Mastering Non-Blocking IO Challenges in Akka Framework
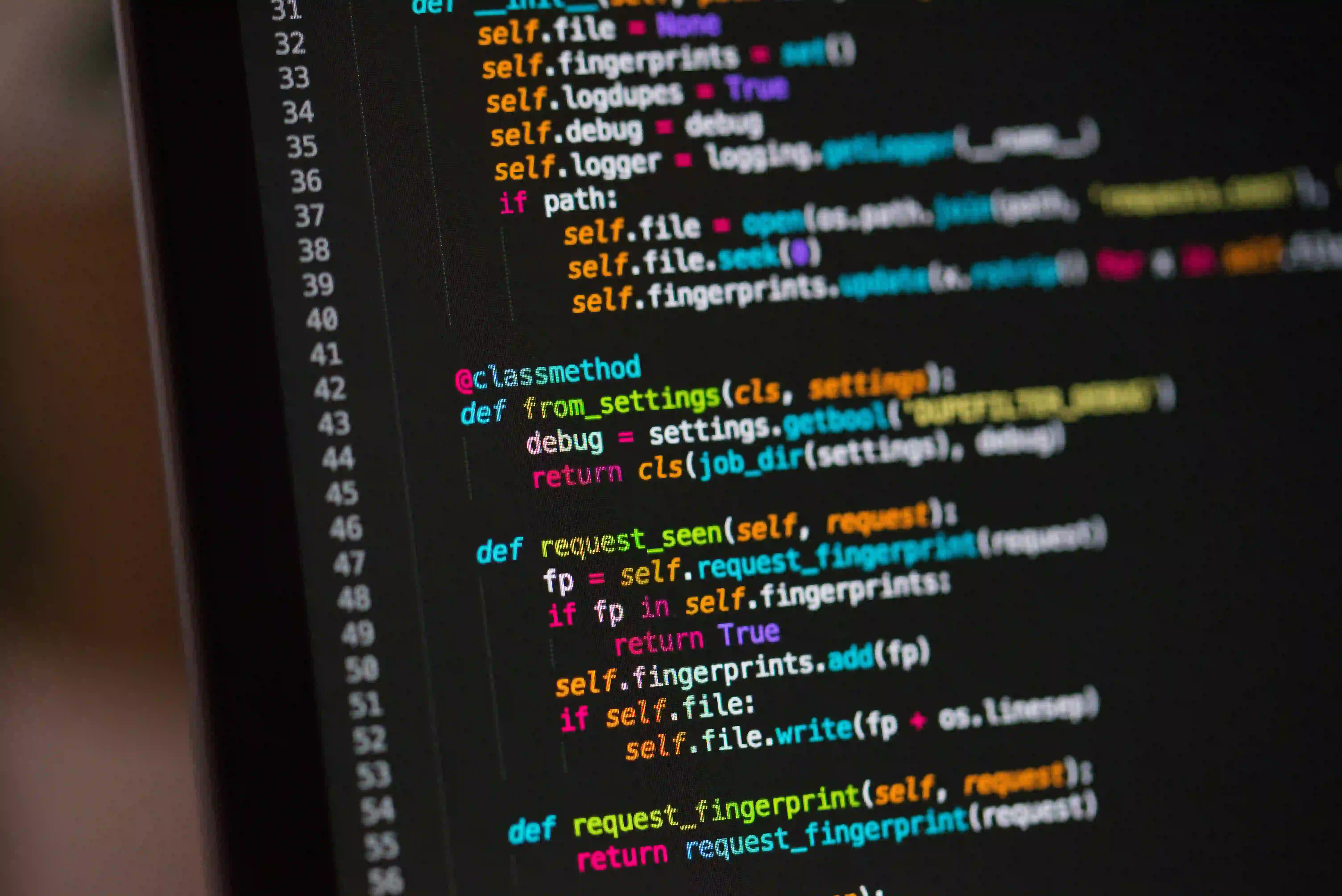
Mastering Non-Blocking IO Challenges in Akka Framework
In the realm of concurrent programming, handling input/output (IO) operations efficiently can often be a daunting challenge. When building responsive applications, especially at scale, developers must address the complexities of IO without blocking threads, which can lead to suboptimal performance and resource exhaustion. The Akka framework provides robust tools for managing non-blocking IO operations. This blog post will delve deep into mastering non-blocking IO challenges using Akka, bolstering your understanding of this powerful framework while showcasing effective strategies through code snippets and best practices.
Table of Contents
- Understanding Akka and Its Architecture
- Key Concepts of Non-Blocking IO in Akka
- Implementing Non-Blocking IO with Akka Streams
- Using Akka HTTP for Non-Blocking Web Services
- Error Handling in Non-Blocking IO
- Conclusion
Understanding Akka and Its Architecture
Akka is an open-source toolkit designed to simplify the development of concurrent, distributed, and resilient message-driven applications. Built on a powerful actor model, Akka abstracts away the complexities of thread management, enabling developers to focus on business logic rather than the intricacies of synchronization.
At its core, Akka employs Actors, which are lightweight entities capable of processing messages asynchronously. This paradigm is particularly advantageous when dealing with IO operations. By taking advantage of message passing, Akka can efficiently manage a large number of concurrent tasks without sacrificing the responsiveness of the application.
