Optimizing JVM Arguments for Peak Application Performance
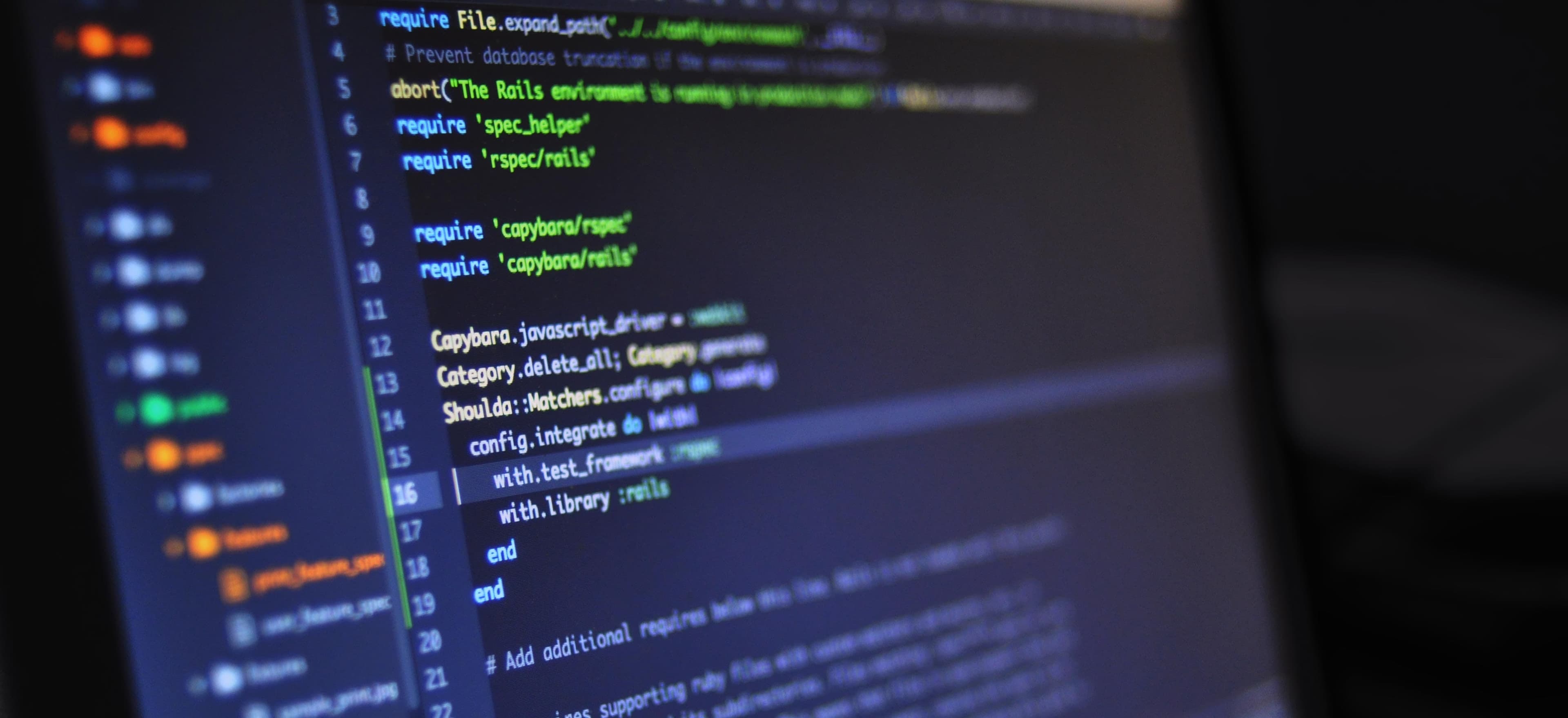
- Published on
Optimizing JVM Arguments for Peak Application Performance
Java applications run on the Java Virtual Machine (JVM), which translates Java code into byte code and executes it with impressive speed and efficiency. However, the performance of a JVM-based application isn’t solely determined by the code itself. The configuration of the JVM arguments plays a critical role in how well your application performs.
This blog post will explore how to optimize JVM arguments for peak application performance. We will cover various aspects like memory allocation, garbage collection tuning, and thread management.
Understanding JVM Arguments
JVM arguments can be broadly categorized into:
- Standard Options - These are fundamental settings that the JVM uses to configure its runtime environment.
- Garbage Collection Options - These determine how Java manages memory and cleans up unused objects.
