How to Choose the Right Selenium Framework for Your Web Tests
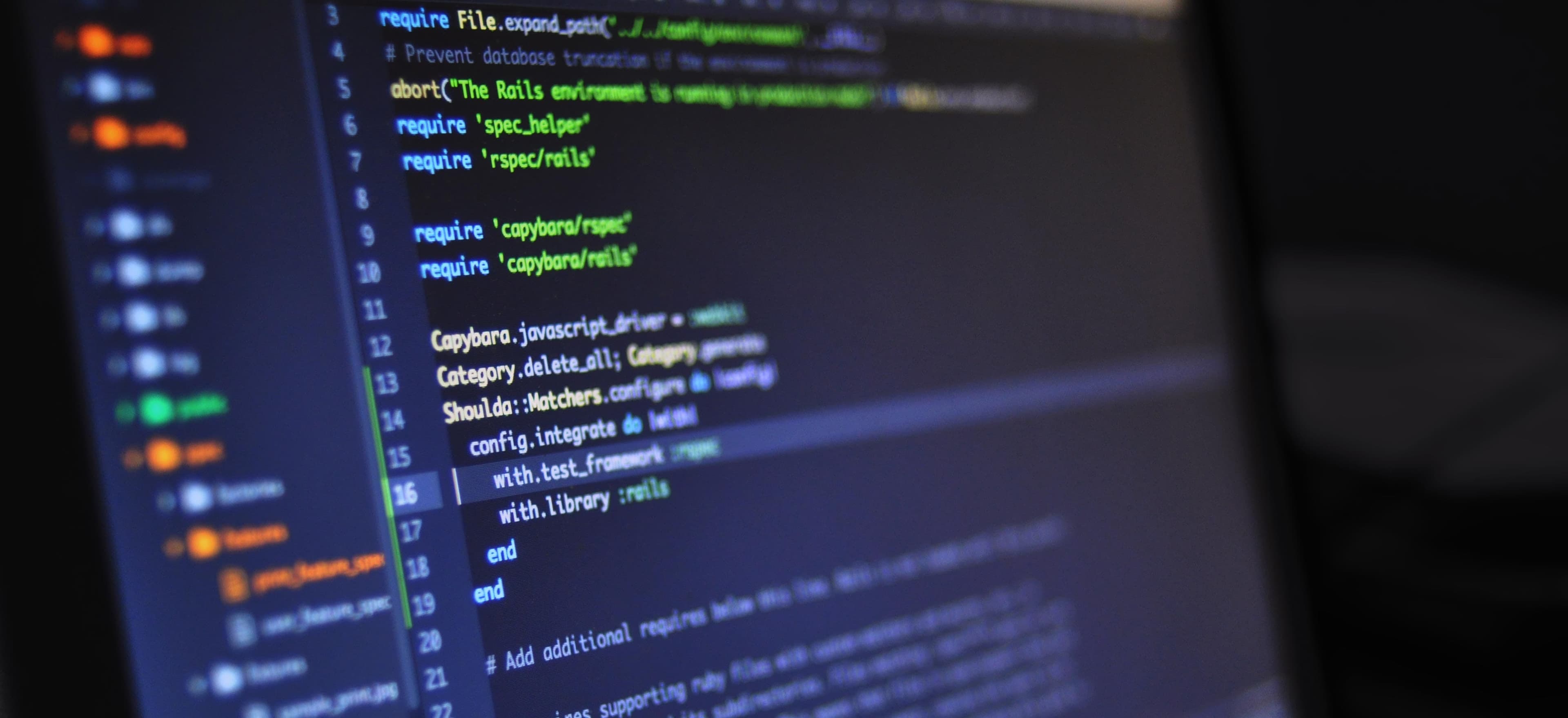
- Published on
How to Choose the Right Selenium Framework for Your Web Tests
In the ever-evolving landscape of web development and testing, Selenium stands out as one of the most popular tools for automating web applications. However, just as critical as choosing Selenium as your automation tool is selecting the right framework to leverage its capabilities efficiently. This blog post will guide you through the considerations and options available when selecting a Selenium framework for your web tests.
What is Selenium?
Before diving into frameworks, let’s briefly review what Selenium is. Selenium is an open-source tool that allows you to automate browsers. You can write test scripts in various programming languages like Java, Python, C#, and more, which then run across different web browsers.
For an in-depth understanding of Selenium, visit the official Selenium site.
Factors to Consider When Choosing a Selenium Framework
Selecting the appropriate Selenium framework involves several critical factors:
-
Project Requirements: Understand the needs of your project; this includes application size, complexity, and critical functionalities you want to automate.
-
Team Skillset: Assess the programming languages and skills your team members possess. It’s usually more efficient to opt for a framework that aligns with your team's expertise.
