Mastering ViewPager: Avoiding Common Implementation Pitfalls
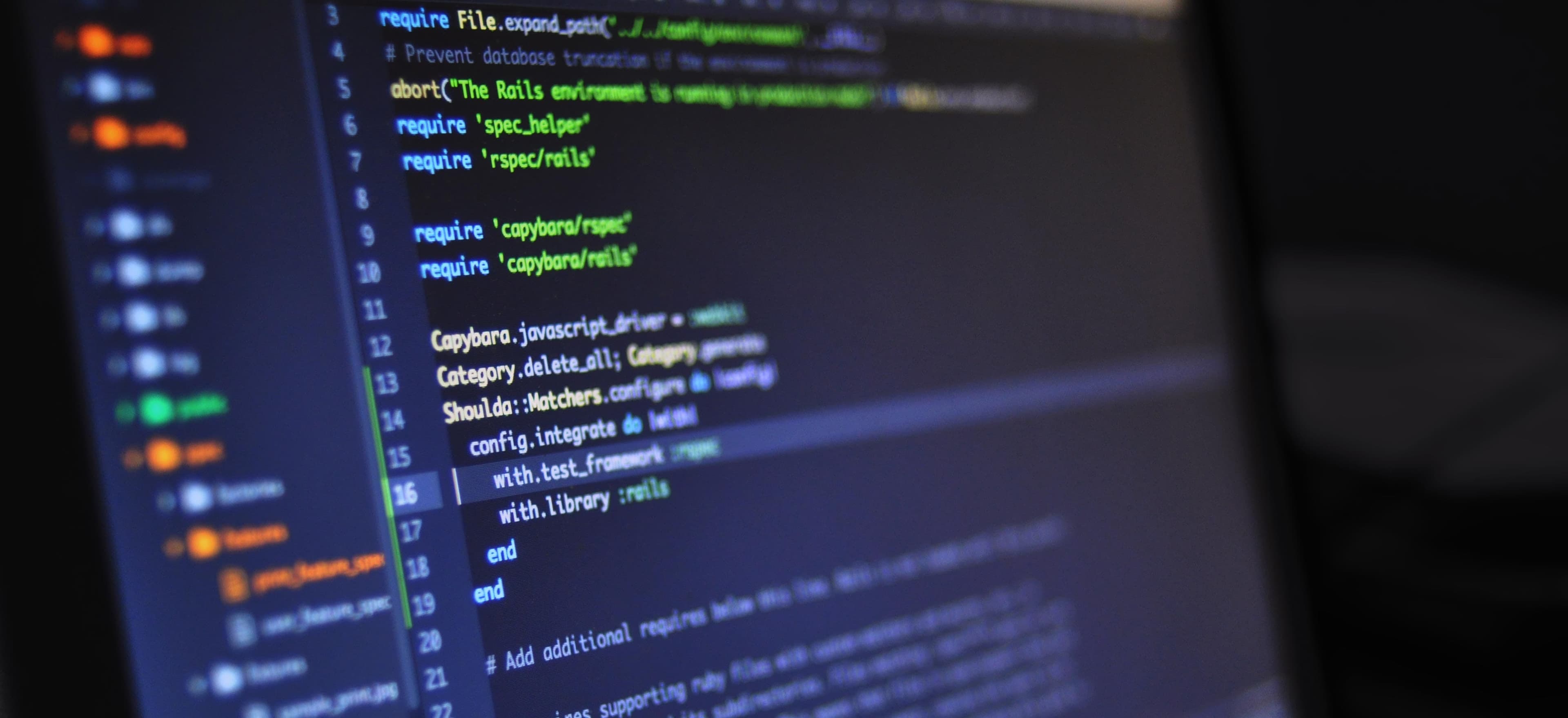
- Published on
Mastering ViewPager: Avoiding Common Implementation Pitfalls
The Android ViewPager is a powerful and versatile widget. It allows users to swipe between different screens or fragments seamlessly. However, improper implementation can lead to performance issues and usability problems. In this guide, we'll explore the common pitfalls of using ViewPager and discuss best practices to help you avoid them. Let’s dive in!
What is ViewPager?
ViewPager is a part of the Android Support Library, specifically designed to allow users to swipe left and right through pages of data. It interacts well with fragments, making it a popular choice for scenarios like swipeable tabs and image galleries.
Importance of Proper Implementation
An incorrectly implemented ViewPager can cause various issues:
- Poor performance due to memory leaks.
- Unexpected behavior when dealing with fragments.
- A non-intuitive user experience.
By understanding the common pitfalls and their solutions, developers can create an efficient and user-friendly experience.
