Mastering Implicit Classes: Common Pitfalls in Java
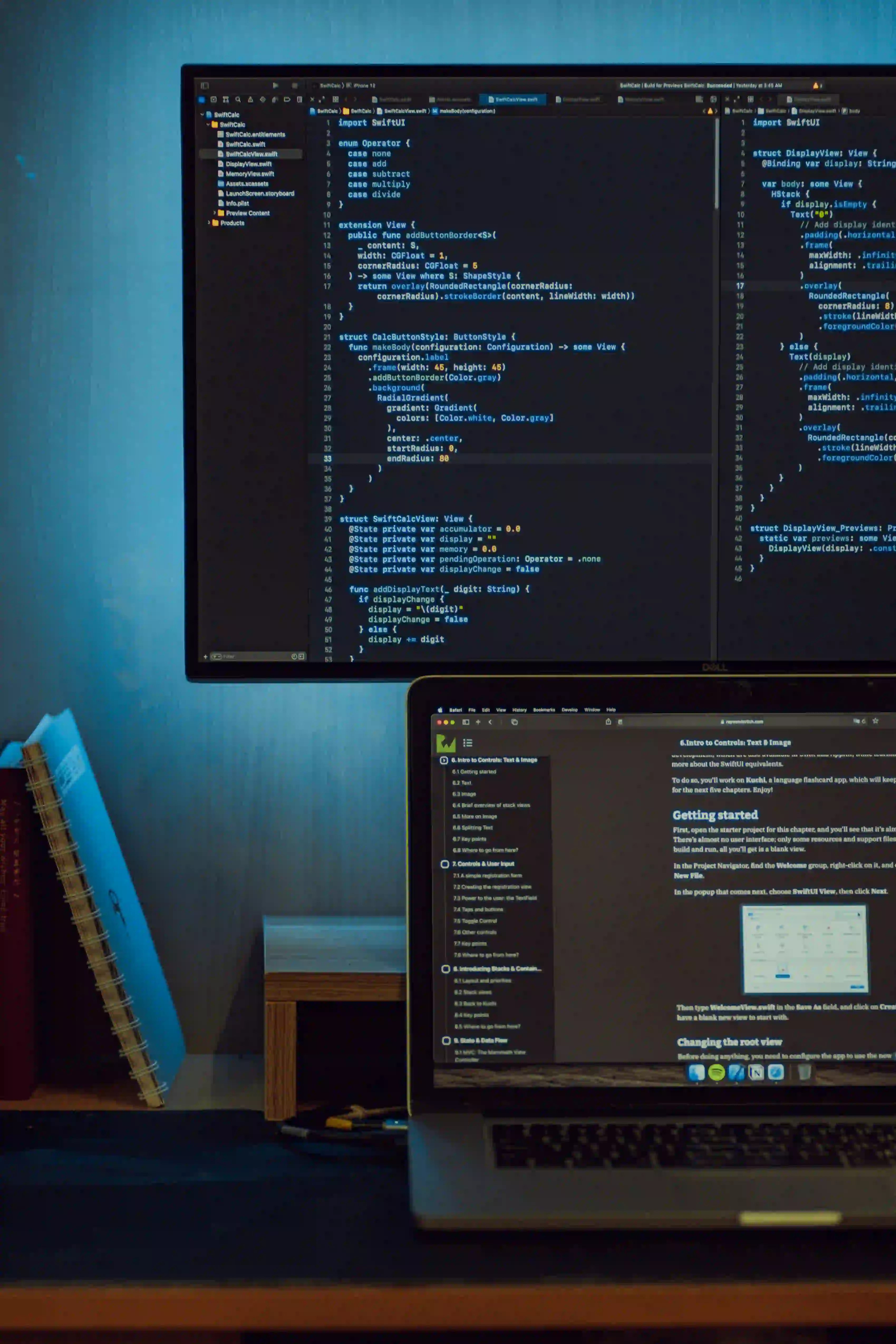
Mastering Implicit Classes: Common Pitfalls in Java
Java is renowned for its strong typing and object-oriented features. Within this sophisticated landscape lies a lesser-known yet powerful concept: implicit classes. Though not a native feature of Java in the same way it is in some other languages like Scala, understanding how to achieve similar functionality with Java's capabilities can significantly enhance your coding strategy. In this post, we will explore implicit classes in Java, highlight common pitfalls, and arm you with best practices to master their usage.
