Common Hibernate JPA Pitfalls and How to Avoid Them
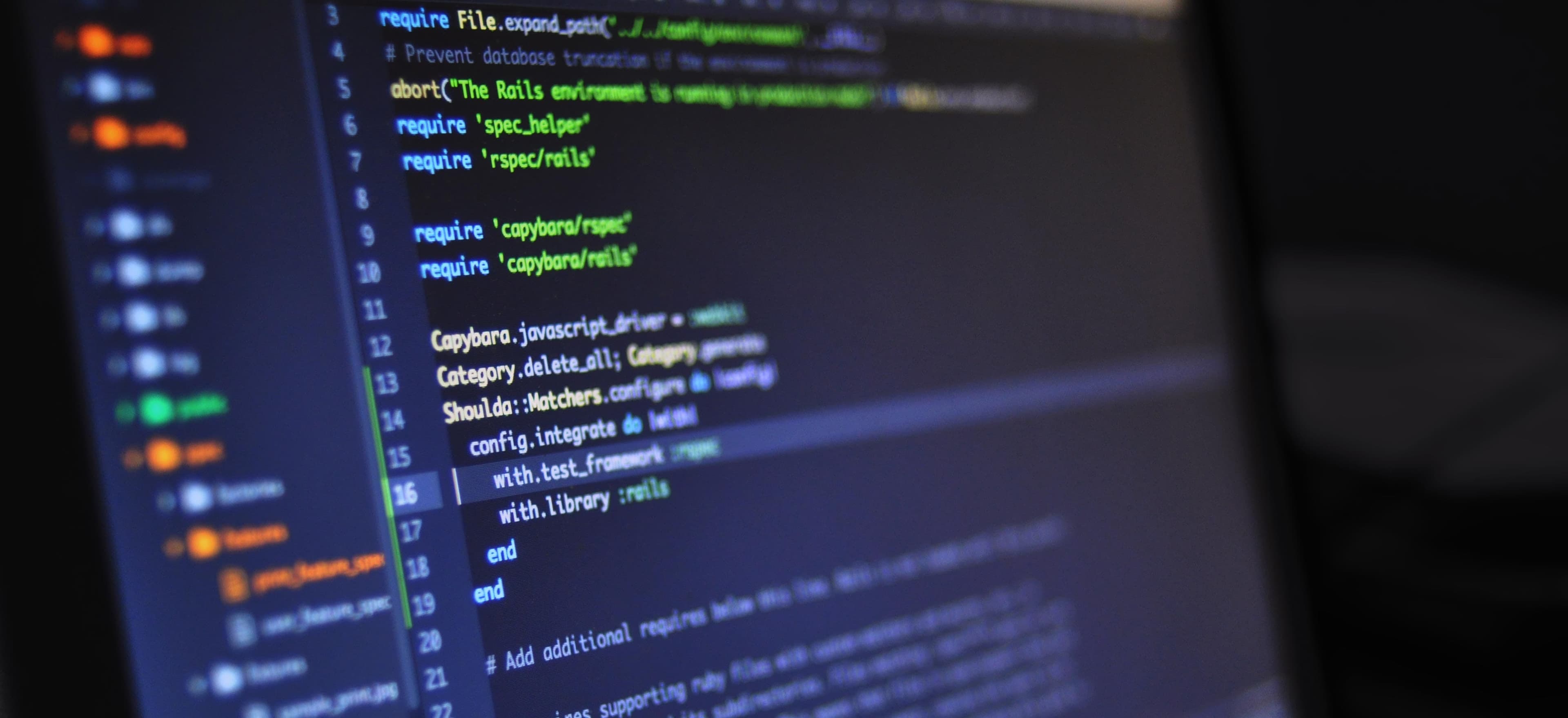
- Published on
Common Hibernate JPA Pitfalls and How to Avoid Them
Hibernate JPA (Jakarta Persistence API) is a powerful framework for object-relational mapping (ORM) in Java applications. While it simplifies database interactions, it can also lead to common pitfalls that new developers might encounter. This blog post outlines several typical challenges and provides practical solutions to help you navigate these issues effectively.
Understanding JPA and Hibernate
Before diving into the common pitfalls, it's essential to have a foundational understanding of what JPA and Hibernate are.
- JPA is a specification that provides a standard for object-relational mapping in Java. It defines how to manage relational data in applications using Java objects.
- Hibernate is a popular implementation of JPA that adds features, such as caching, lazy loading, and transaction management.
