Troubleshooting Common Server-Side Issues in Spring MVC AJAX
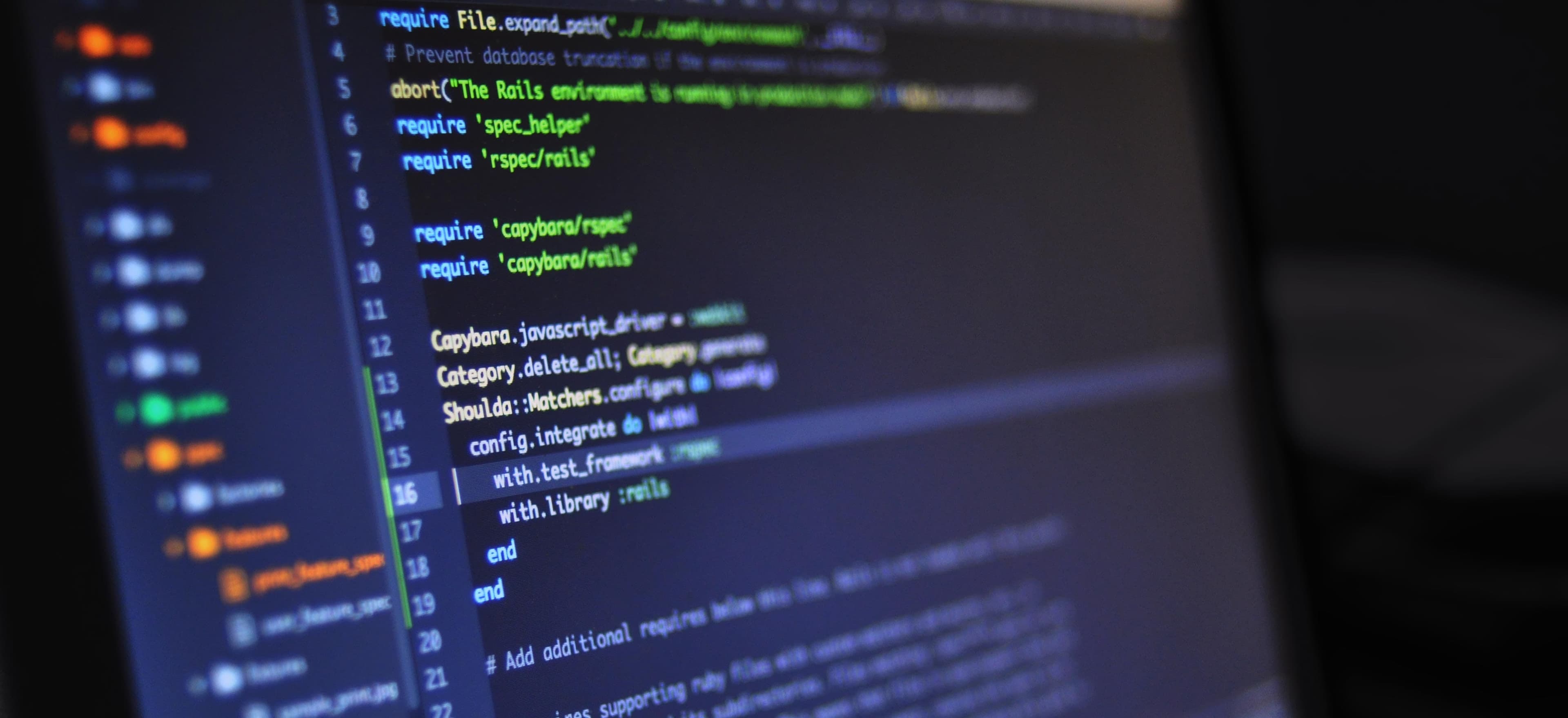
- Published on
Troubleshooting Common Server-Side Issues in Spring MVC AJAX
As web applications integrate more dynamic and interactive features, developers frequently turn to AJAX (Asynchronous JavaScript and XML) combined with Spring MVC to enhance user experience. However, complexities can arise when troubleshooting issues that root from the server-side logic. This post will outline common server-side issues encountered when using Spring MVC with AJAX and provide strategies for effectively resolving them.
Understanding AJAX and Spring MVC
Before diving into troubleshooting, it's essential to have a clear understanding of what AJAX is and how it interacts with Spring MVC.
What is AJAX?
AJAX allows web applications to send and receive data asynchronously without refreshing the entire web page. This means a more responsive user experience, as only portions of the web page get updated.
What is Spring MVC?
Spring MVC is a popular web framework in the Spring ecosystem. It is designed to provide a simple and flexible approach to building web applications. Spring MVC uses a dispatcher servlet to route requests and responses to appropriate controller methods, making it a powerful tool for handling AJAX calls.
