Tackling OutOfMemoryError in Large Java Applications
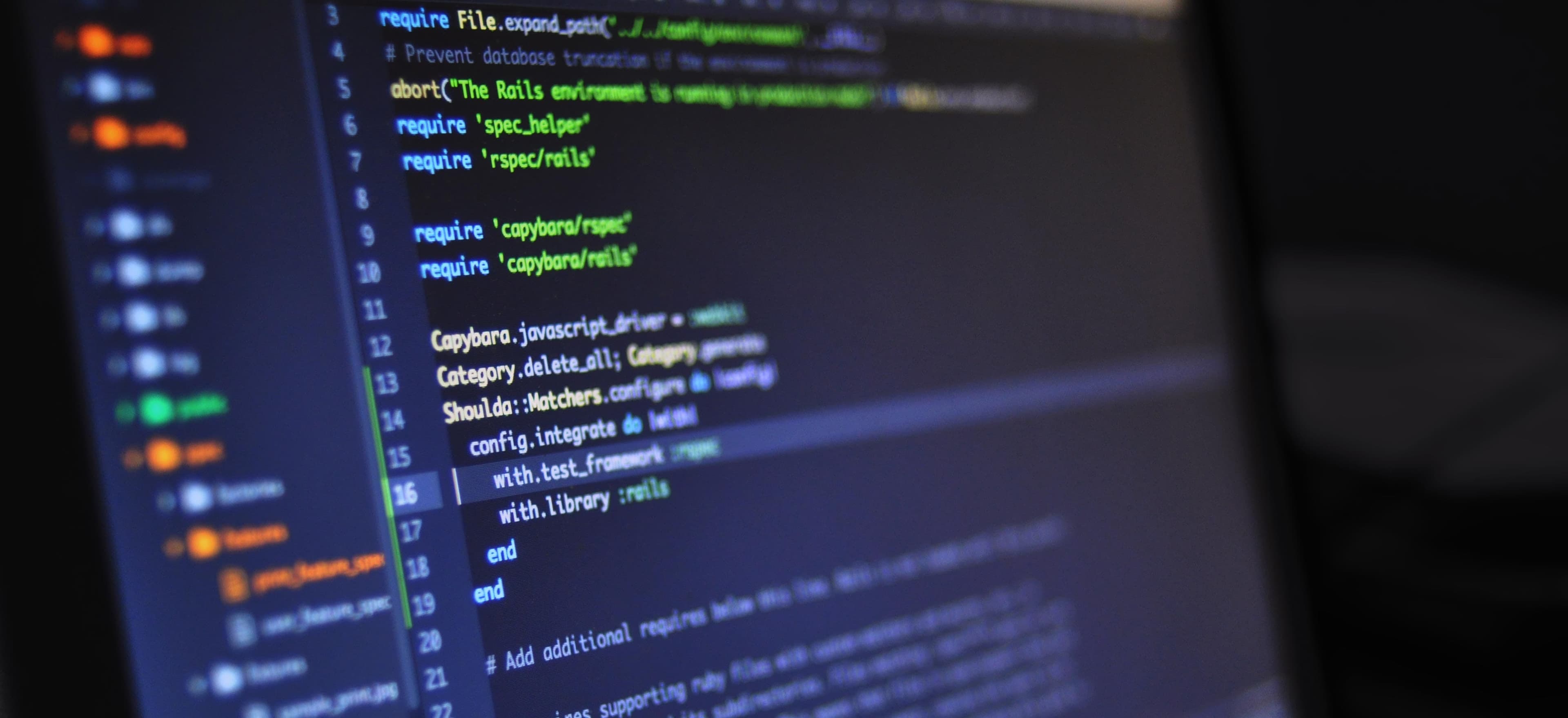
- Published on
Tackling OutOfMemoryError in Large Java Applications
Getting Started
Java is a widely used programming language known for its portability, performance, and extensive libraries. However, with its popularity comes challenges—especially in large applications where memory management becomes critical. One of the most notorious errors developers face is the OutOfMemoryError
. This error can cripple an application, leading to performance degradation or complete failure. In this blog post, we will explore the causes of OutOfMemoryError
, how to diagnose it, and best practices for preventing it.
What is OutOfMemoryError?
An OutOfMemoryError
occurs when Java's memory allocation fails, meaning the Java Virtual Machine (JVM) cannot allocate an object due to insufficient memory. This error can manifest in various forms, including:
- Java heap space
- PermGen space (prior to Java 8)
- Metaspace (Java 8 and onwards)
- Native memory
Understanding each type is essential for effective troubleshooting.
