Maximize UDP Efficiency: Common Aeron Messaging Pitfalls
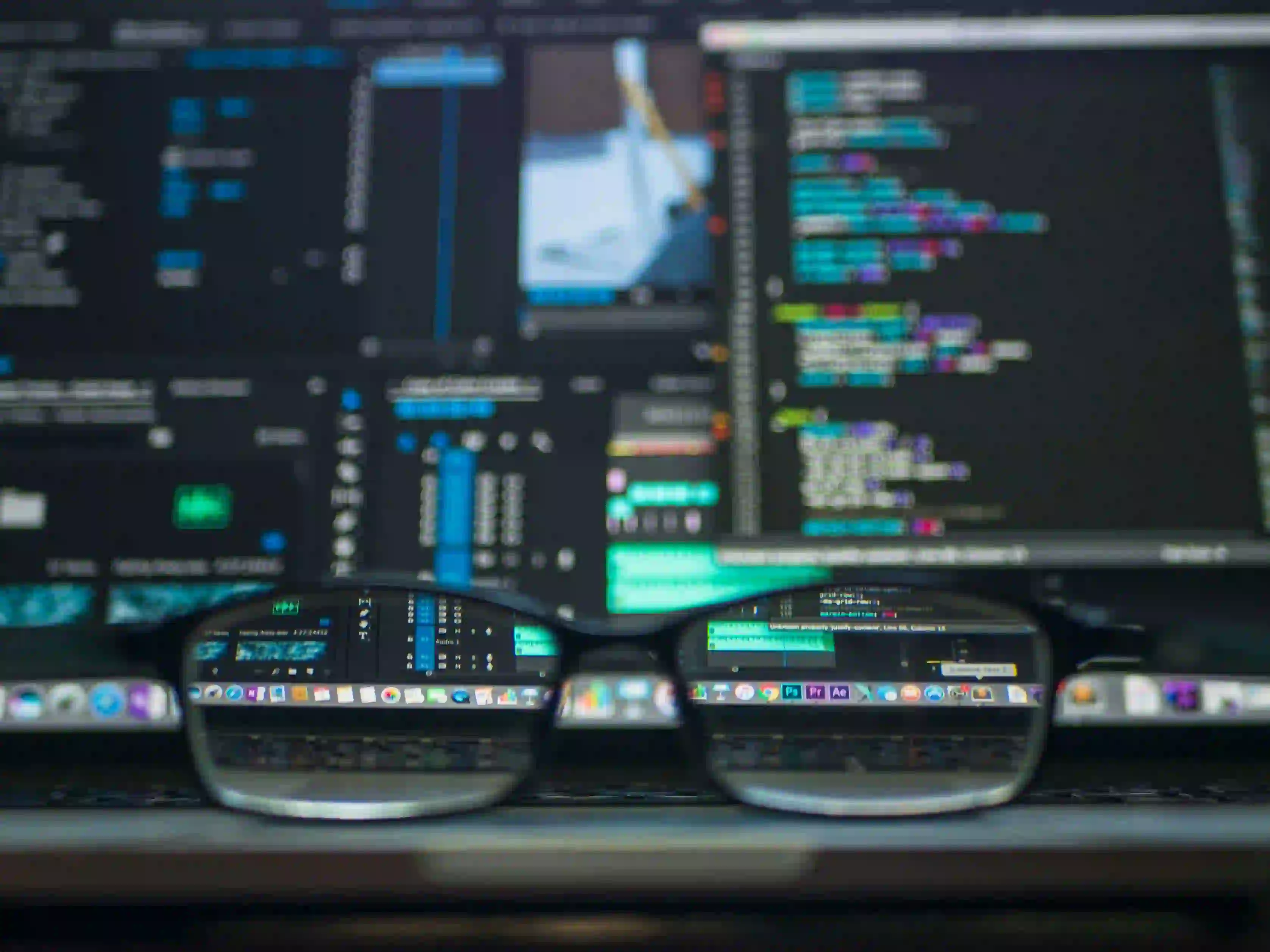
Maximize UDP Efficiency: Common Aeron Messaging Pitfalls
In the fast-paced world of high-performance messaging systems, UDP plays a crucial role. With its low-latency characteristics, it's ideal for real-time applications. This blog post focuses on Aeron, a popular messaging system that leverages UDP, and explores common pitfalls in Aeron messaging. By understanding these pitfalls, you can maximize inefficiencies and ensure your applications run smoothly.
Understanding Aeron and its Architecture
Aeron is a high-throughput messaging system designed for low-latency communication. Its architecture is optimized for modern hardware and network environments. One of the standout features of Aeron is its ability to utilize UDP’s inherent advantages while smoothing out some of its limitations.
