Why Your Java Process Exceeds Xmx Memory Limits!
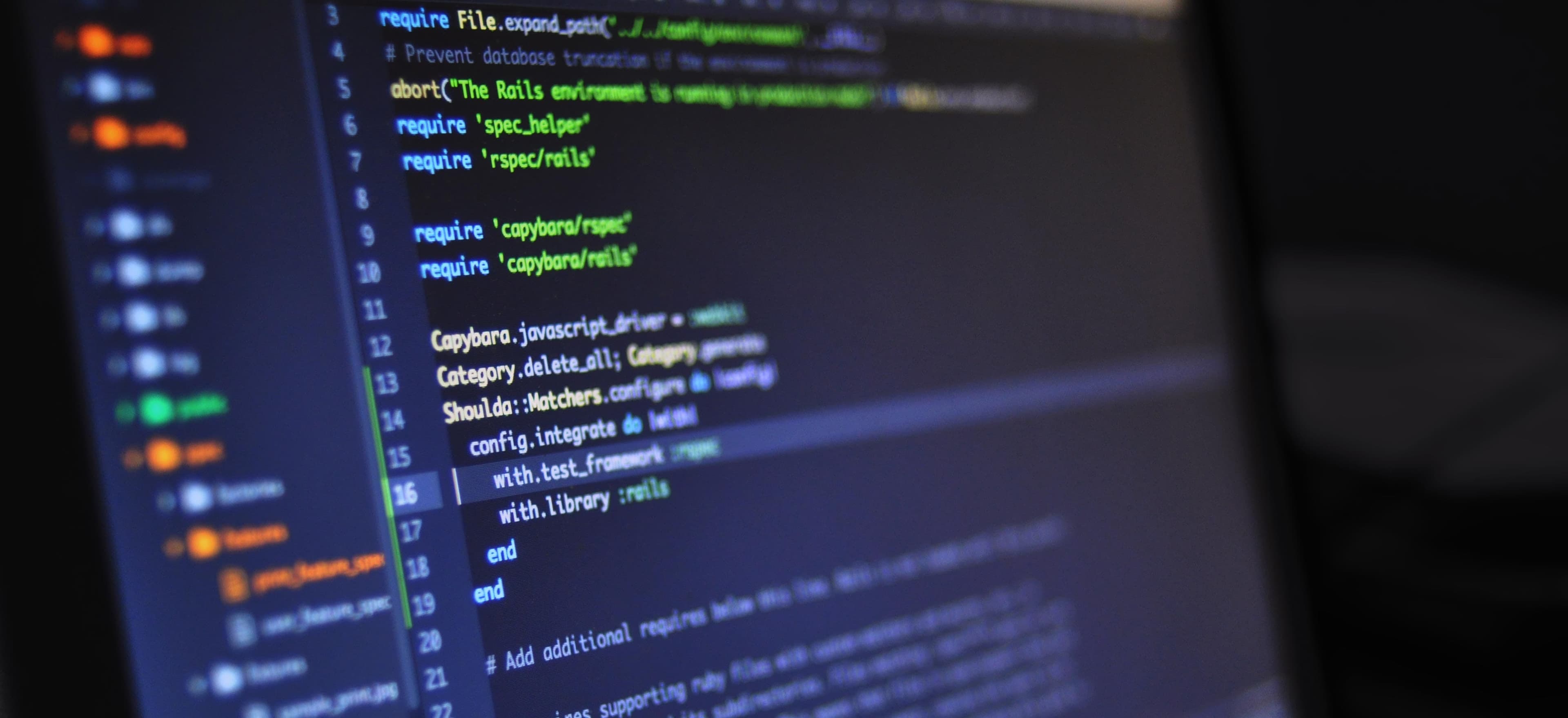
- Published on
Why Your Java Process Exceeds Xmx Memory Limits!
Java is a powerful language, widely used for its platform independence and strong community support. However, one common issue that Java developers face is their application exceeding the allocated heap memory, leading to OutOfMemoryError
. This error typically occurs when the Java Virtual Machine (JVM) runs out of memory allocated to it, specified by the -Xmx
flag. In this blog post, we will explore the reasons behind this memory limit breach, when it happens, and how you can mitigate it.
