Common Mistakes When Enabling Web MVC in Spring Boot
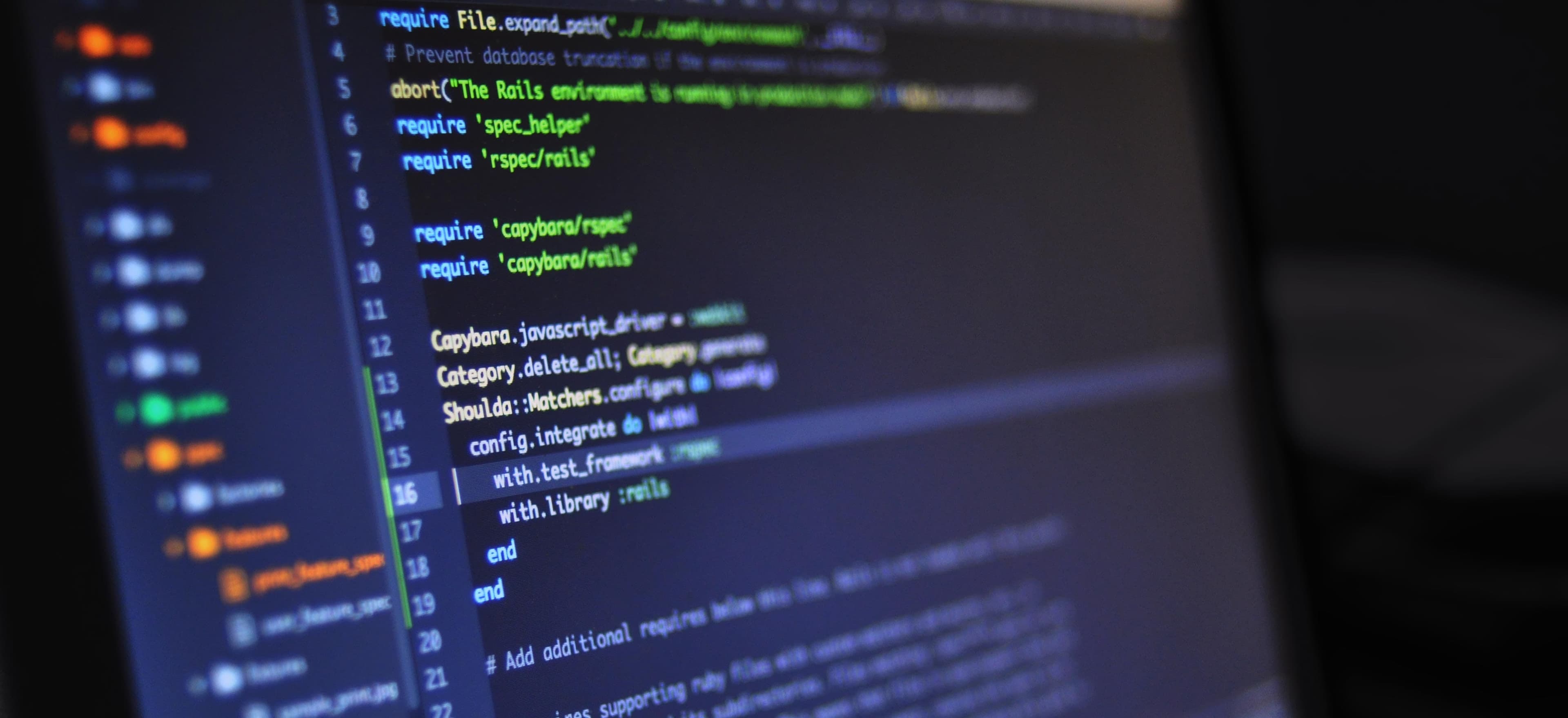
- Published on
Common Mistakes When Enabling Web MVC in Spring Boot
Spring Boot, a project under the broader Spring Framework, simplifies the process of setting up and deploying production-grade applications, particularly web applications. Enabling Web MVC in Spring Boot is a powerful feature, allowing developers to create RESTful applications with ease. However, many developers—both newcomers and experienced—commit common mistakes during this process. In this article, we will explore these pitfalls, provide insights into avoiding them, and illustrate best practices using practical code snippets.
