Common Pitfalls in Java Puzzlers: Learn to Avoid Them!
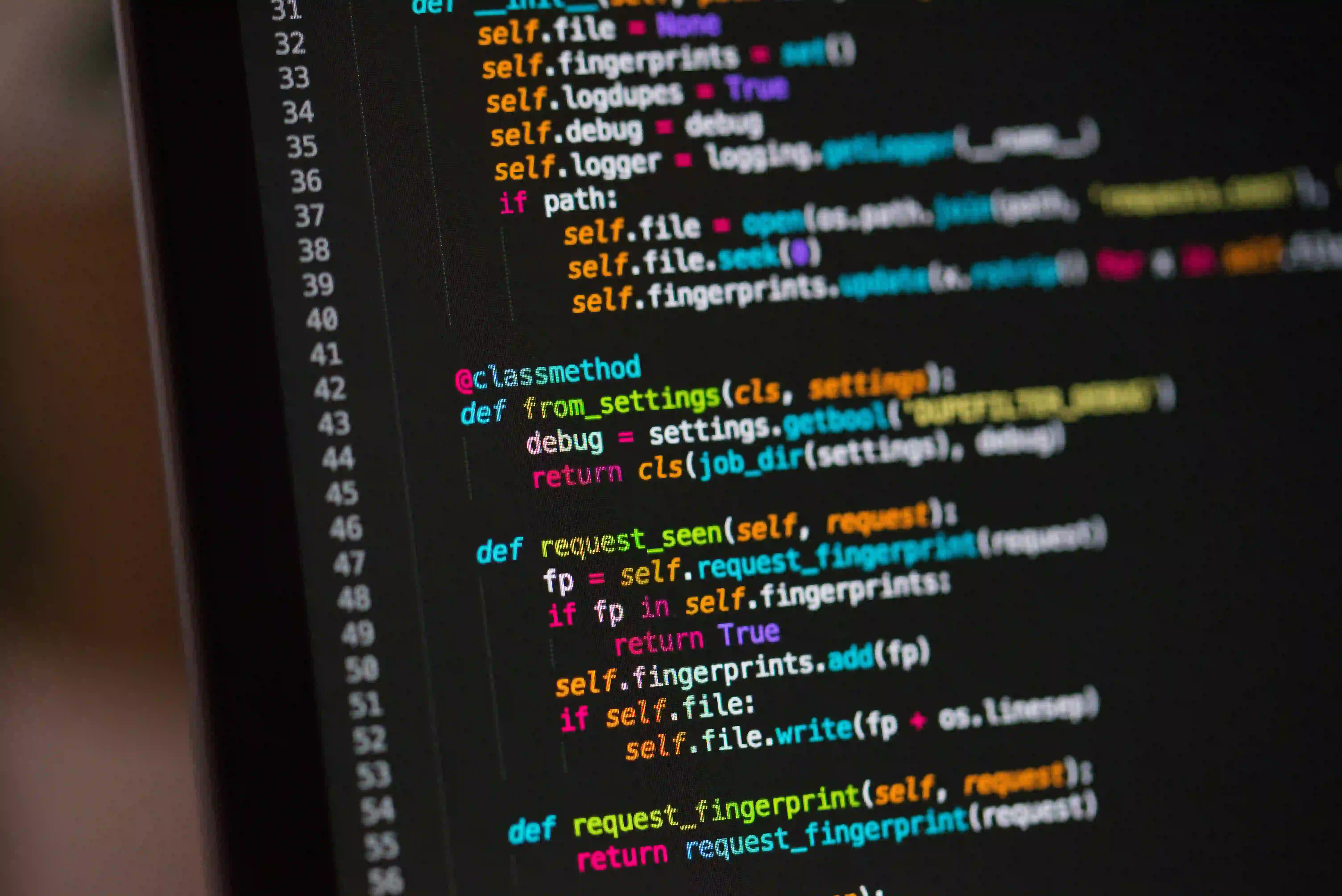
Common Pitfalls in Java Puzzlers: Learn to Avoid Them!
Java is a powerful, high-level programming language known for its portability and robustness. However, like any programming language, it comes with its own set of quirks and nuances. One way to uncover these quirks is through Java Puzzlers, a fantastic book by Joshua Bloch and Neal Gafter that exposes various unexpected behaviors in Java. In this blog post, we will explore some common pitfalls highlighted in Java Puzzlers, and provide actionable insights to help you avoid these traps.
