Mastering Interface Overload: Abstract Factory Simplified
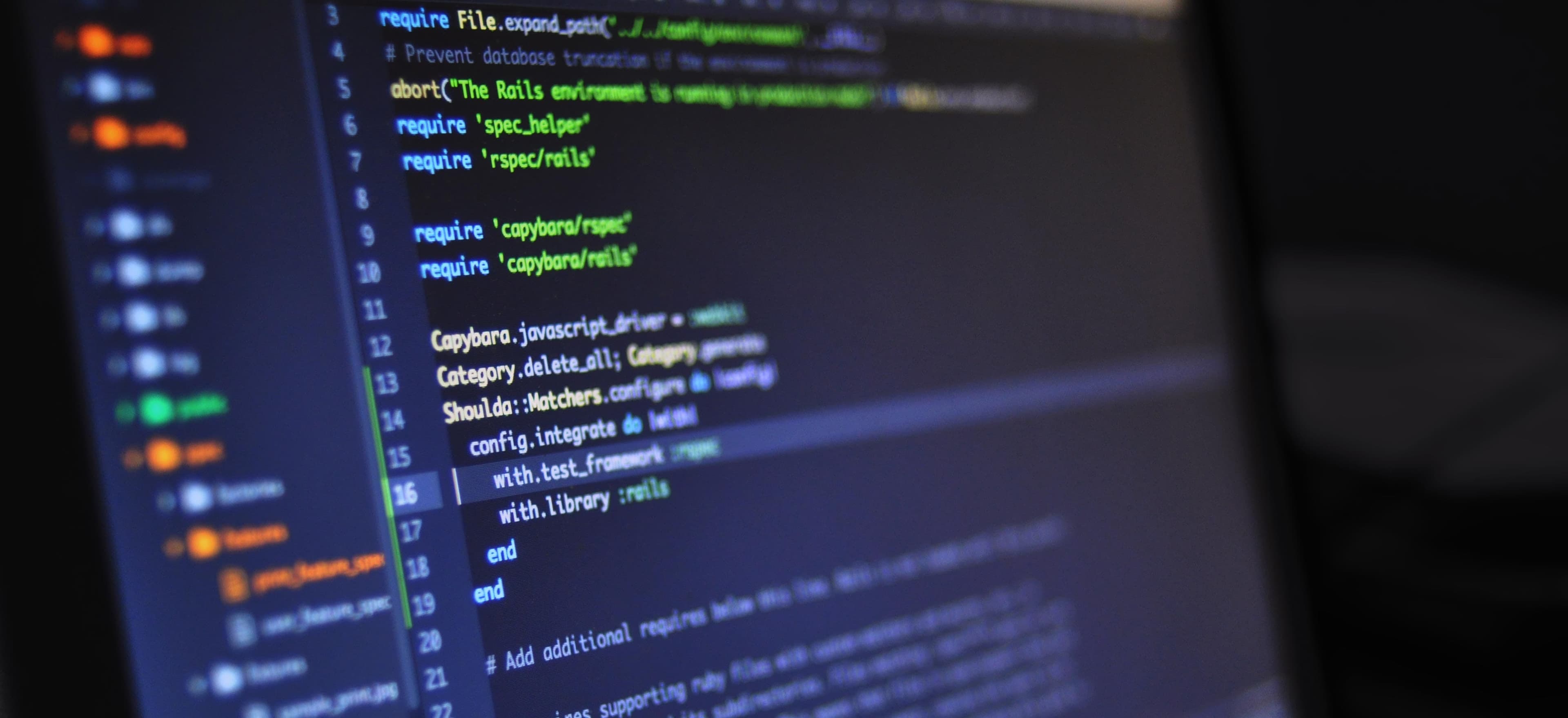
- Published on
Mastering Interface Overload: Abstract Factory Simplified
In the realm of Java programming, design patterns play a vital role in structuring code efficiently and effectively. A commonly discussed pattern in the Java ecosystem is the Abstract Factory. This design pattern is often used when your code requires the creation of objects without specifying the exact classes of the objects being created. Today, we'll delve into the concept of Abstract Factory, focusing on mastering interface overload while simplifying your code. By the end of this article, you’ll have a clearer understanding of how to implement the Abstract Factory pattern in Java.
What is the Abstract Factory Pattern?
The Abstract Factory pattern is a creational design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes. This design pattern is particularly useful when your application needs to be independent of how its objects are created, composed, and represented.
Key Benefits:
- Separation of Concerns: By using an Abstract Factory, you decouple the system from the specifics of object creation.
- Ease of Maintenance: Changes in the object creation process require minimal alterations in your codebase.
- Flexibility: Allows for the creation of different types of objects at runtime.