Table of Contents
- Understanding Spring Boot
- Setting Up Web MVC
- Common Mistakes
- Annotations Misconfiguration
- Dependency Issues
- Missing Configuration Files
- Ignoring Exception Handling
- Improper URL Mapping
- Best Practices
- Conclusion
Understanding Spring Boot
Spring Boot is designed to take an opinionated view of building Spring applications. This means that developers can get started without needing to configure every aspect manually. Enabling Web MVC, central to building web applications, involves setting up controllers, views, and REST endpoints.
Key Features of Spring Boot Web MVC
- Convention over Configuration: It reduces the need for boilerplate code.
- Embedded Servers: Such as Tomcat, Jetty, and Undertow, which allow easy testing.
- Microservice Friendly: Easy to deploy and manage microservices.
For a deeper understanding of Spring Boot, check the official documentation.
Setting Up Web MVC
To enable Web MVC in Spring Boot, you need to include the necessary dependencies in your pom.xml
or build.gradle
. For Maven, add the following dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
For Gradle, include:
implementation 'org.springframework.boot:spring-boot-starter-web'
This starter enables essential components like Spring MVC, embedded servers, and JSON handling capabilities.
Common Mistakes
While setting up Spring Boot Web MVC, developers make several common mistakes. Let's delve into these pitfalls.
Annotations Misconfiguration
The most frequent mistake is improperly using annotations. For instance, forgetting @RestController
or using @Controller
without ensuring a view resolver can lead to confusion.
Example:
@Controller
public class HomeController {
@GetMapping("/")
public String home(Model model) {
model.addAttribute("message", "Welcome!");
return "home"; // This assumes a view resolver is set up
}
}
For REST APIs, you should use @RestController
:
@RestController
public class ApiController {
@GetMapping("/api/data")
public ResponseEntity<String> getData() {
return ResponseEntity.ok("Sample Data");
}
}
Why this Matters?
Using @RestController
prevents the need for a view resolver and serializes responses directly to JSON, which is often necessary in modern API development.
Dependency Issues
Another common oversight is neglecting to include essential dependencies. Without the right starters, features such as JSON processing or MVC support may fail to initialize.
Example:
Missing Jackson for JSON processing could lead to:
@Bean
public MappingJackson2HttpMessageConverter mappingJackson2HttpMessageConverter() {
return new MappingJackson2HttpMessageConverter();
}
Why This Matters?
The absence of Jackson can lead to errors when returning JSON data, making your application non-operational at times.
Missing Configuration Files
Developers sometimes fail to create or misplace the application.properties
or application.yml
files, leading to configuration issues.
Example:
Configuring the server port in the application.properties
:
server.port=8081
Why This Matters?
Not having the correct configuration can result in your application being unreachable, especially in a cloud environment.
Ignoring Exception Handling
Failing to implement global exception handling with @ControllerAdvice
can lead to uninformative error messages, frustrating the end-user.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.getMessage());
}
}
Why This Matters?
Effective exception handling improves user experience and provides additional telemetry for debugging.
Improper URL Mapping
Another prevalent mistake relates to URL mappings. Misconfigured paths lead to 404 errors, deterring users from accessing your application.
Example:
@GetMapping("/path")
public String data() {
return "data";
}
// Accessing "/patah" will lead to 404
Why This Matters?
URL consistency is crucial for API usability and documentation. Tools like SpringFox can help document your endpoints better.
Best Practices
As you progress in mastering Spring Boot Web MVC, adhering to best practices will lend more stability and maintainability to your code.
- Consistency in Naming Conventions: Always stick to a naming pattern for your controllers and methods.
- Utilizing Annotations Appropriately: Understand and utilize relevant Spring annotations effectively.
- Global Exception Handling: Implement global exception handlers to centralize error management.
- Validation and Error Handling: Use JSR-303 annotations for validation and handle validation errors effectively.
- Embedding Tests: Write integration tests to validate your endpoints and ensure they work correctly.
A Final Look
Enabling Web MVC in Spring Boot is straightforward, but it's fraught with pitfalls. By being aware of common mistakes such as annotation misconfigurations, dependency issues, and improper URL mapping, you can enhance the robustness of your application.
As always, the Spring ecosystem is rich with documentation and community support. For further reading, consider delving into the official Spring Framework Documentation or exploring the myriad of online courses on platforms like Udemy or Coursera.
Implement these tips into your development process, and your Spring Boot applications will be better equipped to handle the demands of users and developers alike. Happy coding!