Understanding Xmx and How JVM Handles Memory
The -Xmx
option sets the maximum heap size for the JVM. For example, if you use the command line argument -Xmx512m
, you are telling the JVM that it cannot use more than 512 megabytes of heap memory. This is crucial because it prevents any application from consuming excessive memory resources that could affect the performance of the host machine or other applications.
The JVM memory model has several regions, including:
- Heap Memory: Where objects are allocated.
- Stack Memory: Used for method call frames and local variables.
- Method Area: For class metadata.
When you run a Java application, the heap memory is where most objects are stored. Poor memory management can lead to a scenario where the heap exceeds the specified Xmx limit, resulting in performance degradation or application crashes.
Reasons for Exceeding Xmx Memory Limits
Now that we have a basic understanding of memory management in Java, let's delve into the potential reasons why your Java application might exceed the Xmx memory limits.
1. Memory Leaks
One of the most common culprits is memory leaks. A memory leak occurs when the application retains references to objects that are no longer needed. For example, suppose you have a list that continuously accumulates items without ever clearing it. The memory it uses will not be released, leading to exhaustion.
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private static List<Object> leakList = new ArrayList<>();
public static void createMemoryLeak() {
while (true) {
leakList.add(new Object());
}
}
public static void main(String[] args) {
createMemoryLeak();
}
}
In this example, the leakList
continuously adds new Object
instances. The method never stops, and thus the heap memory will keep growing.
How to avoid this? Use weak references, and be careful when managing collections and caches. You can also manually clear the lists when they are not needed anymore.
2. Large Object Creation
Sometimes, the objects you are working with might be significantly larger than expected. For instance, handling images, videos, or large data sets can quickly consume the set memory.
import java.awt.image.BufferedImage;
public class LargeObjectExample {
public static void main(String[] args) {
// Creating a large image that could exceed memory limits
BufferedImage img = new BufferedImage(5000, 5000, BufferedImage.TYPE_INT_RGB);
// Operations on img...
}
}
In this case, the BufferedImage
of 5000x5000 pixels could quickly consume a lot of memory, especially if you create many of them or do not dispose of them when no longer needed.
Tips:
- Limit object size when possible.
- Consider streaming large datasets instead of loading them entirely into memory.
3. Unbounded Data Structures
Using unbounded data structures, such as ArrayList
, can lead to uncontrolled growth when elements are continuously added. If there are no limits imposed, your application may quickly exhaust memory.
import java.util.ArrayList;
import java.util.List;
public class UnboundedExample {
public static void main(String[] args) {
List<String> unboundedList = new ArrayList<>();
for (int i = 0; i < Integer.MAX_VALUE; i++) {
unboundedList.add("This is a long string that keeps getting added!");
}
}
}
Here, the unboundedList
keeps growing until it eventually runs out of memory.
4. Insufficient Xmx Configuration
Sometimes developers overlook or misconfigure the -Xmx
parameter. Make sure your application's memory settings correspond with the workload characteristics.
To set the maximum heap size, you can update your Java application startup commands as follows:
java -Xmx2048m -jar YourApp.jar
This command will allow up to 2GB of heap memory.
5. Too Many Threads
Creating too many threads can also lead to an OutOfMemoryError
, as each thread requires its own stack space. If you have poorly managed multithreading or excessive thread creation, you can quickly end up depleting memory resources.
public class ThreadExample {
public static void main(String[] args) {
while (true) {
new Thread(() -> {
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
}
}
In this example, an infinite number of threads are spawned, each consuming memory.
6. External Libraries
When using external libraries, it is crucial to ensure they manage memory properly. Memory leaks become difficult to control if they are present in a third-party library.
7. JVM Flags and Tuning
JVM tuning is crucial for optimal performance. The -Xms
(initial heap size) and -Xmx
(maximum heap size) flags should be set according to your application requirements. Neglecting to fine-tune these parameters may lead to suboptimal performance.
Monitoring and Profiling
To get a better understanding of your application's memory usage, employ profiling tools such as:
These tools can assist in identifying memory leaks and help optimize performance.
Bringing It All Together
Managing memory in Java is an essential skill for every developer. Understanding how the JVM handles memory and the common pitfalls can save you time and ensure a smoother development process. Always be on the lookout for memory leaks, avoid unbounded data structures, and ensure that you correctly configure your JVM options to optimize performance.
For more information about Java memory management, check out Oracle's Documentation on Java Memory Management. By keeping these tips in mind, you will pave the way for a robust and efficient Java application.
Happy coding!