Overcoming Common Issues When Releasing to Maven Central
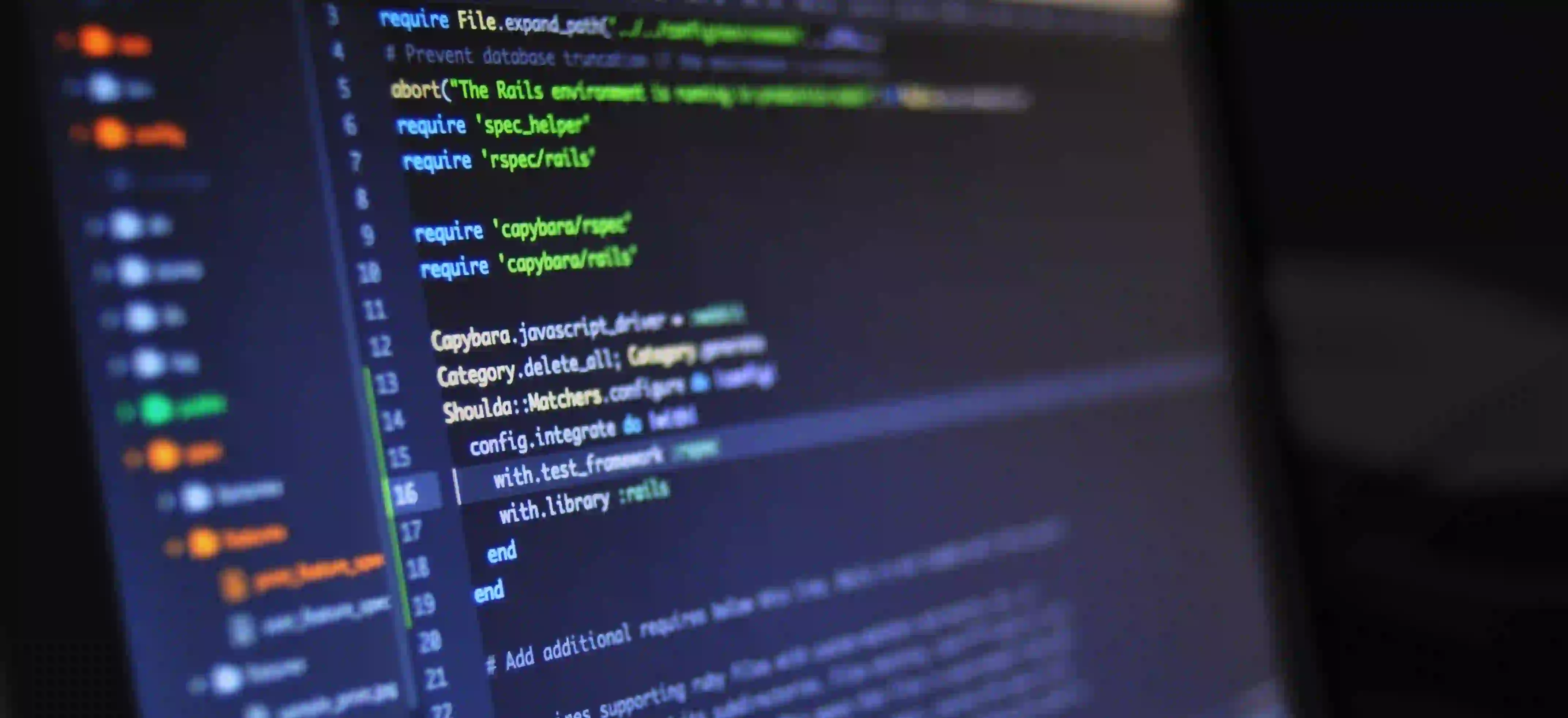
Overcoming Common Issues When Releasing to Maven Central
Releasing a library or application to Maven Central is an important milestone in software development. It provides developers around the globe with access to your project, fostering collaboration, ease of use, and community contributions. However, the process can be fraught with issues that may prevent a successful release. In this article, we will delve into common challenges faced while releasing to Maven Central and how to effectively overcome them.
