Common Messaging Pitfalls in Java: How to Avoid Them
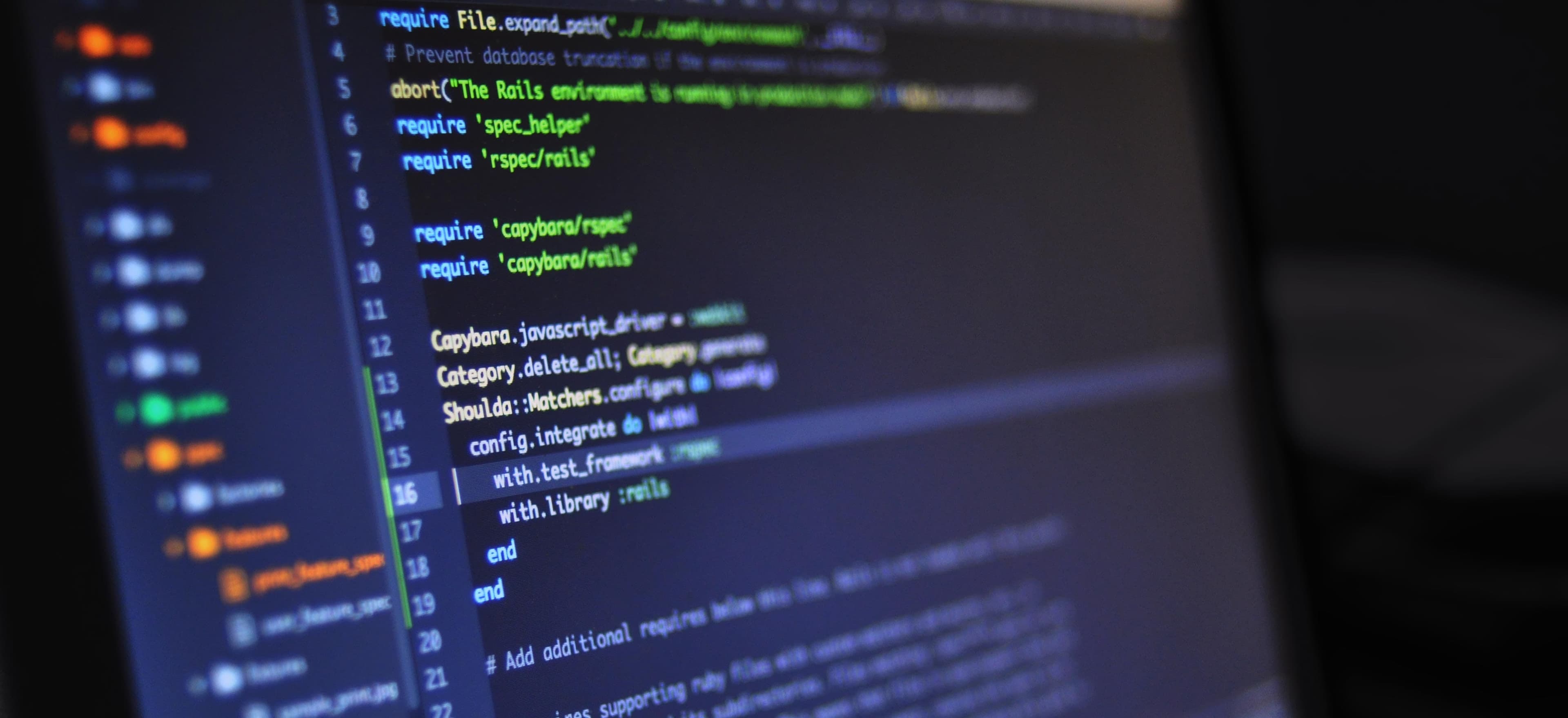
- Published on
Common Messaging Pitfalls in Java: How to Avoid Them
Messaging is a critical aspect of Java applications, particularly in distributed systems. Java developers often rely on various messaging patterns to facilitate communication between different components or services. However, there are common pitfalls that can lead to inefficiencies, bugs, and even application failures. In this blog post, we will explore these pitfalls and provide practical solutions to avoid them.
Table of Contents
- Understanding Messaging in Java
- Pitfall 1: Ignoring Message Serialization
- Pitfall 2: Overlooking Message Acknowledgment
- Pitfall 3: Inefficient Message Queuing
- Pitfall 4: Not Handling Message Failures
- Pitfall 5: Neglecting Security Concerns
- Conclusion
Understanding Messaging in Java
Messaging in Java typically involves sending and receiving messages between applications or components. This is often done using message-oriented middleware (MOM) systems such as Apache Kafka, RabbitMQ, or ActiveMQ. These systems enable asynchronous communication, which is vital for scalability and decoupling.
