Mastering Clean Code: Boost Your Test Automation Skills
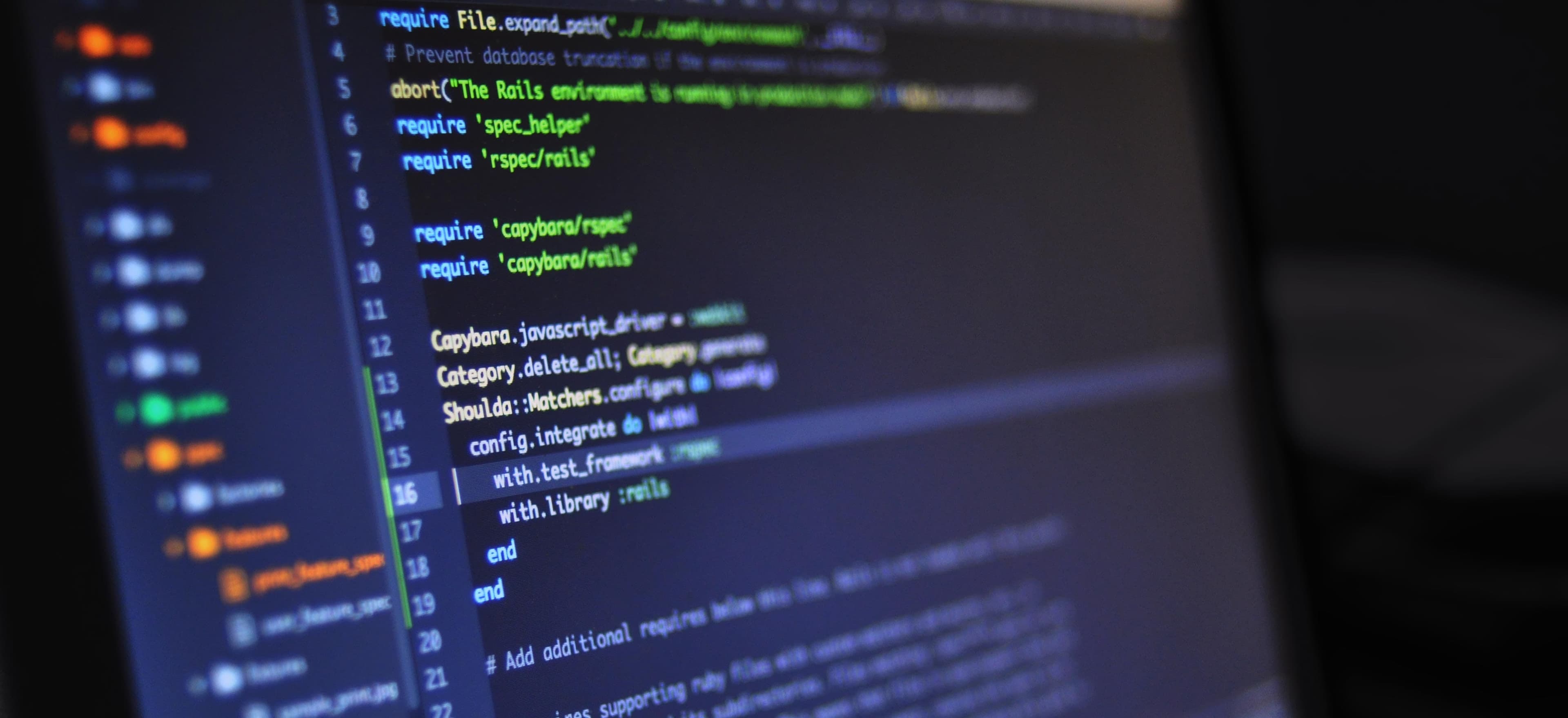
- Published on
Mastering Clean Code: Boost Your Test Automation Skills
In the realm of software development, the ability to write clean, maintainable code is indispensable. This is not just a matter of aesthetics; it's about ensuring that your codebase is easily navigable and testable. In the context of test automation, embracing clean code principles can significantly enhance your efficiency and the reliability of your test suites.
In this blog post, we will delve into the importance of clean code in test automation, present essential clean coding principles, and showcase some Java snippets that illustrate these concepts.
Why Clean Code Matters in Test Automation
When working on test automation, the main objectives are to ensure that your tests are reliable, maintainable, and scalable. Clean code plays a pivotal role in achieving this. Some key reasons include:
- Improved Readability: Clean code improves readability, making it easier for teams to understand your tests. This is critical when multiple developers work on a test suite.