Aeron uses a publish-subscribe model, allowing multiple consumers to read messages from a single stream without blocking the sender. This architecture is built on the concept of channels and streams, where:
- Channel: Represents the means by which data is sent and received.
- Stream: A specific flow of messages identified by a unique identifier.
For further reading on the technical aspects of Aeron, you can check the Aeron GitHub documentation.
Common Messaging Pitfalls with Aeron
Though robust, Aeron is subject to specific pitfalls that, if overlooked, can degrade the performance of your applications. Below are some common issues and their solutions.
1. Ignoring Default Configuration Settings
Aeron provides various customizable settings, but many developers stick with the defaults. While these defaults may suit basic needs, they don't always foster optimal performance.
For instance, consider the following default configuration:
Aeron aeron = Aeron.connect(new Aeron.Context());
If you do not modify the context settings, you might miss opportunities like optimizing the termBufferLength
, which affects how much data can be buffered per term.
Solution: Adjust configuration settings based on your application’s requirements. For example:
Aeron.Context context = new Aeron.Context();
context.termBufferLength(16 * 1024 * 1024); // Set term buffer to 16 MB
Aeron aeron = Aeron.connect(context);
2. Failing to Handle Message Loss
UDP's nature means it does not guarantee message delivery, leading to potential message loss. Aeron implements mechanisms to mitigate this using retransmission requests, but developers often overlook explicit handling.
While Aeron offers built-in mechanisms to recover from message loss, not responding to Nak
messages can lead to significant data gaps.
Solution: Implementing an acknowledgment mechanism is crucial. For example:
aeron.subscribe((message, header) -> {
if (header.isNak()) {
// Handle Nak message, retrigger sending logic
resendMessage();
}
});
This monitoring can help you recognize when messages might not be received and take appropriate action quickly.
3. Skimming Over Flow Control
Flow control ensures that a fast producer does not overwhelm a slower consumer. Aeron provides a flow control mechanism, but developers often assume it operates perfectly without any tuning.
When the publisher sends messages faster than the subscriber can consume them, the impact can lead to increased memory usage and possible system crashes.
Solution: Adjust flow control settings based on observed latency and throughput. Use Aeron's built-in control:
Publication publication = aeron.addPublication("my-channel", streamId);
FlowControl flowControl = new NakFlowControl();
while (isActive) {
long result = publication.offer(buffer);
if (result < 0) {
flowControl.onFailedSend(publication);
// Handle the flow control here
}
}
4. Neglecting the Health of Your Network
A common misconception is that UDP packets travel flawlessly across networks. However, network conditions fluctuate, affecting the delivery of messages. Ignoring these aspects can lead developers to underestimate necessary checks and balances.
To monitor network health, you can implement metrics that assess packet loss, delay, and jitter.
Solution: Incorporate network monitoring in your application. An example could be:
private void monitorNetworkHealth() {
int packetsLost = calculatePacketLoss();
if (packetsLost > threshold) {
log.warning("Packet loss exceeds threshold: " + packetsLost);
// Adjust parameters or alert the system administrators
}
}
5. Inefficient Message Serialization
Serialization is necessary when you convert an object to a format suitable for transmission. However, developers often overlook the importance of efficient serialization techniques, which can slow down the system considerably.
Using inefficient serialization could result in larger message sizes and slow processing times.
Solution: Optimize serialization. Using frameworks like Protocol Buffers or Jackson can improve performance. For example:
MyMessage message = MyMessage.newBuilder()
.setField("data")
.build();
byte[] serialized = message.toByteArray(); // Efficiently serialize
publication.offer(serialized);
6. Overcomplicating the Code Structure
In an effort to implement robust systems, developers sometimes add complexity that hinders performance. Overcomplication can lead to maintenance challenges and hard-to-track performance bottlenecks.
A clear, concise code structure supports better performance and easier debugging.
Solution: Keep your design simple. For example, use streams effectively:
try (Aeron aeron = Aeron.connect(new Aeron.Context())) {
// Using try-with-resources to ensure proper closure
Publication publication = aeron.addPublication("my-channel", streamId);
// Simple sending logic
}
In Conclusion, Here is What Matters
Aeron over UDP is a powerful combination for high-performance messaging systems. However, failing to address the common pitfalls described above may cost you in performance, reliability, and maintainability. By being aware of these issues and implementing the provided solutions, you can significantly enhance the efficiency of your UDP-based messaging application.
To explore more about message handling and performance optimizations, consider reviewing the Aeron Wiki.
Remember, optimizing performance in distributed systems is a continuous process. Stay informed and adaptable to ensure your application's success in the evolving landscape of technology.
I hope this blog post serves as a useful guide as you navigate the nuances of maximizing efficiency with Aeron messaging over UDP. Happy coding!