Common Apache Shiro Misconfigurations to Avoid
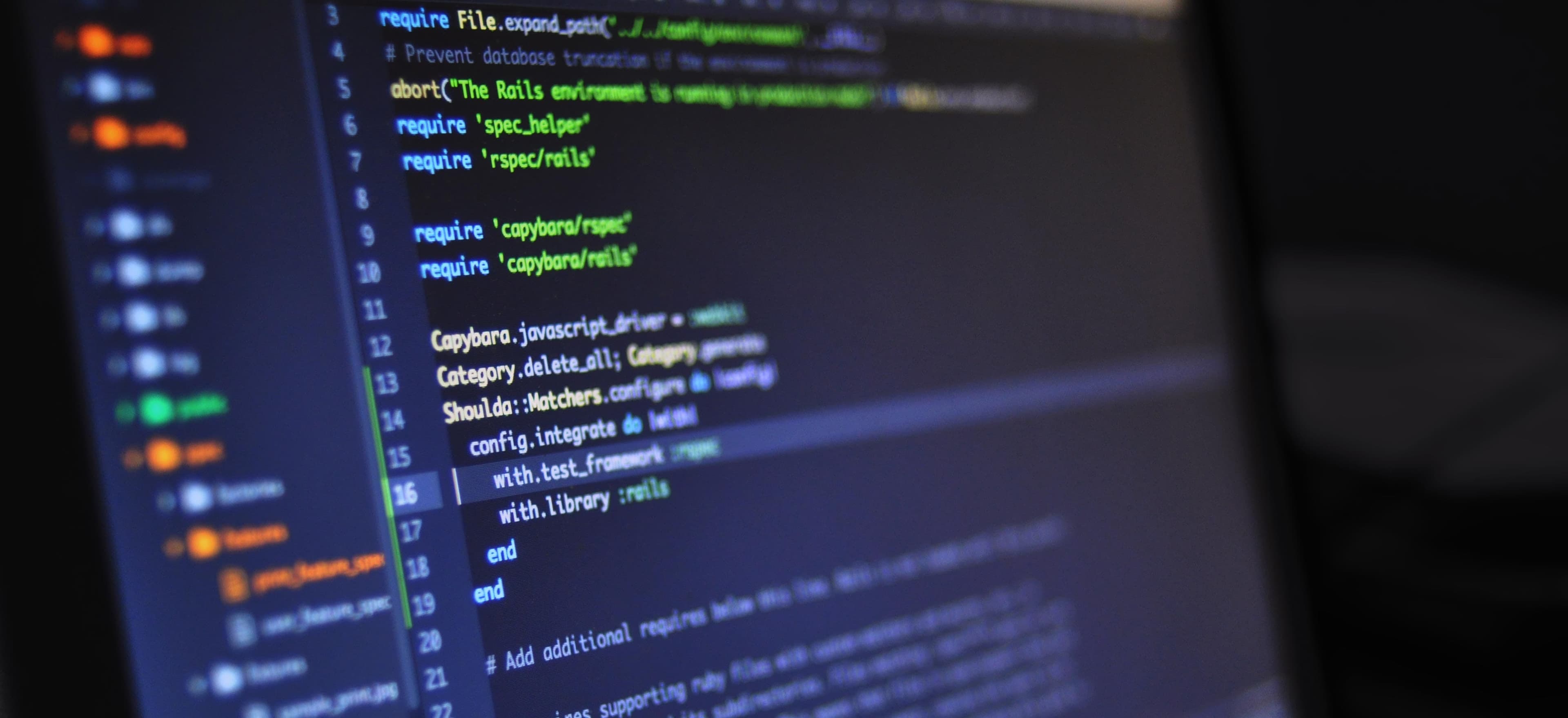
- Published on
Common Apache Shiro Misconfigurations to Avoid
Apache Shiro is a powerful framework for handling security in Java applications. It offers a simple API for authentication, authorization, session management, and cryptography. While it simplifies the security concerns, improper configurations can lead to vulnerabilities that jeopardize the security of your application. In this blog post, we'll delve into common misconfigurations in Apache Shiro and how to avoid them.
