How to Resolve Deadlocks in Your Database Systems
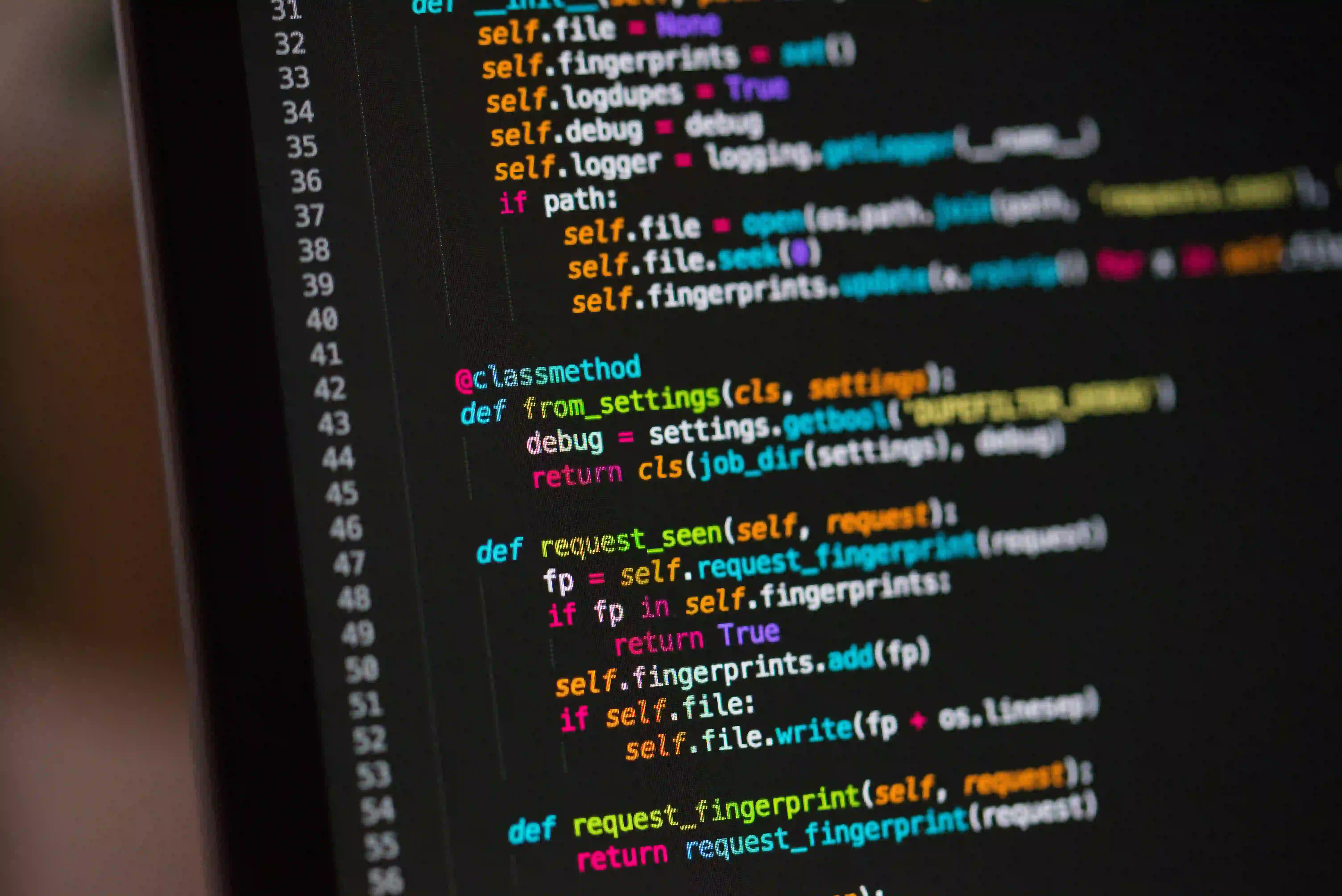
How to Resolve Deadlocks in Your Database Systems
Deadlocks are a common issue in the world of database systems. When one process holds resources that another process needs, and vice versa, both processes become stuck, waiting indefinitely. This results in a deadlock—a situation that can significantly degrade the performance of your application. In this comprehensive guide, we'll look into what deadlocks are, their causes, and practical strategies for resolving them. This post will equip you with the tools needed to effectively tackle deadlocks, ensuring your database maintains optimal performance.
Understanding Deadlocks
A deadlock occurs in a database system when two or more transactions are waiting indefinitely for each other to release resources. Instead of a simple scenario where one transaction finishes and another begins, in a deadlock, each transaction holds a lock that the other one needs to proceed. This not only affects the performance of your application but can also lead to timeouts or data inconsistencies.
