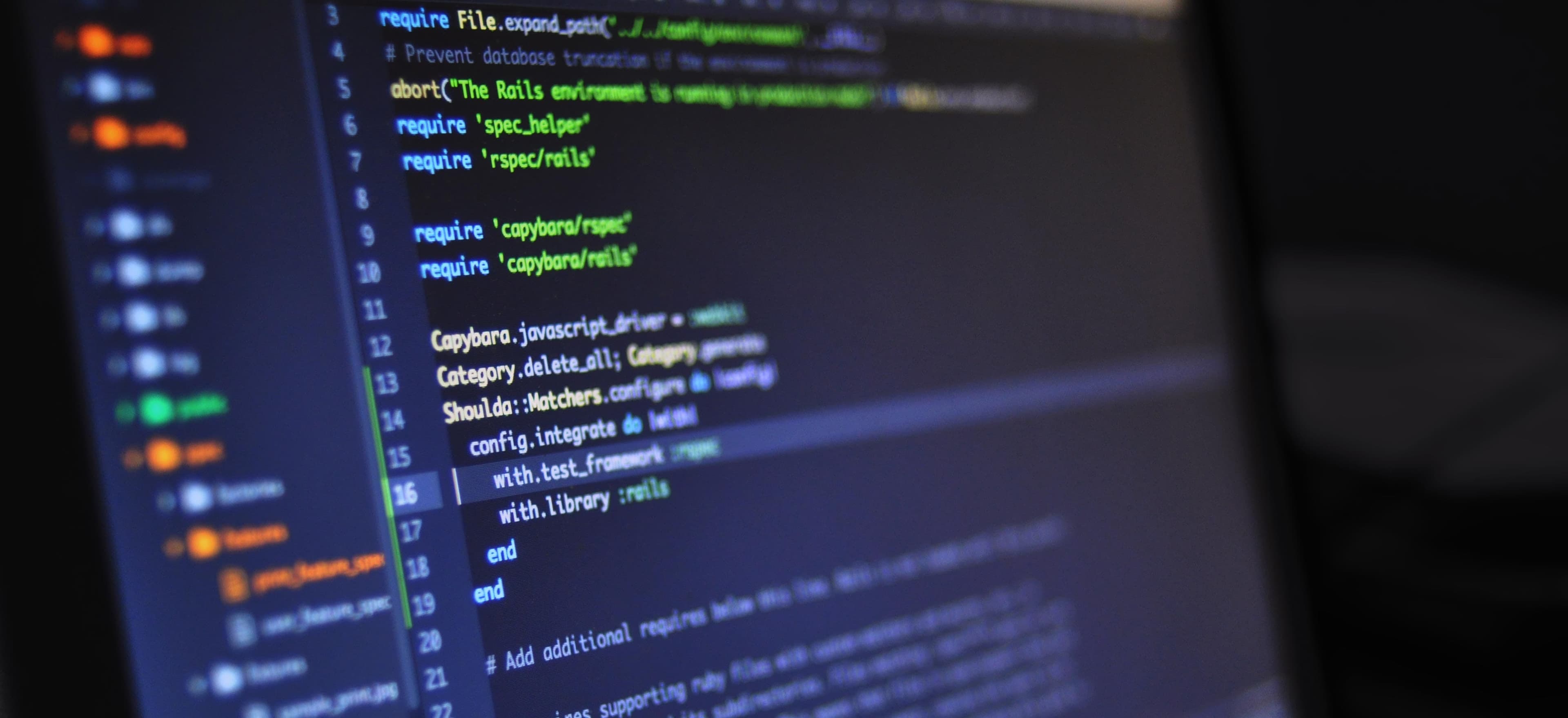
- Published on
Streamlining Java Object Conversion: Common Pitfalls
If you've been coding in Java for any amount of time, you'll know that object conversion is a fundamental task. Converting objects, whether between different types or from one representation to another, is frequent in many applications. However, developers often encounter pitfalls in this process. In this blog post, we will explore these challenges, providing practical examples and solutions to streamline your Java object conversion processes.
Understanding Object Conversion in Java
Object conversion in Java generally involves transforming an object from one type to another. This can be essential when you need to work with various data structures or when interacting with external systems, such as web services or databases.
There are several common scenarios in which object conversion is necessary:
- Type Casting: Converting an object from one type to another within the same hierarchy.
- Mapping: Transforming data objects into Data Transfer Objects (DTOs) for network communication.