1. Avoiding Fragment State Loss
When using a ViewPager, especially with fragments, developers frequently encounter an error called fragment state loss. This happens when a fragment transaction is attempted after the activity has been saved.
Example Code Snippet:
@Override
public void onBackPressed() {
// Avoid committing fragment transactions that can lead to state loss
if (getSupportFragmentManager().findFragmentById(R.id.fragment_container) != null) {
getSupportFragmentManager().beginTransaction()
.remove(getSupportFragmentManager().findFragmentById(R.id.fragment_container))
.commitAllowingStateLoss(); // Be cautious with this method
} else {
super.onBackPressed();
}
}
Commentary:
- Reasoning: By calling
commitAllowingStateLoss()
, you avoid risking a crash if the activity state has already been saved. However, be careful with this approach; it can lead to subtle bugs if the state is lost unexpectedly. - Tip: It’s safer to handle transactions before the state is saved and to leverage saved instance states effectively.
2. Managing Fragment Lifecycle Correctly
Misunderstanding the lifecycle of fragments within ViewPager can also lead to issues like poor performance and views not being updated correctly.
Key Lifecycle States:
- onCreate(): Called when the fragment is first created.
- onCreateView(): Inflate the layout and prepare the view to display.
- onStart(): The fragment becomes visible to the user.
- onResume(): The user is interacting with the fragment.
Example Code Snippet:
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_layout, container, false);
// Initialize views here to avoid unnecessary calls later
setupViews(view);
return view;
}
private void setupViews(View view) {
Button myButton = view.findViewById(R.id.my_button);
myButton.setOnClickListener(v -> {
// Handle button clicks properly
});
}
Commentary:
- Why This Matters: Properly handling the lifecycle prevents memory leaks and ensures that UI components are initialized correctly.
- Best Practice: Always defer view initialization to
onCreateView()
oronActivityCreated()
to ensure views are constructed and not null when needed.
3. Efficient Adapter Management
ViewPager uses an adapter to manage the creation of the pages. Using the wrong adapter type can lead to unnecessary object creation, which can hinder performance.
ViewPager Adapter Types:
- PagerAdapter: For static content.
- FragmentPagerAdapter: For fragments that remain in memory.
- FragmentStatePagerAdapter: For fragments that do not need to remain in memory.
Example Code Snippet:
public class MyFragmentPagerAdapter extends FragmentStatePagerAdapter {
private static final int NUM_PAGES = 5;
public MyFragmentPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
return MyFragment.newInstance(position);
}
@Override
public int getCount() {
return NUM_PAGES;
}
}
Commentary:
- Why Choose FragmentStatePagerAdapter: If your user interface is dynamic and fragment creations are costly, opt for
FragmentStatePagerAdapter
. This ensures that only the currently visible fragments are kept in memory. - Best Practice: Evaluate your fragment management needs carefully. If excessive fragment instantiation occurs, even with caching, consider using data-driven UI elements.
4. Avoiding Overdraw
Overdraw occurs when multiple layers of identical pixels are drawn on top of each other. This can significantly impact performance, especially with complex view hierarchies in ViewPager.
Code Snippet for Optimization:
<androidx.viewpager.widget.ViewPager
android:id="@+id/viewPager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/transparent" />
Commentary:
- Why It Matters: Setting the background to transparent minimizes overdraw, as the ViewPager will only paint background for the visible views.
- Performance Tip: Utilize the Android Profile GPU Rendering tool in the developer options on your device to monitor overdraw while you test.
5. Handling Orientation Changes
One of the common issues faced while working with ViewPager is proper handling of state during configuration changes, such as screen rotations.
Saving State in ViewPager:
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putInt("current_page", viewPager.getCurrentItem());
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
if (savedInstanceState != null) {
currentPage = savedInstanceState.getInt("current_page", 0);
viewPager.setCurrentItem(currentPage);
}
}
Commentary:
- Reasoning: Saving and restoring instance state maintains the user's position in the ViewPager after orientation changes, thereby improving the user experience.
- Best Practice: Follow the Android's best practice guidelines for handling configuration changes.
The Bottom Line
Effectively utilizing ViewPager can provide a fluid and pleasurable user experience. Avoiding the common pitfalls outlined in this guide enhances performance, maintains state, and adheres to best practices in Android development.
Additional Resources
- Android ViewPager Documentation
- Understanding Fragment Lifecycle
- Fragment Transactions Guide
By following these guidelines, developers can avoid common pitfalls and ensure that their implementation of ViewPager is both efficient and user-friendly. Happy coding!