Decoding Data: Overcoming Storage Limitations Effectively
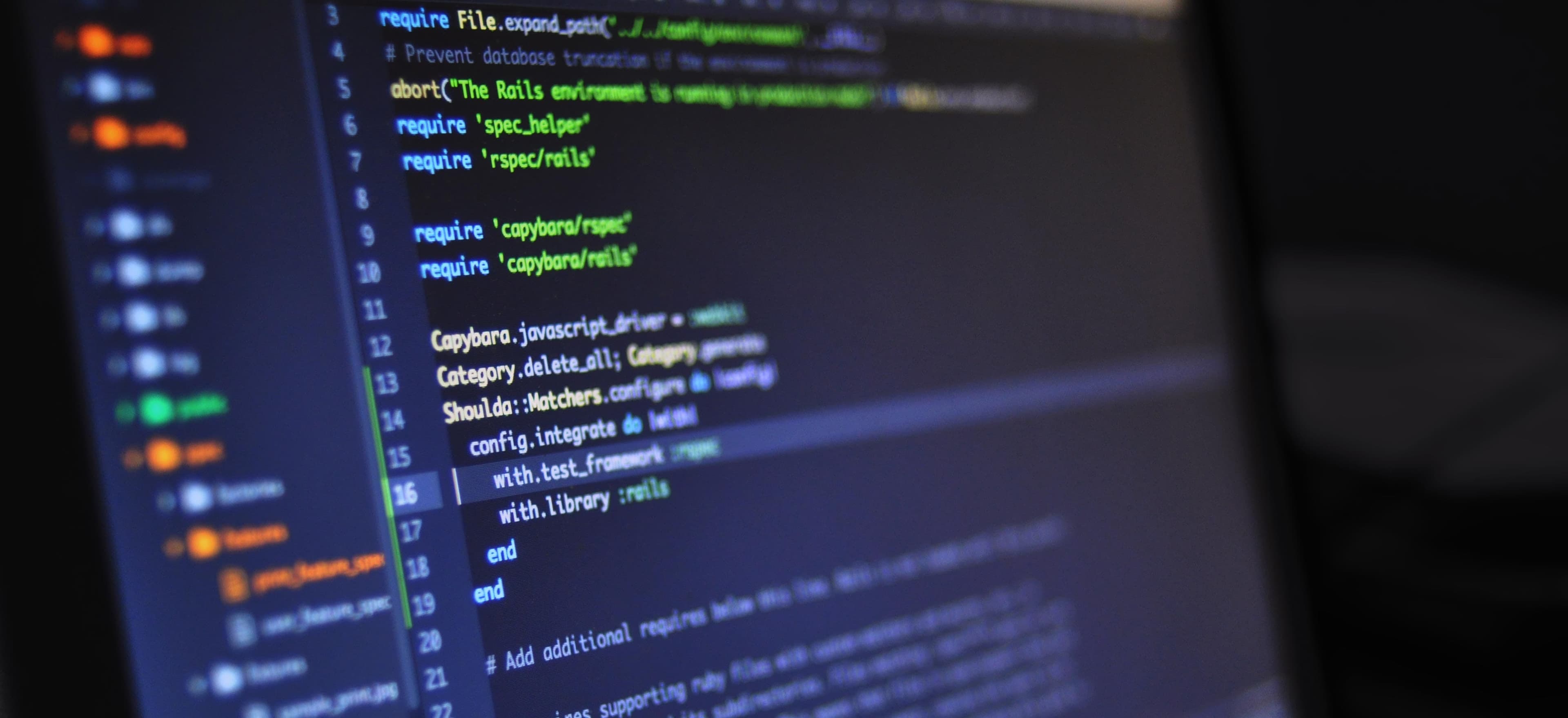
- Published on
Decoding Data: Overcoming Storage Limitations Effectively
In today's fast-paced digital world, big data is a key player in driving business decisions, scientific research, and technological advancements. While the term "big data" is often thrown around, the underlying challenge remains: how to store, manage, and interpret vast amounts of information efficiently. In this blog post, we'll explore effective strategies for overcoming storage limitations, focusing on Java for implementation.
Understanding Data Storage Challenges
As data continues to expand exponentially, traditional storage methods can become cumbersome. Here are some common pitfalls:
- Scalability Issues: As data volume magnifies, systems may struggle to maintain performance.
