Overcoming Common Pitfalls in Command Design Pattern
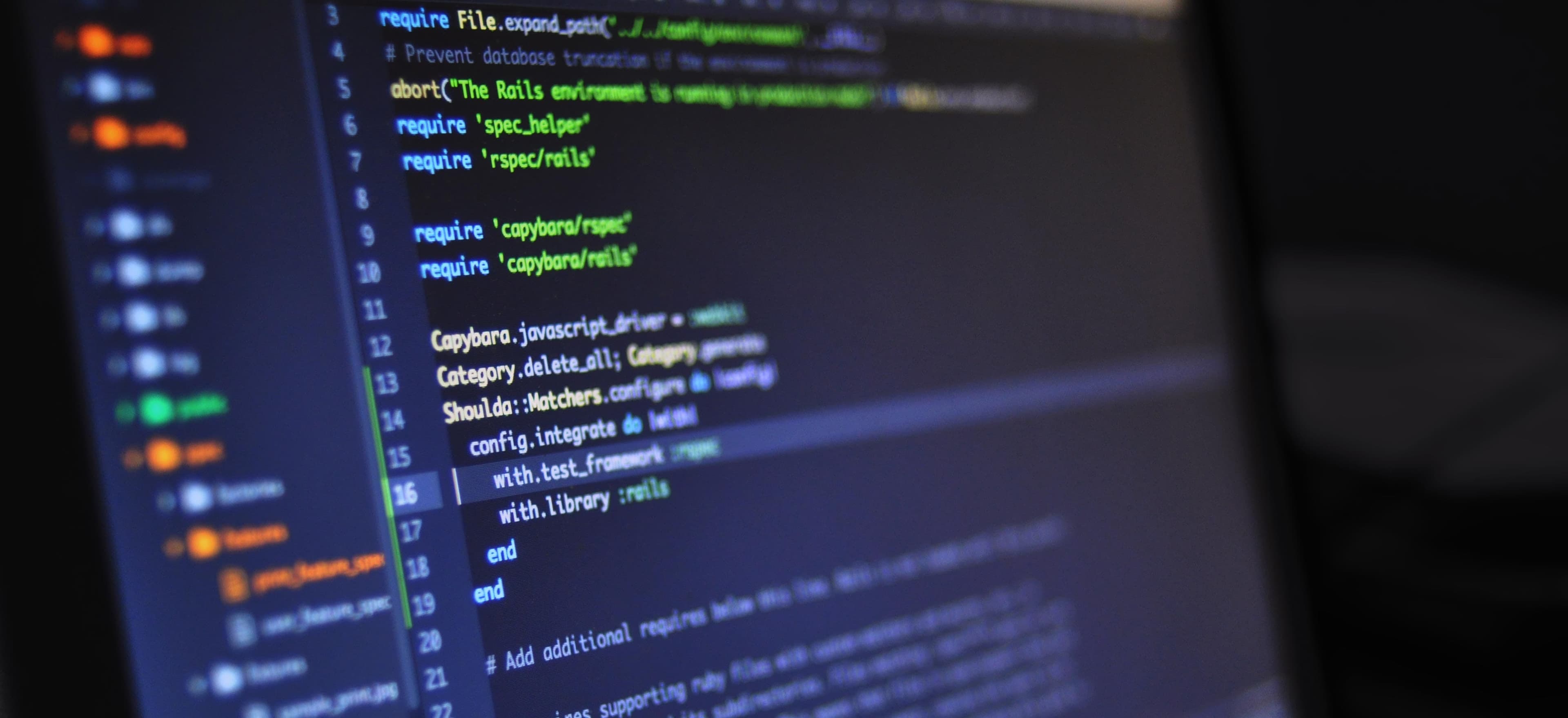
- Published on
Overcoming Common Pitfalls in Command Design Pattern
The Command Design Pattern is a powerful behavioral design pattern that encapsulates a request as an object, thereby allowing for parameterization of clients with different requests, queuing of requests, and logging of the requests. Additionally, it offers the capability to support undoable operations. However, like any other design pattern, improper implementation can lead to pitfalls that may hinder your application's performance and maintainability. This blog post will delve into the common pitfalls you may encounter while implementing the Command Design Pattern and how to overcome these challenges.
What is the Command Design Pattern?
Before diving into the pitfalls, let's briefly recap the Command Design Pattern. The core of this pattern revolves around four main components:
- Command: An interface that declares a method for executing operations.
- Concrete Command: A class that implements the Command interface and defines the binding between a Receiver and an action.
- Receiver: The object that contains the business logic to execute a command.