- Reduced Maintenance Costs: Well-structured code reduces the cost and effort required to maintain and update the codebase.
- Easy Debugging: Identifying the root cause of test failures becomes simpler when the code is clean and well-organized.
- Scalable Framework: Clean code facilitates the enhancement and scaling of your test suite as your application evolves.
Understanding Clean Code Principles
1. Meaningful Naming
Names are an essential facet of clean Java code. They should convey the purpose and functionality of variables, classes, and methods.
// Bad Naming
int n;
// Good Naming
int numberOfTestCases;
Why It Matters
Meaningful names improve code readability. Instead of deciphering what "n" represents, anyone reading the code can instantly grasp that it refers to the number of test cases.
2. Small Functions
Limited function length leads to better clarity. Ideally, functions should have one responsibility.
// Bad Function
public void processUserInfo(User user) {
if (userIsValid(user)) {
saveUser(user);
sendEmailNotification(user);
updateUserAnalytics(user);
}
}
// Good Function
public void processUser(User user) {
if (userIsValid(user)) {
saveUser(user);
}
}
public void notifyUser(User user) {
sendEmailNotification(user);
updateUserAnalytics(user);
}
Why It Matters
When a function does one thing, it is easier to test the function in isolation. More granular functions improve both readability and recency.
3. Avoiding Comments When Possible
If your code requires extensive comments, you might need to refine it. Well-structured code should tell the story itself.
// Bad Code with Comments
// Check if user is valid
if (user.isValid()) {
// Save user to database
saveUser(user);
}
// Good Code
if (user.isValid()) {
saveUser(user);
}
Why It Matters
Comments can become outdated or misleading. Striving for self-explanatory code minimizes the risk of confusion.
4. Consistent Formatting
Maintain consistency in your code formatting. Adhering to standard conventions, such as indentation and spacing, helps maintain clarity.
// Bad Formatting
public void testUser() {
User user=new User("test");
if(user.isValid()){System.out.println("Valid");}
}
// Good Formatting
public void testUser() {
User user = new User("test");
if (user.isValid()) {
System.out.println("Valid");
}
}
Why It Matters
Consistent formatting aids readability and allows team members to navigate the code swiftly.
5. Test-Driven Development (TDD)
Implementing clean code principles in conjunction with Test-Driven Development is a powerful combination. Writing tests before coding encourages you to think about design and leads to cleaner, more efficient code.
public class UserTest {
@Test
public void testUserCreation() {
User user = new User("John", "Doe");
assertNotNull(user);
assertEquals("John", user.getFirstName());
assertEquals("Doe", user.getLastName());
}
}
Why It Matters
TDD aligns tests with established specifications, reinforcing the importance of clean and functional code.
Code Example: Building a Simple Test Automation Framework
Let’s put these principles into action by building a minimal Java test automation framework. This example will demonstrate how to apply clean coding practices while creating a simple test case scenario using JUnit.
Step 1: Create a User class
In a real-world scenario, you’d have various classes. For this example, we’ll create a User
class to demonstrate clean coding principles.
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public boolean isValid() {
return firstName != null && !firstName.isEmpty()
&& lastName != null && !lastName.isEmpty();
}
}
Step 2: Create a Test Class
Now, we will create a testing class following the TDD approach.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
@Test
public void testUserCreation() {
User user = new User("Jane", "Doe");
assertNotNull(user);
assertEquals("Jane", user.getFirstName());
assertEquals("Doe", user.getLastName());
}
@Test
public void testUserValidation() {
User user = new User("Jane", "");
assertFalse(user.isValid(), "User should not be valid with empty last name");
}
}
Why This Framework Works
- Readability: Each test is descriptive and focuses on a specific aspect of the
User
class. - Simplicity: The framework is minimal yet functional, allowing for easy expansion.
- Isolated Tests: Each test carefully examines the functionality of the
User
, promoting solid practices.
Final Considerations
Mastering clean code is not merely about making your software more visually appealing; it is about creating robust, effective, and easily maintainable tests. By adhering to naming conventions, maintaining a clear structure, and adopting methodologies like TDD, you can dramatically improve your test automation capabilities.
For more insights and resources on clean code principles, consider visiting Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin. Implement these practices and witness a transformation in your development workflow!
By integrating clean coding standards into your test automation practices, you can ensure not just operational efficiency, but also contribute valuably to your team's collective knowledge and skills. Remember, clean code is not just a personal asset; it is a team benefit!