Boost Your JavaFX XYCharts with Java 7 Features!
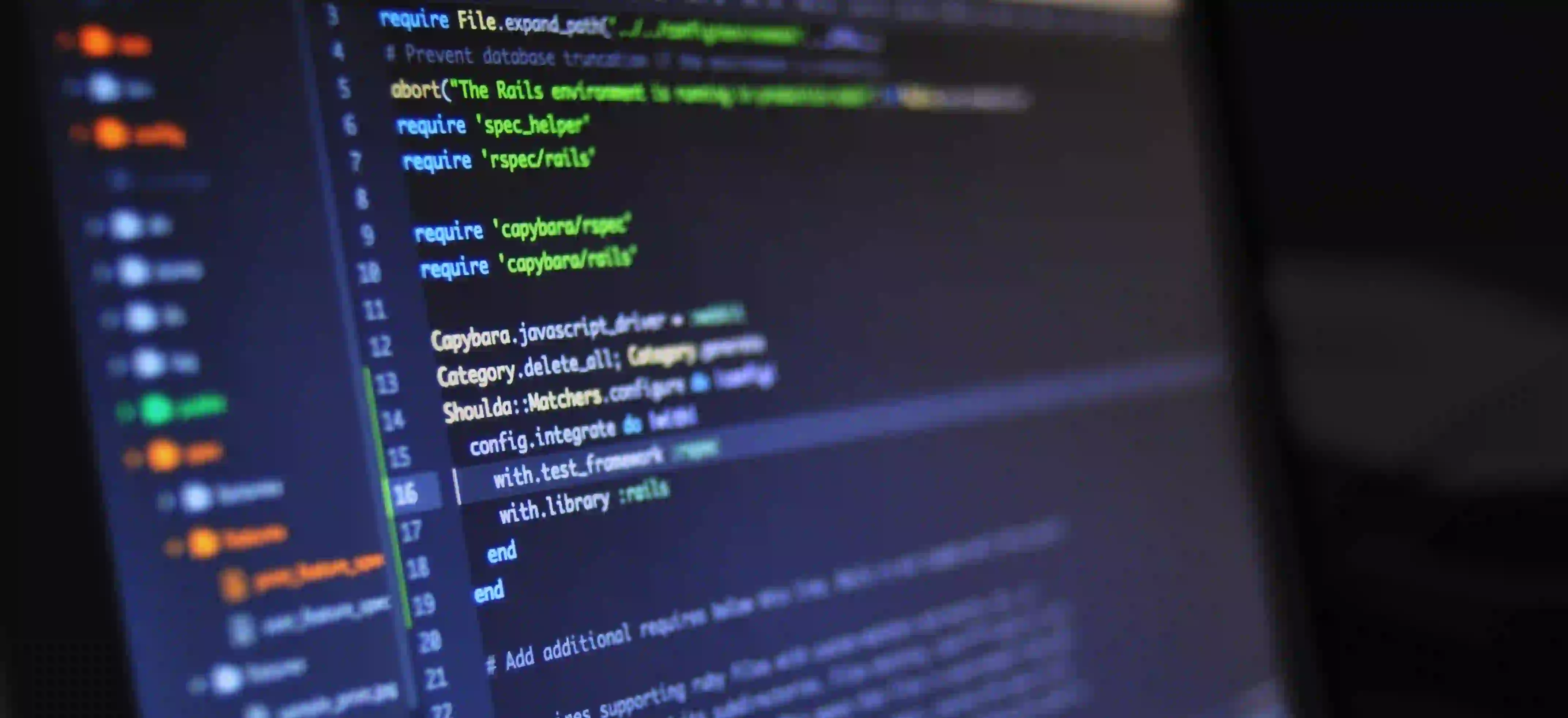
Boost Your JavaFX XYCharts with Java 7 Features
JavaFX provides a powerful framework for building rich client applications with a modern UI and advanced graphics capabilities. One of the noteworthy features in JavaFX is the XYChart, which allows you to create various types of charts and visualize data effectively. In this blog post, we’ll explore how to enhance your JavaFX XYCharts using features introduced in Java 7. We'll discuss key features such as the diamond operator, strings in switch statements, and try-with-resources, all of which can improve your JavaFX applications.
Diving Into the Subject to XYCharts in JavaFX
XYCharts in JavaFX are used for representing data in Cartesian coordinates. They include various chart types like LineChart
, ScatterChart
, and AreaChart
. These charts help convey complex data through simple visual means.