- Invoker: The object that knows how to execute a command, but does not know the details of the operation being performed.
To illustrate this, consider the following simple example:
Simple Example of Command Pattern
// Command interface
interface Command {
void execute();
}
// Receiver class
class Light {
public void turnOn() {
System.out.println("The light is on");
}
public void turnOff() {
System.out.println("The light is off");
}
}
// Concrete Command to turn on the light
class TurnOnLightCommand implements Command {
private Light light;
public TurnOnLightCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOn();
}
}
// Concrete Command to turn off the light
class TurnOffLightCommand implements Command {
private Light light;
public TurnOffLightCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOff();
}
}
// Invoker class
class RemoteControl {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
command.execute();
}
}
In this illustrative code, we see how the Command Design Pattern decouples the object that invokes the operation (the RemoteControl
) from the object that performs it (the Light
).
Common Pitfalls
1. Overuse of Commands
One of the most common pitfalls in the Command Design Pattern is the overuse of commands. It can be tempting to create a command for every minor operation or state change, leading to an overwhelming number of classes.
Solution: Group Related Operations
Instead of creating separate command classes for similar actions, consider grouping related operations into a single command class. This simplifies the design and enhances readability.
Example:
Instead of having separate commands for each light fixture, you could create a single command that can handle multiple fixtures.
class MultiLightCommand implements Command {
private List<Light> lights;
private boolean turnOn;
public MultiLightCommand(List<Light> lights, boolean turnOn) {
this.lights = lights;
this.turnOn = turnOn;
}
@Override
public void execute() {
for (Light light : lights) {
if (turnOn) {
light.turnOn();
} else {
light.turnOff();
}
}
}
}
2. Lack of Undo Functionality
The Command Design Pattern allows for undoable operations, yet some implementations neglect to incorporate this feature, thereby missing out on a strength of the pattern.
Solution: Implement Undo Mechanism
You can enhance your command implementations by maintaining a stack of executed commands that allow the user to undo the most recent actions.
Example:
import java.util.Stack;
class UndoableInvoker {
private Stack<Command> commandStack = new Stack<>();
public void executeCommand(Command command) {
command.execute();
commandStack.push(command);
}
public void undo() {
if (!commandStack.isEmpty()) {
commandStack.pop();
System.out.println("Last command undone.");
// You would typically call an undo method here
}
}
}
3. Tight Coupling Between Command and Receiver
Another common issue arises when commands are tightly coupled with their receivers. This can make testing difficult and reduce flexibility.
Solution: Use Dependency Injection
By employing dependency injection, you can make your commands less dependent on concrete receiver implementations.
Example:
class TurnOnLightCommand implements Command {
private Light light;
public TurnOnLightCommand(Light light) {
this.light = light;
}
// Use a factory or a provider to get the light instance
}
// In the context of a dependency injection framework
@Inject
public void setLight(Light light) {
this.light = light;
}
4. Ignoring Non-Command Logic in Commands
Sometimes, developers inadvertently include non-command logic, such as complex decision-making or data manipulation, within the command classes. This can result in a code smell known as "God Object."
Solution: Keep Commands Slim
Ensure that your commands focus solely on executing the command action. Complex business logic should be handled separately, within the receiver or a dedicated service class.
Example:
Instead of this:
class ComplexCommand implements Command {
public void execute() {
if (someComputation()) {
// business logic
}
}
}
Do this:
class BusinessLogic {
public boolean process() {
// Complex logic here
return true;
}
}
class SimpleCommand implements Command {
private BusinessLogic logic;
public SimpleCommand(BusinessLogic logic) {
this.logic = logic;
}
public void execute() {
if (logic.process()) {
// Command action here
}
}
}
5. Neglecting Thread Safety
If multiple commands are executed in a multithreaded environment, thread safety can become a concern, leading to unpredictable behavior.
Solution: Utilize Synchronization Where Necessary
Whenever shared states or resources are involved, consider using synchronization techniques to ensure thread safety.
Example:
class ThreadSafeCommand implements Command {
private final Object lock = new Object();
public void execute() {
synchronized(lock) {
// Command logic that modifies shared state
}
}
}
Lessons Learned
While the Command Design Pattern offers numerous advantages, avoiding its pitfalls is essential for maintaining clean, efficient, and scalable code. By being mindful of over-engineering, tight coupling, and other common issues, you can harness the true power of this pattern in your applications.
Would you like to learn more about design patterns? Consider exploring Refactoring Guru and GeeksforGeeks on Design Patterns. These resources provide excellent coverage of various design patterns with examples in multiple programming languages.
Embrace these insights and improve your command pattern implementations today!