Troubleshooting Java Mail SSL Connection Issues
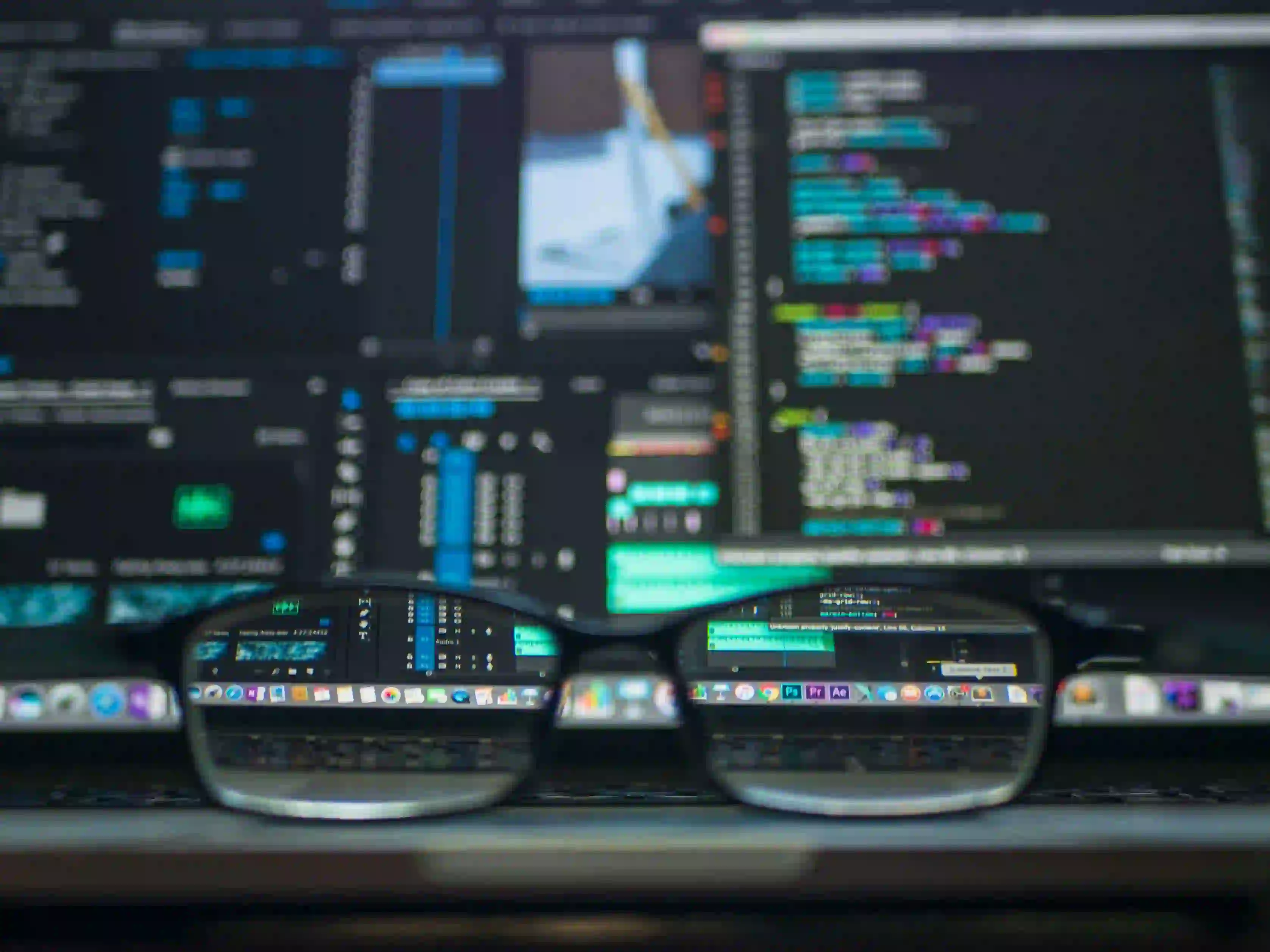
Troubleshooting Java Mail SSL Connection Issues
Sending emails programmatically is a common use case for many Java applications. JavaMail, the standard API for sending and receiving emails in Java, is a powerful tool for developers. However, like any technology, it can have its quirks and issues, particularly when dealing with SSL connections. In this blog post, we will explore common SSL connection issues that can arise while using JavaMail and how to troubleshoot them effectively.