The fundamental parts of creating an XYChart in JavaFX are:
- Creating the Chart Instance
- Populating Data
- Displaying the Chart in a Scene
Here’s a quick example of how to create a basic LineChart
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.chart.LineChart;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.XYChart;
import javafx.stage.Stage;
public class SimpleXYChart extends Application {
@Override
public void start(Stage stage) {
stage.setTitle("Simple XY Chart Example");
// Define the x and y axes
NumberAxis xAxis = new NumberAxis();
NumberAxis yAxis = new NumberAxis();
xAxis.setLabel("X Axis");
yAxis.setLabel("Y Axis");
// Create the LineChart object
LineChart<Number, Number> lineChart = new LineChart<>(xAxis, yAxis);
// Adding data
XYChart.Series<Number, Number> series = new XYChart.Series<>();
series.setName("Sample Data");
series.getData().add(new XYChart.Data<>(1, 1));
series.getData().add(new XYChart.Data<>(2, 4));
series.getData().add(new XYChart.Data<>(3, 9));
lineChart.getData().add(series);
// Create the scene and add the chart to it
Scene scene = new Scene(lineChart, 800, 600);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why the Basic Structure Matters
Understanding the basic structure of an XYChart in JavaFX allows you to build upon it. Here, we utilize the NumberAxis
for both axes, but you can also use CategoryAxis
for categorical data. The XYChart.Series
represents a series of data points that will be plotted against your X and Y axes.
Leveraging Java 7 Features to Enhance Your Code
Java 7 introduced some new features that can help streamline and enhance your code in JavaFX applications. Below, we’ll discuss three key features that can be particularly beneficial for your XYCharts.
1. The Diamond Operator (<>
)
The diamond operator allows for type inference when creating instances of generic classes, reducing boilerplate code and increasing readability. This is particularly useful when working with generics in JavaFX.
Using the Diamond Operator
XYChart.Series<Number, Number> series = new XYChart.Series<>();
In this case, instead of repeatedly specifying the type parameters, we let the compiler infer them using the diamond operator. This improves both the readability and maintainability of your code.
2. Try-With-Resources
The try-with-resources statement makes it easier to manage resources, such as file streams and database connections. In the context of JavaFX, if you're loading data from files or databases, this feature can be particularly useful.
Example of Try-With-Resources
Suppose we want to read data from a CSV file to populate our XYChart:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class CSVChartReader {
public void readCSVData(String filePath, XYChart.Series<Number, Number> series) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
String[] values = line.split(",");
int xValue = Integer.parseInt(values[0]);
int yValue = Integer.parseInt(values[1]);
series.getData().add(new XYChart.Data<>(xValue, yValue));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Try-With-Resources?
This approach automatically closes your resources when done, minimizing the risk of resource leaks. Efficient resource management is crucial for any application and particularly beneficial while dealing with datasets that can grow large or when running in environments where resources are limited.
3. Strings in Switch Statement
Switching on strings can enhance your code's readability and organization. For applications that require user input to select data categories for plotting charts, using strings in switch statements can simplify decision-making processes.
Example Using String in Switch
public void updateChartBasedOnSelection(String selection, XYChart<Number, Number> chart) {
switch (selection) {
case "Option 1":
// Add data for Option 1
break;
case "Option 2":
// Add data for Option 2
break;
default:
// Handle default case
break;
}
}
Why Use Switch Statements for Strings?
By utilizing switch statements, you can manage various cases more neatly and efficiently. This creates a clearer structure for your code and simplifies future modifications—turning a potential mess of if-else statements into a concise switch statement.
Complete Example: Combining All Features
Now that we've discussed the features, let's look at an example that combines them for a fuller XYChart application.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.chart.LineChart;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.XYChart;
import javafx.stage.Stage;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class AdvancedXYChart extends Application {
@Override
public void start(Stage stage) {
stage.setTitle("Advanced XY Chart Example");
NumberAxis xAxis = new NumberAxis();
NumberAxis yAxis = new NumberAxis();
xAxis.setLabel("X Axis");
yAxis.setLabel("Y Axis");
LineChart<Number, Number> lineChart = new LineChart<>(xAxis, yAxis);
XYChart.Series<Number, Number> series = new XYChart.Series<>();
series.setName("Loaded Data");
// Use try-with-resources to read data
readCSVData("data.csv", series);
lineChart.getData().add(series);
Scene scene = new Scene(lineChart, 800, 600);
stage.setScene(scene);
stage.show();
}
private void readCSVData(String filePath, XYChart.Series<Number, Number> series) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
String[] values = line.split(",");
int xValue = Integer.parseInt(values[0]);
int yValue = Integer.parseInt(values[1]);
series.getData().add(new XYChart.Data<>(xValue, yValue));
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
Why This Example is Powerful
The advanced example combines several Java 7 features resulting in cleaner, more efficient code. Moreover, with support for external data file loading, it demonstrates a near-complete application ready for further enhancements, such as user interaction or dynamic data updating.
Closing the Chapter
JavaFX XYCharts are an excellent way to visualize data effectively, and with the adoption of Java 7 features, the process can be made even more seamless. From using the diamond operator and string switch statements to employing try-with-resources for resource management, each feature serves to simplify and enhance your JavaFX application.
Additional Resources
Remember that understanding the tools and features at your disposal is key to writing better, more maintainable code. So go ahead—experiment with these features and unlock the full potential of your JavaFX applications!