Debugging R2DBC Issues in Reactor Applications: A Guide
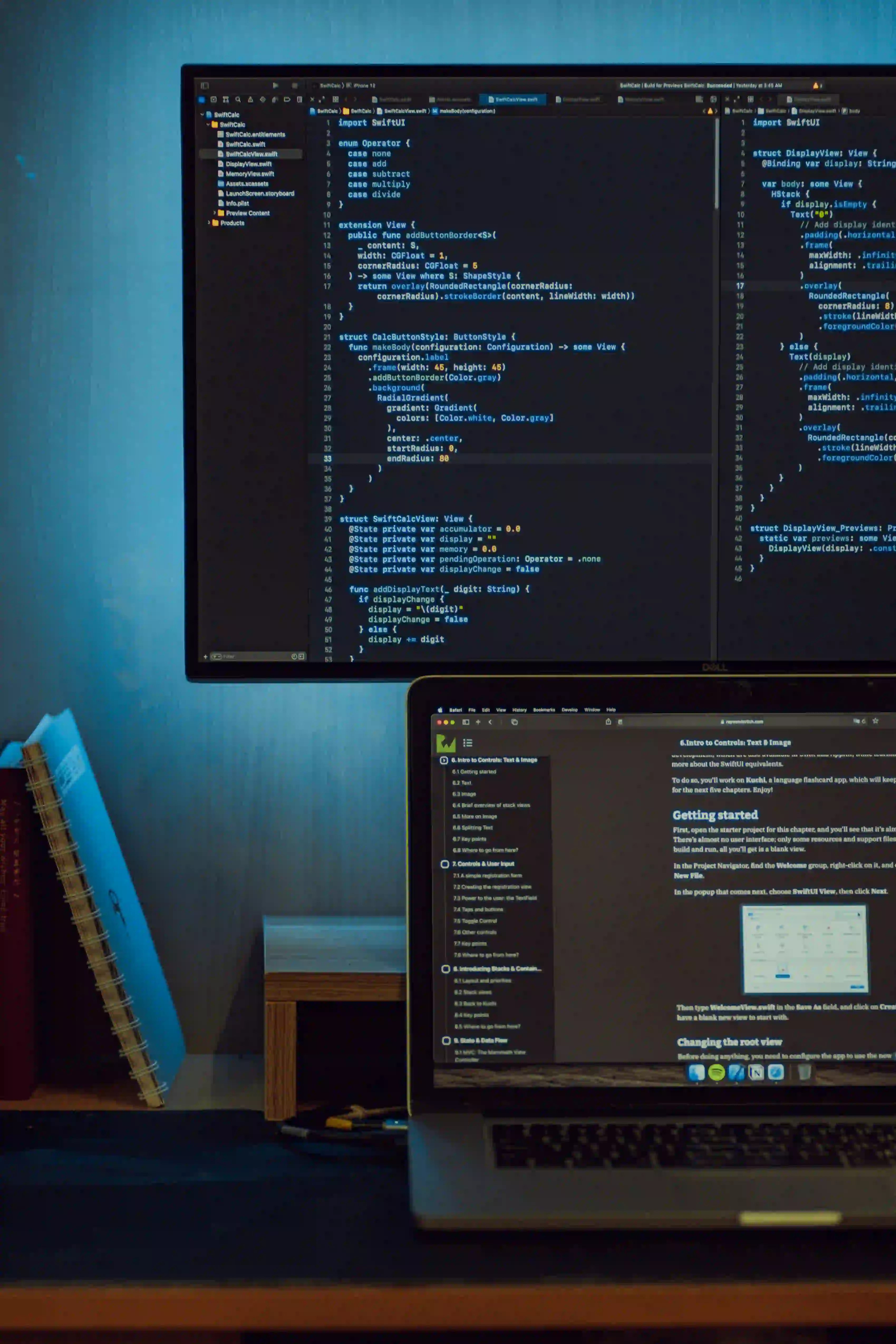
Debugging R2DBC Issues in Reactor Applications: A Guide
Reactor applications provide a rich ecosystem for developing responsive, event-driven applications in Java. When you couple Reactor with R2DBC (Reactive Relational Database Connectivity), you unlock the power of non-blocking databases, enabling efficient data access. However, debugging issues that arise during this asynchronous process can be challenging. In this guide, we’ll explore common R2DBC issues in Reactor applications and provide strategies for troubleshooting and resolving them effectively.
Understanding R2DBC and Reactor
R2DBC is a specification for reactive programming that allows developers to connect to relational databases in a non-blocking manner. It employs reactive streams to handle database interactions, making it suitable for high-throughput applications.