While JPA defines how to interact with databases through entities and repositories, Hibernate offers implementations that enhance JPA's core functionalities.
Common Pitfalls
Let's explore some of the prevalent pitfalls developers encounter while using Hibernate JPA and how to avoid them.
1. Not Using the Right Fetch Type
The Pitfall
One of the most common mistakes is misconfiguring fetch types. JPA supports two types of fetching: EAGER and LAZY.
- EAGER fetching retrieves all associated entities immediately.
- LAZY fetching only retrieves associated entities when they are accessed.
While EAGER fetching can simplify querying, it often leads to performance issues, especially with large datasets.
The Solution
Use LAZY fetching by default to optimize performance. This way, data is only loaded when it's necessary. Here’s an example:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(fetch = FetchType.LAZY, mappedBy = "user")
private Set<Post> posts;
// Getters and Setters
}
Why: This setup helps avoid the "N+1 Selects" problem—you won’t load user posts until you explicitly call them. Always analyze your specific use case to decide on fetch types.
2. Forgetting to Properly Handle Transactions
The Pitfall
Many developers forget to begin and commit transactions, leading to data integrity issues or incomplete operations.
The Solution
Always ensure you manage transactions using EntityTransaction
. Here is a simple pattern:
EntityManagerFactory emf = Persistence.createEntityManagerFactory("my-persistence-unit");
EntityManager em = emf.createEntityManager();
EntityTransaction transaction = em.getTransaction();
try {
transaction.begin();
// Perform operations
transaction.commit();
} catch (Exception e) {
if (transaction.isActive()) {
transaction.rollback();
}
e.printStackTrace();
} finally {
em.close();
}
Why: Encapsulating operations within transactions ensures data consistency, protecting against partial updates that could corrupt your database state.
3. Ignoring Cascade Types
The Pitfall
Ignoring or incorrectly configuring cascade types can lead to orphaned records or poorly managed entity states.
The Solution
Use the appropriate cascading options when setting up your entity relationships. Example:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(cascade = CascadeType.ALL, mappedBy = "product")
private List<Review> reviews;
// Getters and Setters
}
Why: Setting CascadeType.ALL
allows Hibernate to handle associated records automatically. When you save or delete a product, its reviews will follow suit.
4. Mismanaging Entity States
The Pitfall
Not understanding the lifecycle of entities (managed, detached, transient, removed) can lead to unexpected consistency errors in your application.
The Solution
Learn how entity states operate in Hibernate. For example, eagerly attaching entities to the session:
User user = new User();
user.setName("Alice");
// Attach to the EntityManager
em.persist(user);
Why: Understanding these states enables more controlled and predictable behavior regarding how your entities interact with the Hibernate session. Be mindful of using merge()
and detach()
methods appropriately.
5. Using Unoptimized Queries
The Pitfall
While JPA's Criteria API or JPQL abstract away SQL complexities, they can lead to unoptimized queries, especially as applications scale.
The Solution
Always consider query performance—use named queries or the Criteria API efficiently. Check the generated SQL for optimization:
@NamedQuery(
name = "User.findAll",
query = "SELECT u FROM User u"
)
Why: Named queries can offer performance boosts by preventing runtime parsing of JPQL every time the query is called.
6. Failing to Establish Proper Indexing
The Pitfall
Not indexing your database can lead to slow query performance and application bottlenecks.
The Solution
Make use of database indexes when creating large tables or running frequent queries. Add indexes when needed:
CREATE INDEX idx_user_name ON users (name);
Why: Indexes enhance search speed, making your application more responsive. Be careful to balance indexing with write speed, as too many indexes can slow down insert/update operations.
7. Overlooking Transaction Isolation Levels
The Pitfall
Understanding transaction isolation levels in Hibernate is essential. Misconfigurations can lead to dirty reads, phantom reads, and other anomalies.
The Solution
Set the transaction isolation level to meet your application’s consistency needs:
transaction.setIsolationLevel(Connection.TRANSACTION_SERIALIZABLE);
Why: Setting the right isolation level ensures your application behaves correctly under concurrent access. It’s a crucial aspect in maintaining data integrity.
Lessons Learned
While Hibernate JPA offers numerous advantages for Java applications, developers must remain vigilant about potential pitfalls. By understanding and avoiding the common issues outlined in this post, you can greatly enhance your application's performance and reliability.
Recommended Resources:
When approaching Hibernate JPA, keep the best practices in mind. Armed with this knowledge, you can confidently design robust applications that make the most of the powerful features provided by Hibernate. Happy coding!