Common Server-Side Issues
1. Incorrect Request Mappings
One common issue occurs when the URI mappings in the Spring controller do not perfectly align with the AJAX call.
Example Code Snippet
@Controller
public class MyController {
@RequestMapping(value = "/api/data", method = RequestMethod.GET)
@ResponseBody
public ResponseEntity<MyData> getData() {
MyData data = new MyData();
// Populate your data
return new ResponseEntity<>(data, HttpStatus.OK);
}
}
In this example, if the AJAX call is made to a different URL, for instance, /api/datas
, the request will not reach the intended controller method.
Solution
Ensure that the URL in the AJAX call matches the mapping in the Spring controller. Check for typos and HTTP method mismatches.
2. Serialization Issues
AJAX calls often exchange data in JSON format, which requires correct serialization of Java objects. Spring uses Jackson by default for JSON serialization. When objects are not serialized correctly, AJAX will receive an improper response.
Example Code Snippet
public class MyData {
private String name;
private int age;
// Getters and setters omitted for brevity
}
If there are any private fields without getters or if they have improper naming conventions, Jackson will not serialize them correctly.
Solution
Use proper JavaBean naming conventions containing public getters and setters. If you need advanced control over serialization, consider using annotations such as @JsonProperty
.
3. HTTP Status Codes
Using incorrect HTTP status codes can mislead the AJAX handling on the client-side. For example, returning an error code (like 404 or 500) can cause AJAX to trigger error handling unnecessarily.
Example Code Snippet
@ExceptionHandler(MyCustomException.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public void handleCustomException(MyCustomException e) {
// Log and handle the exception, response will carry status 500
}
Solution
Make sure to use the appropriate status codes. For successful operations, leverage 200 OK, while distinguishing various error types using 400, 401, 404, and 500 statuses accordingly. Proper usage helps AJAX methods to respond correctly.
4. CORS (Cross-Origin Resource Sharing) Issues
In many cases, AJAX calls can be affected by CORS policies, especially when the frontend and backend are hosted on different domains.
Example Code Snippet
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("http://localhost:8080")
.allowedMethods("GET", "POST");
}
}
Solution
Ensure that your Spring application is configured for CORS (Cross-Origin Resource Sharing), allowing specific origins, headers, and methods.
5. Data Validation Errors
AJAX sends data that needs validation on the server-side. If validation failure happens, it should be appropriately communicated back to the client.
Example Code Snippet
@PostMapping("/api/user")
@ResponseBody
public ResponseEntity<?> createUser(@RequestBody @Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return new ResponseEntity<>(bindingResult.getAllErrors(), HttpStatus.BAD_REQUEST);
}
// Save user logic here
return new ResponseEntity<>(HttpStatus.CREATED);
}
In the above example, if the validation fails for the User
object, a readable error response will be provided back to the AJAX call.
Solution
Implement validation and handle it correctly. Utilize Spring's @Valid
annotation along with BindingResult
to manage validation errors effectively.
6. JSON Parsing Errors
If the response sent from the server is not valid JSON, AJAX will not be able to parse it.
Example Code Snippet
Make sure that the response being sent is properly formatted:
@GetMapping("/api/greeting")
@ResponseBody
public String greeting() {
return "{\"message\": \"Hello World\"}"; // Valid JSON format
}
Solution
Always ensure that the data being returned from the server is in valid JSON format. Use tools such as JSONLint for validation as needed.
Best Practices for AJAX in Spring MVC
-
Use Descriptive Error Messages: When something goes wrong, provide meaningful messages that can assist in debugging efforts.
-
Test AJAX Requests: Tools such as Postman or cURL can be beneficial for testing your endpoints before connecting them to your frontend.
-
Maintain Versioning: As your API evolves, consider versioning your endpoints. This minimizes breakage for existing clients.
-
Log Request and Response: Logging can significantly aid in debugging, especially when dealing with asynchronous communications.
-
Consistency Across Controllers: Maintain a consistent approach for handling requests across your controllers to minimize confusion.
Resources for Further Learning
- Spring MVC Documentation
- Understanding JSON in Java
- AJAX with Spring MVC
A Final Look
Troubleshooting server-side issues in Spring MVC AJAX requires a keen understanding of both the server logic and the client-side expectations. By following best practices and implementing the solutions outlined above, you can significantly reduce the complexities associated with AJAX calls. Keep your endpoints clean and well-structured, validate inputs efficiently, and ensure your responses are correctly formatted. This way, you can deliver a seamless user experience while minimizing the time spent troubleshooting server-side issues.