Understanding Implicit Classes
Before diving into common pitfalls, let's clarify what we mean by implicit classes. An implicit class, in its essence, enables developers to add new functionalities to existing classes without altering their definitions. This is possible using Java's ability to employ extension methods through static methods or helper classes.
To illustrate, consider the utility nature of implicit classes. For instance, adding functionalities to the String
class for enhanced string manipulations can be accomplished through:
public class StringUtils {
public static String toUpperCaseFirstLetter(String str) {
if (str == null || str.isEmpty()) {
return str;
}
return str.substring(0, 1).toUpperCase() + str.substring(1);
}
}
In the example above, toUpperCaseFirstLetter
is a static method that could serve as your ‘implicit’ addition to the String
class. However, the use of static methods instead of true implicit classes creates some challenges.
Common Pitfall #1: Lack of Inheritance
One major pitfall developers face when using static utility classes is that these methods do not support inheritance. Consequently, when you attempt to override such methods in subclasses, you will find it impossible.
For instance, consider the following scenario:
public class EnhancedStringUtils extends StringUtils {
public static String toUpperCaseFirstLetter(String str) {
return super.toUpperCaseFirstLetter(str).toLowerCase(); // Compilation error
}
}
Attempting to override toUpperCaseFirstLetter
will result in a compilation error because static methods are bound to the class itself and are not polymorphic.
To avoid this, consider utilizing interfaces or abstract classes:
public interface StringTransformer {
String transform(String str);
}
public class UpperCaseTransformer implements StringTransformer {
@Override
public String transform(String str) {
return str.toUpperCase();
}
}
Incorporating interfaces promotes flexibility and avoids the pitfalls associated with static methods.
Common Pitfall #2: Static Context Limitations
Another pitfall in working with implicit classes is the inability to access instance variables or methods. Since static methods belong to the class rather than instances, you cannot access non-static members of the class.
Here is an example:
public class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public static String greet() {
return "Hello, " + name; // Compilation error
}
}
The greet
method cannot access the name
variable because it is defined as a static method. To solve this issue, convert the static method into an instance method:
public class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public String greet() {
return "Hello, " + name; // Now it works
}
}
Common Pitfall #3: Overloading Ambiguity
Overloading methods can sometimes lead to confusion when static methods masquerade as implicit functionality. Consider the following situation with overloaded methods:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
public static double add(double a, double b) {
return a + b;
}
}
Invoking add(2, 3)
results in an integer addition, while add(2.0, 3.0)
results in double addition. Now imagine if we have classes that utilize MathUtils
heavily; determining which method is called can lead to hard-to-trace errors.
Best Practices for Implicit Class-like Behavior
-
Use Interfaces or Abstract Classes: To achieve extensibility, rely on interfaces or abstract classes instead of standalone utility classes. This allows you to create concrete implementations that can be inherited and overridden.
-
Favor Instance Methods: Where applicable, make use of instance methods instead of static methods, so that they can operate on the state of an object, supporting encapsulation and polymorphism.
-
Keep It Simple: A utility class can quickly become cumbersome. If you find yourself adding multiple methods, consider splitting them into separate, clearly defined classes.
-
Document Your Code: Especially when utility methods are heavily used, document the purpose and parameters of each method. Clear documentation can prevent future misuse and confusion.
Key Takeaways
Although Java does not natively support implicit classes like some other programming languages, you can still mimic this behavior through clever design and structure. By being aware of the common pitfalls associated with static utility methods and by applying best practices, you can harness the power of implicit classes to write cleaner and more efficient code.
Additional Resources:
- Java 8 Functional Interfaces and Lambda Expressions
- Effective Java by Joshua Bloch
- Java Documentation
Whether you are new to Java or an experienced developer, mastering the techniques surrounding implicit class-like functionality is a tremendous step toward writing efficient, clean code. Embrace the challenges and make implicit classes your ally. Happy coding!