Table of Contents
- Introduction to Apache Shiro
- Importance of Proper Configuration
- Common Misconfigurations
- Insecure Session Management
- Incorrect Realm Configuration
- Potential Security Risks with Caching
- Overly Permissive Access Control
- Misconfigured Authorization Filters
- Best Practices to Avoid Misconfigurations
- Conclusion
1. Introduction to Apache Shiro
Apache Shiro is a powerful and flexible security framework that allows you to secure your Java applications with minimal fuss. Shiro is centered around four core features: Authentication, Authorization, Session Management, and Encryption. These features can be used independently or together, depending on your application criteria.
For a deeper understanding of how Shiro works, check out the official Apache Shiro documentation.
2. Importance of Proper Configuration
Proper configuration of Apache Shiro is crucial because any misstep can lead to security vulnerabilities. These vulnerabilities can result in unauthorized access to sensitive information, integrity breaches, or even denial of service.
By understanding the common pitfalls and adhering to best practices, you can secure your application more effectively.
3. Common Misconfigurations
Insecure Session Management
One of the major elements of user state management in Shiro is sessions. Sessions can be misconfigured, leading to vulnerabilities. For instance, failing to configure session timeouts can leave a user logged in indefinitely.
Code Example:
Session session = SecurityUtils.getSubject().getSession();
session.setTimeout(3600000); // Session timeout of 1 hour
Why: This code snippet ensures that the session times out after one hour of inactivity. Configuring an appropriate session timeout protects against session hijacking.
Incorrect Realm Configuration
Realms are pivotal for authentication and authorization in Shiro. A common misconfiguration occurs when developers overlook the details in realm setup.
Code Example:
CustomRealm customRealm = new CustomRealm();
customRealm.setCredentialsMatcher(new HashedCredentialsMatcher("SHA-256"));
Why: Using a hashing algorithm for credentials ensures that sensitive information is not stored in plain text. In this example, configuring the credentials matcher with SHA-256 adds an important layer of security.
Potential Security Risks with Caching
Shiro allows caching of authentication and authorization data for performance. However, caching without proper invalidation can lead to stale permissions being granted to users who no longer have those rights.
Code Example:
EhCacheManager ehCacheManager = new EhCacheManager();
ehCacheManager.setCacheName("myCache");
securityManager.setCacheManager(ehCacheManager);
Why: While enabling caching enhances performance, ensure to specify appropriate caching strategies. For sensitive applications, always validate user permissions against the database periodically.
Overly Permissive Access Control
It's easy to become lax with access control in the early development stages. However, granting overly permissive permissions can lead to significant risks.
Code Example:
[roles]
admin = *
user = view
Why: Avoid using wildcards (*) in configurations for roles. This grants all permissions and can lead to data exposure. Instead, define specific permissions for each role to limit access appropriately.
Misconfigured Authorization Filters
Authorization filters can become a point of error if they are not properly configured. It's critical to define filters precisely to prevent unauthorized access.
Code Example:
<filter chain>
<filter name="authc" />
<filter name="anon" />
</filter chain>
Why: Filters like authc
and anon
are key in protecting URLs. Ensure you understand which filters to use and their implications. It's best to prioritize authenticated access to protected endpoints.
4. Best Practices to Avoid Misconfigurations
- Follow the Principle of Least Privilege: Grant users the minimum permissions necessary to perform their tasks.
- Regular Code Reviews: Conduct code reviews focused exclusively on security configurations.
- Employ Proper Session Management: Implement session timeouts and expiration strategies effectively.
- Secure Password Storage: Always use a reliable hashing algorithm for storing passwords.
- Testing and Validation: Regularly test your application for vulnerabilities using tools such as OWASP ZAP or similar.
- Keep Documentation Updated: Adhere to Shiro's documentation for configuration updates.
5. Conclusion
Apache Shiro is a robust framework for securing Java applications, but even the strongest frameworks can be rendered ineffective with improper configurations. By avoiding the common misconfigurations highlighted above and adhering to best practices, developers can create applications that are not only feature-rich but also secure.
For further reading, explore the Shiro Security Best Practices.
With proactive security measures and continuous learning, you can significantly enhance your application's security posture and deliver a safer experience for your users.
By maintaining vigilance and awareness about these common pitfalls, developers can significantly enhance the security of their applications while utilizing Apache Shiro. Remember, security is an ongoing process, not a one-time configuration.
Checkout our other articles