Example Scenario
Consider two transactions, T1 and T2:
- T1 locks Table A and then tries to lock Table B.
- T2 locks Table B and then tries to lock Table A.
Both transactions are now waiting for each other to release locks, resulting in a deadlock situation.
// Example of Deadlocks in Java
public void transactionA() {
synchronized (tableA) {
// Simulating some processing
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (tableB) {
// processing on tableB
}
}
}
public void transactionB() {
synchronized (tableB) {
// Simulating some processing
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (tableA) {
// processing on tableA
}
}
}
In the above Java code, both transactions A and B can create a deadlock if they are executed concurrently.
Causes of Deadlocks
Understanding the underlying causes of deadlocks is crucial for prevention. Here are some common factors:
-
Resource Contention: Multiple transactions trying to lock the same set of resources in different orders can result in deadlocks.
-
Long-Running Transactions: Transactions that take a significant amount of time to execute increase the likelihood of conflicts.
-
Inadequate Lock Granularity: If locks are too coarse (e.g., locking an entire table when only a row is needed), the chances of deadlocks increase.
-
Locking Order: If the ordering of resource acquisition is not consistent among transactions, it can lead to circular wait conditions.
Strategies for Resolving Deadlocks
1. Detecting Deadlocks
Many modern database management systems (DBMS) have built-in mechanisms for detecting deadlocks. These systems periodically check for transactions that are waiting indefinitely and can abort one of the transactions to resolve the deadlock.
For example, in MySQL, the InnoDB storage engine automatically detects deadlocks when they occur and will choose one transaction to roll back.
2. Timeouts
Utilizing timeouts is a practical strategy that can help resolve deadlocks. By setting a timeout on transactions, you can force a transaction to abort and try again if it has waited longer than a specified duration.
// Setting a timeout in Java
try {
connection.setNetworkTimeout(Executors.newFixedThreadPool(1), 2000); // 2 seconds
// Perform your database operations
} catch (SQLException e) {
System.out.println("Transaction timed out!");
// Handle the timeout scenario
}
This code snippet demonstrates how to set a timeout for a database connection in Java. If a transaction doesn’t complete within the allocated time, it is aborted.
3. Locking Strategies
Lock Granularity: Use fine-grained locking where possible—lock only the specific rows needed instead of entire tables. This reduces contention and the likelihood of deadlocks.
Lock Ordering: Establish a consistent order in which transactions lock resources. If all transactions lock Table A before Table B, for example, you can avoid circular waits.
4. Redesigning Transactions
Review your transactions to ensure they are as short as possible. Here are a few strategies to accomplish this:
- Avoid long-running transactions by breaking them into smaller units of work.
- Minimize the number of locks required in a transaction.
- Optimize queries to reduce execution time.
5. Monitoring Tools
Utilize monitoring tools to gain insights into transaction behavior. These tools can help identify problematic areas in your code or database design, such as bottlenecked transactions or frequent deadlocks. Modern DBMS offer performance dashboards that can help in understanding transaction flow.
6. Using Retry Logic
Implementing a retry mechanism is a popular way to minimize the impact of deadlocks. When a transaction fails due to a deadlock, the transaction can be retried after a brief pause.
public void executeWithRetry() {
final int MAX_RETRIES = 3;
int attempts = 0;
while (attempts < MAX_RETRIES) {
try {
// Perform your database operation
return; // Exit on success
} catch (TransactionDeadlockException e) {
attempts++;
if (attempts >= MAX_RETRIES) {
throw e; // Re-throw the exception if retries exceeded
}
// Optional: Exponential backoff
try {
Thread.sleep((long)Math.pow(2, attempts) * 100); // Wait before retrying
} catch (InterruptedException interruptedException) {
Thread.currentThread().interrupt();
}
}
}
}
Bringing It All Together
Deadlocks are an unavoidable part of database systems, but with appropriate strategies, they can be effectively managed and minimized. By investing time in understanding the factors contributing to deadlocks and implementing the discussed strategies, you can ensure smooth, efficient operations in your applications. Whether it's through consistent locking orders, timeout settings, or retry logic, a proactive approach goes a long way in maintaining database integrity.
For further understanding of database locking mechanisms, you may refer to these resources:
- Understanding Locking in Databases
- Deadlocks in SQL Server: What to Know
By applying these techniques, you will be equipped to handle deadlocks effectively, leading to a more robust and resilient database system. Happy coding!