Handling Concurrency Issues with AtomicInteger in Quarkus Qute
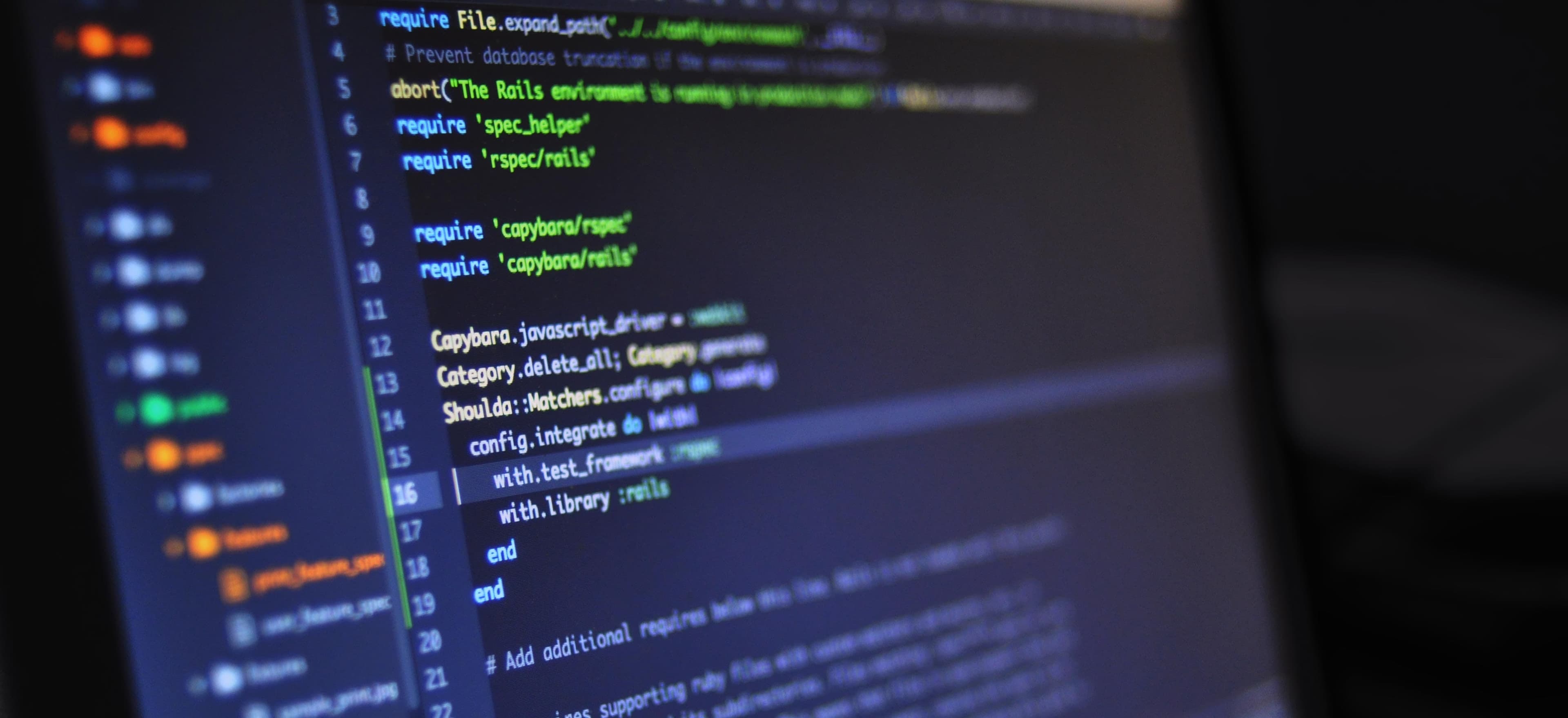
- Published on
Handling Concurrency Issues with AtomicInteger in Quarkus Qute
Concurrency is a critical aspect of modern software development, particularly as applications increasingly rely on multi-threading to improve performance and responsiveness. In Java, managing concurrent access to shared resources is a common challenge. One effective solution for thread safety is using AtomicInteger
. This blog post delves into how to handle concurrency issues with AtomicInteger
within the Quarkus framework, specifically when working with Qute, Quarkus's templating engine.
What is AtomicInteger?