- Serialization and Deserialization: Converting objects into a byte stream for storage or transmission and vice versa.
While these processes are commonplace, they can lead to issues if not handled correctly. Let's explore the common pitfalls and how to avoid them.
Common Pitfalls in Object Conversion
1. Lack of Proper Inheritance and Interfaces
Problem: When converting objects, especially in large applications, developers might overlook the importance of using the correct class hierarchy and interfaces. This can lead to ClassCastException
during runtime.
Solution: Ensure that your classes implement the appropriate interfaces and that you’re aware of the inheritance structure.
Code Example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
void sound() {
System.out.println("Cat meows");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.sound(); // Correctly calls Dog's sound method
// Avoid this: Trying to cast to an incompatible type
// Cat cat = (Cat) animal; // This will throw ClassCastException
}
}
2. Inadequate Error Handling
Problem: Conversions can fail for numerous reasons, such as incompatible types or null references. Proper error handling is crucial to prevent runtime crashes.
Solution: Use try-catch blocks to manage exceptions during conversion.
Code Example:
public class Converter {
public static Dog convertToDog(Animal animal) {
try {
return (Dog) animal;
} catch (ClassCastException e) {
System.out.println("Conversion failed: The provided animal is not a Dog.");
return null; // or throw a custom exception
}
}
public static void main(String[] args) {
Animal animal = new Cat();
Dog dog = convertToDog(animal);
if (dog == null) {
// Handle the failed conversion appropriately
}
}
}
3. Static Method Conversions
Problem: Using static methods for conversion can create confusion regarding the method context and can lead to code that is less maintainable.
Solution: Implement instance methods or utility classes to encapsulate conversion logic.
Code Example:
public class Dog {
private String name;
public Dog(String name) {
this.name = name;
}
public static Dog fromAnimal(Animal animal) {
if (animal instanceof Dog) {
return (Dog) animal;
}
throw new IllegalArgumentException("Requires a Dog instance to convert.");
}
public static void main(String[] args) {
Animal animal = new Dog("Rex");
Dog dog = Dog.fromAnimal(animal); // Cleaner and clearer
}
}
4. Forgetting to Handle Null Values
Problem: Null values can often lead to unexpected behavior during conversion processes, resulting in unwanted NPE (NullPointerException).
Solution: Always validate inputs and handle null references gracefully.
Code Example:
public class Converter {
public static Dog convertToDog(Animal animal) {
if (animal == null) {
throw new IllegalArgumentException("Animal cannot be null.");
}
return (Dog) animal; // Be cautious and ensure animal is of type Dog
}
public static void main(String[] args) {
Dog dog = null;
try {
dog = convertToDog(null);
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage()); // Output: Animal cannot be null.
}
}
}
5. Using Incorrectly Implemented toString()
Problem: Not properly implementing the toString()
method can lead to confusing outputs for converted objects. This can cause debugging nightmares.
Solution: Always customize the toString()
method in your data objects to enhance readability and debugging.
Code Example:
class Dog {
private String name;
public Dog(String name) {
this.name = name;
}
@Override
public String toString() {
return "Dog{name='" + name + "'}";
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Rex");
System.out.println(dog.toString()); // Output: Dog{name='Rex'}
}
}
6. Not Using Libraries for Complex Conversions
Problem: For complex object transformations, developers may not utilize existing libraries to handle conversions effectively. This can lead to reinventing the wheel and potentially introduce bugs.
Solution: Always consider using libraries like MapStruct or Dozer for mapping complex objects.
Code Example with MapStruct:
@Mapper
public interface DogMapper {
DogMapper INSTANCE = Mappers.getMapper(DogMapper.class);
DogDTO dogToDogDTO(Dog dog);
}
class DogDTO {
private String name;
// getters and setters
}
This example showcases a simple mapping interface to convert Dog
objects to DogDTO
objects seamlessly.
Final Thoughts
Navigating the complexities of object conversion in Java requires adherence to good design principles and proper handling of potential pitfalls. By understanding and addressing these common issues, you can enhance the efficiency and maintainability of your code.
Embrace best practices such as utilizing proper inheritance, robust error handling, and leveraging existing libraries for complex mappings. With these tools and strategies, you are well on your way to streamlining object conversion in your Java applications.
For further reading on enhancing your Java skills, consider visiting Oracle’s Java Tutorials and Baeldung, which offer a wealth of knowledge on Java programming. Happy coding!