Understanding Interfaces
Before diving into the implementation, it’s important to understand interfaces in Java. An interface is an abstract type that allows for a contract to be established without having a defined implementation.
Why Use Interfaces?
- Multiple Implementations: Different classes can implement the same interface in various ways.
- Polymorphism: It allows you to use different classes interchangeably if they implement the same interface.
Abstract Factory Pattern in Action
To illustrate the Abstract Factory Pattern, let’s consider the scenario of a furniture shop. In this example, the shop offers different styles of furniture, namely modern and Victorian. We can implement a family of related products (Chairs, Tables) using the Abstract Factory.
Step 1: Define the Product Interfaces
Here are interfaces for the two types of furniture: Chair
and Table
.
// Chair interface
public interface Chair {
void sitOn();
}
// Table interface
public interface Table {
void eatOn();
}
Step 2: Create Concrete Products
Next, we define concrete implementations for these interfaces.
// Modern chair implementation
public class ModernChair implements Chair {
@Override
public void sitOn() {
System.out.println("Sitting on a modern chair.");
}
}
// Victorian chair implementation
public class VictorianChair implements Chair {
@Override
public void sitOn() {
System.out.println("Sitting on a Victorian chair.");
}
}
// Modern table implementation
public class ModernTable implements Table {
@Override
public void eatOn() {
System.out.println("Eating on a modern table.");
}
}
// Victorian table implementation
public class VictorianTable implements Table {
@Override
public void eatOn() {
System.out.println("Eating on a Victorian table.");
}
}
Step 3: Define the Abstract Factory Interface
We need an interface for the factory that will produce our families of products.
// Abstract furniture factory interface
public interface FurnitureFactory {
Chair createChair();
Table createTable();
}
Step 4: Implement Concrete Factories
Now that we have our products and the factory interface, let's create concrete factories for both styles.
// Modern furniture factory
public class ModernFurnitureFactory implements FurnitureFactory {
@Override
public Chair createChair() {
return new ModernChair();
}
@Override
public Table createTable() {
return new ModernTable();
}
}
// Victorian furniture factory
public class VictorianFurnitureFactory implements FurnitureFactory {
@Override
public Chair createChair() {
return new VictorianChair();
}
@Override
public Table createTable() {
return new VictorianTable();
}
}
Step 5: Using the Abstract Factory
Now that everything is set up, let’s utilize our factories to create furniture.
public class FurnitureClient {
private Chair chair;
private Table table;
public FurnitureClient(FurnitureFactory factory) {
this.chair = factory.createChair();
this.table = factory.createTable();
}
public void useFurniture() {
chair.sitOn();
table.eatOn();
}
}
Main Class to Test the Implementation
Here’s how you can test this entire setup.
public class Main {
public static void main(String[] args) {
FurnitureFactory modernFactory = new ModernFurnitureFactory();
FurnitureClient modernClient = new FurnitureClient(modernFactory);
modernClient.useFurniture();
FurnitureFactory victorianFactory = new VictorianFurnitureFactory();
FurnitureClient victorianClient = new FurnitureClient(victorianFactory);
victorianClient.useFurniture();
}
}
Explanation of the Implementation
- Product Interfaces: Our product interfaces (
Chair
,Table
) establish a contract for the different types of furniture. - Concrete Products: Each concrete chair and table class implements the respective interfaces, providing specific behavior.
- Factory Interface: The
FurnitureFactory
interface is the dustpan that collects various products, keeping related items together. - Concrete Factories:
ModernFurnitureFactory
andVictorianFurnitureFactory
determine which concrete products to create. - Client Code: The
FurnitureClient
interacts with the factory, demonstrating its usage without needing to know about the concrete classes.
Avoiding Interface Overload
While the Abstract Factory pattern offers numerous advantages, be cautious of interface overload. Too many interfaces can lead to a complex structure that lacks clarity. Consider the following tips:
Tips to Avoid Interface Overload:
- Keep It Simple: Define interfaces with clear, focused responsibilities.
- Group Related Functions: If possible, group related functionalities into a single interface to avoid fragmentation.
- Unused Interfaces: Regularly review and prune interfaces that are no longer in use or necessary.
The Closing Argument
Mastering the Abstract Factory pattern in Java allows you to create families of related objects while keeping your system decoupled from specific implementations. By focusing on interfaces and factory methods, you pave the way for more flexible and maintainable code.
For further reading on design patterns and their applications, check out Refactoring Guru and Java Design Patterns. These resources provide excellent insights and examples to enhance your knowledge and expertise in software design.
Now that you have a comprehensive understanding of the Abstract Factory pattern, it's time to put it into practice and see how it can simplify your Java applications. Happy coding!
Checkout our other articles