Overcoming Common Pitfalls in Java EE 7 Deployment Pipelines
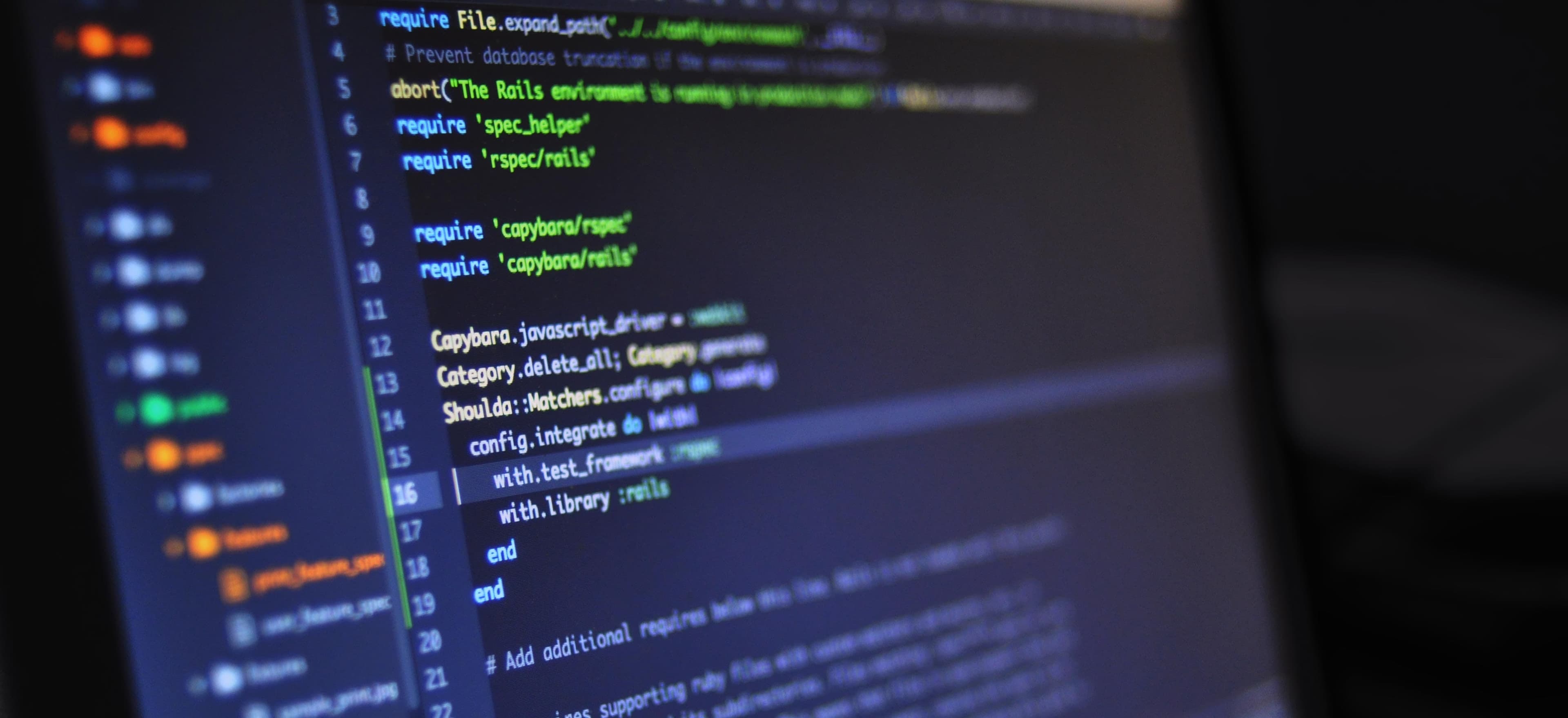
- Published on
Overcoming Common Pitfalls in Java EE 7 Deployment Pipelines
Java EE 7, now rebranded as Jakarta EE, is a powerful platform that allows developers to build enterprise-level applications with robust features. However, deploying applications on Java EE 7 can present numerous challenges that could derail even the most careful planning efforts. Today, we'll explore the common pitfalls encountered in Java EE 7 deployment pipelines and provide actionable strategies to overcome these challenges.
Importance of a Reliable Deployment Pipeline
Before diving into the pitfalls, it's important to understand why a reliable deployment pipeline is essential. A well-structured deployment pipeline ensures that your application is delivered efficiently, consistently, and without interruption. Furthermore, automating the deployment pipeline helps in maintaining the integrity of the application through various stages, from code commit to production deployment.