- X Options - These are non-standard options that offer advanced tuning capabilities.
For a deep dive into the JVM options you can utilize, consider reviewing the official documentation from Oracle.
Memory Management: Allocation and Sizing
Set the Heap Size
The Java heap is where Java objects are allocated. By default, the JVM sets initial and maximum heap sizes automatically. However, this can lead to suboptimal performance in high-demand applications.
You can set the initial heap size using -Xms
and the maximum heap size using -Xmx
. The values should be tuned according to your application's needs:
java -Xms512m -Xmx4096m -jar MyApp.jar
Why Set Heap Sizes?
Setting these arguments helps reduce garbage collection (GC) overhead, as the JVM will spend less time resizing the heap during runtime.
Example of Memory Management
Suppose you are running a large-scale application, and you notice performance degradation. An initial configuration may look like this:
java -Xms256m -Xmx1024m -jar MyApp.jar
After profiling your application, you find that it requires more memory. You can optimize this configuration as follows:
java -Xms2g -Xmx8g -jar MyApp.jar
This adjustment allocates more memory, allowing for better performance during peak loads.
Garbage Collection Tuning
Garbage Collection (GC) is crucial for any JVM application as it reclaims memory used by objects that are no longer reachable. It's important to choose the right GC algorithm and tune its parameters.
Choosing the Right GC Algorithm
Java 9 introduced new garbage collectors that can be tweaked to improve performance:
- G1 Garbage Collector (-XX:+UseG1GC): A good choice for applications that require low-latency performance with manageable heap sizes.
java -XX:+UseG1GC -jar MyApp.jar
- Z Garbage Collector (-XX:+UseZGC): Ideal for large heap sizes and short GC pauses.
java -XX:+UseZGC -Xmx32g -jar MyApp.jar
Why Choose a GC Algorithm?
The right algorithm minimizes lag time and improves throughput, which is essential for maintaining a responsive user experience.
Tuning GC Parameters
When using G1GC, consider tuning its parameters for better performance:
java -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -XX:G1ReservePercent=20 -jar MyApp.jar
Here, MaxGCPauseMillis
sets the maximum pause time during GC, while G1ReservePercent
specifies the percentage of memory the GC can reserve for itself.
Thread Management
Configure Thread Stack Size
Java applications use threads to run concurrent tasks. However, threads consume memory. You can specify the stack size for each thread using the -Xss
JVM argument:
java -Xss512k -jar MyApp.jar
Why Adjust Thread Stack Size?
A smaller stack size allows you to start more threads, which is critical for applications that rely on concurrency. However, setting it too low might lead to StackOverflowError
.
Example of Thread Management
If your application serves web requests, it may depend on handling thousands of concurrent threads. Optimizing the thread stack size would look something like this:
java -Xss256k -jar MyApp.jar
In crowded scenarios, this might allow you to adequately handle your request load while ensuring minimal thread overhead.
Additional Optimizations
Enable Class Data Sharing
Class Data Sharing (CDS) allows class metadata to be shared among different Java processes, reducing startup time. To enable this feature, add the following to your configuration:
java -XX:+UseAppCDS -jar MyApp.jar
Why Use CDS?
For applications frequently instantiated, this can significantly speed up startup times—an essential factor in cloud-based environments.
JIT Compiler Settings
Just-In-Time (JIT) compilation optimizes frequently executed bytecode into machine code, enhancing performance. You can tweak JIT settings as follows:
java -XX:TieredStopAtLevel=1 -jar MyApp.jar
This setting tells the JVM to stop tiered compilation at a specific level, potentially reducing CPU usage for less-critical tasks.
Monitoring and Profiling
Finally, it is crucial to monitor and adjust JVM settings based on live metrics and profiling data. Tools such as VisualVM or JConsole can help you visualize memory usage, CPU load, and thread activity.
Example of Monitoring
You might use VisualVM to determine memory consumption patterns:
jvisualvm
By connecting to your running JVM instance, you can inspect heap dumps and GC activity, allowing for data-driven optimization.
Final Thoughts
Optimizing JVM arguments is essential for maximizing the performance of your Java applications. From memory settings to garbage collection algorithms, each parameter has a role in defining how your application runs. Always profile your application and adjust parameters based on real-life usage patterns.
For more information on JVM tuning, consider checking out Java Performance: The Definitive Guide by Scott Oaks. Happy coding!
Checkout our other articles