AtomicInteger
is a part of the java.util.concurrent.atomic
package. It provides a simple, thread-safe way to work with integers in a concurrent environment. It is designed for scenarios where multiple threads are simultaneous updating the same variable, mitigating the risks of race conditions without needing to explicitly synchronize methods or blocks of code.
Key Features of AtomicInteger
- Thread Safety: Operations on
AtomicInteger
are atomic. - Lock-Free: It employs low-level atomic operations, avoiding locking mechanisms.
- Performance: Typically faster than synchronized blocks for read-heavy scenarios.
Setting Up Quarkus and Qute
To get started, you need a Quarkus project. If you don’t already have Quarkus set up, refer to the official Quarkus documentation for guidance on creating your first project.
Once you have a Quarkus application, integrate Qute by adding the appropriate dependency to your pom.xml
:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-qute</artifactId>
</dependency>
To maintain cleanliness and ensure efficient rendering in concurrent scenarios, you can manage state with AtomicInteger
.
Example Scenario: Counting Page Visits
For this example, let’s create a simple web application that counts and displays the number of visits to a page.
Step 1: Create an AtomicInteger
First, we’ll create a resource class that uses AtomicInteger
to track the number of visits.
import javax.enterprise.context.ApplicationScoped;
import java.util.concurrent.atomic.AtomicInteger;
@ApplicationScoped
public class VisitCounter {
private final AtomicInteger visitCount = new AtomicInteger(0);
// Increment the visit count
public int incrementAndGet() {
return visitCount.incrementAndGet();
}
}
Why AtomicInteger?
By using AtomicInteger
, we can ensure that even if multiple threads access the incrementAndGet
method simultaneously, the increment operation will remain atomic, preventing race conditions.
Step 2: Create a Resource Endpoint
Next, we’ll implement a REST endpoint to access and display this visit count.
import javax.inject.Inject;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import io.quarkus.qute.Qute;
@Path("/visit")
public class VisitResource {
@Inject
VisitCounter visitCounter;
@GET
@Produces(MediaType.TEXT_HTML)
public String countVisit() {
int count = visitCounter.incrementAndGet();
return Qute.getTemplate("visit.html")
.data("visitCount", count)
.render();
}
}
Key Concept: Dependency Injection
By using CDI (Contexts and Dependency Injection), we inject the VisitCounter
into our resource. This ensures a single instance of VisitCounter
is used across requests.
Step 3: Create the Qute Template
Now, create a Qute template file named visit.html
in src/main/resources/templates
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Visit Counter</title>
</head>
<body>
<h1>Welcome to the Visit Counter</h1>
<p>The page has been visited <strong>{visitCount}</strong> times.</p>
</body>
</html>
Rendering Dynamic Data
The template uses the visitCount
variable passed from the resource to render the current count of visits.
Testing Concurrent Access
To test how well the visit counter works under concurrency, you can simulate multiple users by sending multiple requests to the /visit
endpoint in quick succession. Use tools like Apache JMeter or Gatling to see how the counter increments and remains accurate.
Step 4: Combine with a Thread Pool
You might want to take it a step further by creating a test that simulates concurrent access more systematically. For this, you can utilize a thread pool that makes several requests to the endpoint concurrently.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ConcurrencyTest {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.submit(() -> {
// Simulate visiting the counter
// Use an HTTP client to call the /visit endpoint
// For demo, it could be a simple print statement
// System.out.println(visitCounter.incrementAndGet());
});
}
executor.shutdown();
}
}
Code Explanation
In this sample, we create a fixed thread pool of 10 threads and submit 100 tasks. This simulates 100 users visiting the page at once. Each thread will access the incrementAndGet
method of AtomicInteger
, demonstrating its thread safety.
The Bottom Line
This article discussed the importance of handling concurrency issues in Java applications, especially when using frameworks like Quarkus and Qute. With AtomicInteger
, we can efficiently and safely manage access to numeric state without the pitfalls associated with traditional synchronization mechanisms.
For further reading on Java concurrency and the Atomic classes, take a look at the Java Concurrency Tutorial.
With the combination of Atomic types and Quarkus, developers can build reactive, concurrent applications that are efficient and scalable. Feel free to share your thoughts, questions, or experiences with concurrency in Java, and happy coding!