Common Mistakes to Avoid with Builder Design Pattern
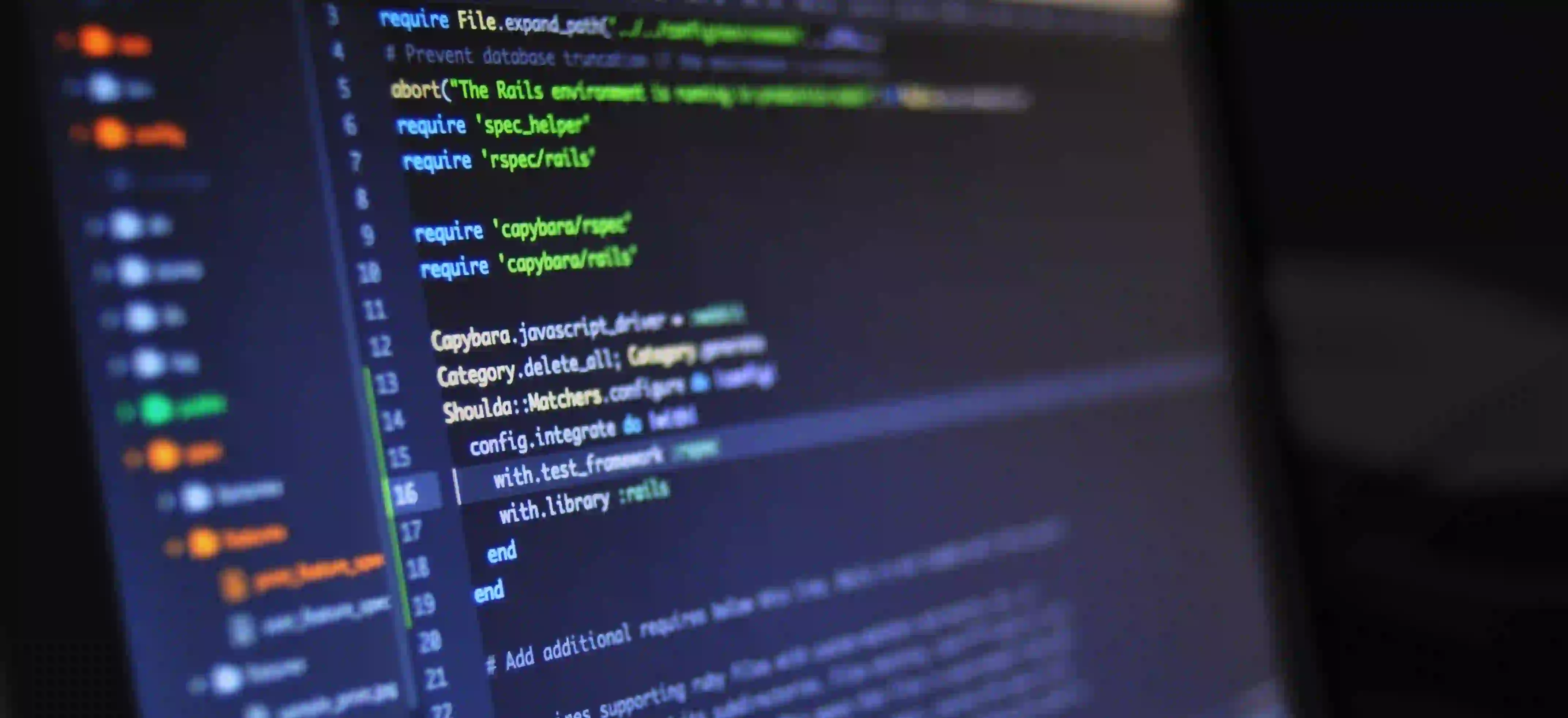
Common Mistakes to Avoid with the Builder Design Pattern
The Builder design pattern is one of the most powerful patterns in software design, specifically useful when constructing complex objects. It encapsulates the construction of a product and allows you to create different representations of that product. While the Builder pattern has its strengths, there are common mistakes developers make when implementing it. This blog post will discuss these mistakes and provide clear suggestions to help you avoid them.