Reactor, on the other hand, is a popular reactive programming library for building non-blocking applications in Java. Together, R2DBC and Reactor foster a robust architecture for data-driven applications, but they also bring a set of unique challenges.
Why Debugging is Crucial
Debugging issues in Reactor applications using R2DBC is essential for maintaining application performance and reliability. As data accesses are executed asynchronously, timing issues, resource leaks, or configuration problems can lead to performance bottlenecks, inconsistent data states, or application crashes.
Common R2DBC Issues
1. Connection Issues
Connection problems can manifest when the application cannot reach the database, often due to configuration errors or network issues.
Symptoms:
- Unable to connect to the database.
- Timeouts or connection refused errors.
Solution:
Verify the database connection details (URL, username, password). For example, in a Spring Boot application, ensure your application.yml
contains the correct settings:
spring:
r2dbc:
url: r2dbc:mysql://localhost:3306/mydb
username: myuser
password: mypassword
Check your database logs for any authentication or access-denied messages. Network configurations, such as firewalls, should also be examined for potential blocks.
2. Backpressure Management
Backpressure occurs when a producer sends messages at a faster rate than the consumer can handle.
Symptoms:
- Application crashes or hangs.
- High memory usage.
Solution:
Utilize operators like onBackpressureBuffer
, onBackpressureDrop
, and onBackpressureLatest
in your data flow. They help manage the flow of data and prevent overwhelming subscribers.
Here's an example:
Flux<Integer> integerFlux = Flux.range(1, 100)
.onBackpressureBuffer(10); // Buffering 10 items;
integerFlux.subscribe(System.out::println);
By implementing backpressure controls, you can ensure that your application remains responsive even under heavy loads.
3. Timeout Errors
Timeout issues are prevalent in asynchronous programming when a query takes longer than expected.
Symptoms:
- Timeout exceptions during queries.
- Delayed responses in the application.
Solution:
Set explicit timeouts for database operations to handle cases where the query may take too long. For example:
databaseClient.select()
.from("employees")
.fetch()
.one()
.timeout(Duration.ofSeconds(5)) // Set a timeout of 5 seconds
.doOnError(e -> System.err.println("Error: " + e))
.subscribe();
By managing timeouts effectively, you can enhance the reliability of your applications.
4. Resource Leaks
It's vital to ensure that connections and resources are properly closed after usage. Resource leaks can lead to performance degradation and stability problems.
Symptoms:
- Increasing memory usage over time.
- Database connection limit reached errors.
Solution:
Always use the doFinally
operator to ensure resources are released after operations:
Mono<Employee> employeeMono = databaseClient.select()
.from("employees")
.matching(query -> query.where("id").is(1))
.fetch()
.one()
.doFinally(signalType -> {
// Clean up resources if necessary
System.out.println("Operation finished with signal: " + signalType);
});
Regularly monitor resource usage to detect leaks early.
5. Incorrect Query Results
When working with R2DBC, you might experience issues with incorrect or unexpected query results due to improperly structured queries.
Symptoms:
- Wrong data returned.
- Null or empty responses.
Solution:
Use debugging techniques, such as logging queries or inspecting the data returned. The following example logs SQL queries:
databaseClient.execute("SELECT * FROM employees")
.fetch()
.all()
.doOnNext(row -> System.out.println("Row: " + row))
.subscribe();
Incorporate meaningful logging to pinpoint the root cause of issues.
Implementing Best Practices
Beyond debugging, adopting best practices can greatly mitigate issues in R2DBC transactions. Here are a few to keep in mind:
Use the Latest Versions
Bug fixes and updates in libraries can significantly enhance the stability of your application. Always ensure you're using the latest versions of R2DBC, Reactor, and database libraries.
Monitor Performance
Integrate monitoring tools like Micrometer to gain insights into the performance of your reactive streams and database calls. This helps in identifying bottlenecks early.
Conduct Thorough Testing
Unit tests and integration tests should cover asynchronous and database-related functionality. Use tools like Mockito and JUnit to implement effective tests.
Educate Your Team
Ensure your team is well-versed in reactive programming principles and the R2DBC specification. Familiarity with concepts like reactive streams, operators, and backpressure is crucial for effective debugging.
My Closing Thoughts on the Matter
Debugging R2DBC issues in Reactor applications requires a thorough understanding of both technologies. By recognizing common problems, implementing best practices, and continuously monitoring your application, you can enhance its performance and stability.
To dive deeper into R2DBC, consult the official R2DBC documentation and to understand more about Reactor, check the Reactor project page.
As data-driven applications become increasingly essential, mastering the nuances of R2DBC and Reactor will position you for success in the reactive programming landscape.
By implementing the strategies outlined in this guide, you'll be well-equipped to tackle R2DBC-related challenges and ensure a smooth-running application. Happy debugging!