Common Pitfalls in Java EE 7 Deployment Pipelines
Below are several common pitfalls that developers often face when setting up deployment pipelines for Java EE 7 applications, along with recommended solutions to navigate through them.
1. Ignoring Dependency Management
Pitfall: Java EE 7 applications often rely on multiple libraries and frameworks, which can lead to dependency conflicts and version mismatches. Ignoring these dependencies can result in runtime errors that are difficult to trace.
Solution: Utilize a robust dependency management system like Maven or Gradle. Here’s how you can specify dependencies in a Maven pom.xml
file:
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
Why?: Specifying the scope as "provided" ensures that the Java EE implementation will supply the required libraries during runtime, preventing unnecessary bloat in your packaged application. For more on Maven, check Maven Documentation.
2. Failure to Automate Builds
Pitfall: Manual builds can quickly lead to inconsistency and errors. Developers may forget to run necessary tasks, leading to incomplete builds.
Solution: Use Continuous Integration (CI) tools like Jenkins to automate your build process. Here’s an example of a Jenkins pipeline configuration:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'mvn clean install'
}
}
}
}
}
Why?: Automating the build process guarantees that any changes made to the codebase are compiled and tested systematically. This reduces human error and ensures that any defects are caught early. For more insights, visit the Jenkins Documentation.
3. Inadequate Testing Strategies
Pitfall: Skipping unit tests or integration tests prior to deployment can lead to serious performance issues or bugs in production.
Solution: Implement a comprehensive testing strategy using JUnit or TestNG. For instance, you may use JUnit for unit testing as follows:
import static org.junit.Assert.*;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why?: Automated tests like this ensure that your methods behave as expected. Executing these tests during the pipeline will give you confidence that your application will function correctly in the production environment. For more information about JUnit, refer to JUnit 5 User Guide.
4. Neglecting Configuration Management
Pitfall: Hardcoding values within your application can lead to deployment inconsistencies across different environments (development, testing, production).
Solution: Utilize external configuration management tools like Spring Cloud Config or Apache ZooKeeper. Here’s an example of how you can externalize properties in Spring:
# application.properties
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
Why?: By externalizing your configuration, you can easily change parameters without modifying the codebase. It makes the application more flexible and easier to manage, as configuration can adapt to the environment it’s running in. For deeper insights, visit Spring Cloud Config.
5. Ignoring Logging and Monitoring
Pitfall: Overlooking logging and monitoring can make it difficult to diagnose issues as they arise in production.
Solution: Integrate logging frameworks like Log4j or SLF4J and leverage monitoring tools like Prometheus or Grafana. Here’s how to set up Log4j in your application:
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
And configure it like this:
# log4j.properties
log4j.rootLogger=DEBUG, stdout
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n
Why?: Implementing a robust logging and monitoring solution allows you to trace faults and performance issues promptly. This proactive approach empowers your team to mitigate risks before they escalate. For comprehensive guidance, check out Log4j Documentation.
6. Underestimating Deployment Time
Pitfall: Developers often underestimate the time needed for deployment, leading to rushed releases and last-minute issues.
Solution: Establish a proper timeline for your deployments, including buffer time for unexpected circumstances. This should be part of your DevOps culture. Here’s a simple outline you could follow:
1. Code Freeze
2. Testing
3. Review
4. Staging Deployment
5. Production Deployment
Why?: Having a clear timeline with dedicated periods for testing and reviews minimizes the chances of encountering last-minute problems. It can also enhance collaboration and accountability within the team.
In Conclusion, Here is What Matters
Navigating the complexities of deployment pipelines in Java EE 7 can be challenging, but by being aware of these common pitfalls and their respective solutions, you can streamline your development process. From ensuring proper dependency management to implementing a robust testing and monitoring strategy, these steps will enhance your deployment pipeline’s reliability.
While there’s no one-size-fits-all approach to deploying Java EE 7 applications, keeping these best practices in mind will significantly reduce your risks and enhance your workflow. For further reading, exploring the official Jakarta EE documentation will provide you with a broader understanding and additional resources.
By investing in a reliable deployment pipeline today, your team can focus more on innovation and less on troubleshooting, setting the stage for long-term productivity and success.
Happy coding!
Checkout our other articles