Understanding Maven Central
Maven Central is a repository for Java libraries, allowing users to depend on these libraries in their Maven projects. Before you can release to Maven Central, you must ensure that your project complies with certain criteria and standards. This involves proper project structure, POM configuration, and signing artifacts.
Common Issues
1. POM Configuration Errors
The Project Object Model (POM) file is central to every Maven project. Errors in your POM can lead to failures during the release process. Common mistakes include missing elements, wrong versions, or incorrect dependencies.
Solution:
- Verify Structure: Ensure your POM follows the correct structure. Your POM file should include
<groupId>
,<artifactId>
,<version>
, and<packaging>
among other mandatory attributes.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
</project>
This basic configuration serves as the foundation. Correct group and artifact IDs help prevent namespace conflicts.
- Use the
mvn validate
Command: This command checks if your POM is correctly configured before deploying.
mvn validate
2. Authentication Issues
Maven Central uses Sonatype’s OSS Repository Hosting to manage the release process. Issues can arise during authentication if your settings are incorrect or if your credentials are not set up.
Solution:
- Check the
settings.xml
: Make sure your Mavensettings.xml
file, usually located in~/.m2/
, has the correct<server>
entries for bothsnapshots
andreleases
.
<servers>
<server>
<id>ossrh</id>
<username>your-username</username>
<password>your-password</password>
</server>
</servers>
- Use a Token: Consider using a personal access token instead of your password for added security and better control.
3. Repository Signing
As part of the release process, you need to sign your artifacts. Not signing them can lead to rejection by Maven Central.
Solution:
- Use GPG for Signing: First, ensure you have GPG installed. Create a key pair if you haven’t done so already.
gpg --full-generate-key
- Sign Artifacts During Release: Integrate signing into your build process using the
maven-gpg-plugin
.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-gpg-plugin</artifactId>
<version>1.6</version>
<executions>
<execution>
<goals>
<goal>sign</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This saves you from manual signing and ensures every artifact is authenticated properly.
4. Incomplete Metadata
Maven Central requires certain metadata including the project URL, description, and issue tracker. Omitting these can slow down the review process.
Solution:
- Add Metadata: Enhance your POM file with relevant metadata.
<project>
...
<description>A brief description of what my library does</description>
<url>http://example.com/my-library</url>
<scm>
<connection>scm:git:git://github.com/example/my-library.git</connection>
<developerConnection>scm:git:ssh://git@github.com:example/my-library.git</developerConnection>
<url>http://github.com/example/my-library</url>
</scm>
<issueManagement>
<system>GitHub</system>
<url>http://github.com/example/my-library/issues</url>
</issueManagement>
...
</project>
This helps users find relevant information quickly while also aiding in the approval process.
5. Snapshot Versions
Releasing snapshot versions to Maven Central is not allowed. Attempting to do so will lead to a failure and confusion.
Solution:
- Confirm Versioning: Ensure that your version follows proper semantic versioning (e.g., 1.0.0 instead of 1.0.0-SNAPSHOT).
# Before release, ensure you change to a release version
mvn versions:set -DnewVersion=1.0.0
- Use the
mvn clean deploy
Command: When ready to deploy a final version, execute the following command to ensure snapshots don't go live.
mvn clean deploy -DskipTests
Final Considerations
Releasing to Maven Central need not be a daunting endeavor if you anticipate potential pitfalls and address them proactively. By carefully configuring your POM file, ensuring authentication, signing your artifacts, adding necessary metadata, and avoiding snapshots, you position yourself for a smoother release process.
For more information, consider checking out the Sonatype OSSRH guide. This resource will provide further insights and best practices on Maven Central releases.
In summary, while there are several hurdles to overcome, the benefits of sharing your work through Maven Central are substantial. As you work through these challenges, remember that each issue encountered aids in your growth as a developer and can improve the quality of your contributions to the community. Happy coding!
Feel free to customize the solutions and snippets as per your needs.