Java Heap Space
The Java heap is where JVM stores objects created by Java applications. The OutOfMemoryError
in this area typically happens when:
- Your application creates too many objects.
- Your objects are not properly released or garbage collected.
PermGen/Metaspace
Prior to Java 8, the PermGen area was fixed in size, which could lead to memory exhaustion when loading too many classes or resources. Starting with Java 8, PermGen was replaced with Metaspace, which dynamically grows but can still run out of memory if resources are improperly managed.
Native Memory
The JVM also makes use of native memory outside the heap, which may lead to exhaustion from excessive JNI calls, large allocations, or improper resource management.
Diagnosing OutOfMemoryError
Diagnosing an OutOfMemoryError
can be challenging, but the right tools and techniques enable developers to pinpoint the issue effectively.
1. JVM Memory Settings
Understanding JVM memory configurations is crucial. Here’s how you can check and set memory limits:
java -Xms512m -Xmx4g -jar your-application.jar
-Xms
sets the initial heap size (512MB in this case).-Xmx
sets the maximum heap size (4GB).
Setting the right memory limits can prevent OutOfMemoryError
when tuned based on the application's requirements.
2. Heap Dumps
A heap dump captures the memory of the JVM at a specific point in time. You can generate it using the following command:
java -XX:+HeapDumpOnOutOfMemoryError -XX:HeapDumpPath=/path/to/heapdump.hprof -jar your-application.jar
Use tools like Eclipse MAT (Memory Analyzer Tool) to analyze heap dumps and identify memory leaks or large object allocations.
3. Profiling Tools
Profiling tools like VisualVM or YourKit can provide insights into memory usage, object counts, and memory leaks. Using these tools will help you monitor your application in real-time and take action before an OutOfMemoryError
occurs.
Common Causes of OutOfMemoryError
1. Memory Leaks
Memory leaks occur when objects are unintentionally referenced, preventing them from being garbage collected. For instance:
public class MemoryLeakExample {
List<Object> list = new ArrayList<>();
public void createObject() {
while (true) {
list.add(new Object()); // Objects never released, leading to memory leak
}
}
}
To resolve this, ensure that you remove references to objects when they are no longer needed.
2. Large Collections
Storing excessive data in collections can quickly exhaust memory. For example:
List<String> largeList = new ArrayList<>();
for (int i = 0; i < Integer.MAX_VALUE; i++) {
largeList.add("Number: " + i);
}
Solution
Use efficient data structures and consider external storage if dealing with large datasets.
3. Inefficient Caching
Caching can significantly enhance performance, but improper management can lead to excessive memory usage.
Example
Using a cache without a size limit can fill up the heap space:
Map<String, Object> cache = new HashMap<>(); // No size limit
Solution
Consider implementing a caching framework like Ehcache or Guava with size limits. For instance:
Cache<String, Object> cache = CacheBuilder.newBuilder()
.maximumSize(1000) // Maximum cache size
.expireAfterAccess(10, TimeUnit.MINUTES) // Auto-expiry
.build();
Best Practices for Managing Memory
1. Optimize Data Structures
Select the appropriate data structure based on your use case. For instance, use ArrayList
for fast iterations instead of LinkedList
if frequent additions and deletions are not required.
2. Conduct Code Reviews
Regular code reviews can help identify possible memory leaks early in the development process.
3. Use Weak References
Weak references help in situations where you want objects to be garbage collected when memory is tight. For example:
WeakHashMap<String, Object> weakMap = new WeakHashMap<>();
4. Test Memory Limits
Perform load testing to understand how your application behaves under stress and determine the maximum load it can handle without crashing due to OutOfMemoryError
.
5. Regular Cleanup
Ensure that you manage resources effectively. For instance, close database connections and file streams promptly to free up native memory.
The Last Word
Tackling OutOfMemoryError
in large Java applications requires diligence, effective memory management strategies, and proactive measures. By diagnostics and prevention, you can ensure your application remains performant. Remember to continually monitor your application, and learn from memory exhaustion issues to fortify your codebase.
For an in-depth understanding of Java memory management concepts, you might want to check out the official Java documentation.
Further Reading
- Understanding Garbage Collection in Java
- Best Practices for Memory Management in Java
By implementing the techniques discussed in this blog post, you will be better equipped to manage memory in your large Java applications, ultimately leading to smoother performance and increased reliability.