Handling Null Values in JSON Serialization: Common Pitfalls
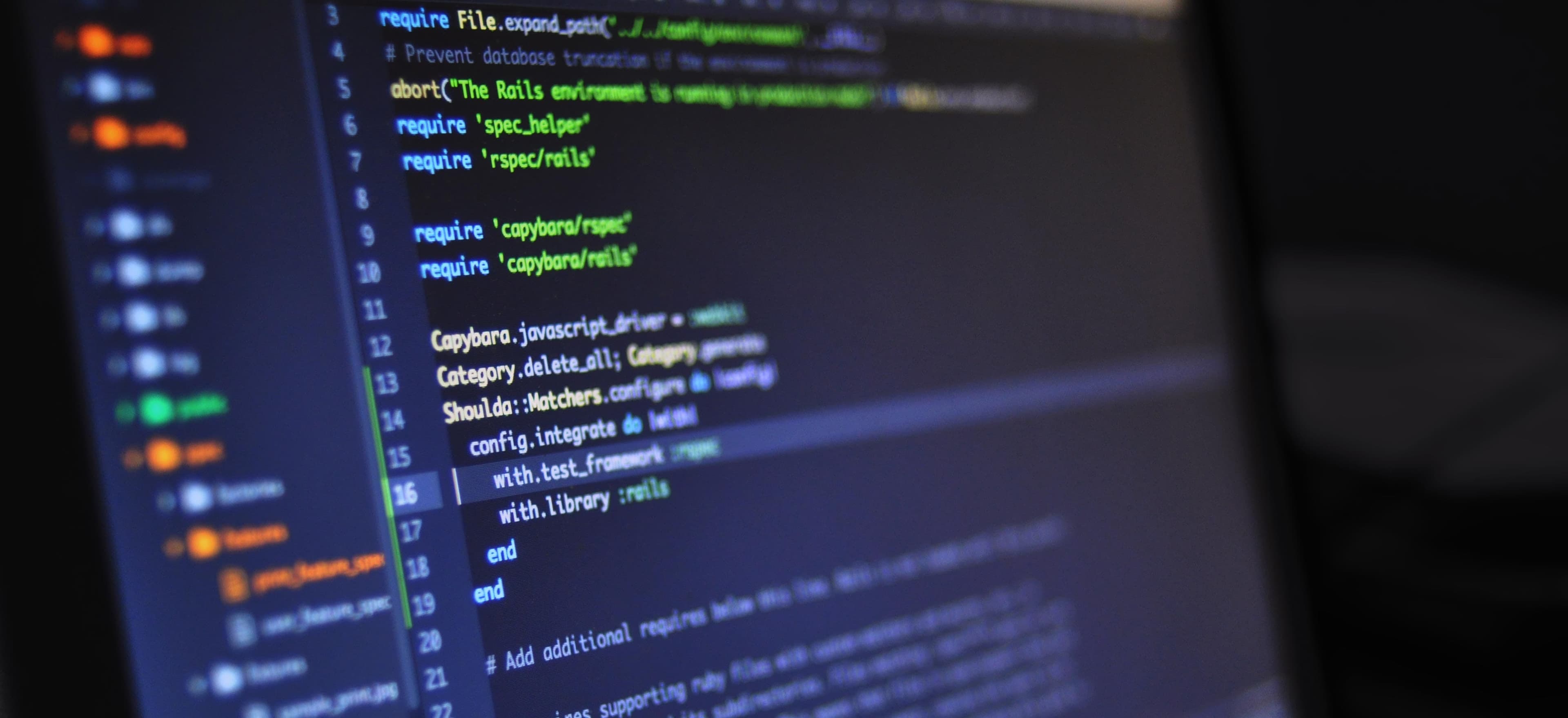
- Published on
Handling Null Values in JSON Serialization: Common Pitfalls
JSON (JavaScript Object Notation) has become a ubiquitous format for data interchange in modern applications. With its simplicity and ease of use, it allows developers to serialize and transfer complex data structures between systems seamlessly. However, in the realm of JSON serialization, handling null values can present a set of challenging pitfalls. In this post, we'll explore these common issues, decode the intricacies of JSON serialization in Java, and provide practical solutions to manage null values effectively.
What is JSON Serialization?
JSON serialization is the process of converting Java objects into a JSON format. This transformation is crucial for data transmission over networks, particularly in RESTful web services. In many scenarios, when an attribute does not hold a value or is not applicable, it may be defined as null. As a developer, you must manage such null values to avoid unexpected behaviors.
Why is Handling Null Values Important?
Handling null values in JSON serialization is essential for several reasons:
- Data Integrity: Null values can lead to data inconsistency and loss of valuable information.
- User Experience: When null values are not handled correctly, it can lead to confusing errors on the user interface.
- API Robustness: Properly managing nulls ensures that your API can handle unexpected values gracefully, which is critical for consumer applications.