Key Concepts of Non-Blocking IO in Akka
To effectively navigate the challenges of non-blocking IO, the following concepts must be grasped:
-
Asynchronous Programming: By leveraging futures and promises, Akka allows for operations to be executed without blocking the execution thread.
-
Backpressure: This mechanism helps regulate data flow in streams to prevent overwhelming consumers by allowing them to signal when they are ready to accept more data.
-
Supervision Strategies: Akka enables the establishment of error handling strategies for actors, ensuring that failures are managed gracefully.
These principles will guide us through practical implementations as we move forward.
Implementing Non-Blocking IO with Akka Streams
Akka Streams is a powerful module designed to work with reactive streams, providing an elegant way to handle non-blocking data processing. Below, we will take a look at setting up a simple non-blocking file reader using Akka Streams.
Example: Reading a File Asynchronously
import akka.actor.ActorSystem
import akka.stream.scaladsl._
import akka.stream.{ActorMaterializer, Materializer}
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.Future
import scala.io.Source
object NonBlockingIOExample extends App {
implicit val system: ActorSystem = ActorSystem("NonBlockingIO")
implicit val materializer: Materializer = ActorMaterializer()
// Define a source for reading lines from a file
val filePath = "path/to/your/file.txt"
val fileSource: Source[String, _] = Source.fromIterator(() => Source.fromFile(filePath).getLines())
// Create a simple flow that prints each retrieved line
val printFlow = Flow[String].map(line => {
println(line) // Output the line to the console
})
// Run the stream
val result: Future[Unit] = fileSource.via(printFlow).runWith(Sink.ignore)
result.onComplete(_ => {
system.terminate() // Clean up the actor system after completion
})
}
Commentary
In the above example:
- We initiate an
ActorSystem
and aMaterializer
, the latter being crucial for executing our stream. Source.fromIterator
allows us to create a stream from the lines of a file without blocking the main thread.- The
Flow
processes each line, outputting it to the console. This is where the robustness of non-blocking IO shines: while one line is being processed, others can be read from the file seamlessly.
Using Akka HTTP for Non-Blocking Web Services
Building non-blocking web services is necessitated in today’s high-traffic applications. With Akka HTTP, you can create powerful, non-blocking REST APIs that scale efficiently. Below is an example of a simple Akka HTTP server that responds to HTTP requests.
Example: Simple Akka HTTP Server
import akka.actor.ActorSystem
import akka.http.scaladsl.Http
import akka.http.scaladsl.model.HttpResponse
import akka.http.scaladsl.server.Directives._
import akka.stream.ActorMaterializer
import scala.concurrent.Future
object HttpServerExample extends App {
implicit val system: ActorSystem = ActorSystem("HttpServer")
implicit val materializer: ActorMaterializer = ActorMaterializer()
implicit val executionContext = system.dispatcher
val route =
path("hello") {
get {
complete(HttpResponse(entity = "Hello, World!"))
}
}
val bindingFuture: Future[Http.ServerBinding] = Http().bindAndHandle(route, "localhost", 8080)
println("Server online at http://localhost:8080/")
println("Press RETURN to stop...")
scala.io.StdIn.readLine() // For stopping the server
bindingFuture
.flatMap(_.unbind()) // Trigger unbinding from the port
.onComplete(_ => system.terminate()) // And shutdown when done
}
Commentary
Here, our HTTP server:
- Defines a route for handling GET requests to the
/hello
endpoint. - Responds with a simple message without blocking operations.
- Akka HTTP automatically handles incoming requests concurrently and efficiently, allowing for high throughput and low latency.
To learn more about Akka HTTP, you can explore the Akka HTTP documentation.
Error Handling in Non-Blocking IO
While non-blocking operations deliver remarkable performance, they can also lead to unexpected challenges, particularly with error handling. Akka provides robust tools for managing failures gracefully. Using supervision strategies can help define how your application reacts to errors.
Example: Supervision Strategy
import akka.actor.{Actor, OneForOneStrategy, Props}
import akka.actor.SupervisorStrategy._
class MyActor extends Actor {
override def receive: Receive = {
case msg: String => println(s"Received: $msg")
case _ => throw new RuntimeException("Unexpected message")
}
override val supervisorStrategy: SupervisorStrategy = OneForOneStrategy() {
case _: RuntimeException =>
// Log error, resume processing
println("Caught RuntimeException, resuming!")
Resume
}
}
object SupervisionExample extends App {
val system = ActorSystem("SupervisionSystem")
val myActor = system.actorOf(Props[MyActor], "myActor")
myActor ! "Hello!"
myActor ! 42 // This will cause an error
}
Commentary
In this example, the actor MyActor
demonstrates:
- The ability to handle messages and respond appropriately.
- The implementation of a supervision strategy that gracefully handles runtime exceptions by resuming, allowing the actor to continue processing subsequent messages without crashing.
This flexibility allows your applications to remain resilient even in the face of unexpected errors.
Lessons Learned
Mastering non-blocking IO challenges in Akka can lead to the creation of high-performance, resilient applications capable of scaling efficiently under heavy load. By leveraging Akka’s actor model, Akka Streams, and Akka HTTP, developers can craft systems that handle concurrent tasks while maintaining responsiveness.
Continue to experiment with Akka features, and as you do, the complexities of non-blocking IO will begin to untangle themselves, revealing a landscape of opportunities for improving your applications.
To further enhance your understanding of non-blocking IO in Akka, consider exploring additional resources such as the Akka documentation and reactive design principles. Happy coding!