While the benefits are substantial, developers must be mindful of potential pitfalls. Recognizing and addressing these issues can save valuable time and resources.
Pitfall 1: Ignoring Message Serialization
One common pitfall when working with messaging in Java is neglecting the importance of message serialization and deserialization. In essence, serialization is the process of converting an object into a byte stream for transmission, while deserialization is the reverse process.
Example of Serialization
import java.io.*;
public class User implements Serializable {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and toString()
}
In this example, we're creating a User
class that implements Serializable
. This is essential because Java's messaging systems require the ability to transform objects into a format suitable for transmission.
Why This Matters
If you ignore proper serialization, you risk losing data integrity. Deserializing objects in a different format or with a different version can lead to NotSerializableException
.
Solution
Always ensure that your messages are properly serialized using standard serialization libraries like Java's built-in Serializable
, or leverage frameworks like Jackson for JSON-based communication or Protobuf for binary serialization.
Pitfall 2: Overlooking Message Acknowledgment
Message acknowledgment is another area where developers can stumble. When a message is sent to a queue, it is crucial to acknowledge that the message has been received and processed. Without acknowledgment, messages may be lost or duplicated.
Example of Acknowledgment
import javax.jms.*;
public class MessageConsumerExample {
public void consumeMessages(Session session, MessageConsumer consumer) throws JMSException {
while (true) {
Message message = consumer.receive();
if (message instanceof TextMessage) {
TextMessage textMessage = (TextMessage) message;
System.out.println("Received: " + textMessage.getText());
consumer.acknowledge();
}
}
}
}
Why This Matters
If you fail to acknowledge messages appropriately, the message will remain in the queue indefinitely, potentially clogging your system and causing delays. Additionally, you might face issues with duplicate processing if the consumer retries.
Solution
Use the proper acknowledgment modes provided by the messaging framework being used, such as client acknowledgment for explicit handling, or transactions for ensuring message delivery guarantees.
Pitfall 3: Inefficient Message Queuing
Another pitfall is creating inefficient message queues. This frequently occurs when developers do not consider the message volume or the performance characteristics of their messaging system.
Best Practices
- Set an Appropriate Message Expiration: Unprocessed messages that linger too long can clog your system. Always set an expiration time suitable to your use case.
- Limit the Queue Size: Most messaging systems allow you to impose a limit on the queue size to prevent excessive resource consumption.
Why This Matters
If your queues become too congested, it can lead to increased latency and resource exhaustion. This could eventually compromise your application’s performance.
Solution
Utilize monitoring tools to keep track of message throughput and queue size. Libraries like Spring Cloud Stream provide abstractions that can make this easier to handle.
Pitfall 4: Not Handling Message Failures
Neglecting to handle message failures appropriately is a significant oversight. This can manifest as unhandled exceptions leading to system crashes or lost messages.
Example of Error Handling
public void consumeData() {
try {
// Receiving logic here
} catch (Exception e) {
System.err.println("Failed to process message: " + e.getMessage());
// Implement a retry mechanism or log the error
}
}
Why This Matters
Failing to incorporate robust error handling can lead to critical failures in your application, resulting in loss of data and a poor user experience.
Solution
Implement fallback mechanisms, such as dead-letter queues for storing messages that just can't be processed. Use libraries such as Resilience4j for handling retries effectively.
Pitfall 5: Neglecting Security Concerns
In the age of cybersecurity threats, neglecting the security of your messaging systems can expose your application to vulnerabilities. Unsanctioned access to messages could lead to sensitive information leaks or unwarranted data manipulation.
Best Practices
- Use TLS/SSL: Always encrypt your messages in transit using secure transport protocols.
- Authenticate Users: Employ authentication mechanisms to restrict access to your messaging systems.
Why This Matters
By overlooking security, you create a potential entry point for attackers. With increasing GDPR and data protection regulations, secure messaging is not just best practice, but often a legal obligation.
Solution
Leverage existing security protocols within your messaging service, and consider educational resources like the OWASP Messaging Security Cheat Sheet.
Final Thoughts
Java messaging is a powerful tool for developing scalable, distributed applications. However, it's essential to stay vigilant against common pitfalls that can undermine those benefits. By understanding serialization, acknowledgment, queuing efficiency, failure handling, and security, you can significantly improve the reliability and performance of your Java messaging systems.
With these insights, you are now equipped to build a robust messaging architecture. Happy coding!
Additional Resources
Feel free to explore these resources for deeper insights into enhancing your Java messaging proficiency!