Understanding the Builder Design Pattern
Before diving into the mistakes to avoid, it's essential to understand what the Builder design pattern is and how it functions. The Builder pattern separates the construction of a complex object from its representation. This allows the same construction process to create various representations.
Example Code Snippet
Here is a simple example to illustrate the Builder pattern in Java:
public class Car {
private String color;
private String model;
private int year;
private Car(CarBuilder builder) {
this.color = builder.color;
this.model = builder.model;
this.year = builder.year;
}
public static class CarBuilder {
private String color;
private String model;
private int year;
public CarBuilder setColor(String color) {
this.color = color;
return this;
}
public CarBuilder setModel(String model) {
this.model = model;
return this;
}
public CarBuilder setYear(int year) {
this.year = year;
return this;
}
public Car build() {
return new Car(this);
}
}
}
In this example, the Car
class represents the complex object we are constructing. The CarBuilder
class simplifies the instantiation of Car
and provides a fluent interface, enabling a cleaner and more readable object creation process.
Common Mistakes to Avoid
1. Overcomplicating the Builder
Mistake: Developers often implement the Builder pattern only for trivial classes or when not required, increasing unnecessary complexity.
Solution: Use the Builder pattern only when constructing an object has multiple parameters, especially when those parameters have defaults or optional configurations. If a class has just a couple of fields, consider using a simple constructor instead.
2. Making the Builder Immutable
Mistake: Making the builder immutable may sound appealing but can lead to limitations.
Solution: Builders should maintain mutability to allow chaining method calls. This enhances readability and makes the construction process fluid.
3. Not Validating the Inputs
Mistake: Failing to check for validity in the provided parameters can lead to the creation of an invalid object.
Solution: Implement validation within the build()
method or in the builder's setters. It ensures that when the object is finally constructed, it will meet intended constraints or requirements.
public Car build() {
if (color == null || model == null || year <= 0) {
throw new IllegalArgumentException("Invalid parameters for Car creation");
}
return new Car(this);
}
4. Keeping the Builder Class Public
Mistake: Making the builder too public can expose unnecessary implementation details.
Solution: Consider making the builder static and package-private if possible. This encapsulation reduces the exposure of internal workings and promotes cleaner APIs.
5. Overusing Static Builder Methods
Mistake: Using static methods for every configuration can lead to multiple builders unnecessarily.
Solution: When practical, use the inner Builder class instead of static builder methods, enabling more coherent usage while maintaining organization.
Car myCar = new Car.CarBuilder()
.setColor("Red")
.setModel("Sedan")
.setYear(2023)
.build();
6. Ignoring Thread Safety
Mistake: Builders that are mutable and shared between threads can lead to concurrency issues.
Solution: If you anticipate your builder might be accessed from multiple threads, consider using synchronization or, alternatively, creating a new instance of the builder for each construction process.
7. Inconsistent Naming Conventions
Mistake: Using inconsistent naming conventions for builder methods can confuse users of the API.
Solution: Ensure method names are consistent and expressive. For instance, if you use setX
for one parameter, use it for all parameters. This clarity enhances usability.
8. Not Considering a Fluent Interface
Mistake: Developers may not leverage the fluent interface that Builders facilitate.
Solution: Implement method chaining in your builder methods. This means returning this
at the end of your methods, which allows the user to call multiple methods in a single line.
public CarBuilder setColor(String color) {
this.color = color;
return this; // Enables chaining
}
When to Use the Builder Pattern
The Builder design pattern shines in scenarios that involve:
- Creating complex objects with many constructor parameters, especially when not all need to be filled.
- Handling optional parameters for an object.
- Constructing an object that requires multiple steps or validations before it can be considered valid.
In Conclusion, Here is What Matters
When used correctly, the Builder design pattern can significantly improve the readability and maintainability of your code. Avoiding the common mistakes discussed throughout this post will not only refine your implementation but also ensure that the Builder pattern serves its purpose efficiently.
If you're interested in further reading about design patterns in Java, I recommend checking out Oracle's documentation on design patterns for more insights.
By understanding and sidestepping these pitfalls, you will harness the true power of the Builder design pattern and improve the overall quality of your Java applications.