What Are Java Puzzlers?
Java Puzzlers is a collection of puzzling problems that challenge conventional wisdom about the Java programming language. These puzzles often reveal surprising behaviors that could lead to incorrect assumptions or errors in logic. Understanding these puzzlers helps developers become more proficient in Java, avoiding common mistakes.
1. Equality and Identity Confusion
One of the most fundamental concepts in Java is the difference between reference equality and object equality. Java provides two main methods for comparing objects:
==
checks for reference equality..equals()
checks for logical equality.
Example
String a = new String("hello");
String b = new String("hello");
System.out.println(a == b); // false
System.out.println(a.equals(b)); // true
Commentary
In the above example, a
and b
are two different instances in memory, so a == b
is false. However, they hold the same content, making a.equals(b)
true. Always ensure you use .equals()
when comparing the content of objects instead of their references. Using the wrong comparison can lead to unexpected behavior, especially when working with collections or data structures.
More to Explore
For further reading on the intricacies of equals and hashCode, refer to Effective Java by Joshua Bloch.
2. Floating Point Precision Issues
Floating-point numbers can lead to precision issues, which many developers are often unaware of. This can result in incorrect calculations.
Example
double a = 0.1 + 0.2;
System.out.println(a == 0.3); // false
Commentary
The above statement might intuitively seem true, but due to the binary representation of floating-point numbers, the result of 0.1 + 0.2
is not exactly 0.3
. Instead, it's slightly greater. When working with decimals, consider using BigDecimal
for accuracy.
import java.math.BigDecimal;
BigDecimal b1 = new BigDecimal("0.1");
BigDecimal b2 = new BigDecimal("0.2");
BigDecimal b3 = new BigDecimal("0.3");
System.out.println(b1.add(b2).equals(b3)); // true
More to Explore
For an in-depth exploration of floating-point arithmetic, check out the IEEE 754 standard and how Java implements it.
3. Misunderstanding Static Initialization Blocks
Static initialization blocks can prove to be quite puzzling, especially when combined with class loading. Static blocks execute in the order that they appear, and only when the class is loaded.
Example
public class Test {
static int x;
static { x = 5; }
static { x *= 2; }
public static void main(String[] args) {
System.out.println(x); // 10
}
}
Commentary
Here, we define x
as a static variable. The static blocks run in the order defined, so x
is first assigned 5 and then multiplied by 2, resulting in a final value of 10. Such behaviors can be surprising, especially when many variables are being initialized.
More to Explore
For more on class loading and initialization, check the Java Language Specification.
4. Overusing final
Modifier
Using the final
keyword can cause confusion in Java, especially when applied to method parameters, local variables, or types.
Example
final int x = 10;
x = 20; // This will cause a compilation error
Commentary
When you declare a variable as final
, it cannot be reassigned. This is useful for constants but can lead to confusion if you are attempting to change a variable's value later in code. Use final
judiciously, keeping in mind that while it enforces immutability, excessive use can lead to code that is difficult to manage.
More to Explore
For more about the nuances of the final
keyword, the Java tutorial on the final keyword is helpful.
5. Exception Handling Pitfalls
Java’s exception handling model can be misleading, especially concerning the checked and unchecked exceptions.
Example
void method() throws IOException {
throw new IOException("An I/O error occurred.");
}
public static void main(String[] args) {
method(); // checked exceptions need to be declared or caught
}
Commentary
In the above snippet, the method()
declares that it may throw an IOException
— a checked exception. Any method that calls method()
must either handle that exception or declare that it too can throw it. Many developers overlook this, leading to compilation errors. It’s often best to use unchecked exceptions (subclasses of RuntimeException
) when possible.
More to Explore
You may refer to the Java Documentation on Exceptions for better handling techniques.
The Bottom Line
Java is a rich and complex language with many features and pitfalls. The Java Puzzlers offer valuable insights into some of its quirks, but they’re not just about code puzzles; they teach important lessons about the language. By understanding these common traps—such as equality vs. identity, floating-point precision, static initialization blocks, misuse of final
, and exception handling—you can write more robust and maintainable Java code.
Remember, the key to becoming a more proficient Java developer is to continually learn and adapt, keeping an eye on how the language evolves. So dive deeper into Java Puzzlers, and stay on top of the latest Java developments!
For more topics on Java, check out Java SE Documentation or follow my blog for future posts. Happy coding!