Breaking the Cycle: Why Dependency Management Matters
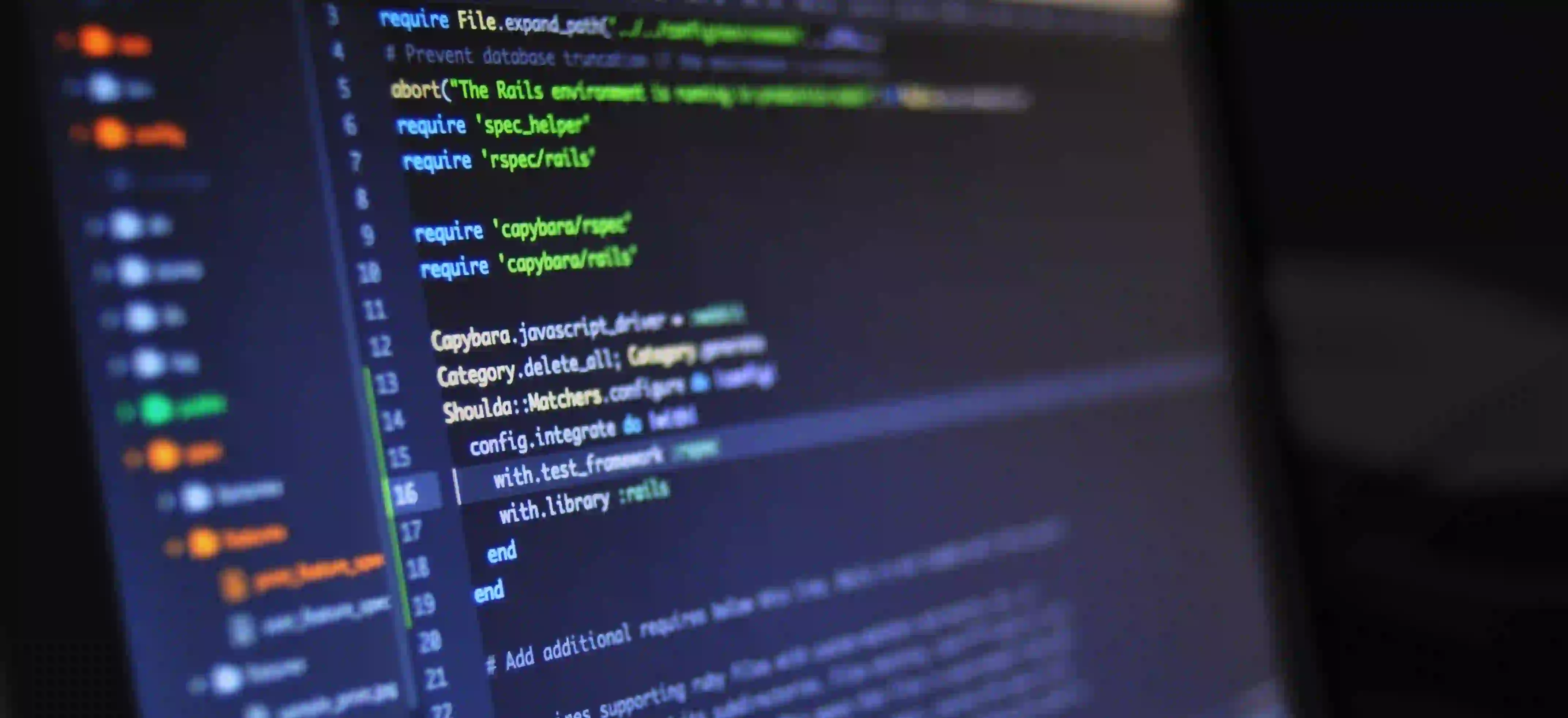
Breaking the Cycle: Why Dependency Management Matters
In the world of software development, dependency management is an essential yet often overlooked aspect of creating robust and maintainable applications. As projects grow in complexity, the importance of effectively handling dependencies becomes evident. This blog post will explore why dependency management matters, focusing particularly on Java applications. We will cover the consequences of poor dependency management, provide practical examples, and share best practices to help you break the cycle of dependency chaos.