Well-done serialization ensures a smooth interaction between frontend and backend systems, where your applications can effectively communicate without data mishaps.
Common Pitfalls in JSON Serialization
1. Null Values Included in the Output
One of the most common pitfalls is the inclusion of null fields in the serialized output. This can lead to unnecessarily verbose JSON. For example:
public class User {
private String name;
private String email;
private String phoneNumber;
// Getters and Setters
}
Assuming an instance is serialized but the phoneNumber
is null:
User user = new User();
user.setName("John Doe");
user.setEmail("john@example.com");
user.setPhoneNumber(null);
// Serialize to JSON
Gson gson = new Gson();
String json = gson.toJson(user);
System.out.println(json);
Output:
{"name":"John Doe","email":"john@example.com","phoneNumber":null}
In this scenario, including phoneNumber
is superfluous since it adds no value.
Solution: Use Configuration to Exclude Nulls
Most serialization libraries offer configuration options. For example, with Gson, you can instruct the library to skip null fields:
Gson gson = new GsonBuilder().serializeNulls().create();
To exclude null values:
Gson gson = new GsonBuilder().serializeNulls().create();
String jsonFiltered = gson.toJson(user);
System.out.println(jsonFiltered);
Black and white, right?
2. Unexpected Serialization Behavior with Collections
Another common pitfall is how collections containing null values are serialized. For instance, consider a list of user addresses where some entries might be null.
public class User {
private List<String> addresses;
// Getter and Setter
}
When you serialize a User
object with a list where some entries are null, the output may not reflect your intentions:
User user = new User();
user.setAddresses(Arrays.asList("123 Main St", null, "456 Elm St"));
// Serialize to JSON
Gson gson = new Gson();
String json = gson.toJson(user);
System.out.println(json);
Output:
{"addresses":["123 Main St",null,"456 Elm St"]}
Solution: Filter Out Nulls from Collections
To avoid such issues, you can filter out null values before serialization. This can be achieved using Java Streams:
List<String> filteredAddresses = user.getAddresses().stream()
.filter(Objects::nonNull)
.collect(Collectors.toList());
user.setAddresses(filteredAddresses);
By doing so, the serialized JSON will only contain meaningful addresses.
3. Misleading Structure Due to Nested Objects
When you have nested objects, handling null values can lead to misleading JSON output. For instance, consider a User
class that has another class, Profile
. If the profile is null, the output can create confusion.
public class User {
private String name;
private Profile profile;
}
public class Profile {
private String bio;
}
When serialized:
User user = new User();
user.setName("John Doe");
user.setProfile(null);
// Serialize User
String jsonUser = gson.toJson(user);
System.out.println(jsonUser);
Output:
{"name":"John Doe","profile":null}
Solution: Use Optional or Custom Logic for Nested Objects
Java’s Optional
can be used to manage nullable fields more effectively, ensuring your serialized output is more meaningful:
public class User {
private String name;
private Optional<Profile> profile;
// Getters and Setters
}
When the profile is absent, it can be represented more gracefully:
User user = new User();
user.setName("John Doe");
user.setProfile(Optional.empty());
// Serialize to JSON
String jsonUser = gson.toJson(user);
System.out.println(jsonUser);
Output:
{"name":"John Doe"}
4. Missing Fallbacks for Default Values
When not explicitly set, null values can lead to errors when deserializing JSON. Suppose you have a situation where default values are crucial for functionality, and null can cause method failures.
Solution: Use Default Values
You can implement a method to handle nulls and assign default values during serialization or deserialization:
public class User {
private String name;
private String email;
private String phoneNumber = "N/A"; // Default value
// Getters and Setters
}
This way, even if a value is left unassigned during object creation, serialization and subsequent usage will not break.
Best Practices for Handling Null Values
- Configure Serialization Libraries: Leverage library features to manage null values correctly.
- Use Optional Types: Use
Optional
for fields that may or may not be present. - Employ Default Values: Set defaults for attributes where applicable.
- Filter Collections: Remove nulls from collections before serialization.
- Document API Behavior: Clearly document how your API handles null values.
Further Reading
If you're interested in delving deeper into JSON serialization in Java, consider referring to the following resources:
- Gson Documentation - Learn more about Gson’s configuration and features.
- Jackson Library - Another popular library for JSON processing that handles null values in interesting ways.
- Java Optional - Understanding how to use the
Optional
class to manage nullability gracefully.
The Last Word
Handling null values in JSON serialization is a vital skill for any Java developer. The common pitfalls outlined in this post show how easily things can go awry without careful management. Implementing the suggested solutions not only helps you maintain data integrity but also enhances the overall robustness of your applications.
By placing emphasis on how nulls are treated, we can build cleaner, more maintainable code and ultimately provide a better experience for end-users and clients. Always remember, a little caution with nulls goes a long way!