- Read/Write Speed: High latency can suppress efficiency, affecting real-time applications.
- Data Integrity: With large datasets, ensuring accuracy and consistency can be challenging.
- Cost Implications: High storage requirements often translate into increased costs.
Navigating these challenges requires innovative strategies for data management. Let's delve into some of these methodologies.
1. Implementing Database Sharding
Database sharding is a technique that involves splitting a large database into smaller, more manageable pieces, or shards. Each shard operates independently, allowing for increased read/write speeds and more efficient resource usage.
Example: Sharding with Java
import java.util.HashMap;
import java.util.Map;
// A simple representation of a Sharding mechanism
public class Shard {
private Map<Integer, String> dataStore = new HashMap<>();
// Method to add data to a specific shard
public void addData(int id, String data) {
dataStore.put(id, data);
}
// Method to retrieve data from a specific shard
public String getData(int id) {
return dataStore.get(id);
}
}
Why This Matters: By sharding data, we significantly reduce the load on individual database instances, allowing for quicker access times. This is especially critical for applications with fluctuating data loads.
Additional Resources on Sharding
To better understand database sharding, consider reading this in-depth article on sharding.
2. Utilizing Cloud Storage Solutions
Cloud storage has revolutionized the way we approach data storage. High scalability, reliability, and flexible pricing models make cloud services like Amazon S3 and Google Cloud Storage ideal for managing massive datasets.
Example: Interfacing with Amazon S3
Before diving into code, ensure you have the AWS SDK for Java included in your project.
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.PutObjectRequest;
import java.io.File;
// Uploading a file to S3
public class S3Uploader {
private AmazonS3 s3;
public S3Uploader() {
s3 = AmazonS3ClientBuilder.standard().withRegion("us-west-2").build();
}
public void uploadFile(String bucketName, String keyName, String filePath) {
File file = new File(filePath);
s3.putObject(new PutObjectRequest(bucketName, keyName, file));
System.out.println("File uploaded successfully.");
}
}
Why This Matters: The versatility of cloud storage enables you to scale your storage needs according to data growth without the burden of physical hardware maintenance.
3. Data Compression Techniques
Data compression is all about reducing the size of data, which means saving storage space and improving data transfer speeds. Various algorithms, like GZIP or LZ4, can effectively compress data.
Example: Using GZIP in Java
import java.io.*;
import java.util.zip.GZIPOutputStream;
// Method to compress data
public class DataCompressor {
public void compressFile(String sourceFile, String destinationFile) throws IOException {
try (FileInputStream fis = new FileInputStream(sourceFile);
FileOutputStream fos = new FileOutputStream(destinationFile);
GZIPOutputStream gzipOS = new GZIPOutputStream(fos)) {
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) != -1) {
gzipOS.write(buffer, 0, length);
}
}
System.out.println("File compressed successfully.");
}
}
Why This Matters: Compression not only saves space but can also enhance network transfer speeds, which is crucial for applications dealing with large datasets.
4. Caching Strategies
Sometimes, the best way to alleviate storage pressures is to implement caching strategies. Caching allows frequently accessed data to reside closer to the application, reducing latency and improving speed.
Example: Implementing Caching in Java with Guava
Make sure to add Guava as a dependency to your project.
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
import java.util.concurrent.TimeUnit;
public class SimpleCache {
private Cache<Integer, String> cache;
public SimpleCache() {
cache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build();
}
public void putData(int key, String value) {
cache.put(key, value);
}
public String getData(int key) {
return cache.getIfPresent(key);
}
}
Why This Matters: A well-implemented cache system reduces the load on the primary database and improves application responsiveness.
Wrapping Up
As we navigate through the digital information age, it's clear that overcoming data storage limitations is paramount for successful operations. From implementing sharding and utilizing cloud storage solutions to adopting data compression techniques and caching strategies, we have various means to manage massive datasets effectively.
Each of these strategies comes with its advantages and suits different contexts. The key is to assess your specific needs and choose the methods that align best with your operational objectives. By leveraging tools like Java, we can craft more sophisticated data management systems that not only cope with today’s data deluge but also set the stage for future advancements.
For more detailed discussions on advanced data management techniques, you can check additional resources like Apache Kafka Documentation and AWS Documentation.
Together, let's embrace the challenge of big data head-on, transforming problems into opportunities for innovation and growth.