Understanding JavaMail and SSL
JavaMail provides an easy-to-use API for sending mail from Java applications. It is built on top of the Java platform, allowing you to communicate with mail servers, whether local or remote. SSL (Secure Sockets Layer) is a protocol for establishing a secure connection over the internet, ensuring that data exchanged between the mail client and server remains private and protected from eavesdropping.
The javax.mail
package handles all aspects of email processing, including authentication, sending and receiving messages, and using SSL/TLS for secure communication. However, misconfigurations or environmental issues often lead to SSL connection failures.
Common SSL Connection Issues
1. Incorrect SSL Port Configuration
One of the simplest yet most common issues is using the wrong port for connecting to the mail server. SMTP servers typically use the following ports:
- 587: Used for secure submission with STARTTLS.
- 465: Used for SMTPS (SMTP over SSL).
- 25: Commonly used for non-encrypted SMTP.
How to Troubleshoot:
- Ensure that you are using the right port for your email service provider.
- If you are unsure about the correct port, check the provider's documentation.
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.example.com");
props.put("mail.smtp.port", "587"); // Ensure this matches the service provider's configuration.
props.put("mail.smtp.starttls.enable", "true"); // Use this for STARTTLS.
2. Java Security Settings
Java applications run with certain security settings that may prevent SSL connections. Especially, the JVM may not trust the mail server's SSL certificate.
How to Troubleshoot:
- Check if the certificate of your mail server is signed by a trusted Certificate Authority (CA).
- If it’s a self-signed certificate, you need to import it into your Java keystore.
keytool -import -alias smtp-server -file server.crt -keystore cacerts
Make sure you replace server.crt
with the path to your server’s certificate file.
3. Incorrect Username and Password
Authentication failures can also lead to SSL connection issues. Ensure that you are using the correct username (often the email address) and password for the SMTP server.
How to Troubleshoot:
- Confirm your email credentials with your provider.
- Ensure your application is handling credentials securely.
props.put("mail.smtp.auth", "true");
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("username@example.com", "password"); // Replace with your credentials
}
});
4. Firewall and Network Issues
Sometimes the issue lies outside of your application. Firewalls or network policies might block connections to the mail server's port.
How to Troubleshoot:
- Verify that your network allows outbound connections on the specified SMTP port.
- Temporarily disable the firewall to check if it's causing the issue.
5. JavaMail Session Debugging
JavaMail provides a debugging feature that can help diagnose connection issues. By setting the debug property to true, you can receive detailed logs about the email sending operation.
How to Enable Debugging:
props.put("mail.debug", "true"); // Turn on debugging
Session session = Session.getInstance(props, auth);
session.setDebug(true); // Enable debugging for the session
Transport.send(message);
The debug output will give you insight into what the JavaMail API is attempting to do, including the SSL handshake process, which can be invaluable in diagnosing problems.
6. Check SSL/TLS Protocols
Older versions of Java might not support the latest SSL/TLS protocols required by your mail server. For example, TLS 1.2 is preferred these days, and Java versions less than 7u95 do not support it.
How to Troubleshoot:
- Update your Java version to the latest, which will provide support for the latest protocols.
- Specify the desired SSL/TLS protocols in your properties if necessary.
props.put("mail.smtp.ssl.protocols", "TLSv1.2");
Example Code Snippet
Here’s a complete example of how to configure JavaMail with SSL:
import javax.mail.*;
import javax.mail.internet.*;
import java.util.Properties;
public class EmailSender {
public static void main(String[] args) {
String to = "recipient@example.com";
String from = "sender@example.com";
String host = "smtp.example.com";
final String username = "username@example.com";
final String password = "password";
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", host);
props.put("mail.smtp.port", "587");
props.put("mail.debug", "true");
Session session = Session.getInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to));
message.setSubject("Test Email");
message.setText("Hello, this is a test email!");
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (MessagingException e) {
e.printStackTrace();
}
}
}
Bringing It All Together
Troubleshooting Java Mail SSL connection issues involves understanding the configuration and environment. By carefully examining the points discussed in this blog post, developers can resolve common problems and ensure reliable email delivery in their applications. If you continue to experience issues, consider checking out the JavaMail official documentation for more detailed information and updates.
Engaging with SSL connections in JavaMail can seem daunting, but with the right understanding and troubleshooting techniques, you can efficiently resolve any issues that arise. Happy coding!