Understanding Dependencies
In software development, a dependency is a library, framework, or module that a project relies on for its functionality. For instance, if you are developing a Java application that requires JSON parsing, you might use a dependency like Jackson or Gson. Here is a simple example of how you might include a dependency in your Java project using Maven:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.1</version>
</dependency>
Why Dependency Management is Critical
Dependency management is critical for several reasons:
-
Avoiding Conflicts: With multiple libraries potentially relying on different versions of the same dependency, conflicts can arise. This is known as "dependency hell." For example, if your project depends on
Library A
version 1.0 andLibrary B
requiresLibrary A
version 2.0, you’ll face compatibility issues. -
Ensuring Stability: Dependencies are often updated with new features, bug fixes, and security patches. By managing these dependencies effectively, you ensure that your project is stable, secure, and benefitting from the latest improvements in your libraries.
-
Simplifying Builds: Using a dependency management tool, such as Maven or Gradle, streamlines the building process. You can easily declare dependencies, and the tool will handle downloading and linking required libraries, reducing setup time and configuration errors.
-
Promoting Team Collaboration: In team environments, having a clear dependency management strategy ensures that all team members are using the same library versions. This reduces discrepancies when checking code into version control.
-
Improving Performance: Proper management leads to optimized performance by ensuring that only necessary libraries are included in the build.
The Consequences of Poor Dependency Management
Imagine a situation where you're heavily involved in a project, and over time, you accumulate libraries that are either outdated or conflicting. This is a recipe for disaster. Here’s a brief overview of what can go wrong:
-
Increased Build Times: As a project accumulates unnecessary dependencies, build times can significantly increase. You are likely to notice slower response times when trying to compile or package your application.
-
Security Vulnerabilities: Outdated libraries may contain security vulnerabilities. Failing to update can lead to serious security threats, especially given the constant emergence of new exploits.
-
Diminished Developer Productivity: Time spent troubleshooting dependency issues is time not spent writing actual code. Poorly managed dependencies can lead to frustration and burnout among developers.
-
Technical Debt: Technical debt accumulates when shortcuts are taken in development. The longer poor dependency management practices are ignored, the more technical debt you’ll accrue, making future updates and changes more difficult.
Best Practices for Managing Dependencies in Java
Effective dependency management doesn't happen by chance; it requires attention and systematic practices. Below are several best practices that you can adopt to break the cycle of dependency chaos:
1. Use Dependency Management Tools
Utilize tools such as Maven or Gradle to manage your dependencies. These tools enable you to declare libraries in a centralized manner, addressing dependency resolution automatically.
Example Using Gradle
In Gradle, you can manage dependencies in your build.gradle
file like this:
dependencies {
implementation 'org.springframework:spring-web:5.2.9.RELEASE'
}
Here, Spring is an essential library for Java development, and specifying it in the build.gradle
file makes it straightforward for all developers to work with the same version.
2. Keep Dependencies Updated
Regularly update your dependencies to benefit from the latest features, performance improvements, and security patches. Tools like Dependabot can automatically open pull requests for outdated dependencies.
3. Use Version Ranges Where Appropriate
Instead of pinning your dependencies to a specific version, you may want to specify a version range. This approach allows for flexibility during updates.
Example
In Maven, you can specify a version range like so:
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>[1.8.0, 1.14.0]</version>
</dependency>
This will use any version between 1.8.0 and 1.14.0.
4. Avoid Unused Dependencies
Periodically audit your project to remove unused dependencies. Tools such as Apache Maven Dependency Plugin can help identify these.
5. Use Dependency Scopes
Most dependency management tools allow you to define scopes. This restricts how the dependency is used, helping to avoid unwanted transitive dependencies.
Example Using Maven
In Maven, you might define scopes as follows:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
<scope>test</scope>
</dependency>
In this case, the Guava library will only be available during testing, which helps minimize runtime overhead.
6. Consider Binary Size
Always be aware of the impact your dependencies have on the application size. A bloated binary can affect deployment and performance.
7. Document Dependencies
Maintain documentation detailing why each dependency is included in your project, especially for opaque libraries. This context can prove invaluable for future developers spending time in the codebase.
Wrapping Up
Dependency management is not merely a technical necessity; it is the foundation for building scalable, manageable, and secure applications. Implementing effective practices can help teams avoid the pitfalls of dependency chaos, improve collaboration, and increase overall productivity.
As you delve into your next Java project, remember the importance of making informed decisions regarding dependencies. With proper management, you can break the cycle of dependency issues and set the stage for a successful software development experience.
By staying proactive in managing your dependencies, you’ll ensure that your Java applications remain resilient and robust, thus propelling your project to long-term success.
For further reading on dependency management and best practices in Java, consider checking out the following resources:
- Maven Documentation
- Gradle User Manual
- Effective Java by Joshua Bloch
By embracing these practices, you’ll not only improve your current projects but also elevate your skills as a developer.