-
Framework Architecture: Familiarize yourself with various architectural patterns such as Model-View-Controller (MVC) or Page Object Model (POM) and how they can simplify test maintenance.
-
Reporting and Logging: Quality reporting tools help analyze test results and failures promptly. Choose a framework that incorporates robust logging and reporting mechanisms.
-
Community Support and Documentation: Frameworks with a supportive community and extensive documentation can significantly reduce troubleshooting time.
-
Integration with CI/CD Tools: If you're adopting continuous integration and continuous deployment, ensure the framework integrates smoothly with CI/CD tools like Jenkins or Travis CI.
Popular Selenium Frameworks
Here are some widely used Selenium frameworks you might consider:
1. TestNG
Overview: TestNG is a testing framework inspired by JUnit and NUnit and is particularly suited for Selenium testing. It allows for easy creation of test suites and supports parallel test execution.
Advantages:
- Supports data-driven testing via parameterization.
- Allows dependent test methods.
- Excellent reporting capabilities.
Example: Let's look at how you can set up a basic TestNG test in Java.
import org.testng.annotations.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumTestNGExample {
@Test
public void openGoogle() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Opening Google
driver.get("https://www.google.com");
// Asserting the title
assert driver.getTitle().equals("Google") : "Page title is not as expected!";
driver.quit();
}
}
In the example above, TestNG’s annotation (@Test
) makes it clear this is a test method. This clarity enhances maintenance and readability.
2. JUnit
Overview: JUnit is another popular testing framework in the Java ecosystem that integrates easily with Selenium, especially for unit tests.
Advantages:
- Simple to use and lightweight.
- Excellent integration with IDEs like Eclipse or IntelliJ IDEA.
Example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumJUnitExample {
@Test
public void testOpenGoogle() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Navigate to Google
driver.get("https://www.google.com");
// Assert title
assertEquals("Google", driver.getTitle());
driver.quit();
}
}
With JUnit, you can see the test structure is clear, and the assertion method communicates exactly what's being tested.
3. Page Object Model with Selenium
Overview: Page Object Model (POM) is not a framework per se but a design pattern that enhances test maintenance and readability by creating an object repository for web elements.
Advantages:
- Improves code reusability.
- Reduces code duplication.
Example:
public class GooglePage {
WebDriver driver;
By searchBox = By.name("q");
public GooglePage(WebDriver driver) {
this.driver = driver;
}
public void enterSearchTerm(String term) {
driver.findElement(searchBox).sendKeys(term);
}
public void submitSearch() {
driver.findElement(searchBox).submit();
}
}
You can then use this page object in your test like so:
import org.junit.Test;
public class GooglePageTest {
@Test
public void testGoogleSearch() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
GooglePage google = new GooglePage(driver);
driver.get("https://www.google.com");
google.enterSearchTerm("Selenium");
google.submitSearch();
driver.quit();
}
}
This modular approach makes it clear which page elements are interacted with and allows easy updates if changes are needed in the future.
4. Behavior-Driven Development (BDD)
Cucumber: Cucumber is a BDD framework that integrates with Selenium and allows you to write tests in a more human-readable format.
Advantages:
- Promotes collaboration between non-technical stakeholders and developers.
Example:
Feature: Google Search
Scenario: User searches for Selenium
Given the user is on the Google search page
When the user enters "Selenium" in the search box
Then the user should see results related to Selenium
With the proper setup in Java (using Selenium), you can define the step definitions that drive this feature, facilitating a clear understanding of what the automated tests are doing.
5. Robot Framework
Overview: Robot Framework is an open-source automation framework that uses an easy-to-understand tabular syntax to write tests, making it approachable for both developers and testers.
Advantages:
- Utilizes a keyword-driven approach.
- Supports extensive libraries, including Selenium.
Example:
*** Settings ***
Library SeleniumLibrary
*** Test Cases ***
Open Google And Search Selenium
Open Browser https://www.google.com chrome
Input Text name:q Selenium
Submit Form name:q
Close Browser
Robot Framework's simplicity makes it an excellent choice for teams that require quick readability or who involve stakeholders in the testing process.
Bringing It All Together
Choosing the right Selenium framework is crucial and can substantially impact the quality and maintainability of your web tests. While TestNG and JUnit are excellent for structured testing, models like Page Object and BDD using Cucumber can enhance collaboration and ease of testing. Robot Framework stands out for its simplicity and approachability.
Evaluate your project requirements, team skill sets, and specific features you need before making a decision. Ultimately, the right framework can save you time, effort, and headaches in your automation journey.
For further reading, you can check out the following links to enhance your understanding:
- Official TestNG Documentation
- JUnit Official Site
- Cucumber Documentation
- Robot Framework Official Site
Exploring these options and understanding their strengths and weaknesses will empower you to make an informed decision for your Selenium testing needs. Happy testing!
Checkout our other articles